This week's physical condition is not good, so updates are limited. Anyway, it's not urgent for this time and a half, and less is more confusing, isn't it? I'm thinking about a problem now. When resume production is completed and delivered, Nima personnel should install a Node environment in the terminal for npm operation. Eh.......... No IP pain. ~No more thinking, just give a Png picture, after all, it doesn't matter. Some...
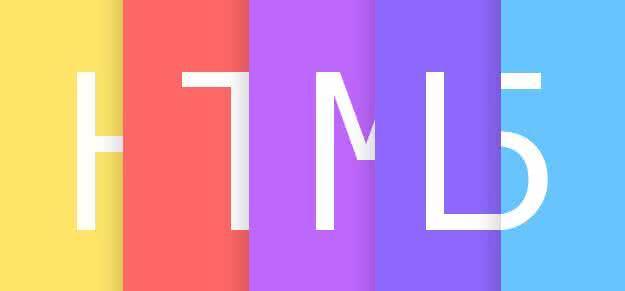
In bad condition, I don't have to press too much. I'm going to work on our project directly. Last week, we finished the development of home and project modules. Today, we are going to do the next work. First, let's take a look at today's technical points.
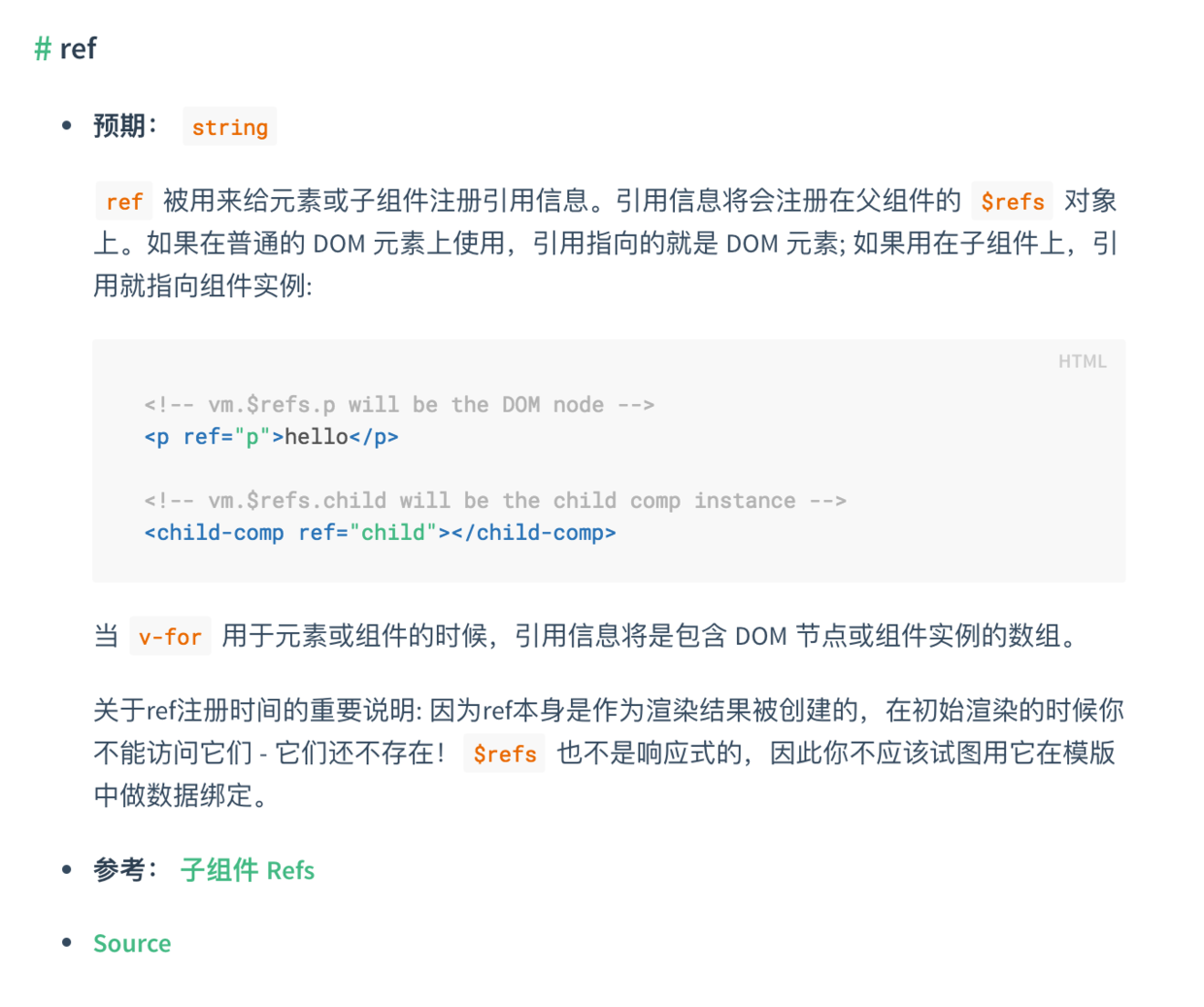
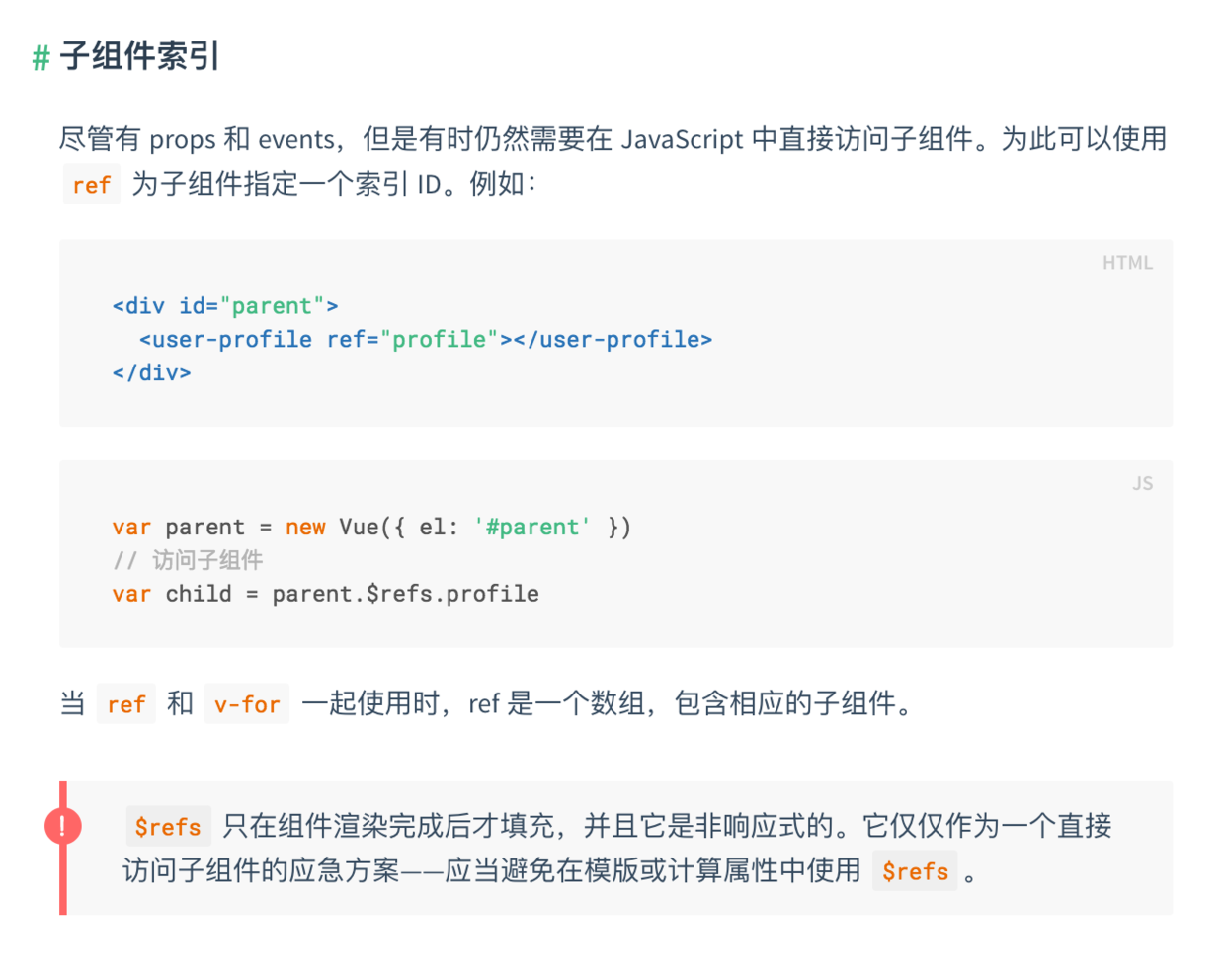
The reason for choosing Vue is that the company did not develop HTML5 last year. Let me take the lead in front-end development. I chose Vue as the framework for technology selection. So far I have not used react and angluar, but it is not important. When I was a technical secondary school, I was born in a class. It's easy to learn a language like front-end. To be honest, looking at official documents is the best way to learn new technology. But it does not rule out that some official documents are badly written or something.
Simple things are not too much to elaborate, directly encapsulating components:
Parent component github.vue
<template> <div> <div class="subtitle"> <h1>GitHub</h1> </div> <div class="github"> <div class="github-block"> <target name="coderZsq.project.oc" description="Use the SQExtension & SQTemplate to quickly build a project architecture, quickly use wheel components, and quickly integrate animated effects." href="https://github.com/coderZsq/coderZsq.project.oc" color="#438eff" language="Objective-C" star="113"></target> <target name="coderZsq.target.swift" description="Learn the advanced path of Swift Including Hybrid, RxSwift, Animations, SpriteKit, SceneKit, CoreData, LLDB, iOS, watchOS, tvOS etc." href="https://github.com/coderZsq/coderZsq.target.swift" color="#ffac45" language="Swift" star="4"></target> <target name="coderZsq.display.js" description="Through Vue.js to build a personal resume, hope that most companies to discuss cooperation." href="https://github.com/coderZsq/coderZsq.display.js" color="#2C3E50" language="Vue" star="0"></target> <target name="coderZsq.github.io" description="Through Hexo to build a personal blog, hoping to leave a good memory moments with you." href="https://github.com/coderZsq/coderZsq.github.io" color="#563D7C" language="CSS" star="1"></target> </div> </div> </div> </template>
Subcomponent target.vue
<template> <div class="target"> <div class="target-block"> <h2>{{name}}</h2> <p>{{description}}</p> <div class="download"> <a :href="href">Clone or download</a> </div> <div class="comment"> <div class="dot" ref="dot"></div> <div class="language"> {{language}} </div> <div class="star"> ★ {{star}} </div> </div> </div> </div> </template>
Most of the concepts here have been explained in previous articles. This time I want to talk about ref, which is the key word. Looking at the instructions in the official documents above, I can see that ref is used by javascript to access dom. But most people would think of using jquery. Yesterday I asked my colleagues who are front-end users. Now, are there people using jquery? He said that all the JSPS on the official website are used, with a mockery face. The tone of voice,
Because I personally hate using third-party things, so since Vue has this keyword, it's good to use it. ~ Actually, the real reason why jQuery is not suitable here is that Vue is a single-page web framework, so using jquery, whether to get class es or ids, is always repetitive in the process of cycling, and ref and scope avoid this problem.
Subcomponent target.vue
<script> export default { data() { return { } }, props: { href: String, name: String, description: String, color: String, language: String, star: String }, methods: { setDotColor: function() { this.$refs.dot.style.backgroundColor = this.color; } }, mounted: function() { this.setDotColor(); } } </script>
There are also points of knowledge here, methods are used to write functions, while mounted is the lifecycle of a Vue component similar to viewDidLoad, by which. $refs. dot. style. backgroundColor = this. color; css for dom can be set.
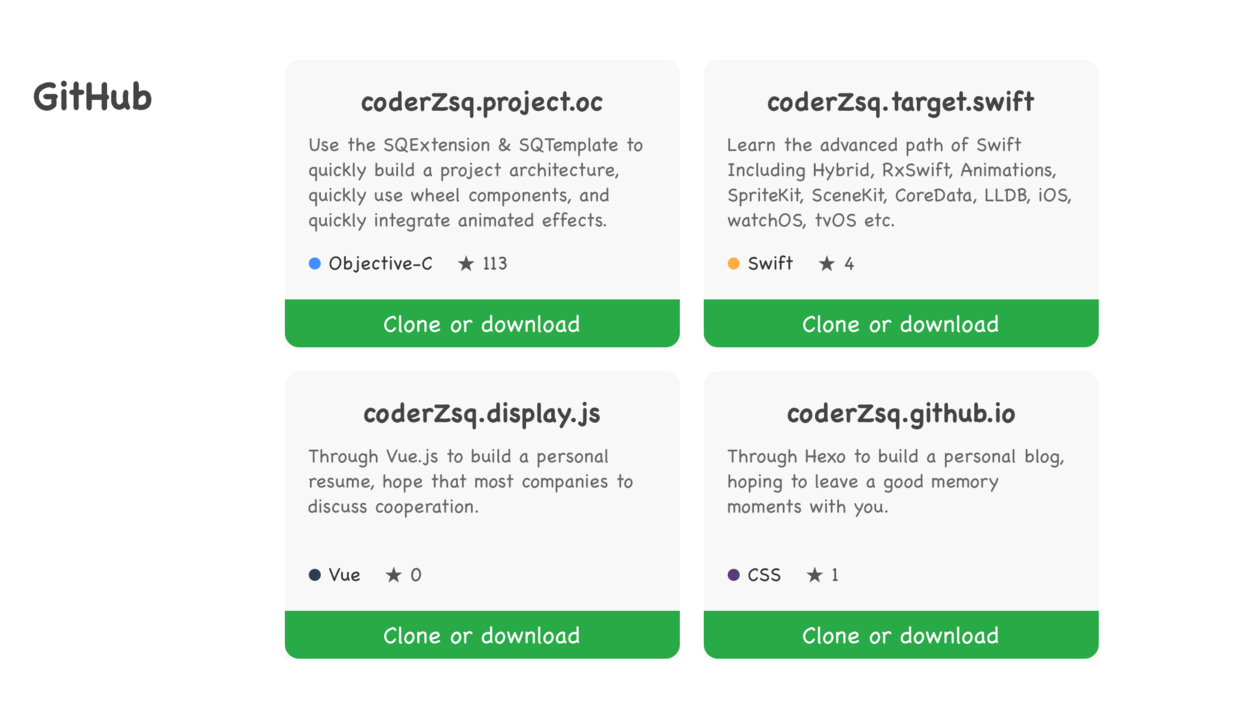
After completing the github module, we will implement the articles module. Here we need to understand a knowledge point:
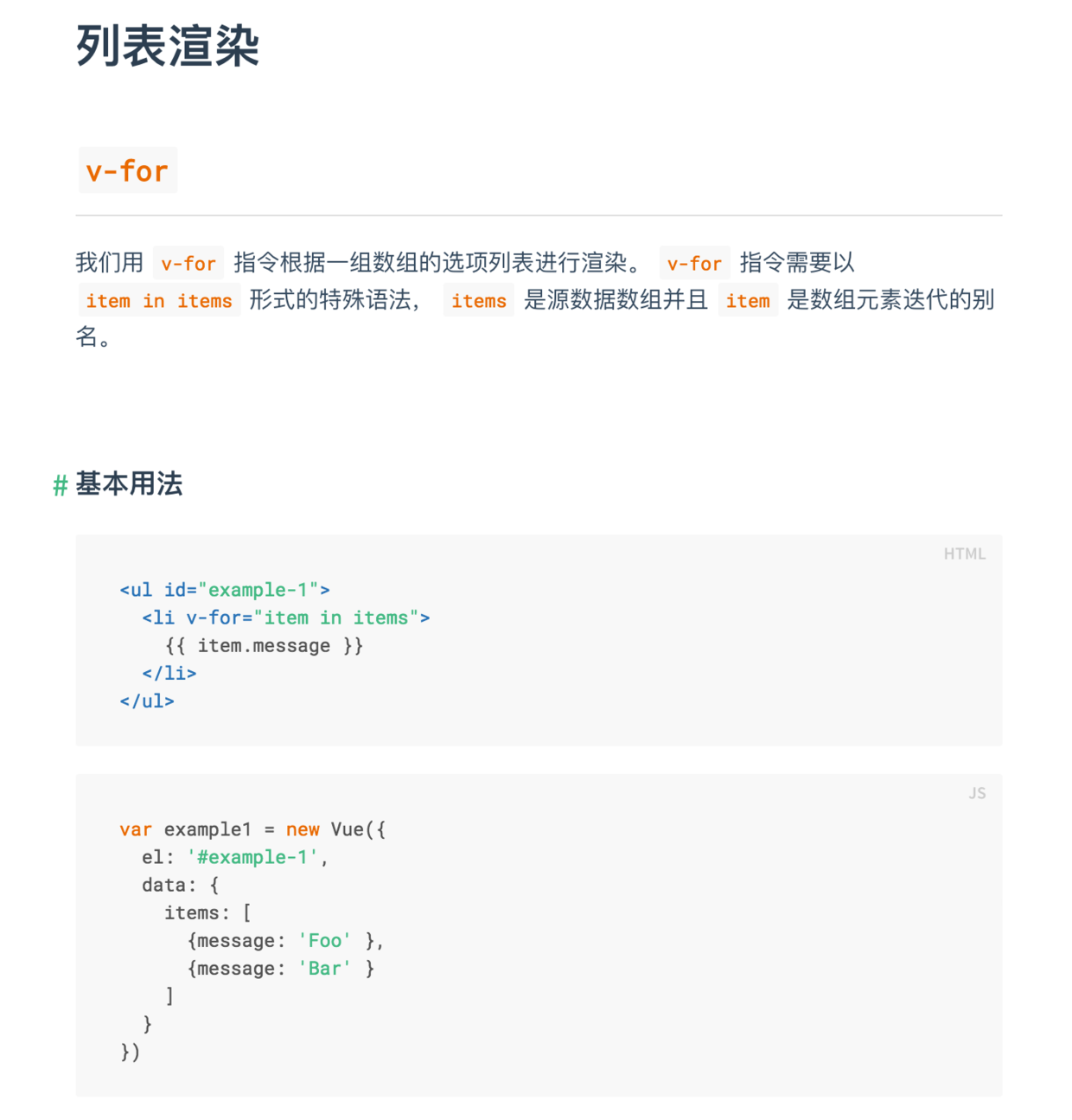
Understanding the basic usage of v-for, let's look at component communication:
articles.vue
<template> <div> <div class="subtitle"> <h1>Articles</h1> </div> <div class="articles"> <div class="articles-block"> <div v-for="item in items"> <column :name="item.name" :articles="item.articles"></column> </div> </div> </div> </div> </template>
<script> import column from './column' export default { data() { return { items: [{ name: "SQTemplate Column", articles: [{ name: "Hybird Building Zero Coupling Architecture MVC start", href: "http://www.jianshu.com/p/5a03995a6ce1" }, { name: "Hybird Build the back end Koa.js And excessive MVVM", href: "http://www.jianshu.com/p/846b9f181cb7" }, { name: "Hybird Build the front end Vue.js And upgrade to MVP", href: "http://www.jianshu.com/p/8d4a84e3ddaa" }, { name: "Hybird Routing Router Implementing Componentization", href: "http://www.jianshu.com/p/36314d0c0032" }, { name: "Hybird Establishment of Real-time Client Degradation Architecture", href: "http://www.jianshu.com/p/7054a694cfeb" }, { name: "iOS implement.py Script generation decoupling architecture", href: "http://www.jianshu.com/p/47d565bf200e" }, { name: "iOS implement.py Script generation UI Layer structure", href: "http://www.jianshu.com/p/d15379908582" }] } ... }, components: { column } }
This is the basic use of v-for. Data binding by default parameters in data is much simpler than model mapping in iOS. Note here that <column: name="item.name": articles="item.articles"> the input parameters have the following: the input reference type, but not: the input string.
column.vue
<template> <div class="column"> <div class="column-block"> <div class="column-list"> <div class="row" v-for="article in articles"> <a :href="article.href">{{article.name}}</a> </div> </div> <div class="column-title"> {{name}} </div> </div> </div> </template>
<script> export default { data() { return { } }, props: { articles: Object, name: String } } </script>
Here's a double v-for use. Note articles: Object, which is passed in as an object type instead of a String type.
... .column-list { height: 460px; overflow: scroll; } ...
Other css don't need to be read, as I've mentioned before. Here's an overflow: scroll setup that turns a normal div into a scrollView.
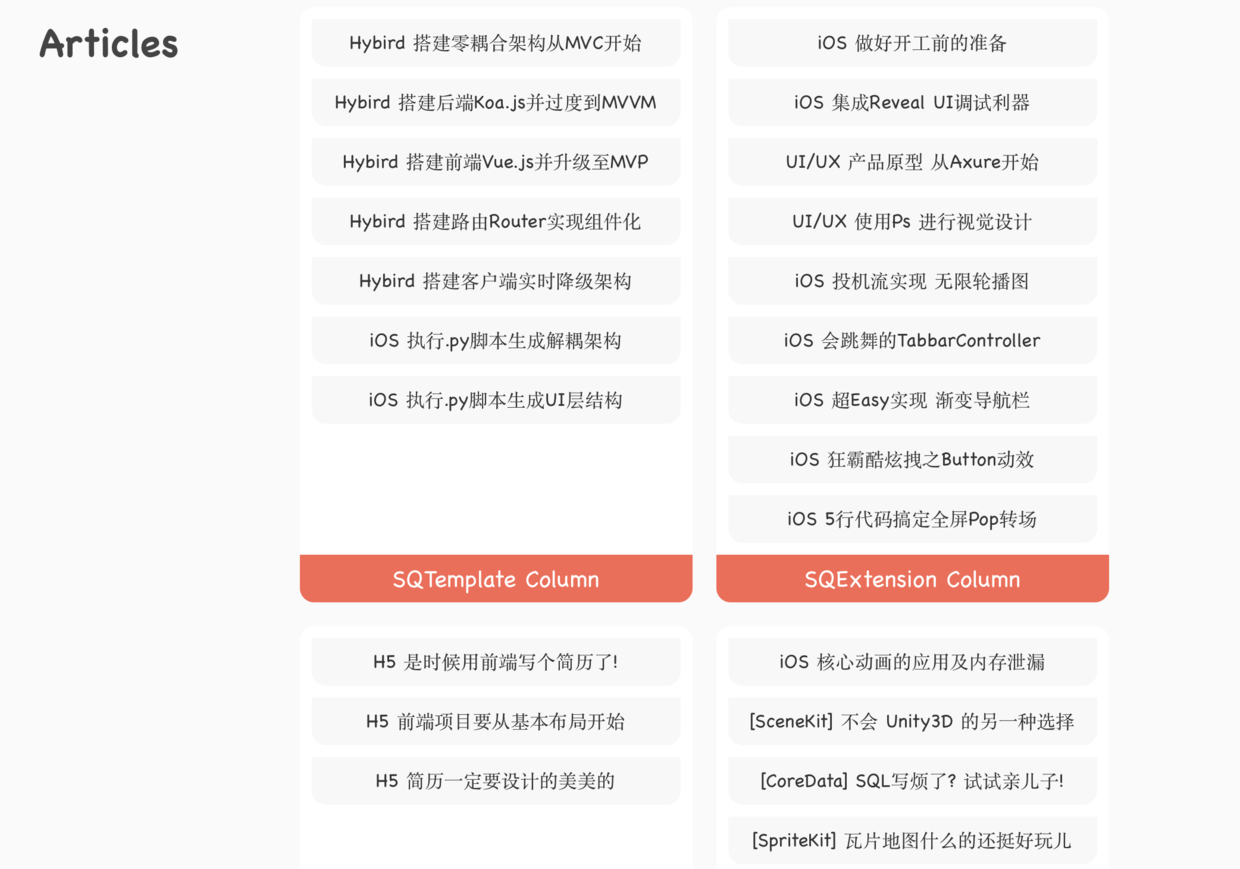
The effect is not too bad, and more articles will support the sliding up and down, the color is based on the color of the brief book. It's still pretty, well, let's look at the overall effect to end this kind of content.
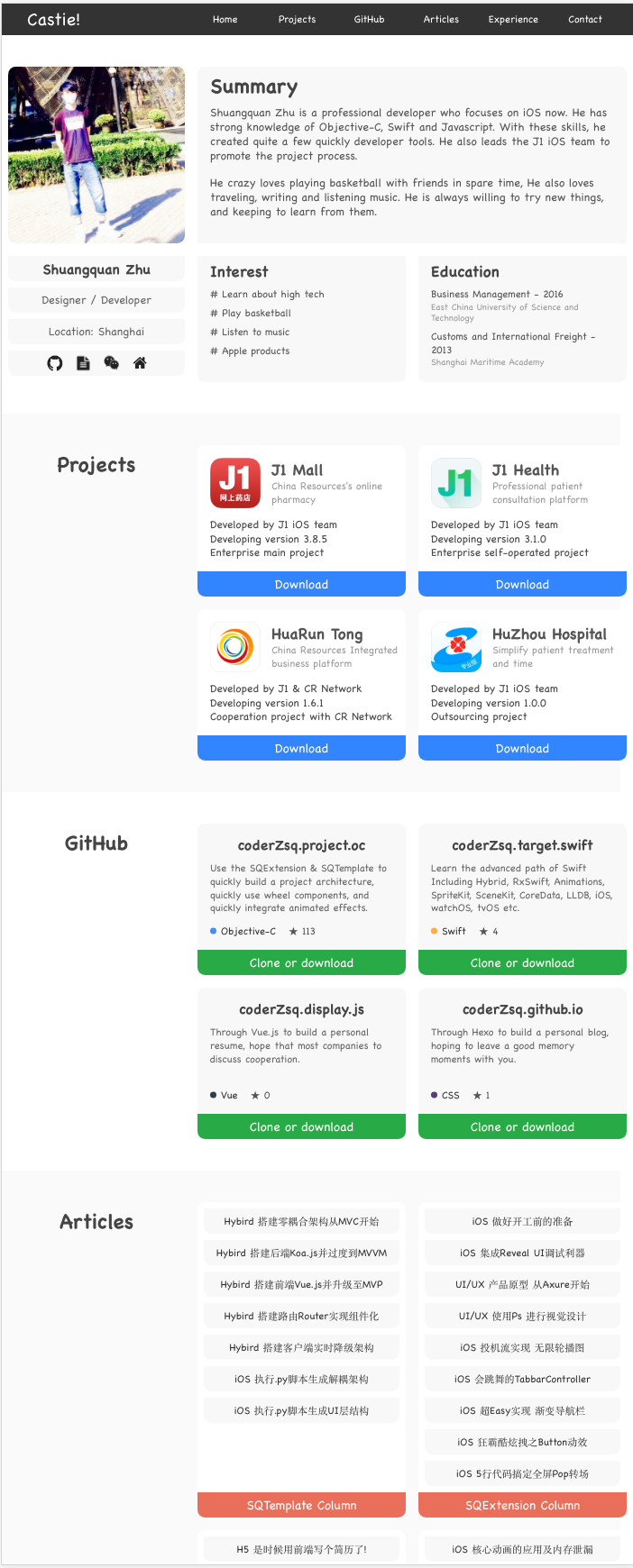
Okay, that's all for today. See you next week.
About:
