Vue 3.0
1, Introduction to Vue3
1. Vue3 introduction
- September 18, 2020, Vue JS release version 3.0, code: One Piece (pirate king)
2. What does vue3 bring
- Performance improvement
- Package size reduced by 41%
- 55% faster for initial rendering and 133% faster for update rendering
- 54% less memory
- Upgrade of source code
- Using Proxy instead of defineProperty to implement responsive
- Implementation of rewriting virtual DOM and tree shaking
- Embrace TypeScript
- Vue3 can better support TypeScript
- New features
- Composition API
- setup configuration
- ref and reactive
- watch and watchEffect
- provide and inject
- New built-in components
- Other changes
- New life cycle hook
- The data option should always be declared as a function
- Remove keyCode support as a modifier for v-on
2, Create vue3 0 project
1. Create with Vue cli
## Check the version of @ vue/cli and ensure that the version of @ vue/cli is above 4.5.0
vue --version
## Install or upgrade your @ vue/cli
npm install -g @vue/cli
## establish
vue create vue3_test
## start-up
cd vue3_test
npm run serve
2. Create with vite
- What is vite—— A new generation of front-end building tools.
- The advantages are as follows:
- In the development environment, there is no need for packaging operation, and it can be quickly cold started.
- Lightweight and fast thermal overload (HMR).
- Real on-demand compilation, no longer waiting for the completion of the whole application compilation.
- Comparison between traditional construction and vite construction

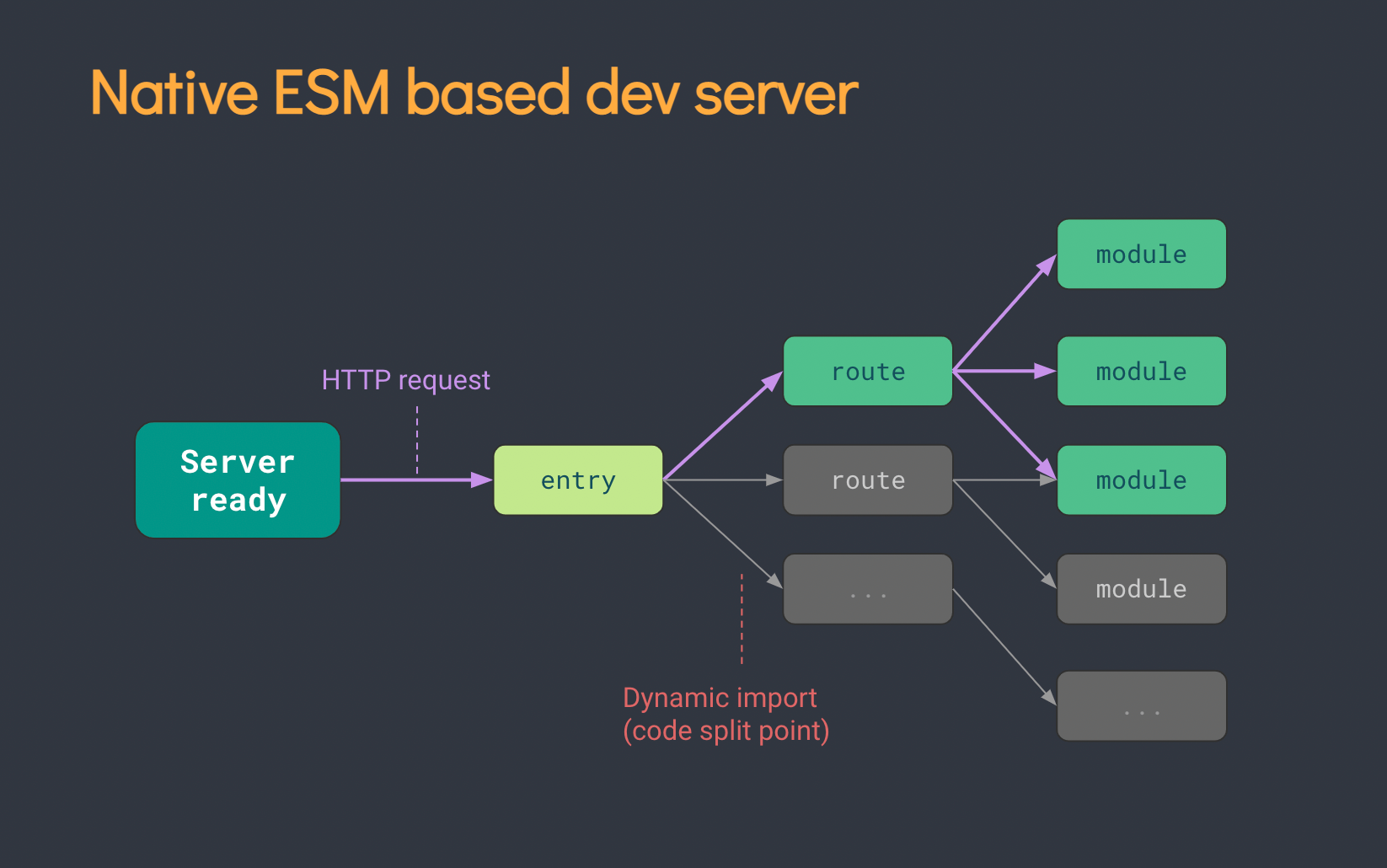
## Create project
npm init vite-app <project-name>
## Enter project directory
cd <project-name>
## Installation dependency
npm install
## function
npm run dev
3. Analysis of engineering structure
// main.js
//The Vue constructor is no longer introduced, but a factory function called createApp
import { createApp } from 'vue'
import App from './App.vue'
//Create an application instance object - app (similar to the vm in Vue2, but app is "lighter" than vm)
const app = createApp(App)
//mount
app.mount('#app')
// App.vue
<template>
<!-- Vue3 A template structure in a component can have no root tag -->
<img alt="Vue logo" src="./assets/logo.png">
<HelloWorld msg="Welcome to Your Vue.js App"/>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
components: {
HelloWorld
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
3, Common Composition API
1. Kick off setup
- Understanding: vue3 A new configuration item in 0. The value is a function.
- setup is the "stage of performance" of all Composition API s.
- The data and methods used in the component should be configured in the setup.
- There are two return values of the setup function:
- If an object is returned, the properties and methods in the object can be used directly in the template. (focus!)
- If you return a rendering function: you can customize the rendering content. (understand)
- Note:
- Try not to contact vue2 X configuration mix
- Vue2. The properties and methods in setup can be accessed in X configuration (data, methods, computed...).
- However, vue2.0 cannot be accessed in setup X configuration (data, metrics, computed...).
- If there are duplicate names, setup takes precedence.
- setup cannot be an async function, because the return value is no longer the return object, but Promise. The template cannot see the attributes in the return object. (you can also return a Promise instance later, but you need the cooperation of suspend and asynchronous components)
// App.vue
<template>
<h1>A person's information</h1>
<h2>full name:{{name}}</h2>
<h2>Age:{{age}}</h2>
<h2>Gender:{{sex}}</h2>
<h2>a The values are:{{a}}</h2>
<button @click="sayHello">speak(Vue3 Configured—— sayHello)</button>
<br>
<br>
<button @click="sayWelcome">speak(Vue2 Configured—— sayWelcome)</button>
<br>
<br>
<button @click="test1">Test it in Vue2 Read in the configuration of Vue3 Data and methods in</button>
<br>
<br>
<button @click="test2">Test it in Vue3 of setup Read in configuration Vue2 Data and methods in</button>
</template>
<script>
// import {h} from 'vue'
export default {
name: 'App',
data() {
return {
sex:'male',
a:100
}
},
methods: {
sayWelcome(){
alert('Welcome to Xiaomao school')
},
test1(){
console.log(this.sex) // male
console.log(this.name) // Zhang San
console.log(this.age) // 10
console.log(this.sayHello) // f sayHello()
}
},
//Here is just a test of setup, and the problem of responsiveness is not considered for the time being.
async setup(){
//data
let name = 'Zhang San'
let age = 18
let a = 200
//method
function sayHello(){
alert(`My name is ${name},I ${age}Years old, Hello!`)
}
function test2(){
console.log(name) // Zhang San
console.log(age) // 18
console.log(sayHello) // f sayHello
console.log(this.sex) // undefined
console.log(this.sayWelcome) // undefined
}
//Return an object (common)
return {
name,
age,
sayHello,
test2,
a
}
//Returns a function (render function)
// Return() = > H ('h1 ',' Shangsi valley ')
}
}
</script>