Vue3 quick start
1.Vue3 introduction
- September 18, 2020, Vue JS release version 3.0, code: One Piece (pirate king)
- It took more than 2 years 2600 + submissions,30 + RFC s,600 + PR times,99 contributors
- tags address on github: https://github.com/vuejs/vue-next/releases/tag/v3.0.0
2. What does vue3 bring
1. Performance improvement
-
Package size reduced by 41%
-
55% faster for initial rendering and 133% faster for update rendering
-
54% less memory
...
2. Upgrade of source code
-
Using Proxy instead of defineProperty to implement responsive
-
Implementation of rewriting virtual DOM and tree shaking
...
3. Embrace TypeScript
- Vue3 can better support TypeScript
4. New features
-
Composition API
- setup configuration
- ref and reactive
- watch and watchEffect
- provide and inject
- ...
-
New built-in components
- Fragment
- Teleport
- Suspense
-
Other changes
- New life cycle hook
- The data option should always be declared as a function
- Remove keyCode support as a modifier for v-on
- ...
1, Create vue3 0 project
1. Create with Vue cli
Official documents: https://cli.vuejs.org/zh/guide/creating-a-project.html#vue-create
Vue CLI 4.x requires Node js v8. 9 or higher (v10 or higher is recommended). You can use n, nvm or nvm windows to manage multiple Node versions on the same computer.
If this is the first installation, it is recommended to take the following steps
#1. Install node JS, download from the official website, the next step all the way, after the installation is completed node -v # View node version v10.16.3 # Indicates success #2. Configure npm Taobao image: npm config set registry https://registry.npm.taobao.org # npm is relatively slow. You can also replace npm with cnpm from Taobao (if the above is not available, you can use the following one, but you will use cnpm instead of npm in the future) # 3 npm install -g cnpm --registry=https://registry.npm.taobao.org #4 project to create vue (need a vue scaffold) npm install -g @vue/cli #5 if there is a problem, follow the steps below npm cache clean --force #5. When you click vue on the command line, you will be prompted. For example, vue ui is the visual operation of vue # 6 create vue project (switch to the directory you want to create) vue create luffycity
## Check the version of @ vue/cli and ensure that the version of @ vue/cli is above 4.5.0 vue --version ## Install or upgrade your @ vue/cli npm install -g @vue/cli ## establish vue create vue_test ## start-up cd vue_test npm run serve
2. Create with vite
Official documents: https://v3.cn.vuejs.org/guide/installation.html#vite
vite official website: https://vitejs.cn
- What is vite—— A new generation of front-end building tools.
- The advantages are as follows:
- In the development environment, there is no need for packaging operation, and it can be quickly cold started.
- Lightweight and fast thermal overload (HMR).
- Real on-demand compilation, no longer waiting for the completion of the whole application compilation.
- Comparison between traditional construction and vite construction
## Create project npm init vite-app <project-name> ## Enter project directory cd <project-name> ## Installation dependency npm install ## function npm run dev
2, Common Composition API
Official documents: https://v3.cn.vuejs.org/guide/composition-api-introduction.html
1. Kick off setup
- Understanding: vue3 A new configuration item in 0. The value is a function.
- setup is the "stage of performance" of all Composition API s.
- The data and methods used in the component should be configured in the setup.
- There are two return values of the setup function:
- If an object is returned, the properties and methods in the object can be used directly in the template. (focus!)
- If you return a rendering function: you can customize the rendering content. (understand)
- Note:
- Try not to contact vue2 X configuration mix
- Vue2. The properties and methods in setup can be accessed in X configuration (data, methods, computed...).
- However, vue2.0 cannot be accessed in setup X configuration (data, metrics, computed...).
- If there are duplicate names, setup takes precedence.
- setup cannot be an async function, because the return value is no longer the return object, but Promise. The template cannot see the attributes in the return object. (you can also return a Promise instance later, but you need the cooperation of suspend and asynchronous components)
- Try not to contact vue2 X configuration mix
<template> <h1>A person's information</h1> <h2>full name:{{name}}</h2> <h2>Age:{{age}}</h2> <h2>Gender:{{sex}}</h2> <h2>a The values are:{{a}}</h2> <button @click="sayHello">speak(Vue3 Configured—— sayHello)</button> <br> <br> <button @click="sayWelcome">speak(Vue2 Configured—— sayWelcome)</button> <br> <br> <button @click="test1">Test it in Vue2 Read in the configuration of Vue3 Data and methods in</button> <br> <br> <button @click="test2">Test it in Vue3 of setup Read in configuration Vue2 Data and methods in</button> </template> <script> // import {h} from 'vue' export default { name: 'App', data() { return { sex:'male', a:100 } }, methods: { sayWelcome(){ alert('Welcome to Silicon Valley') }, test1(){ console.log(this.sex) console.log(this.name) console.log(this.age) console.log(this.sayHello) } }, //Here is just a test of setup, and the problem of responsiveness is not considered for the time being. async setup(){ //data let name = 'Zhang San' let age = 18 let a = 200 //method function sayHello(){ alert(`My name is ${name},I ${age}Years old, Hello!`) } function test2(){ console.log(name) console.log(age) console.log(sayHello) console.log(this.sex) console.log(this.sayWelcome) } //Return an object (common) return { name, age, sayHello, test2, a } //Returns a function (render function) // Return() = > H ('h1 ',' Shangsi valley ') } } </script>
2.ref function
- Function: define a responsive data
- Syntax: const xxx = ref(initValue)
- Create a reference object (ref object for short) containing responsive data.
- Operation data in JS: XXX value
- Read data from template: not required value, direct: < div > {{XXX}} < / div >
- remarks:
- The received data can be basic type or object type.
- Basic type of data: the response type still depends on object get and set of defineproperty() are completed.
- Data of object type: internal "help" vue3 A new function in 0 - reactive function.
3.reactive function
- Function: define the responsive data of an object type (do not use it for basic types, but use ref function)
- Syntax: const proxy object = reactive (source object) receives an object (or array) and returns a proxy object (proxy instance object, proxy object for short)
- reactive defines responsive data as "deep-seated".
- The internal Proxy implementation based on ES6 operates the internal data of the source object through the Proxy object.
4.Vue3. Responsive principle in 0
vue2. Response of X
-
Implementation principle:
-
Object type: through object Defineproperty() intercepts the reading and modification of properties (data hijacking).
-
Array type: intercept by rewriting a series of methods to update the array. (the change method of the array is wrapped).
Object.defineProperty(data, 'count', { get () {}, set () {} })
-
-
Existing problems:
- If you add or delete attributes, the interface will not be updated.
- Modify the array directly through subscript, and the interface will not be updated automatically.
Vue3.0's response
- Implementation principle:
- Through Proxy: intercept the changes of any attribute in the object, including reading and writing attribute values, adding attributes, deleting attributes, etc.
- Through Reflect: operate on the properties of the source object.
- Proxy and Reflect described in MDN document:
-
Proxy: https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Global_Objects/Proxy
-
Reflect: https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Global_Objects/Reflect
new Proxy(data, { // Intercept reading attribute values get (target, prop) { return Reflect.get(target, prop) }, // Intercept setting property values or adding new properties set (target, prop, value) { return Reflect.set(target, prop, value) }, // Block delete attribute deleteProperty (target, prop) { return Reflect.deleteProperty(target, prop) } }) proxy.name = 'tom'
-
5.reactive comparison ref
- From the perspective of defining data:
- ref is used to define: basic type data.
- reactive is used to define: object (or array) type data.
- Note: ref can also be used to define object (or array) type data. It will be automatically converted into proxy object through reactive.
- From the perspective of principle:
- ref through object get and set of defineproperty () to implement responsive (data hijacking).
- reactive implements responsive (data hijacking) by using Proxy, and operates the data inside the source object through Reflect.
- From the perspective of use:
- ref defined data: required for operation data Value, which is not required for direct reading in the template when reading data value.
- reactive defined data: neither operation data nor read data: required value.
6. Two points for attention in setup
-
Timing of setup execution
- Execute once before beforeCreate. this is undefined.
-
Parameters of setup
- props: the value is an object, including the attributes passed from outside the component and declared and received inside the component.
- Context: context object
- Attrs: the value is an object, including: attributes passed from outside the component but not declared in the props configuration, which is equivalent to this$ attrs.
- Slots: received slot content, equivalent to this$ slots.
- Emit: a function that distributes custom events, equivalent to this$ emit.
7. Calculation attribute and monitoring
1.computed function
-
With vue2 The computed configuration function in X is consistent
-
Writing method
import {computed} from 'vue' setup(){ ... //Calculation attribute - abbreviation, not considered when writing let fullName = computed(()=>{ return person.firstName + '-' + person.lastName }) //Computational properties - complete, readable and writable let fullName = computed({ get(){ return person.firstName + '-' + person.lastName }, set(value){ const nameArr = value.split('-') person.firstName = nameArr[0] person.lastName = nameArr[1] } }) }
2.watch function
-
With vue2 The watch configuration function in X is consistent
-
Two small "pits":
- When monitoring the reactive data defined by reactive: oldValue cannot be obtained correctly and deep monitoring is forced on (deep configuration fails).
- When monitoring an attribute in the reactive data defined by reactive: the deep configuration is valid.
//Scenario 1: monitor the responsive data defined by ref watch(sum,(newValue,oldValue)=>{ console.log('sum Changed',newValue,oldValue) },{immediate:true}) //Case 2: monitor the responsive data defined by multiple ref s watch([sum,msg],(newValue,oldValue)=>{ console.log('sum or msg Changed',newValue,oldValue) }) /* Scenario 3: monitor reactive defined responsive data **** If the watch monitors the reactive data defined, the oldValue cannot be obtained correctly!! If the watch monitors responsive data defined by reactive, the deep monitoring is forced on */ watch(person,(newValue,oldValue)=>{ console.log('person Changed',newValue,oldValue) },{immediate:true,deep:false}) //The deep configuration here no longer works //Scenario 4: monitor an attribute in the responsive data defined by reactive watch(()=>person.job,(newValue,oldValue)=>{ console.log('person of job Changed',newValue,oldValue) },{immediate:true,deep:true}) //Scenario 5: monitor some attributes in the reactive data defined by reactive watch([()=>person.job,()=>person.name],(newValue,oldValue)=>{ console.log('person of job Changed',newValue,oldValue) },{immediate:true,deep:true}) //exceptional case watch(()=>person.job,(newValue,oldValue)=>{ console.log('person of job Changed',newValue,oldValue) },{deep:true}) //Here, the deep configuration is valid because it monitors an attribute in the object defined by the reactive element
3.watchEffect function
-
The routine of watch is to specify not only the monitored properties, but also the monitored callbacks.
-
The routine of watchEffect is: you don't need to specify which attribute to monitor, and which attribute is used in the monitored callback, then monitor which attribute.
-
watchEffect is a bit like computed:
- However, computed focuses on the calculated value (the return value of the callback function), so the return value must be written.
- watchEffect pays more attention to the process (the function body of the callback function), so there is no need to write the return value.
//As long as the data used in the callback specified by watchEffect changes, the callback will be directly re executed. watchEffect(()=>{ const x1 = sum.value const x2 = person.age console.log('watchEffect The configured callback was executed') })
8. Life cycle
vue2. Life cycle of X
vue3.0's lifecycle
- Vue3. Vue2.0 can continue to be used in 0 Life cycle hooks in X, but two have been renamed:
- beforeDestroy renamed beforeUnmount
- destroyed renamed unmounted
- Vue3.0 also provides a lifecycle hook in the form of Composition API, which is similar to vue2 The corresponding relationship of hooks in X is as follows:
- beforeCreate===>setup()
- created=======>setup()
- beforeMount ===>onBeforeMount
- mounted=======>onMounted
- beforeUpdate===>onBeforeUpdate
- updated =======>onUpdated
- beforeUnmount ==>onBeforeUnmount
- unmounted =====>onUnmounted
9. Custom hook function
-
What is hook—— It is essentially a function that encapsulates the Composition API used in the setup function.
-
Similar to vue2 mixin in X.
-
Advantages of custom hook: reuse code to make the logic in setup clearer and easier to understand.
10.toRef
-
Function: create a ref object whose value points to an attribute in another object.
-
Syntax: const name = toRef(person,'name')
-
Application: when you want to provide a property in a responsive object to external users separately.
-
Extension: the function of toRefs is the same as that of toRef, but multiple ref objects can be created in batch. Syntax: toRefs(person)
3, Other composition APIs
1.shallowReactive and shallowRef
-
shallowReactive: deals only with the response of the outermost attribute of the object (shallow response).
-
shallowRef: only the response of basic data type is processed, and the response of object is not processed.
-
When will it be used?
- If there is an object data, the structure is relatively deep, but the change is only the outer attribute change = = = > shallowreactive.
- If there is an object data, the subsequent function will not modify the attributes in the object, but generate a new object to replace = = = > shallowref.
2.readonly and shallowReadonly
- readonly: make a responsive data read-only (deep read-only).
- shallowReadonly: make a responsive data read-only (shallow read-only).
- Application scenario: when you do not want the data to be modified.
3.toRaw and markRaw
- toRaw:
- Function: convert a responsive object generated by reactive into a normal object. ref cannot be converted
- Usage scenario: it is used to read the ordinary object corresponding to the responsive object. All operations on this ordinary object will not cause page update.
- markRaw:
- Purpose: mark an object so that it will never become a responsive object again.
- Application scenario:
- Some values should not be set to be responsive, such as complex third-party class libraries.
- Skipping responsive transformations can improve performance when rendering large lists with immutable data sources.
4.customRef
-
Function: create a custom ref and explicitly control its dependency tracking and update trigger.
-
Achieve anti shake effect:
<template> <input type="text" v-model="keyword"> <h3>{{keyword}}</h3> </template> <script> import {ref,customRef} from 'vue' export default { name:'Demo', setup(){ // let keyword = ref('hello ') / / use the built-in ref prepared by Vue //Customize a myRef function myRef(value,delay){ let timer //Realize customization through customRef return customRef((track,trigger)=>{ return{ get(){ track() //Tell Vue that this value needs to be "tracked" return value }, set(newValue){ clearTimeout(timer) timer = setTimeout(()=>{ value = newValue trigger() //Tell Vue to update the interface },delay) } } }) } let keyword = myRef('hello',500) //Use programmer defined ref return { keyword } } } </script>
5.provide and inject
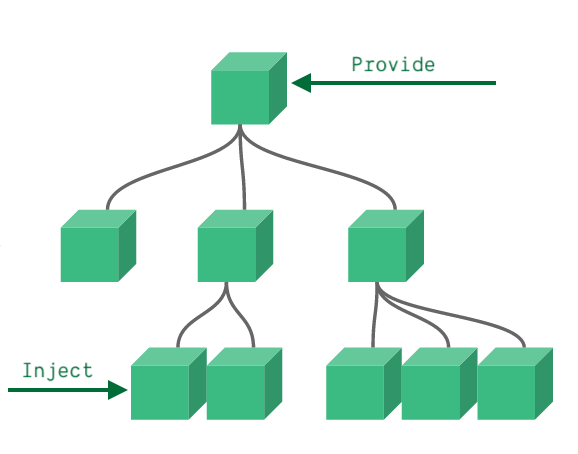
-
Function: realize (cross level) communication between progeny and descendant components
-
Routine: the parent component has a provide option to provide data, and the descendant component has an inject option to start using these data
-
Specific wording:
-
In the parent assembly:
setup(){ ...... let car = reactive({name:'Benz',price:'40 ten thousand'}) provide('car',car) ...... }
-
In descendant components:
setup(props,context){ ...... const car = inject('car') return {car} ...... }
-
6. Judgment of responsive data
- isRef: check whether a value is a ref object
- isReactive: checks whether an object is a reactive proxy created by reactive
- isReadonly: checks whether an object is a read-only proxy created by readonly
- isProxy: check whether an object is a proxy created by the reactive or readonly method
4, Advantages of Composition API
1. Problems in options API
If you use the traditional options API to add or modify a requirement, you need to modify it in data, methods and calculated respectively.
2. Advantages of composition API
We can organize our code and functions more gracefully. Make the code of related functions more orderly organized together.
5, New components
1.Fragment
- In Vue2: the component must have a root label
- In Vue3: components can have no root tag, and multiple tags will be included in a Fragment virtual element
- Reduced memory usage, label hierarchy, benefits
2.Teleport
-
What is teleport—— Teleport is a technology that can move our component html structure to a specified location.
<teleport to="Move position"> <div v-if="isShow" class="mask"> <div class="dialog"> <h3>I am a pop-up window</h3> <button @click="isShow = false">Close pop-up window</button> </div> </div> </teleport>
3.Suspense
-
Render some extra content while waiting for asynchronous components, so that the application has a better user experience
-
Use steps:
-
Asynchronous import component
import {defineAsyncComponent} from 'vue' const Child = defineAsyncComponent(()=>import('./components/Child.vue'))
-
Use suspend to wrap components and configure default and fallback
<template> <div class="app"> <h3>I am App assembly</h3> <Suspense> <template v-slot:default> <Child/> </template> <template v-slot:fallback> <h3>Loading.....</h3> </template> </Suspense> </div> </template>
-
6, Other
1. Transfer of global API
-
Vue 2.x has many global API s and configurations.
-
For example: register global components, register global instructions, etc.
//Register global components Vue.component('MyButton', { data: () => ({ count: 0 }), template: '<button @click="count++">Clicked {{ count }} times.</button>' }) //Register global directives Vue.directive('focus', { inserted: el => el.focus() }
-
-
Vue3. These API s have been adjusted in 0:
-
The global API, Vue XXX is adjusted to the application instance (app)
2.x global API (Vue) 3.x instance API (app) Vue.config.xxxx app.config.xxxx Vue.config.productionTip remove Vue.component app.component Vue.directive app.directive Vue.mixin app.mixin Vue.use app.use Vue.prototype app.config.globalProperties
-
2. Other changes
-
The data option should always be declared as a function.
-
Excessive class name change:
-
Vue2.x writing
.v-enter, .v-leave-to { opacity: 0; } .v-leave, .v-enter-to { opacity: 1; }
-
Vue3.x writing
.v-enter-from, .v-leave-to { opacity: 0; } .v-leave-from, .v-enter-to { opacity: 1; }
-
-
Remove keyCode as the modifier of v-on and no longer support config keyCodes
-
Remove v-on Native modifier
-
Binding events in parent component
<my-component v-on:close="handleComponentEvent" v-on:click="handleNativeClickEvent" />
-
Declare custom events in subcomponents
<script> export default { emits: ['close'] } </script>
-
-
Remove filter
Although the filter looks very convenient, it needs a custom syntax to break the assumption that the expression in braces is "just JavaScript", which has not only learning cost, but also implementation cost! It is recommended to replace filters with method calls or calculated properties.