This article uses Tencent cloud Face recognition Ability to quickly realize face search, which can be applied to business scenarios that need to match faces.
1. Create API key: https://console.cloud.tencent.com/cam/capi , the key is the only credential, please keep it properly

2. Log in to the face recognition console and create a new face database in the face database with a meaningful name

3. Pass in the photos to be tested in the personnel library
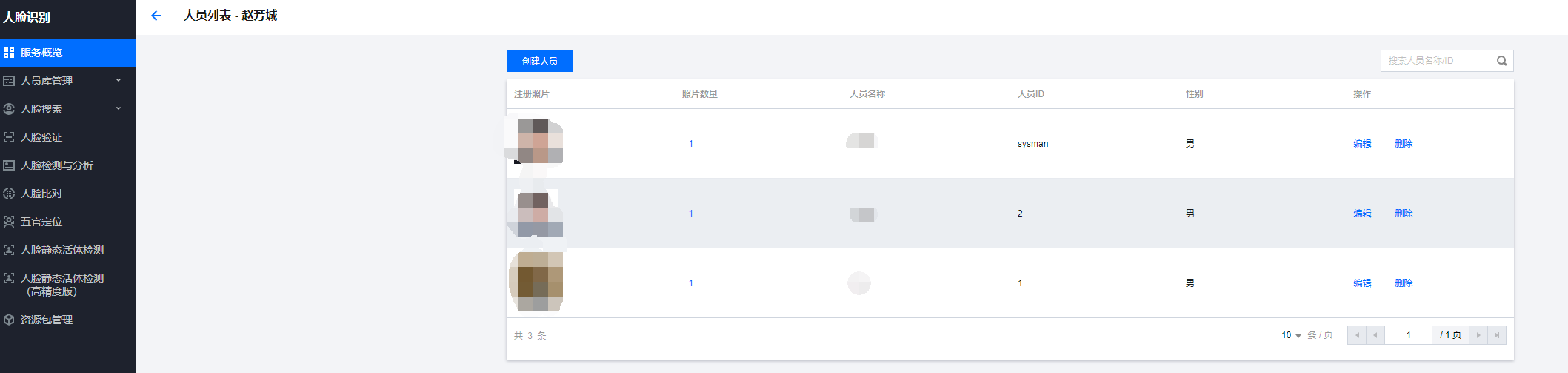
Among them, the person id can be entered into the person id of the business system. For example, here I enter the person primary key of the business system to facilitate the rapid combination with the business after the person is identified.
If you need to import personnel library information in batches, you need to process it through the interface. This function is not provided on the console for the time being,
4. Add Tencent cloud dependency to maven dependency
<dependency> <groupId>com.tencentcloudapi</groupId> <artifactId>tencentcloud-sdk-java</artifactId> <!-- go to https://search.maven.org/search?q=tencentcloud-sdk-java and get the latest version. --> <!-- succeed in inviting sb. https://search. maven. org/search? Q = tencentcloud SDK Java query all versions. The latest version is as follows -- > <version>3.1.322</version> </dependency>
5. Create a new method in the local code and prepare a photo to be tested. Add the following methods:
Prepare a picture and convert it to base64 format,
import com.tencentcloudapi.common.Credential; import com.tencentcloudapi.common.profile.ClientProfile; import com.tencentcloudapi.common.profile.HttpProfile; import com.tencentcloudapi.common.exception.TencentCloudSDKException; import com.tencentcloudapi.iai.v20200303.IaiClient; import com.tencentcloudapi.iai.v20200303.models.*; public class SearchPersons{ public static void main(String [] args) { try{ // To instantiate an authentication object, you need to pass in the secret ID and secret key of Tencent cloud account. Here, you also need to pay attention to the confidentiality of the key pair // Key can go to https://console.cloud.tencent.com/cam/capi Refer to step 1 of this article for the website Credential cred = new Credential("SecretId", "SecretKey"); // Instantiate an http option, which is optional. There are no special requirements to skip HttpProfile httpProfile = new HttpProfile(); httpProfile.setEndpoint("iai.tencentcloudapi.com"); // Instantiate a client option, which is optional. There are no special requirements to skip ClientProfile clientProfile = new ClientProfile(); clientProfile.setHttpProfile(httpProfile); // Instantiate the client object to request the product, and the clientProfile is optional IaiClient client = new IaiClient(cred, "ap-beijing", clientProfile); // Instantiate a request object, and each interface will correspond to a request object SearchPersonsRequest req = new SearchPersonsRequest(); String[] groupIds1 = {"zfc-face"}; req.setGroupIds(groupIds1); //Pictures to be tested req.setImage("data:image/jpg;base64,/9j/4A"); // The returned resp is an instance of SearchPersonsResponse, which corresponds to the request object SearchPersonsResponse resp = client.SearchPersons(req); // Output json format string back package System.out.println(SearchPersonsResponse.toJsonString(resp)); Result[] result=resp.getResults(); for (int i=0;i<result.length;i++){ Candidate candidates[]= result[i].getCandidates(); Candidate person=candidates[0]; Float compare=person.getScore(); //According to the matching result, the threshold of accuracy is judged according to the size of face database if(compare>80f){ person.getPersonId(); System.out.println(person.getPersonId()); }else { //return null; System.out.println("Unrecognized"); } } } catch (TencentCloudSDKException e) { System.out.println(e.toString()); } } }
The returned results are as follows, where the Score node is the matching Score.
{ "Results": [{ "Candidates": [{ "PersonId": "sysman", "FaceId": "", "Score": 100.0 }, { "PersonId": "2", "FaceId": "", "Score": 94.44817 }, { "PersonId": "1", "FaceId": "", "Score": 4.404487 }], "FaceRect": { "X": 84, "Y": 112, "Width": 206, "Height": 251 }, "RetCode": 0 }], "PersonNum": 3, "FaceModelVersion": "3.0", "RequestId": "1fa8e80b-ca8e-40be-92b2-7971b771f73c" }
For the accuracy of matching results, you can refer to it Official description
What is the recommended threshold for face search?
- Under the 10000 size face base database, the corresponding score of 1% of the error recognition rate is 70 points, the corresponding score of 1 / 1000 of the error recognition rate is 80 points, and the corresponding score of 1 / 10000 of the error recognition rate is 90 points.
- Under the 100000 size face base database, the corresponding score of one percent of the error recognition rate is 80 points, the corresponding score of one thousandth of the error recognition rate is 90 points, and the corresponding score of one thousandth of the error recognition rate is 100 points.
- Under the 300000 size face base database, the corresponding score of one percent of the error recognition rate is 85 points, and the corresponding score of one thousandth of the error recognition rate is 95 points.
- Generally, about 80 points can be applied to most scenes. It is recommended that the score should not exceed 90 points. You can choose the appropriate score according to the actual situation.