Recent projects need to generate two-dimensional codes, and decode to obtain information. jquery.qrcode.js generates two-dimensional codes to compare conveniently. Write a tutorial to share with you. Welcome to test the message, I will improve the code.
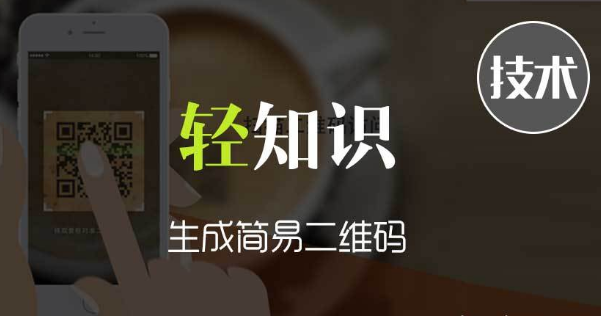
Now two-dimensional code is becoming more and more popular, pay attention to scanning two-dimensional code, pay attention to sweeping two-dimensional code, raffle sweeping two-dimensional code... In short, two-dimensional code is everywhere, so how to make a two-dimensional code? Let's discuss this issue today.
I. What is a two-dimensional code?
First, let's get to know what two-dimensional codes are. Baidu Encyclopedia explains as follows: 2-dimensional bar code is used to record data symbolic information with black-and-white graphics distributed in a plane (two-dimensional direction) according to a certain rule by a specific geometric figure; in code compilation, it ingeniously uses the concept of "0", "1" bit stream, which constitutes the logic basis of the computer, and uses several and "1" bitstreams. Binary corresponding geometric shapes represent numeric information of text, and automatic information processing is realized by automatic reading of image input equipment or photoelectric scanning equipment. It has some common features of barcode technology: each code system has its own specific character set; each character occupies a certain width; and has certain checking function. At the same time, it has the function of automatic recognition of information for different rows and processing of graphic rotation change points.
II. Specific Realization
How to make a two-dimensional code? There are many small partners in the server side to generate two-dimensional code, as a front-end control, Xiaowu today and everyone to discuss how to generate two-dimensional code in the front. In fact, Xiaowu has taken a shortcut, using a two-dimensional code plug-in jquery-qrcode, which can be obtained from https://github.com/jeromeetienne/jquery-qrcode, a free open source plug-in. Qrcode.js is the core class to realize two-dimensional code data calculation. jquery.qrcode.js encapsulates it in jQuery mode and uses it to realize graphics rendering. In fact, it is drawing (supporting canvas and table).
1. The main functions of support are:
text : " http://www.h5-share.com/"// Setting up two-dimensional code content render : "canvas",//Set up the rendering mode (there are two ways table and canvas, default is canvas) width : 256, //Set width height : 256, //Setting Height typeNumber : -1, //Computational model correctLevel : 0,//Error Correction Level background : "#ffffff ",//background color foreground : "#000000 "// Foreground Color
2. The way to use it is very simple.
You need to introduce jQuery and jquery.qrcode.js
①$('#qr_container').qrcode({render:"table",height:180,width:180,correctLevel:0,text:$('#qr_text').val()});
②$('#qr_container').qrcode({render:"canvas",height:180,width:180,correctLevel:0,text:$('#qr_text').val()});
When you execute the above examples separately, you can see that the two-dimensional code is drawn using canvas and a canvas node is output on the web page. But when we use tables, we will find that the two-dimensional codes actually use tables to draw the dots of each two-dimensional code, which leads to a lot of Dom elements on the web page.
Choose canvas, ie8 browser can not support. Choose table. Everything looks good. But in the actual use process, when the content of the two-dimensional code is large and the size of the two-dimensional code is small (such as 120 PX * 120 px), it will be difficult to recognize the generated two-dimensional code when it is rendered with table.
As a mobile developer, modern smartphones basically support canvas, so let's use canvas, but the new problem is that jquery.qrcode.js does not support Chinese by default.
This is related to the mechanism of js. jquery-qrcode is a library that uses charCodeAt() as its encoding conversion method. This method will acquire its Unicode encoding by default. Generally, decoders use UTF-8, ISO-8859-1 and other methods. English is no problem. If Chinese is used, Unicode is usually implemented in UTF-16 with 2 bits in length, while UTF-8 encoding is 3 bits. So the encoding and decoding of two-dimensional codes do not match.
The solution, of course, is to convert the string to UTF-8 before encoding the two-dimensional code. The specific code is as follows:
function utf16to8(str) { var out, i, len, c; out = ""; len = str.length; for(i = 0; i < len; i++) { c = str.charCodeAt(i); if ((c >= 0x0001) && (c <= 0x007F)) { out += str.charAt(i); } else if (c > 0x07FF) { out += String.fromCharCode(0xE0 | ((c >> 12) & 0x0F)); out += String.fromCharCode(0x80 | ((c >> 6) & 0x3F)); out += String.fromCharCode(0x80 | ((c >> 0) & 0x3F)); } else { out += String.fromCharCode(0xC0 | ((c >> 6) & 0x1F)); out += String.fromCharCode(0x80 | ((c >> 0) & 0x3F)); } } return out; }
Now the problem of generating two-dimensional codes in Chinese has been solved, but!!! How do you save your generated two-dimensional code in canvas? Simply translate the information in canvas into img pictures.
// Picture image extraction from canvas function convertCanvasToImage(canvas){ //The new Image object can be understood as DOM. var image = new Image(); //The canvas.toDataURL returns a list of Base64-encoded URLs image.src = canvas.toDataURL("image/png"); return image; }
Complete examples:
html:
Reference tutorial: http://www.h5-share.com/articles/201701/createqr.html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Generating two-dimensional codes</title> //Introducing library files <script src="js/jquery-2.1.4.min.js"></script> <script src="js/jquery.qrcode.min.js"></script> </head> <body> <h1>jquery.qrcode.js Generating Simple Two-Dimensional Codes</h1> <div> <label for="qr_text">URL:</label> <input id="qr_text" type="text" value="http://www.h5-share.com/" style="width:200px;" /> <button id="qr_btn" value="button">button</button> <br /> </div> <div id="qr_container" style="margin:auto; position:relative;"></div> <div id="imgDiv"></div> <script> $(document).ready(function(){ // Click to generate two-dimensional code $('#qr_btn').click(function(){ /*If the two-dimensional code has been generated, the img image and canvas of the two-dimensional code are deleted and regenerated; otherwise, the two-dimensional code is generated directly.*/ if($('#imgDiv:has(img)').length!=0){ $('#imgDiv img').remove(); $('canvas').remove(); createQr(); }else{ createQr(); } }); // Generating two-dimensional codes function createQr(){ document.createElement('canvas').getContext('2d'); var valText = $('#qr_text').val(); // Use regular expressions to determine whether the input is Chinese or not? var reg=/^[\u0391-\uFFE5]+$/; if(valText!=""&&!reg.test(valText)){ // If it's not Chinese, translate the input directly into two-dimensional code $('#qr_container').qrcode({render:"canvas",height:180, width:180,correctLevel:0,text:valText}); }else{ // In Chinese, the input content string is converted directly to UTF-8 and then to two-dimensional code. $('#qr_container').qrcode({render:"canvas",height:180, width:180,correctLevel:0,text:utf16to8(valText)}); } //Getting canvas objects in web pages var mycanvas1=document.getElementsByTagName('canvas')[0]; //Insert the converted img tag into html var img = convertCanvasToImage(mycanvas1); $('#imgDiv').append(img);//imgDiv indicates the container id you want to insert } // Character encoding function utf16to8(str) { var out, i, len, c; out = ""; len = str.length; for(i = 0; i < len; i++) { c = str.charCodeAt(i); if ((c >= 0x0001) && (c <= 0x007F)) { out += str.charAt(i); } else if (c > 0x07FF) { out += String.fromCharCode(0xE0 | ((c >> 12) & 0x0F)); out += String.fromCharCode(0x80 | ((c >> 6) & 0x3F)); out += String.fromCharCode(0x80 | ((c >> 0) & 0x3F)); } else { out += String.fromCharCode(0xC0 | ((c >> 6) & 0x1F)); out += String.fromCharCode(0x80 | ((c >> 0) & 0x3F)); } } return out; } //Extracting image from canvas function convertCanvasToImage(canvas) { //New Image Object, which can be understood as DOM var image = new Image(); // The canvas.toDataURL returns a list of Base64-encoded URLs // Specified format PNG image.src = canvas.toDataURL("image/png"); return image; } }); </script> </body> </html>
Want to experience more two-dimensional code generation case tutorials, quickly pick up the mobile phone to scan the following two-dimensional code of Wechat. Pay attention to the response tutorial and receive the front-end learning video.
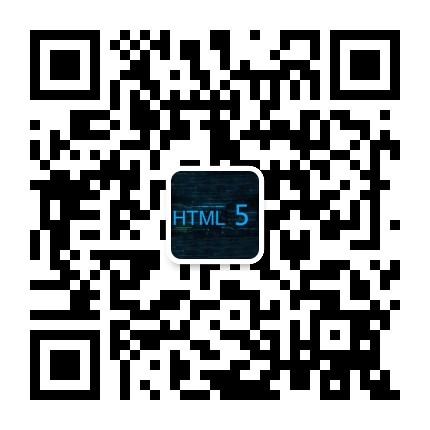