background
At present, the training of Tencent cloud self-developed products such as GTS academy, TCS, TBDS, TSF, TDSQL and TBASE requires the trainees to bring their own environment. During the training, the trainees use their own environment for practical exercises. The training environment of each product has different requirements for the number and configuration of CVM. A set of practical operation environment for product training is less than 4 sets, more than close to 20 sets, and the configuration of CVM is different. If students use manual purchase when preparing the environment, they need to spend more time preparing the environment, which is inefficient and cumbersome. Based on the current environment of GTS academy, this paper will introduce the function of automatic creation of CVM, and appendix the code of the creation machine related to the main training products for students to download and run by themselves.
Solution
Through the introduction of this article, even users who do not know programming can realize the function of creating CVM through code.
Get and create CVM program
For students with zero basic programming, they do not need to write their own programs. There is a corresponding program generation interface on Tencent cloud website, which can automatically obtain the code for automatically creating CVM.
stay New server Select the information to purchase CVM according to your needs (including billing mode, region, model, network, configuration and other information). In the third stage, "3. Confirm configuration information", you can see the option of "generate API Explorer best practice script". Click to get the program for creating CVM.
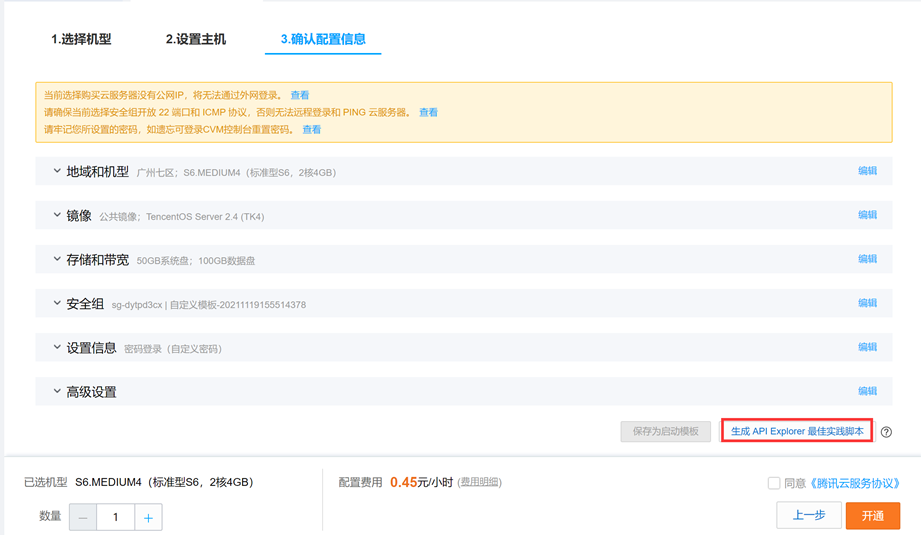
The following key configuration information of the current machine will be displayed on the left. After selecting the language of API script on the right, you can see the automatically generated program.
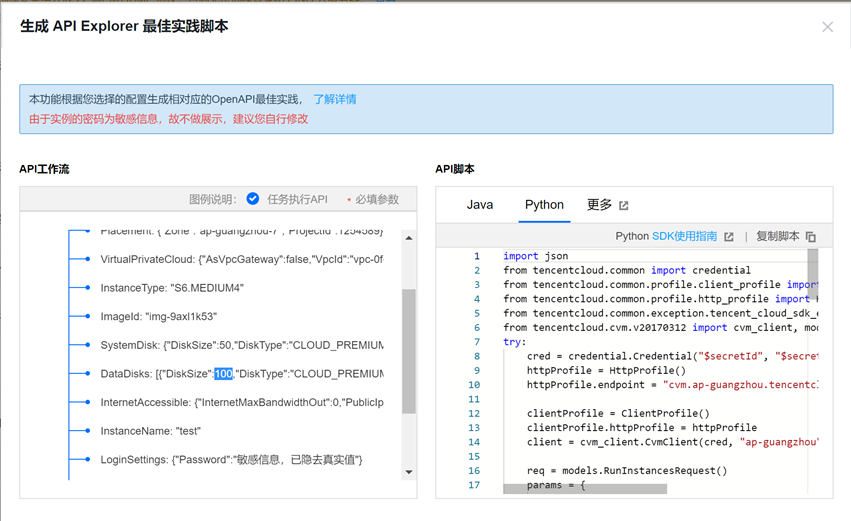
Run code
By adding "$secretId", "$secretKey" and server login password information to the obtained code, you can directly run the program on the machine with tencentcloud SDK Python installed to create CVM.
Key information acquisition
The secretId and secretKey information can be accessed directly: https://console.cloud.tencent.com/cam/capi Available
SDK installation
Please refer to the connection for the way to install tencentcloud SDK Python: https://cloud.tencent.com/document/sdk/Python Install Tencent cloud SDK directly according to the method of installing SDK.
Since the obtained code can only create machines with unified configuration, it needs to be modified to create multiple experimental environments with different configurations.
Code modification
Basic configuration according to requirements can realize automatic creation of multiple CVM s with different configurations
#!/usr/bin/env python # -*- coding: UTF-8 -*- # coding: utf8 # author: johnnyxsu # Create: 2022-2-17 import sys import json import time from tencentcloud.common import credential from tencentcloud.common.profile.client_profile import ClientProfile from tencentcloud.common.profile.http_profile import HttpProfile from tencentcloud.common.exception.tencent_cloud_sdk_exception import TencentCloudSDKException from tencentcloud.cvm.v20170312 import cvm_client, models # https://console.cloud.tencent.com/cam/capi Available SecretId = "" SecretKey = "" #Fill in the model information as required, and fill in as many units as required InstanceType = [ {"InstanceType": "SA3.2XLARGE16"}, {"InstanceType": "SA3.2XLARGE16"}, {"InstanceType": "SA3.2XLARGE16"}, {"InstanceType": "SA3.2XLARGE16"}, ] #The data disk information of the machine corresponds to InstanceType one by one DataDisks = [ [{"DiskSize": 100, "DiskType": "CLOUD_PREMIUM"}], [{"DiskSize": 100, "DiskType": "CLOUD_PREMIUM"}], [{"DiskSize": 100, "DiskType": "CLOUD_PREMIUM"}], [{"DiskSize": 100, "DiskType": "CLOUD_PREMIUM"}, {"DiskSize": 200, "DiskType": "CLOUD_PREMIUM"}], ] def loopCreateCVM(datas): cvms = list() print("Start creating CVM:") for data in datas: inData = dict() inData['projectId'] = data['projectId'] inData['VpcId'] = data['VpcId'] inData['SubnetId'] = data['SubnetId'] for i in range(len(InstanceType)): inData['InstanceType'] = InstanceType[i]['InstanceType'] inData['InstanceName'] = data['groupId'] + '-'+ InstanceType[i]['InstanceType'].split('.')[-1] + "-{}".format(i+1) inData['DataDisks'] = DataDisks[i] #print(inData) #inData = {"InstanceName": "", "projectId": 1222686, "VpcId": "vpc-ay2tio8z", "SubnetId": "subnet-1tgw7cos","InstanceType": "SA2.8XLARGE64", "DataDisks": []} msg = createCVM(inData) print(msg) retD = json.loads(msg) if 'InstanceIdSet' in retD and retD['InstanceIdSet']: cvms = cvms + retD['InstanceIdSet'] return cvms def createCVM(inData): # inData = {"groupId": "", "projectId": 1222686, "VpcId": "vpc-ay2tio8z", "SubnetId": "subnet-1tgw7cos", "InstanceType": "SA2.8XLARGE64", "DataDisks":[]} try: cred = credential.Credential(SecretId, SecretKey) httpProfile = HttpProfile() httpProfile.endpoint = "cvm.ap-guangzhou.tencentcloudapi.com" clientProfile = ClientProfile() clientProfile.httpProfile = httpProfile client = cvm_client.CvmClient(cred, "ap-guangzhou", clientProfile) req = models.RunInstancesRequest() params = { "InstanceChargeType": "POSTPAID_BY_HOUR", # PREPAID POSTPAID_BY_HOUR "InstanceChargePrepaid": { "Period": 1, "RenewFlag": "NOTIFY_AND_MANUAL_RENEW" }, "Placement": { "Zone": "ap-guangzhou-7", # Available area "ProjectId": inData['projectId'] # Project ID needs to be modified }, "VirtualPrivateCloud": { "AsVpcGateway": False, "VpcId": inData['VpcId'], # VPC needs to be modified "SubnetId": inData['SubnetId'], # The subnet needs to be modified "Ipv6AddressCount": 0 }, "InstanceType": inData['InstanceType'], # The model needs to be modified "ImageId": "img-hdt9xxkt", "SystemDisk": { "DiskSize": 100, ## System disk size "DiskType": "CLOUD_PREMIUM" }, "DataDisks": inData['DataDisks'], # Disk information needs to be modified "InternetAccessible": { "InternetMaxBandwidthOut": 0, "PublicIpAssigned": False }, "InstanceName": inData['InstanceName'], # Instance ID needs to be modified "LoginSettings": { "Password": "Tcdn@2007" }, "SecurityGroupIds": ["sg-5etqc5mt"], # Configure security groups as required "InstanceCount": 1, # Number of instances created "EnhancedService": { "SecurityService": {"Enabled": True}, "MonitorService": {"Enabled": True}, "AutomationService": {"Enabled": True} } } req.from_json_string(json.dumps(params)) resp = client.RunInstances(req) return resp.to_json_string() except TencentCloudSDKException as err: return err def batchcreateCVM(data): cvmslist = list() print("inputData:{}".format(json.dumps(data))) print("---------------------------------------------") cvms = loopCreateCVM([data]) cvmslist.append(cvms) print("---------------------------------------------") print("establish CVM List information:{}".format(json.dumps(cvmslist))) print("Wait 120 s After creating the virtual machine, start the query CVM IP information") time.sleep(120) describeCVM(cvmslist) return cvmslist def describeCVM(cvmslist): for cvms in cvmslist: try: cred = credential.Credential(SecretId, SecretKey) httpProfile = HttpProfile() httpProfile.endpoint = "cvm.tencentcloudapi.com" clientProfile = ClientProfile() clientProfile.httpProfile = httpProfile client = cvm_client.CvmClient(cred, "ap-guangzhou", clientProfile) req = models.DescribeInstancesRequest() params = { "InstanceIds": cvms } req.from_json_string(json.dumps(params)) #print(json.dumps(cvms)) resp = client.DescribeInstances(req) retD = json.loads(resp.to_json_string()) #print(resp.to_json_string()) if 'InstanceSet' in retD and retD['InstanceSet'] and 'TotalCount' in retD and retD['TotalCount'] > 0: for cvm in cvms: for ret in retD['InstanceSet']: if ret['InstanceId'] == cvm: print("{data[InstanceId]} {data[PrivateIpAddresses][0]}".format(data=ret)) break except TencentCloudSDKException as err: print(err) print("---------------------------------------------") if __name__ == '__main__': data = {"groupId": "TBASE", "projectId": 1254589, "VpcId": "vpc-lkj4qiln", "SubnetId": "subnet-1ymbb2sw"} ''' groupId: Machine name projectId: project ID,If not, it can be modified to 0 VpcId: establish CVM Corresponding VPC ID SubnetId: establish CVM Corresponding subnet ID,Subnets must be connected with Zone The information is corresponding ''' msg = batchcreateCVM(data) #cvmslist = [] #msg = describeCVM(cvmslist)