structure
go mod init xx initialization file
Compile: go build XX Go generation exe file
go run xx.go execution file
file structure
filename|
- src file code
1. Variables
statement
var age =12 //Declare variable var age int //The specified variable type is not assigned. The default value is 0 age :=12 //If you do not write the type of variable, judge the defined type according to the following data type // Multivariable declaration var num1 ,num2 int n1,n2 :=2,3 //Declare multiple variables at once var ( n1 = 12 n2 = 2 n3 = 12 ) fmt.Printf("%T",n1) //Output variable type
Variable type:
Integer type:
byte =uint 8 range 0-256
Negative binary representation: complement representation: that is, inverse code + 1
-5 the computer indicates 0000 0101, inverse code 111 1010 - > complement code 1111 1011,
int8 RANGE 1000 0000 - 1 = inverse code 01111 1111 - > initial 1000 0000 - > i.e. - 128-127 range
Output variable type package main // Import multi package operation import( "fmt" "unsafe" ) func main() { var n1 int fmt.Printf("%T",n1) //Output variable type fmt.Println(unsafe.Sizeof(n1)) //Bytes occupied by output variable - > 8 }
Floating point number:
There may be loss of accuracy > float64 is recommended
float 64 is used by default
Character type
var a byte = 'a'
fmt.println(a) / / output 67 ASCII code value
The character corresponding to golong is encoded in UTF-8 (Unicode is the corresponding string, and UTF-8 is one of the encoding schemes of Unicode)
func main() { var n1 byte ='a' fmt.Println(n1) //Output corresponding ASCII value fmt.Printf("%c",n1) // Output a character }
Escape character: \ beginning

Boolean type
bool type only allows true and false. The default declaration is false when it is not defined
var f bool
String type
String is immutable, which means that once the string is defined, the value of the characters in it cannot be changed
var s1 string ="abc" s1="nnn" //Can be modified s1[0]="n" //error If there are special characters in the string, Use backquotes var s2 string = `nihao "dea" ` Character splicing var s3 string = "ABC" + "abc" Line feed var s4 string = "123" + "234" //go or add line feed by default, so putting the + sign on the upper line at the end means that the statement is not executed
Data type conversion
The expression T(v) T is a variable whose target type v needs to be converted
func main() { var n1 int =100 var n2 float32 = float32(n1) //Note: the type of n1 is still int, but the value of n1 is converted from 100 to float32 fmt.Println(n2) fmt.Printf("n1:%T\n",n1) // int fmt.Printf("n2:%T",n2) // float32 take int 64 Turn into int 8 It will cause data overflow var n3 int64 = 88888 var n4 int8 = int8(n3) }
Basic data type (integer, floating point, etc.) to string type
Mode 1: fmt.Sprintf() func main() { var n1 int =100 var s1 string = fmt.Sprintf("%d",n1) //%d source data type fmt.Printf("s1 tytpe %T ,value %s",s1,s1) } Mode 2: strconv in FormatXXX method package main import ( "fmt" "strconv" ) func main() { var n1 int =100 var s1 string = strconv.FormatInt(int64(n1),10) //The first parameter must be int 64. The second parameter specifies the value in hexadecimal form, 10 bit hexadecimal form fmt.Printf("s1 tytpe %T ,value %s",s1,s1) }
String - > other types strcon When converting parsexxx string to other data types, you must ensure that it can be converted, otherwise the final result will be the default value of the corresponding data type
package main import ( "fmt" "strconv" ) func main() { var n1 string = "123" var s1,_ = strconv.ParseInt(n1,10 ,8) //Returns 2 values. The second value is_ ellipsis fmt.Printf("s1 tytpe %T ,value %v", s1, s1) }
Pointer
-
&+Variable gets the address in variable memory
-
Pointer variable:
-
Define a variable var pointer variable name pointer type
- var par * int =&age
package main import ( "fmt" ) func main() { var n1 string = "123" var ptr *string =&n1 fmt.Println(ptr) //0xc000038240 fmt.Println(&ptr) // 0xc000006028 fmt.Println(*ptr) //123 }
-
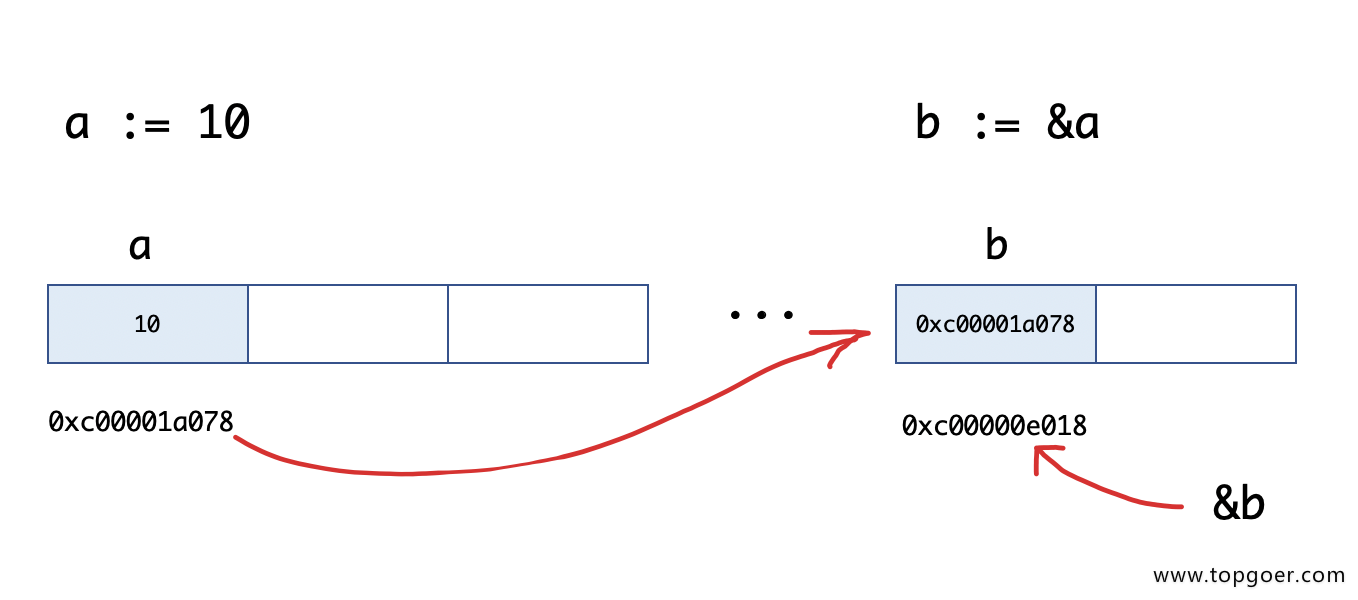
Summarize the two most important symbols & (take the memory address) * (take the value corresponding to the memory address)
Pointer modification value func main() { var n =10 var ptr * int =&n *ptr =12 fmt.Println(n) //12 }
Flag
Variable : Numbers, characters, underscores. Strictly case sensitive, the first letter cannot be a number, cannot contain spaces, and cannot use keywords
- underline is ignored
import ("fmt" _"strconv" )
Naming rules
-
Package name: it is recommended that the name of the package be consistent with the directory
-
The main package is the entry package of a program, so the package of the main function is defined as package main. If it is not defined as the main package, the executable file cannot be obtained
-
If the initial letter is capitalized, it can be accessed by other packages (the package name is placed under GOROOT)
Configure GOPATH
Wrong shooting
go env -w GO111MODULE=off
Method 2
go env -w GO111MODULE=on
go mod init goFIles generate go Mod file
The package name is calculated after $GOPATH/src /
operator
package main import ( "fmt" _ "goFiles/src/test" ) func main() { var n1 int8 = 10 var n2 int8 = 12 var sum int8 = n1+n2 fmt.Println(sum) var c int8= n1*n2 fmt.Println(c) var m int8 = n2%n1 //Take mold and surplus fmt.Println(m) }
Get user input
package main import ( "fmt" ) func main() { var age int fmt.Println("Please enter age:") fmt.Scanln(&age) fmt.Println(age) }
Process control
Branching structure
if
if Conditional expression{ Logic code }else if Conditional expression{ // else must be in the} back room before Logic code }else{ Logic code }
switch
switch The expression has a return value{ case key1,key1.1,key1.2 : //case can be followed by multiple values separated by commas expression case key2: expression default: When the above conditions are not met,Just run }
for
for i:=0 ; i <5; i++{ Circulatory body } be careful for The initial expression of cannot be used var Define the form of the variable Mode 2 i:=1 for i<10{ i++ } for range structure for key,val range dict{ } simulation while var x int = 0 for x < 5 { fmt.Println(x) x++ }
for range
x:=[3]int{1,2,3} x := [3]int{1, 2, 3} for i,j :=range x{ fmt.Println(i,j) }
break can only end the nearest loop
End the cycle corresponding to the specified label func main() { lable1: //Mark the first for loop and label it for i := 0; i < 10; i++ { fmt.Println(i) for j := 0; j < 10; j++ { if j == 1 { break lable1 //Jump out of the first cycle } } } }
goto jumps to the specified line of the program
code goto label1 code lable1: code
return
func function(Formal parameter)(Return value type list){ return Return value }
function
The initial capital letter is equivalent to Public It can be used by this package file and other package files Initial lowercase equals private Can only be used by this package
func main() { n1 := 1 n2 := 2 r1,r2 := calc(n1,n2) fmt.Println(calc(n1, n2)) } func calc(num1 int, num2 int) (int,int) { //If you return a parameter, you can omit () return num1+num2 ,num1-num2 }
Parameter memory processing in python
Parameter passing in Python is "reference passing", which can be divided into two categories:
The "write operation" on the "variable object" directly acts on the original object itself.
A "write operation" on the "immutable object" will generate a new "object space" and fill this space with new values. (it has the effect of "value transfer" in other languages, but not "value transfer")
Variable objects: dictionaries, lists, collections, custom objects
Immutable objects: numbers, tuples, strings, function s, etc
def l(a, b): print("a_id:{},b_id:{}".format(id(a), id(b))) a = 10 print("a_id:",id(a)) b[1] = 20 print("b_id",id(b)) a = 0 b = [0, 0] print("a_id:{},b_id:{}".format(id(a), id(b))) l(a, b) print(a, b) output: a_id:140730996810544, b_id:1879277392584 a_id:140730996810544, b_id:1879277392584 a_id: 140730996810864 b_id 1879277392584 0 [0, 20]
go language
The parameter passing parameter will be copied. When modifying the parameter, it will not affect the parameter. go is different from python, and the variable type will also be copied. Modifying the parameter will not affect the actual parameter
func main() { var a int=0 var b int =1 c:=[3]int{0,0,0} fmt.Println(&a,&b,&c) //88 a0 [0,0,0] l(a,b,c) fmt.Println(a,b,c) // Output 0,1 [0,0,0] } func l(a int ,b int,c [3]int){ fmt.Println(a,b,c) fmt.Println(&a,&b,&c) //E0 E8 [0,0,0] has been copied a=12 b=12 c[0]=12 //Modify the value of variable object in formal parameter fmt.Println(&a,&b,&c) }
Modify argument value func main() { n1 := 1 n2 := 2 swap(&n1, &n2) fmt.Println(n1,n2) //Swap n1 and N2 - > 2, 1 } //Parameter type is pointer func swap(num1 *int, num2 *int) { //If you return a parameter, you can omit () var t int t=*num1 *num1=*num2 *num2=t //Mode 2 *num1, *num2 = *num2, *num1 }
Function does not support overloading (Java with the same function name)
Variable parameter ... To show func main() { n1 := 1 n2 := 2 swap(n1, n2) fmt.Println(n1,n2) //Swap n1 and N2 - > 2, 1 } // Parameter name type func swap(t...int) { //If you return a parameter, you can omit () fmt.Println(t) // Output [1,2] for i:=range t{ fmt.Println(i) }
In go, function is also a data type. If it can be assigned to a variable, the variable is a variable of function type Functions can be called through this variable (similar to aliases)
func main() { n1 := 1 n2 := 2 //A function is also a data type that can be assigned to a variable sw:=swap //A variable is a function type variable //Equivalent to calling a function sw(n1, n2) fmt.Println(n1,n2) } func swap(t...int) { //If you return a parameter, you can omit () fmt.Println(t) for i:=range t{ fmt.Println(i) }
Functions are also data types, and functions can also be used as formal parameters
func main() { //t:=test test2(test) } func test(t ...int) { //If you return a parameter, you can omit () fmt.Println("test") } func test2(fun func(...int)) { fmt.Println("test2") fun(1) // Incoming function }
It supports user-defined types, which is equivalent to taking aliases. Although it is an alias, go recognizes that myint and int are not the same data type during compilation, and supports function type aliases
Type alias data type
type myint int
func main() { type myint int var a int = 10 var b myint b = myint(a) //int -> myint fmt.Println(b) }
type is more than just used to define a series of aliases. You can also define methods for new types.
The above name type can define the method as follows:
type name string func (n name) len() int { return len(n) } func main() { var myname name = "taozs" //It's actually a string type l := []byte(myname) //String to byte array fmt.Println(len(l)) //Byte length fmt.Println(myname.len()) //Method of calling object }
type definition function
func main() { var i int =0 var j int =1 add_d(add,i,j) } type add1 func (a int,b int) //Define two function variables of type int func add(a int,b int) { fmt.Println(a,b) } func add_d(fu add1,a int,b int) { //Declare fu to receive function variables fu(a,b) //Electrophoretic afferent function fmt.Println("---") }
Support function return value naming,
func test(t int) (sun int,sub int){ sun:=t+1 sub:=t-1 return }
Introduction of package
Under the project root directory, use go mod init to generate the x.mod file with the file name of the project root directory
goFiles| |_src |_test |_test.go |_main.py //main.go package main import ( "fmt" "goFiles/src/test" //Package path ) func main() { //t:=test fmt.Println(1) fmt.Println(test.TestNum) //Use test (package name) to locate the package in front TestNUm } //test.go package test //The declaration of the package is the same as the folder name var TestNum int = 10 //Initial capitalization ensures access outside the package
init function
The init function is called before the main function
Step 1: definition of global variables
Step 2: definition of init
Step 3: call the main function
When importing a package, first execute init in the import package file, and finally execute init in main
Anonymous function
If you want to use a function only once, you can use anonymous functions
Use it directly when defining
func main() { Mode 1 // Anonymous function definition calling at the same time test:=func (a int,b int)(int){ return a+b }(10,20) //Incoming parameters fmt.Println(test) //Output 30 Mode 2 //Equivalent to anonymous sub function sub := func(a int, b int) (int) { return a - b //Incoming parameters } result := sub(30, 70) fmt.Println(result) }
closure
A set consisting of a function and its associated introduction environment
Closure: the returned anonymous function + variables other than anonymous functions are closures
// Declare that the function returns a function func getsum() func (a int,)(int){ var sum int =0 return func(a int) int { sum =sum+a return sum } } func main() { f :=getsum() fmt.Println(f(1)) //Return 1 fmt.Println(f(2)) //Return to 3. At this time, sum is 1 + 2 = 3 of the last time //The variable referenced in the anonymous function will always be saved in memory and used all the time }
defer keyword
Resources need to be created in the function in order to release resources in time after the function is executed go language introduces defer keyword
When the defer keyword is encountered in go language, the statements in the defer keyword will be pushed into the stack, and the relevant values will be copied to the stack. After the function is executed, it will be taken out of the stack (first in and last out) for execution
func test(a int){ defer fmt.Println("1") defer fmt.Println(2) //Finally into the stack, so pop up the execution first fmt.Println(a) //First execution } func main() { test(3) }
Output 3 2 1
func test(a int){ defer fmt.Println(a) //Start executing a =3 and push the execution result into the stack a+=2 fmt.Println(a) } func main() { test(3) } Output 5 3
application
Connect to the database, and write the statement of closing the connection to defer
System function
Character function
len()
func main() { var s string ="Hello" //In go language, Chinese characters are UTF_8 character set, 3 bytes for one Chinese character fmt.Println(len(s)) //Output 6 }
String traversal
func main() { var s string = "Hello" //In go language, Chinese characters are UTF_8 character set, 3 bytes for one Chinese character for _, value := range s { //Returns the index and the corresponding value fmt.Printf("Value is:%c\n", value) } //Mode 2 //Slice with [] run (variable) r := []rune(s) for i:=0;i<len(r);i++{ fmt.Printf("%c",r[i]) } }
String conversion strconv package
func main() { var s string = "1" //str to int num, err := strconv.Atoi(s) if err == nil { fmt.Println(num, err) } else { fmt.Println("111", err) } //int to str str := strconv.Itoa(88) fmt.Println(str) }
Count the total number of one character to import - > strings package
func main() { var s string = "1231231233454" count := strings.Count(s, "1") fmt.Println(count) //Case insensitive string comparison fmt.Println(strings.EqualFold("go", "GO")) //Return true //Returns the index value of the first occurrence of a character in a string fmt.Println(strings.Index("abcsac", "h")) //Return 0 does not exist, return - 1 //String substitution //strings.Replace("original character", "original replacement character", "replace with", matching replacement times) str := strings.Replace("abcabcabc", "a", "A", 2) //Replace a with A. replace 2 times. When replacing all, n passes in - 1 fmt.Println(str) //AbcAbcabc //The cutting of string returns an array str1 := strings.Split("abcabc", "a") fmt.Println(str1) //Output [BC] for _, value := range str1 { fmt.Println(value) } //String case switching fmt.Println(strings.ToUpper("go")) //Lowercase to uppercase fmt.Println(strings.ToLower("GO")) //Capital to lowercase //Remove the left and right spaces fmt.Println(strings.TrimSpace(" 12 12 12 ")) fmt.Println(strings.Trim("-go -","-")) //Remove the left and right specified strings //Determines whether a string has been started fmt.Println(strings.HasPrefix("www.baidu.com","www")) //Return bool type true //Determines whether a string has ended fmt.Println(strings.HasSuffix("www.baidu.com","com")) }
Date function time package
package main import ( "fmt" "time" ) func main() { now := time.Now() fmt.Println(now) fmt.Printf("%v\n", now.Year()) fmt.Printf("%v\n", int(now.Month())) //int English to number //Formatting of dates ///Using Sprintf, you can receive Prinf with a string and output the information directly str := fmt.Sprintf("%d-%d-%d %d-%d-%d\n", now.Year(), now.Month()) fmt.Printf(str) //The value in format must be written in this way date1 := now.Format("2006-01-02 15:04:05") fmt.Printf(date1) }
Built in function
new is used to allocate memory. The first parameter is type and returns a pointer of type
func main() { //New allocates memory, and the return value of the new function is the pointer num *int of the corresponding type num := new(int) *num=10 fmt.Printf("%T",num) //The corresponding memory address stored in the num pointer, & num takes the memory address of the pointer, * num goes to the corresponding value in the memory address corresponding to the pointer fmt.Println(num,&num,*num) }
Error handling mechanism defer+recover improves program robustness, similar to try catch
func main() { test() //Prevent program interruption caused by program error in test fmt.Println("main"r) } func test() { defer func(){ err := recover() //If no error is caught, the return value is 0: nil if err != nil { fmt.Println("err:", err) } }() num1 := 1 num2 := 0 result := num1 / num2 fmt.Println(result) }
Custom error
array
Multiple declaration methods var scores [5]int var n [3]int = [3]int{1, 2, 3} var n1 = [3]int{1, 2, 3} var n2 = [...]{1: 1, 2: 22, 3: 33, 0: 44} //Subscript: value
func test() { var scores [5]int //Declaration array scores[0] =15 sum:=0 for i,v :=range scores{ fmt.Println(i) sum+=v } fmt.Println(sum) fmt.Println(scores) }
Two dimensional array
var a[2][3]int output[[0,0,0] [0,0,0]] var b [2][3]int = [2][3]int{{1,2,3},{1,2,3}}
Two dimensional array traversal func main() { var b [2][3]int = [2][3]int{{1, 2, 3}, {1, 2, 3}} fmt.Println(b) for i := 0; i < len(b); i++ { for j := 0; j < len(b[i]); j++ { fmt.Printf("b[%v][%v]=%v\t", i, j, b[i][j]) } } for key, value := range b { for k, v := range value { fmt.Printf("b[%v][%v]=%v\t", key, k, v) } } }
section
func main() { var b [3]int = [3]int{1, 2, 3} c := b[1:2] //Slicing operation fmt.Println(c) }
Original 3,6,7,1,4,7
Slice 1:3, i.e. 6:9. The volume of slice is generally twice that of slice
The slice stores the memory address of the original data in memory
Therefore, modifying the sliced value will affect the value in the original data
var b [3]int = [3]int{1, 2, 3} c := b[1:2] //Slicing operation fmt.Printf("%v\n", &b[1]) fmt.Printf("%v", &c[0]) The two values are consistent, This and python Not the same
func main() { var b []int = []int{1, 2, 3} var c []int = make([]int,10) //Declare a slice with a slice length of 10 //c=b / / this assignment is the same as the corresponding address in memory. Modifying the data in c will affect b copy(c,b) //Copying b array into c will form two arrays. Modifying c will not affect b fmt.Printf("%v\t" ,&c[0]) fmt.Printf("%v",&b[0]) fmt.Println(c) }
mapping
map key value pair: a pile of matching information
func main() { //Only declare that the map memory has no allocated space. The space can only be allocated after initialization through the make function var a map[int]string //Method 1 create a = make(map[int]string,10) //Mode 2 b:=make(map[int]string) //Mode 3 c:=map[int]string{12:"Zhang",13:"king"} a[12]="123" a[13]="234" fmt.Println(a) for key,values :=range a{ fmt.Printf("%v:%v\n",key,values) }
map operation
increase
map[key]=value
delete
Delete (variable, key)
lookup
value,bool_flag: = variable [value]
c:=map[int]string{12:"Zhang",13:"king"} c[14]="Lee" delete(c,12) value,bool_flg :=c[14] fmt.Println(value,bool_flg) fmt.Println(c)
func main() { c:=make(map[int]map[int]string) // [1: [1: Li]] c[1]=make(map[int]string) c[1][1]="Lee" fmt.Println(c) for _,v:= range c{ fmt.Println(v) for k1, v1 := range v{ fmt.Println(k1,v1) } } }
structural morphology
package main import "fmt" //Define structure type animal struct{ name string age int } func main() { //An example of friction mixed shear structure var cat animal cat.age=12 cat.name="cat" //Initialize and assign var dog animal=animal{"dog",12} fmt.Println(dog) fmt.Println(cat) }