Form form submission has two methods: get and post. When submitting to jsp, the way to deal with Chinese scrambling is different.
1. Handling post method to submit Chinese scrambling code
When submitting by post method, there is really no special method, just pay attention to the coding format of html pages and jsp pages. It can be GBK or UTF-8.
As shown in the figure:
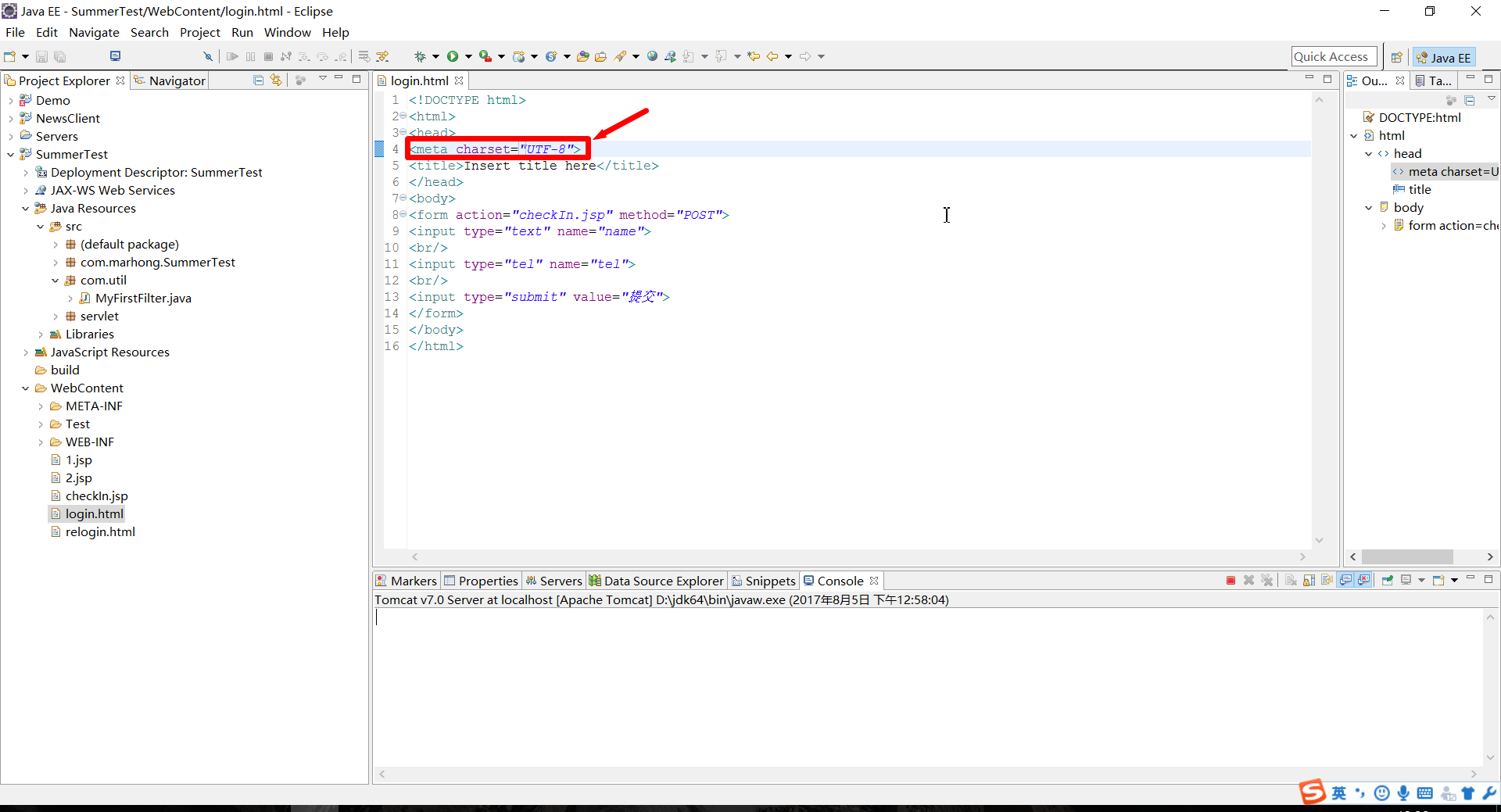
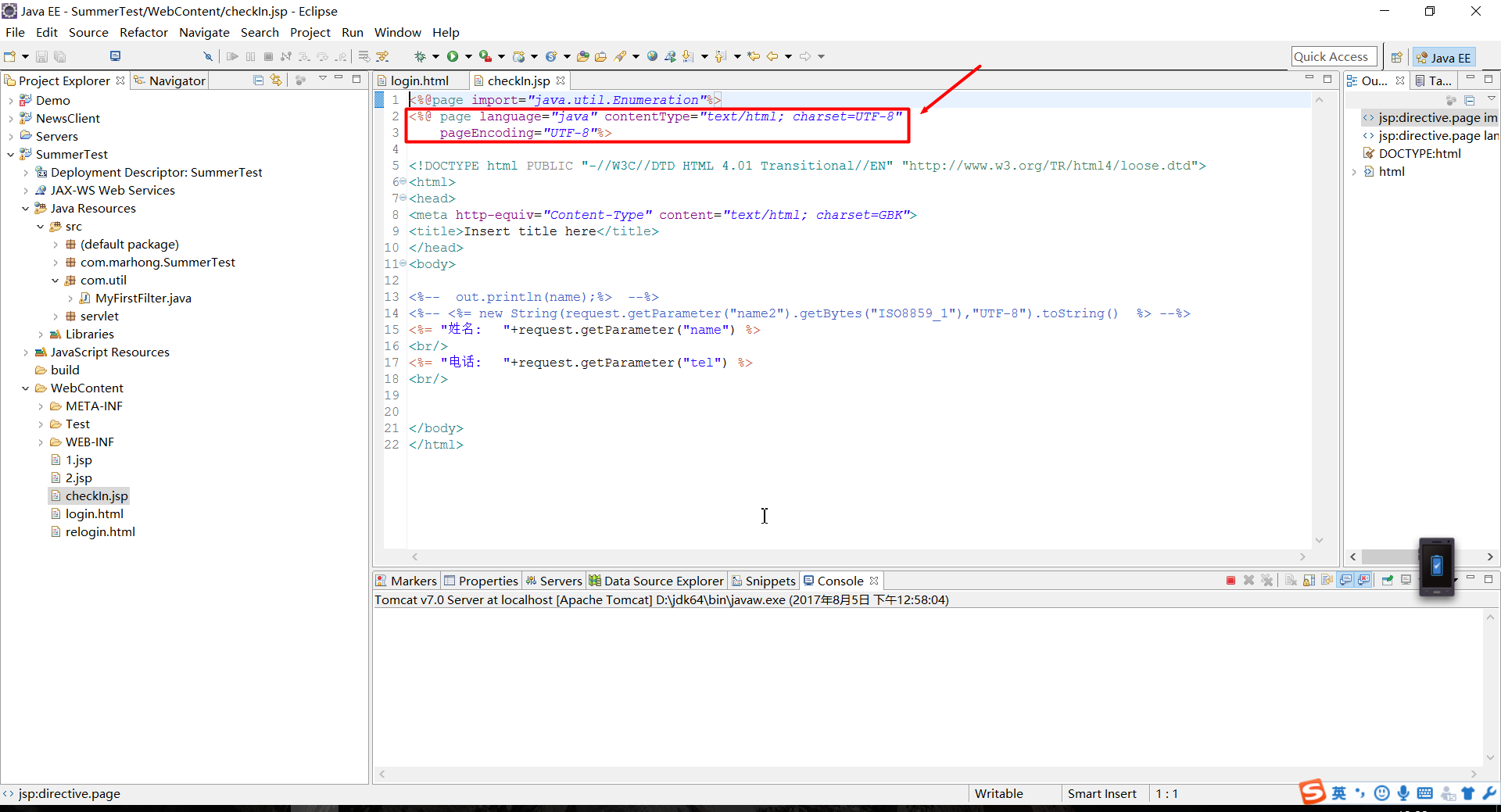
2. Handling get method to submit Chinese scrambling code
If you use the get method to submit, even if your html page and jsp page coding format are consistent, there will be chaotic code, as to why this is not in-depth understanding. All in all, here is the solution.
Method 1:
String value = request.getParameter("parameterName");
value = new String(value.getBytes("ISO859_1"),"UTF-8");
This can solve the scrambling problem, but obviously it's very troublesome. The second method is recommended.
Method 2:
Use filters. For a web application, a filter is a component in a web container that filters requests and responses for specific requests. When a request arrives, the web container will determine whether a filter is associated with the information resource, if so, it will be handed over to the filter, then to the target resource, and then to the filter in the opposite order in response, and finally back to the user's browser.
Following is a concrete example of how to solve scrambling:
MyFirstFilter.java:
package com.util;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletRequestWrapper;
import javax.servlet.http.HttpServletResponse;
import org.apache.catalina.connector.Request;
/**
* Servlet Filter implementation class MyFirstFilter
*/
@WebFilter("/MyFirstFilter")
public class MyFirstFilter implements Filter {
class MyRequest extends HttpServletRequestWrapper{
public MyRequest(HttpServletRequest request) {
super(request);
// TODO Auto-generated constructor stub
}
@Override
public String getParameter(String name) {
// TODO Auto-generated method stub
String value =null;
try {
value = super.getParameter(name);
if(super.getMethod().equalsIgnoreCase("GET")){
if(value != null){
value = new String(value.getBytes("ISO8859_1"), "UTF-8");
}}
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
}
return value;
}
}
public void init(FilterConfig config) throws ServletException {
}
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws java.io.IOException, ServletException {
HttpServletRequest req = (HttpServletRequest)request;
HttpServletResponse response2 = (HttpServletResponse)response;
// req.setCharacterEncoding("GBK");
request.setCharacterEncoding("UTF-8");
response.setContentType("text/html");
response.setCharacterEncoding("UTF-8");
String path = req.getServletPath();
String param = req.getQueryString();
String uri = req.getRequestURL().toString();
request.setAttribute("uri", uri);
request.setAttribute("ServletPath", path+"?"+param);
request.setAttribute("isFiltered", "yes");
// Return the request back to the filter chain
chain.doFilter(new MyRequest((HttpServletRequest)request),response);
}
public void destroy( ){
/* Called before the Filter instance is removed from the service by the Web container */
}
}
web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<filter>
<filter-name>MyFirstFilter</filter-name>
<filter-class>com.util.MyFirstFilter</filter-class>
<init-param>
<param-name>secondAttribute</param-name>
<param-value>filterConfig The second parameter</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>MyFirstFilter</filter-name>
<url-pattern>/checkIn.jsp</url-pattern>
</web-app>
The main thing is to customize a HttpServletRequest class and rewrite the getParameter() method inside. In fact, the core processing method is the same as method 1.