(1) Express routing
1, Concept of routing
1. What is routing
2. Real life routing
3. Routing in Express
4. Examples of routing in Express
5. Route matching process
II. Use of routing
1. Simplest usage (rarely used)
const express = require('express') const app = express() // Mount routing app.get('/' , (req,res )=> { res.send('hello world.') }) app.post('/' , (req , res) => { res.send('Post Request') }) app.listen(8080 , ()=> { console.log('http://127.0.0.1:8080'); })
2. Modular routing
3. Create routing module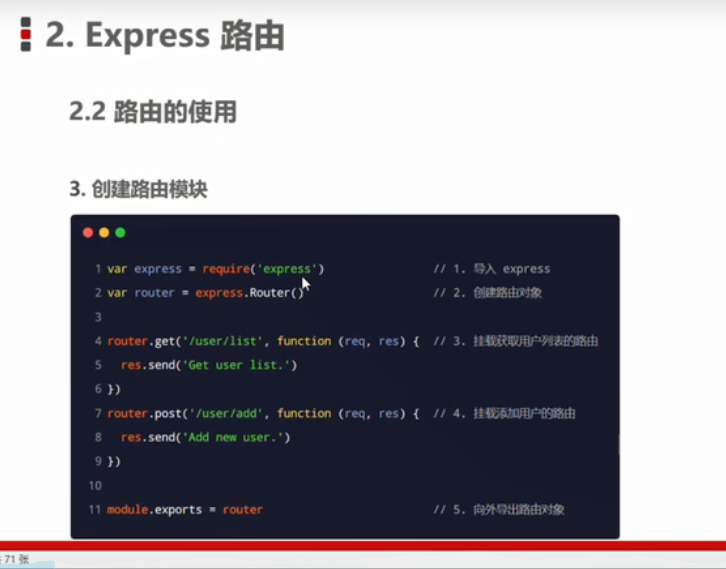
// This is the routing module // 1. Import express var express = require('express') // 2. Create routing object var router = express.Router() // 3. Mount specific routes router.get('/user/list' , (req,res)=> { res.send('Get user list') }) router.post('/user/add' , (req,res) => { res.send('Add new user') }) // 4. Export routing objects module.exports = router
4. Register routing module
var express = require('express') var app = express() // app.use(express.static('./files')) // 1. Import routing module var router = require('./03-router') // 2. Register routing module app.use(router) // Note: app The use () function is used to register the global middleware app.listen(8080 , (req,res)=> { console.log('http://127.0.0.1:8080'); })
5. Prefix routing module
(II) Express Middleware
1, Concept of Middleware
1. What is middleware
2. Examples in real life
3. Call process of Express Middleware
4. Format of Express Middleware
5. Function of next function
2, First experience of Express Middleware
1. Define middleware functions
var express = require('express') var app = express() // Define a box of the simplest middleware functions var mw = function(req, res , next){ console.log('This is the simplest middleware function'); // Transfer the flow relationship to the next middleware or routing next() } app.listen(8080 , (req,res) => { console.log('http://127.0.0.1:8080'); })
2. Globally effective Middleware
var express = require('express') var app = express() // Define a box of the simplest middleware functions var mw = function(req, res , next){ console.log('This is the simplest middleware function'); // Transfer the flow relationship to the next middleware or routing next() } // Register mw as a globally effective Middleware app.use(mw) app.get('/' , (req,res) => { console.log('Called / This route'); res.send('Home Page') }) app.post('/user' , (req,res) => { console.log('Called/user This route'); res.send('User Page') }) app.listen(8080 , (req,res) => { console.log('http://127.0.0.1:8080'); })
3. Define the simplified form of global middleware
var express = require('express') var app = express() // Define a box of the simplest middleware functions // var mw = function(req, res , next){ // console.log('This is the simplest middleware function '); // //Transfer the flow relationship to the next middleware or routing // next() // } // //Register mw as a globally effective Middleware // app.use(mw) // This is a simplified form of defining global middleware app.use(function(rew,res , next){ console.log('This is the simplest middleware function'); next() }) app.get('/' , (req,res) => { console.log('Called / This route'); res.send('Home Page') }) app.post('/user' , (req,res) => { console.log('Called/user This route'); res.send('User Page') }) app.listen(8080 , (req,res) => { console.log('http://127.0.0.1:8080'); })
4. Role of Middleware
var express = require('express') const req = require('express/lib/request') var app = express() // This is a simplified form of defining global middleware app.use(function(rew,res , next){ // Gets the time when the request arrived at the server const time = Date.now() // Mount custom attributes for req objects to share time with all subsequent routes req.StartTime = time next() }) app.get('/' , (req,res) => { res.send('Home Page' +req.StartTime ) }) app.post('/user' , (req,res) => { res.send('User Page' +req.StartTime ) }) app.listen(8080 , (req,res) => { console.log('http://127.0.0.1:8080'); })
5. Define multiple global Middleware
const express = require('express') const app = express() // Define two Middleware in succession // Define the first global Middleware app.use((req,res , next) => { console.log('The first global middleware was called'); next() }) // Define the second global Middleware app.use((req,res , next) => { console.log('The second global middleware is called'); next() }) // Define a route app.get('/user' , (req,res) => { res.send('User Page') }) app.listen(8080 , (req,res) => { console.log('http://127.0.0.1:8080'); })
6. Partially effective Middleware
// Import express module const express = require('express') // Create a server instance of express const app = express() // 1. Define middleware functions const mw1 = (req,res ,next) => { console.log('Partial effective middleware was called'); } // 2. Create route app.get('/' ,mw1, (req,res) => { res.send('Home Page') }) app.post('/user' , (req,res) => { res.send('User Page') }) // Call app Listen method, specify the port number and start the web service app.listen(8080 , function(){ console.log('Express server running at http://127.0.0.1:8080'); })
7. Define multiple local Middleware
8. Understand the five precautions for using middleware
3, Classification of Middleware
1. Application level Middleware
2. Routing level Middleware
3. Error level Middleware
// Import express module const express = require('express') // Create a server instance of express const app = express() // 2. Create a route app.get('/', (req, res) => { // 1.1 human manufacturing errors throw new Error('An error occurred inside the server!') res.send('Home page.') }) // 2. Define the middleware with error level to capture the abnormal errors of the whole project, so as to prevent the collapse of the program app.use((err , req ,res ,next) => { console.log('An error has occurred!' + err.message); res.send('Error' +err.message) }) // Call app Listen method, specify the port number and start the web server app.listen(8080, function () { console.log('Express server running at http://127.0.0.1:8080') })
4. Express built-in Middleware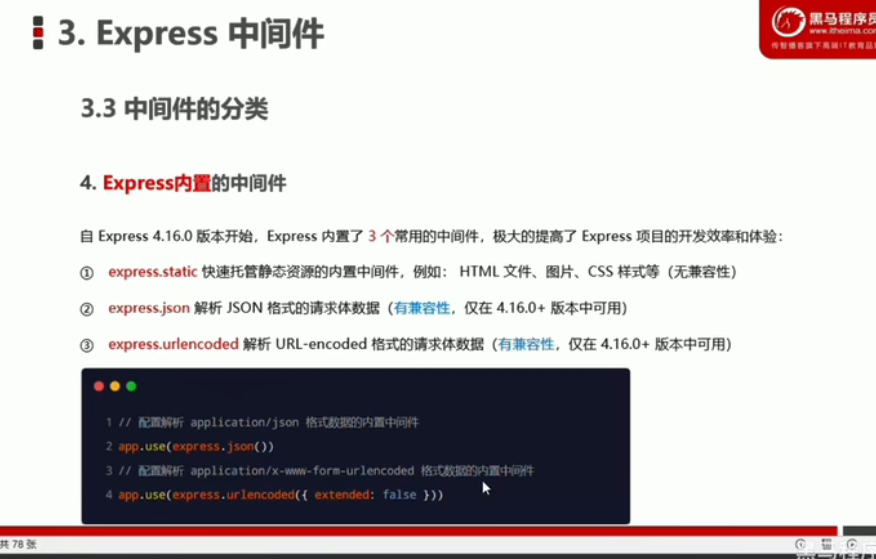
// Import express module const express = require('express') // Create a server instance of express const app = express() // In addition to the error level middleware, other levels of middleware must be configured before routing // Via express JSON () is a middleware that parses the data in JSON format in the form app.use(express.json()) // Via express Urlencoded () is a middleware to parse the data in URL encoded format in the form app.use(express.urlencoded({extended: false})) // Define route app.post('/user' , (req,res) => { // In the server, you can use req The body attribute is used to receive the request body data sent by the client // By default, if the middleware for parsing form data is not configured, req Body is equal to undefined by default console.log(req.body); res.send('User Page') }) app.post('/book' , (req,res) => { // On the server side, you can use req Body to obtain form data in JSON format and data in URL encoded format console.log(req.body ); res.send('ok') }) // Call app Listen method, specify the port number and start the web server app.listen(8080, function () { console.log('Express server running at http://127.0.0.1:8080') }) // Call app Listen method, specify the port number and start the web server app.listen(8080, function () { console.log('Express server running at http://127.0.0.1:8080') })
5. Third party Middleware
IV. customized Middleware
1. Requirements description and implementation steps
2. Define Middleware
3. Listen for data events of req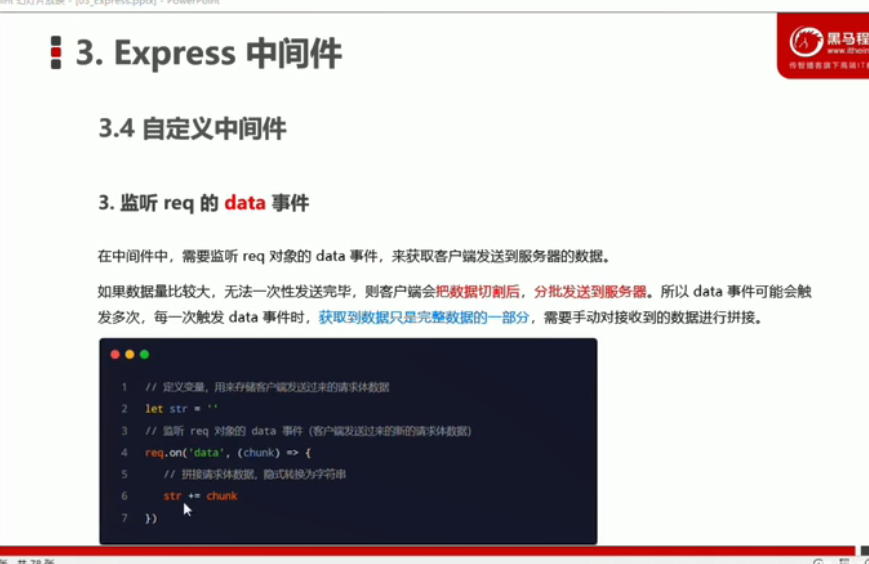
4. Listen for the end event of req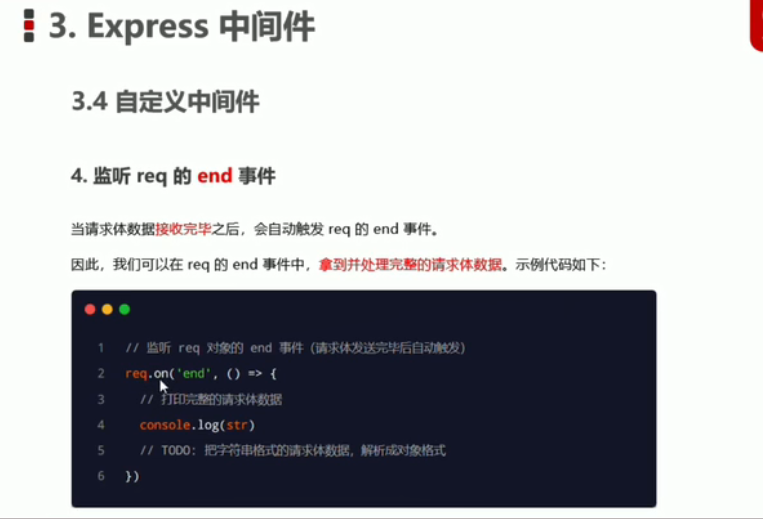
5. Use the querystring module to parse the request body data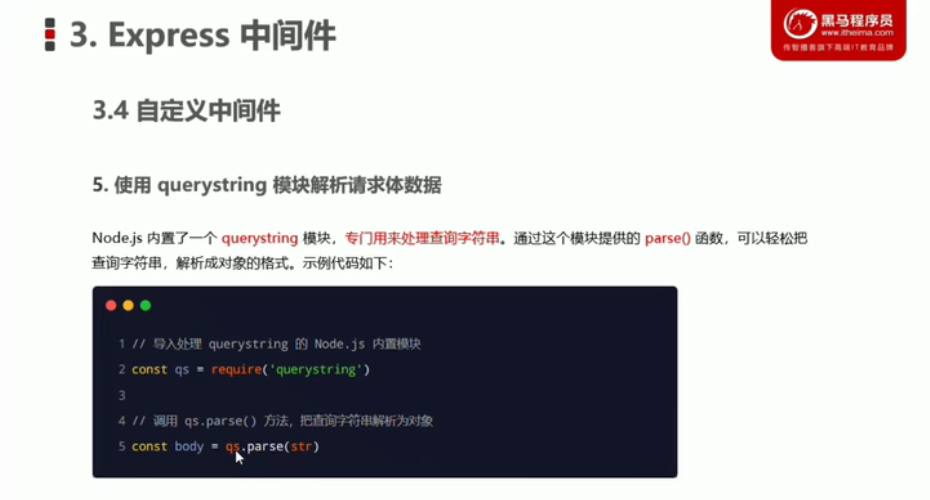
6. Resolve the mounted object as req body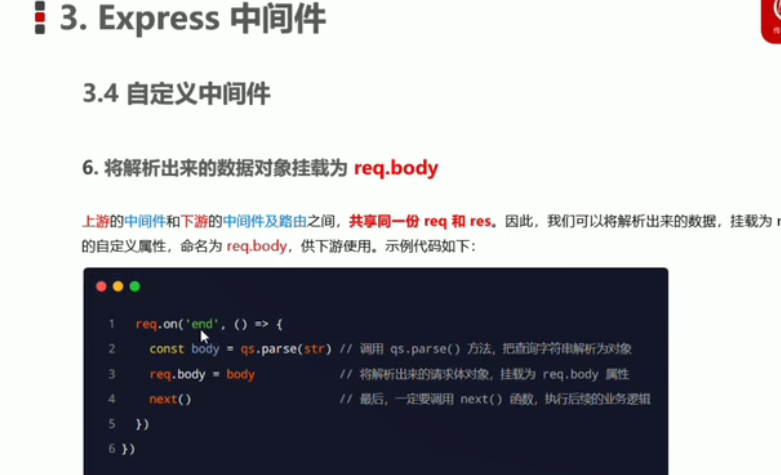
7. Encapsulate custom middleware into modules
// Import node JS built-in querystring module const qs = require('querystring') const bodyParser = (req, res ,next) => { // Define the specific business logic of Middleware // 1. Define a str string, which is specially used to store the request body data sent by the client let str = '' // 2. Listen to the data event of req req.on('data' , (chunk) => { str = chunk }) // 3. Listen to the end event of req req.on('end' , () => { // The complete request body data is stored in str console.log(str); // TODO: parse the request body data in string format into object format const body =qs.parse(str) // console.log(body); req.body = body next() }) } module.exports = bodyParser
// Import express module const { log } = require('console') const express = require('express') // Create a server instance of express const app = express() // This is the middleware for parsing form data // 1. Import the self encapsulated middleware module const customBodyParser = require('./14-custom-body-parser') // 2. Register the customized middleware functions as globally available middleware app.use( customBodyParser) // Define route app.post('/user' , (req,res) => { res.send(req.body) }) // Call app Listen method, specify the port number and start the web server app.listen(8080, function () { console.log('Express server running at http://127.0.0.1:8080') })
(3) Using Express write interface
1, Create basic server
II. Create API routing module
III. write GET interface
IV. Write POST interface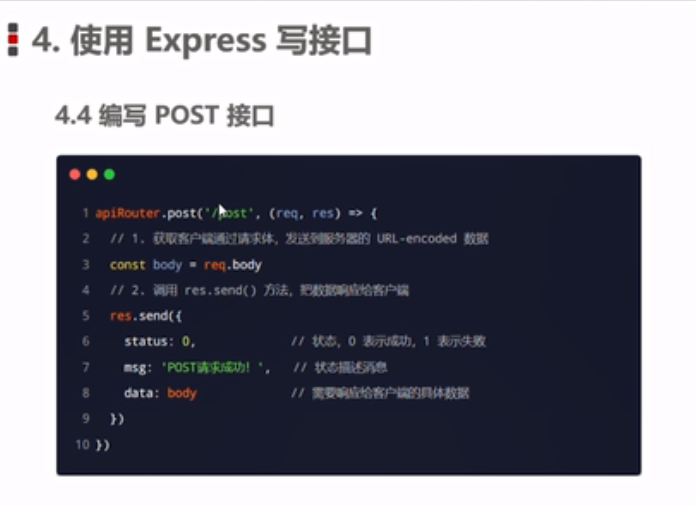
const express = require('express') const router = express.Router() // Mount the corresponding route here router.get('/get' ,(req,res) => { // Through req Query gets the data sent to the server by the client through the query string const query = req.query // Call the res.send () method to respond to the processing results to the client res.send({ status : 0, // 0: indicates successful processing, 1: indicates failed processing msg : 'GET Request succeeded' , //Description of status data : query //Data required to respond to the client }) }) // Define POST interface router.post('/post' , (req,res) => { // Get the data in URL encoded format contained in the request body through req.body const body = req.body // Call the res.send () method to respond to the result to the client res.send({ status : 0 , msg : 'POST Request succeeded' , data :body }) }) module.exports = router
// Import express module const e = require('express') const express = require('express') // Create a server instance of express const app = express() // Configure middleware for parsing form data app.use(express.urlencoded({extended:false})) // Import routing module const router = require('./16-apiRouter') // Register the routing module on the app app.use('/api' , router) // Call app Listen method, specify the port number and start the web server app.listen(8080, function () { console.log('Express server running at http://127.0.0.1:8080') })
V. cross domain resource sharing of CORS
1. Cross domain problem of interface
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <script src="https://cdn.staticfile.org/jquery/3.4.1/jquery.min.js"></script> </head> <body> <button id="get">GET</button> <button id="post">POST</button> <script> $(function(){ // 1. Test GET interface $('#get').on('click ' , function(){ $.ajax({ type : 'GET' , url : 'http://127.0.0.1:8080/api/get' , data : {name : 'zs' , age: 30} , success: function(res) { console.log(res); } }) }) // 2. Test the POST interface $('#post').on('click ' , function(){ $.ajax({ type : 'POST' , url : 'http://127.0.0.1:8080/api/post' , data : {bookname : 'Water Margin' , author: 'Shi Naian'} , success: function(res) { console.log(res); } }) }) }) </script> </body> </html>
2. Use cors middleware to solve cross domain problems
// Import express module const e = require('express') const express = require('express') // Create a server instance of express const app = express() // Configure middleware for parsing form data app.use(express.urlencoded({extended:false})) // We must configure cors middleware before routing, so as to solve the problem of cross domain interface const cors = require('cors') app.use(cors()) // Import routing module const router = require('./16-apiRouter') // Register the routing module on the app app.use('/api' , router) // Call app Listen method, specify the port number and start the web server app.listen(8080, function () { console.log('Express server running at http://127.0.0.1:8080') })
3. What is cors
4. Precautions for cors
5. CORS response header - access control - allow origin
6. CORS response header - access control - allow headers
7. CORS response header - access control - allow methods
8. Classification of CORS requests
9. Simple request
10. Pre inspection request
11. Difference between simple request and pre inspection request
Vi. JSONP interface
1. Review the concept and characteristics of JSONP
2. Precautions for creating JSONP interface
3. Steps of implementing JSONP interface
4. Specific code for implementing JSONP interface
Use of onjquery requests in web pages
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <script src="https://cdn.staticfile.org/jquery/3.4.1/jquery.min.js"></script> </head> <body> <button id="get">GET</button> <button id="post">POST</button> <button id="Delete">Delete</button> <button id="JSONP">JSONP</button> <script> $(function(){ // 1. Test GET interface $('#get').on('click ' , function(){ $.ajax({ type : 'GET' , url : 'http://127.0.0.1:8080/api/get' , data : {name : 'zs' , age: 30} , success: function(res) { console.log(res); } }) }) // 2. Test the POST interface $('#post').on('click ' , function(){ $.ajax({ type : 'POST' , url : 'http://127.0.0.1:8080/api/post' , data : {bookname : 'Water Margin' , author: 'Shi Naian'} , success: function(res) { console.log(res); } }) }) // 3. Bind click event handling function for delete button $('#Delete').on('click' , function(){ $.ajax({ type: 'DELETE' , url: 'http://127.0.0..1:8080/api/delete' , success: function(res){ console.log(res); } }) }) // 4. Bind the click event handling function for the JSONP button $('#JSONP').on('click' , function(){ $.ajax({ type : 'GET' , url :'http://127.0.0.1:8080/api/jsonp', dataType: 'jsonp' , success:function(res){ console.log(res); } }) }) }) </script> </body> </html>
// Import express module const e = require('express') const express = require('express') // Create a server instance of express const app = express() // Configure middleware for parsing form data app.use(express.urlencoded({extended:false})) // The interface of JSONP must be configured before configuring CORS middleware app.get('/api/jsonp' , (req,res) => { // TODO: define the specific implementation interface of JSONP interface // 1. Get the name of the function const funcName =req.query.callback // 2. Define the data object to be sent to the client const data = {name : 'zs ' , age : 20} // 3. Splice a function call const scriptStr = `${funcName}(${JSON.stringify(data)})` // 4. Respond the spliced string to the client res.send(scriptStr) }) // We must configure cors middleware before routing, so as to solve the problem of cross domain interface const cors = require('cors') app.use(cors()) // Import routing module const router = require('./16-apiRouter') // Register the routing module on the app app.use('/api' , router) // Call app Listen method, specify the port number and start the web server app.listen(8080, function () { console.log('Express server running at http://127.0.0.1:8080') })