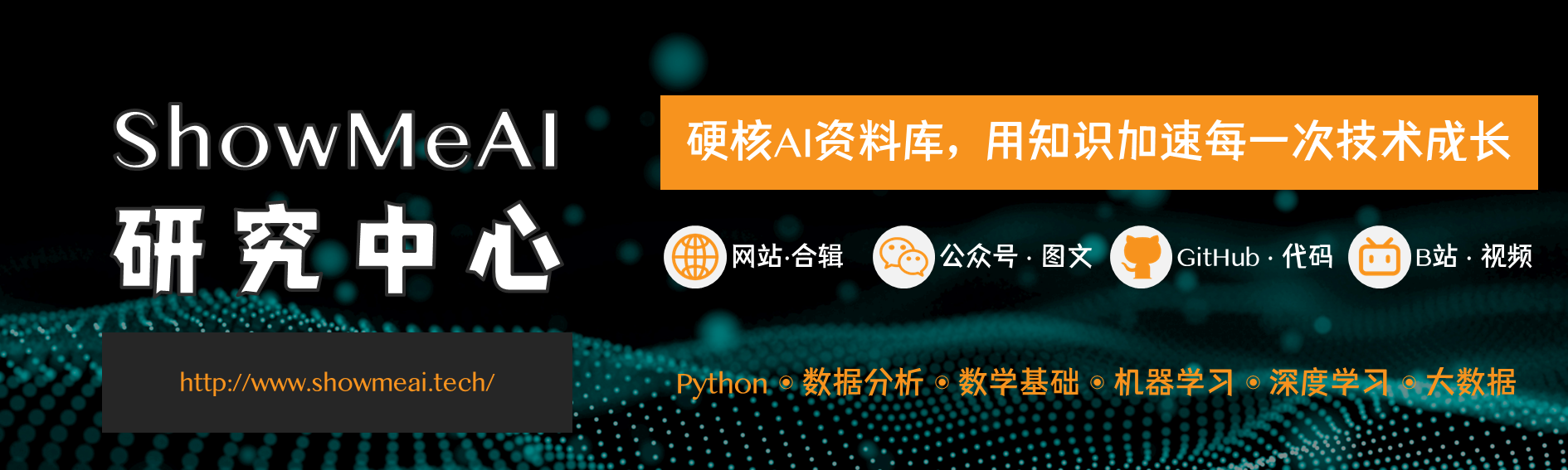
Tutorial address: http://www.showmeai.tech/tutorials/56
Article address: http://www.showmeai.tech/article-detail/80
Notice: All Rights Reserved. Please contact the platform and the author for reprint and indicate the source
1.Python collection
A set is an unordered sequence of non repeating elements.
You can use braces {} or set() function to create a set. Note: to create an empty set, you must use set() instead of {}, because {} is used to create an empty dictionary.

Create format:
parame = {value01,value02,...} perhaps set(value)
The following is the sample code (the code can be found in Online Python 3 environment Running in:
>>> company = {'Baidu', 'ShowMeAI', 'google', 'ByteDance', 'ShowMeAI', 'Taobao', 'Tencent'} >>> print(company) # What is demonstrated here is the de duplication function {'Baidu', 'ShowMeAI', 'google', 'ByteDance', 'Taobao', 'Tencent'} >>> 'Baidu' in basket # Quickly determine whether an element is in a collection True >>> 'Meituan' in basket False
>>> # The operation between two sets is shown below ... >>> a = set('abracadabra') >>> b = set('alacazam') >>> a {'a', 'r', 'b', 'c', 'd'} >>> a - b # Elements contained in set a but not in set b {'r', 'd', 'b'} >>> a | b # All elements contained in set a or b {'a', 'c', 'r', 'd', 'b', 'm', 'z', 'l'} >>> a & b # Both sets a and b contain elements of {'a', 'c'} >>> a ^ b # Elements not included in both a and b {'r', 'd', 'b', 'm', 'z', 'l'}
Similar to list derivation, set derivation is also supported:
>>> a = {x for x in 'abracadabra' if x not in 'abc'} >>> a {'r', 'd'}
2. Basic operation of collection
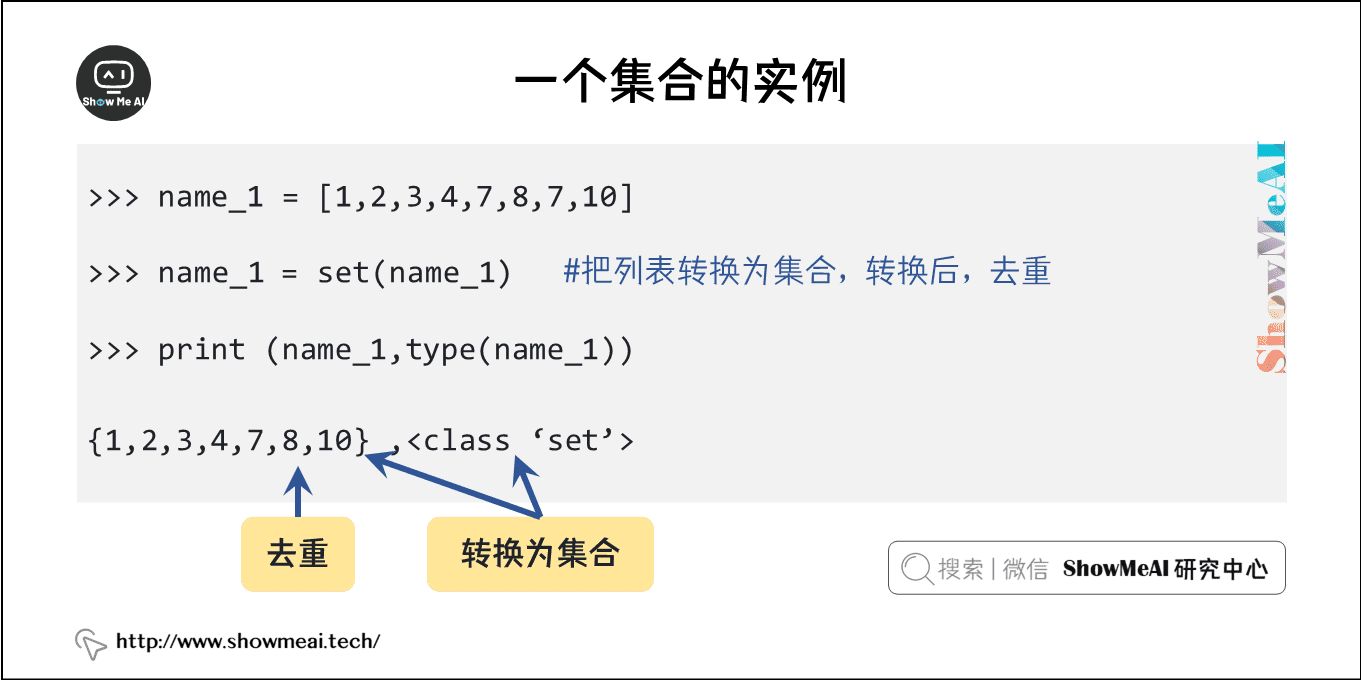
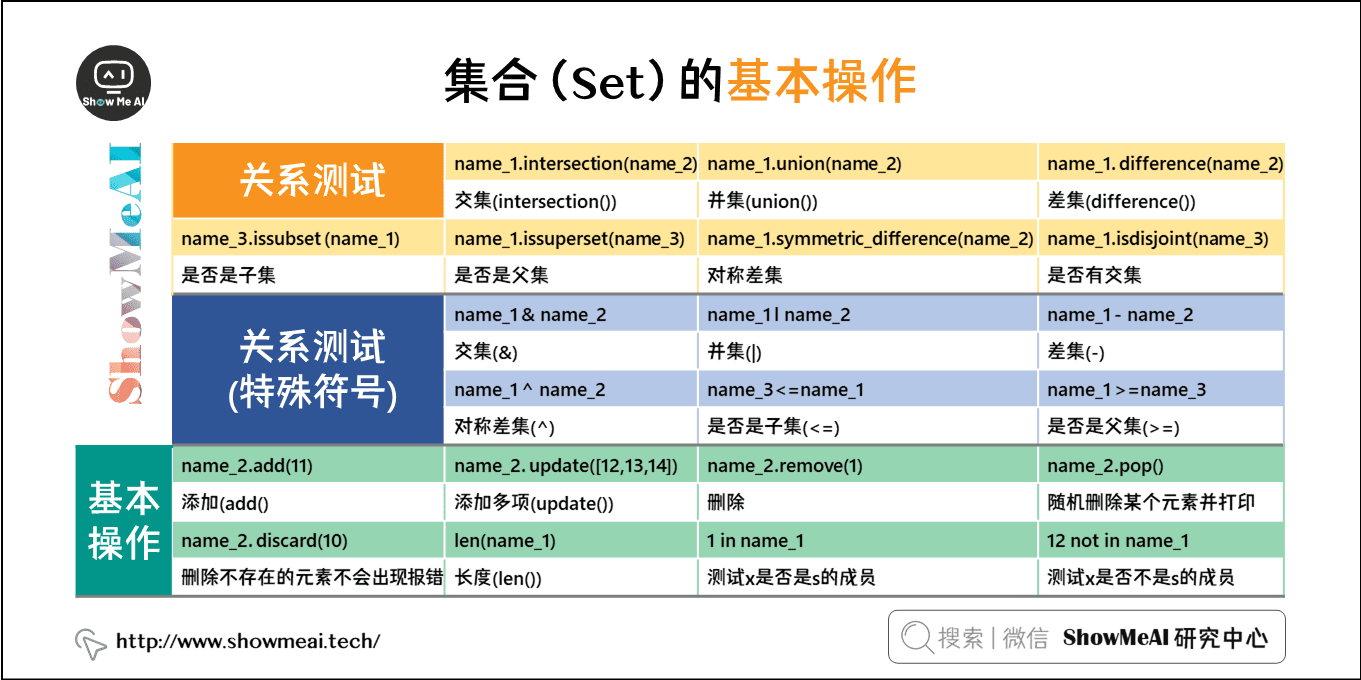
(1) Add element
The syntax format is as follows:
s.add( x )
Add the element x to the collection s. if the element already exists, no action will be taken.
>>> company = set(("Google", "ShowMeAI", "Taobao")) >>> company.add("Facebook") >>> print(company) {'Taobao', 'Facebook', 'Google', 'ShowMeAI'}
There is another method. You can also add elements, and the parameters can be lists, tuples, dictionaries, etc. the syntax format is as follows:
s.update( x )
x can have multiple, separated by commas. The following is the sample code (the code can be found in Online Python 3 environment Running in:
>>> company = set(("Google", "ShowMeAI", "Taobao")) >>> company.update({"Facebook", "LinkedIn"}) >>> print(company) {'LinkedIn', 'Google', 'ShowMeAI', 'Facebook', 'Taobao'} >>> company.update([1,4],[5,6]) >>> print(company) {1, 3, 4, 5, 6, 'Google', 'Taobao', 'Runoob'}
(2) Remove element
The syntax format is as follows:
s.remove( x )
Remove element x from collection s, and an error occurs if the element does not exist. The following is the sample code (the code can be found in Online Python 3 environment Running in:
>>> company = set(("Google", "ShowMeAI", "Taobao")) >>> company.remove("Taobao") >>> print(company) {'Google', 'ShowMeAI'} >>> company.remove("Facebook") # An error will occur if the does not exist Traceback (most recent call last): File "<stdin>", line 1, in <module> KeyError: 'Facebook'
In addition, another method is to remove the elements in the collection, and if the elements do not exist, no error will occur. The format is as follows:
s.discard( x )
>>> company = set(("Google", "ShowMeAI", "Taobao")) >>> company.discard("Facebook") # Does not exist, no error will occur >>> print(company) {'Taobao', 'Google', 'ShowMeAI'}
We can also set to randomly delete an element in the collection. The syntax format is as follows:
s.pop()
company = set(("Google", "ShowMeAI", "Taobao", "Facebook")) x = company.pop() print(x)
Output result:
ShowMeAI
The results of multiple tests are different.
The pop method of the set will arrange the set disorderly, and then delete the first element on the left of the disorderly arranged set.
(3) Calculate the number of collection elements
The syntax format is as follows:
len(s)
Calculate the number of set s elements.
company = set(("Google", "ShowMeAI", "Taobao", "Facebook")) print(len(company))
(4) Empty collection
The syntax format is as follows:
s.clear()
Empty collection s.
company = set(("Google", "ShowMeAI", "Taobao", "Facebook")) company.clear()
(5) Determine whether the element exists in the collection
The syntax format is as follows:
x in s
Judge whether the element x is in the set s, return True if it exists, and return False if it does not exist.
company = set(("Google", "ShowMeAI", "Taobao", "Facebook")) "Facebook" in company
(6) Complete list of collection built-in methods
method | describe |
---|---|
add() | Add elements to the collection |
clear() | Remove all elements from the collection |
copy() | Copy a collection |
difference() | Returns the difference set of multiple sets |
difference_update() | Removes an element from the collection that also exists in the specified collection. |
discard() | Deletes the specified element from the collection |
intersection() | Returns the intersection of sets |
intersection_update() | Returns the intersection of sets. |
isdisjoint() | Judge whether two collections contain the same elements. If not, return True; otherwise, return False. |
issubset() | Judge whether the specified set is a subset of the method parameter set. |
issuperset() | Judge whether the parameter set of the method is a subset of the specified set |
pop() | Randomly remove elements |
remove() | Removes the specified element |
symmetric_difference() | Returns a collection of non repeating elements in two collections. |
symmetric_difference_update() | Removes the same elements in the current set as in another specified set, and inserts different elements in another specified set into the current set. |
union() | Returns the union of two sets |
update() | Adding elements to a collection |
3. Video tutorial
Please click to station B to view the version of [bilingual subtitles]
https://www.bilibili.com/video/BV1yg411c7Nw
Data and code download
The code for this tutorial series can be found in github corresponding to ShowMeAI Download in, you can run in the local python environment. Babies who can surf the Internet scientifically can also directly use Google lab to run and learn through interactive operation!
The Python quick look-up table involved in this tutorial series can be downloaded and obtained at the following address:
Extended references
ShowMeAI related articles recommended
- Introduction to python
- python installation and environment configuration
- python basic syntax
- python basic data type
- python operator
- python conditional control and if statement
- python loop statement
- python while loop
- python for loop
- python break statement
- python continue statement
- python pass statement
- python string and operation
- python list
- python tuple
- python dictionary
- python collection
- python function
- python iterators and generators
- python data structure
- python module
- python file reading and writing
- python file and directory operation
- python error and exception handling
- python object oriented programming
- python namespace and scope
- python time and date
ShowMeAI series tutorial recommendations
- Illustrated Python Programming: a series of tutorials from getting started to mastering
- Graphic data analysis: a series of tutorials from introduction to mastery
- Fundamentals of graphic AI Mathematics: a series of tutorials from introduction to mastery
- Illustrated big data technology: a series of tutorials from introduction to mastery
