1, The second feature of object-oriented -- Inheritance
1. Why should there be class inheritance? (inherited benefits)
* ① The redundancy of code is reduced and the reusability of code is improved * ② Convenient for function expansion * ③ It provides a premise for the use of polymorphism
Illustration:
2. Format of inheritance:
class A extends B{}
A: Subclass, derived class, subclass
B: Parent class, superclass, base class, superclass
3. What are the differences after a child class inherits the parent class?
3.1 embodiment: once subclass A inherits parent class B, subclass A obtains all the attributes and methods declared in parent class B.
In particular, for properties or methods declared as private in the parent class, after the child class inherits the parent class, it is still considered to have obtained the private structure in the parent class. Only because of the influence of encapsulation, the subclass cannot directly call the structure of the parent class.
3.2 after a subclass inherits the parent class, it can also declare its own unique properties or methods: to realize the expansion of functions.
The relationship between class and parent class is different from that between subset and collection.
Extensions: extension
4. Description of inheritance in Java
1. A class can be inherited by multiple subclasses.
2. Single inheritance of classes in Java: a class can only have one parent class
3. Child parent class is a relative concept.
4. The parent class directly inherited by the child class is called direct parent class. The parent class of indirect inheritance is called indirect parent class
5. After the subclass inherits the parent class, it obtains the properties and methods declared in the direct parent class and the indirect parent class
Illustration:
5.java. Understanding of lang.Object class
- If we do not explicitly declare the parent class of a class, this class inherits from Java Lang.Object class
- All Java classes (except java.lang.Object class) directly or indirectly inherit from java.lang.Object class
- This means that the Java class has java Functions declared by lang.Object class.
2, Method rewriting
1. What is method override or overwrite?
After the subclass inherits from the parent class, it can override the methods with the same name and parameters in the parent class
2. Application
After rewriting, when a subclass object is created and a method with the same name and parameter in the child parent class is called through the subclass object, the actual execution is that the subclass rewrites the method of the parent class.
3. Examples
class Circle{ public double findArea(){}//Find area } class Cylinder extends Circle{ public double findArea(){}//Surface area } *************** class Account{ public boolean withdraw(double amt){} } class CheckAccount extends Account{ public boolean withdraw(double amt){} }
4. Rewritten rules
-
Method declaration: permission modifier return value type method name(parameter list ) throws Type of exception{
-
//Method body
-
}
-
The Convention is commonly known as: the method in the subclass is called overridden method, and the method in the parent class is called overridden method
-
① The method name and parameter list of the method overridden by the subclass are the same as those of the method overridden by the parent class
-
② The permission modifier of the method overridden by the subclass is not less than the permission modifier of the method overridden by the parent class
-
>Special case: a subclass cannot override a declaration in a parent class private Method of permission
-
③ Return value type:
-
>The return value type of the method whose parent class is overridden is void,The return value type of the method overridden by the subclass can only be void
-
>The return value type of the method whose parent class is overridden is A Type, the return value type of the method overridden by the subclass can be A Class or A Subclass of class
-
>The return value type of the method whose parent class is overridden is the basic data type(For example: double),The return value type of the method overridden by the subclass must be the same basic data type(Must be double)
-
④ The exception type thrown by the method overridden by the subclass shall not be greater than the exception type thrown by the method overridden by the parent class (specifically in exception handling)
- Methods with the same name and parameters in subclasses and superclasses are either declared non static (consider overriding), or both declared static (not overriding).
## 5. Interview questions: Distinguish between method rewriting and overloading? Answer: ① Concept of both: ② Specific rules for overloading and overriding ③ Overload: not polymorphic. Rewriting: expressed as polymorphism. Overloading means that multiple methods with the same name are allowed, and the parameters of these methods are different. The compiler modifies the name of the method with the same name according to different parameter tables of the method. For the compiler, these methods with the same name become different methods. Their call addresses are bound at compile time. Java Overloading of can include parent and child classes, that is, child classes can overload methods with different parameters of the same name of the parent class. Therefore: for overloads, the compiler has determined the method to be called before the method call, which is called "early binding" or "static binding"; For polymorphism, the interpreter will not determine the specific method to be called until the moment of method call, which is called "late binding" or "dynamic binding". Quote a sentence Bruce Eckel Don't be silly. If it's not late binding, it's not polymorphic # 3, Keywords: super ## 1. The super keyword can be understood as: the of the parent class ## 2. Structures that can be called Properties, methods, constructors ## 3.super calling properties and methods 3.1 We can in subclass methods or constructors. through the use of"super.attribute"or"super.method"Explicitly call the properties or methods declared in the parent class. However, usually, we are used to omitting"super." 3.2 Special case: when the properties of the same name are defined in the subclass and the parent class, we must explicitly use the attributes declared in the parent class in the subclass."super.attribute"Indicates that the property declared in the parent class is called. 3.3 Special case: when a child rewrites the method in the parent class, we want to use the method that is rewritten in the parent class in the subclass method, so we must use it explicitly."super.method"Indicates that the overridden method in the parent class is called. ## 4.super call constructor 4.1 We can use it explicitly in the constructor of subclasses"super(parameter list )"Call the specified constructor declared in the parent class 4.2 "super(parameter list )"The use of must be declared in the first line of the subclass constructor! 4.3 In the constructor of the class, for"this(parameter list )"or"super(parameter list )"Only two and one, not both 4.4 On the first line of the constructor, there is no explicit declaration"this(parameter list )"or"super(parameter list )",The constructor of the parent class null parameter is called by default: super() 4.5 Among multiple constructors of a class, at least one class is used in the constructor"super(parameter list )",Call the constructor in the parent class # 4, The whole process of subclass object instantiation Just understand. ## 1. In terms of results: Inheritance > After the subclass inherits the parent class, it obtains the properties or methods declared in the parent class. > If you create a subclass object, the properties declared in the parent class will be loaded in heap space. ## 2. From the process point of view When we create a subclass object through the subclass constructor, we must directly or indirectly call the constructor of its parent class, and then call the constructor of the parent class of the parent class,...Until called java.lang.Object Class to the constructor of a null parameter. It is precisely because the structure of the parent class has been loaded that the structure of the parent class in memory can be seen, and the subclass object can be considered for calling. Illustration: 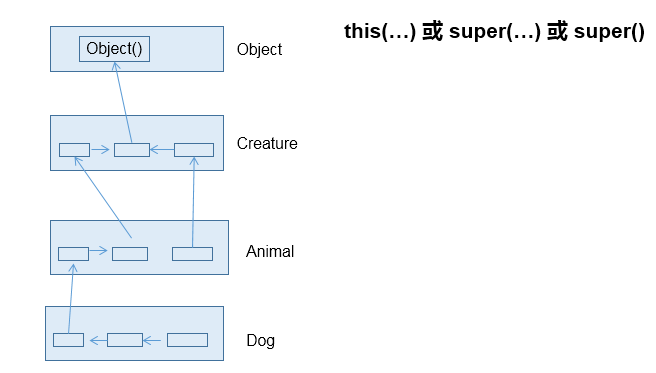 ## 3. Emphasis Although the constructor of the parent class is called when creating a subclass object, an object has been created from beginning to end, that is new Subclass object of. 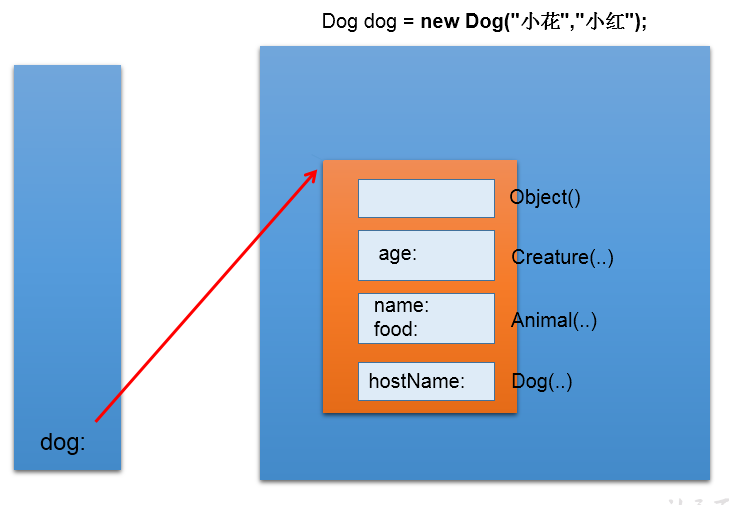 # 5, Object oriented feature three -- polymorphism ## 1. Understanding of polymorphism: it can be understood as multiple forms of a thing. ## 2. What is polymorphism Object polymorphism: the reference of the parent class points to the object of the child class (or the reference assigned to the parent class by the object of the child class) give an example: Person p = new Man(); Object obj = new Date(); ## 3. Use of polymorphism: virtual method call > With object polymorphism, we can only call the methods declared in the parent class at compile time, but at run time, we actually execute the methods of the child class overriding the parent class. > Summary: compile and look at the left; Run, look to the right. ## 4. Premise of polymorphism: ① Class inheritance ② Method rewriting ## 5. Application examples of polymorphism: Example 1: ```java public void func(Animal animal){//Animal animal = new Dog(); animal.eat(); animal.shout(); }
Example 2:
public void method(Object obj){ }
Example 3:
class Driver{ public void doData(Connection conn){//conn = new MySQlConnection(); / conn = new OracleConnection(); //Standardized steps to manipulate data // conn.method1(); // conn.method2(); // conn.method3(); } }
6. Precautions for polymorphism use:
The polymorphism of objects is only applicable to methods, not attributes (see the left for compilation and operation)
7. On upward and downward Transformation:
7.1 upward Transformation: polymorphism
7.2 downward transformation
7.2.1 why use downward Transformation:
After the polymorphism of the object, the memory actually loads the properties and methods unique to the subclass. However, because the variable is declared as the parent type, only the properties and methods declared in the parent class can be called during compilation. Subclass specific properties and methods cannot be called. How can I call subclass specific properties and methods? Use downward transformation.
7.2.2 how to realize downward Transformation:
Use cast character: ()
7.2.3 precautions during use:
① When using strong conversion, an exception of ClassCastException may occur.
② In order to avoid the exception of ClassCastException during the downward transformation, we first judge the instanceof before the downward transformation. Once true is returned, we will carry out the downward transformation. If false is returned, no downward transformation is performed.
7.2.4 use of instanceof:
① a instanceof A: judge whether object a is an instance of class A. If yes, return true; If not, false is returned.
② If a instanceof A returns true, a instanceof B also returns true Where class B is the parent of class A.
③ It is required that the class to which a belongs and class a must be the relationship between subclass and parent class, or compilation error.
7.2.5 diagram:
8. Interview questions:
8.1 what is your understanding of polymorphism?
① Realize the universality of the code.
② public boolean equals(Object obj) {} defined in the Object class
JDBC: use java programs to operate (get database connection, CRUD) databases (MySQL, Oracle, DB2, SQL Server)
③ The use of abstract classes and interfaces must reflect polymorphism. (abstract classes and interfaces cannot be instantiated)
8.2 is polymorphism compile time behavior or runtime behavior?
6, Use of Object class
1.java. Description of lang.Object class
* 1.Object Class is required Java Root parent of class * 2.If not used in the declaration of the class extends Keyword indicates its parent class, then the default parent class is java.lang.Object class * 3.Object Functions in class(Properties and methods)It is universal. * Properties: None * method: equals() / toString() / getClass() /hashCode() / clone() / finalize() * wait() , notify(),notifyAll() * * 4. Object Class only declares a constructor with an empty parameter
2.equals() method
2.1 use of equals
* 1. Is a method, not an operator * 2. Applies only to reference data types * 3. Object In class equals()Definition of: * public boolean equals(Object obj) { return (this == obj); } * explain: Object Class equals()and==The function of is the same: compare whether the address values of two objects are the same.That is, whether two references point to the same object entity * * 4. image String,Date,File,The wrapper class has been rewritten Object Class equals()method. After rewriting, the comparison is not * Whether the addresses of the two references are the same, but compare the addresses of the two objects"Entity content"Whether it is the same. * * 5. Usually, if we use custom classes equals()It is usually used to compare two objects"Entity content"Whether it is the same. So, we * You need to be right Object Class equals()Rewrite. * Rewriting principle: compare whether the entity contents of two objects are the same.
2.2 how to rewrite equals()
2.2.1 example of manual Rewriting:
class User{ String name; int age; //Override its equals() method public boolean equals(Object obj){ if(obj == this){ return true; } if(obj instanceof User){ User u = (User)obj; return this.age == u.age && this.name.equals(u.name); } return false; } }
2.2.2 how to implement in development: automatically generated
2.3 review the use of = = operator
* == : operator * 1. Can be used in basic data type variables and reference data type variables * 2. If you are comparing basic data type variables: compare whether the data saved by the two variables are equal. (not necessarily the same type) * If you are comparing variables of reference data type: compare whether the address values of the two objects are the same.That is, whether two references point to the same object entity * Supplement: == When using the symbol, the variable types on the left and right sides of the symbol must be consistent.
3. toString() method
3.1 use of tostring()
* 1. When we output a reference to an object, we actually call the current object toString() * * 2. Object In class toString()Definition of: * public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); } * * 3. image String,Date,File,The wrapper class has been rewritten Object Class toString()method. * So that when the object is called toString()When, return"Entity content"information * * 4. Custom classes can also be overridden toString()Method, when called, returns the name of the object"Entity content"
3.2 how to rewrite toString()
give an example:
//Automatic implementation @Override public String toString() { return "Customer [name=" + name + ", age=" + age + "]"; }
4. Interview questions:
① What is the difference between final, finally and finalize?
② = = is different from equals()
7, Unit test method (JUnit)
* Java Medium JUnit unit testing * * Steps: * 1.Current project in - Right click to select: build path - add libraries - JUnit 4 - next step * 2.establish Java Class for unit testing. * At this time Java Class requirements:① Such is public of ②This class provides a public parameterless constructor * 3.Unit test methods are declared in this class. * The unit test method at this time: the permission of the method is public,No return value, no formal parameter * * 4.The unit test method needs to declare the following comments:@Test,And import in the unit test class: import org.junit.Test; * * 5.After declaring the unit test method, you can test the relevant code in the method body. * 6.After writing the code, left click and double-click the unit test method name. Right click: run as - JUnit Test * * explain: * 1.If there is no exception in the execution result: green bar * 2.If the execution result is abnormal: red bar
8, Use of packaging
1. Why packaging (or packaging)
In order to make the variables of basic data type have the characteristics of class, packaging class is introduced.
2. Basic data type and corresponding packaging class
3. Conversion between types to be mastered: (basic data type, packing class, String)
Easy version:
Basic data type < --- > packaging class: new features of JDK 5.0: automatic packing and automatic unpacking
Basic data type, wrapper class -- > String: call valueof (xxxxxx) overloaded by String
String --- > basic data type and wrapper class: call parseXxx(String s) of wrapper class
Note: NumberFormatException may be reported during conversion
Examples of application scenarios:
For adding elements in the Vector class, only methods with formal parameters of Object type are defined:
v.addElement(Object obj); // Basic data type -- > wrapper class -- > use polymorphism