- Hotel Room Management System mysql Database Creation Statement
- oracle database creation statement of hotel room management system
- SQL Server database creation statement for hotel room management system
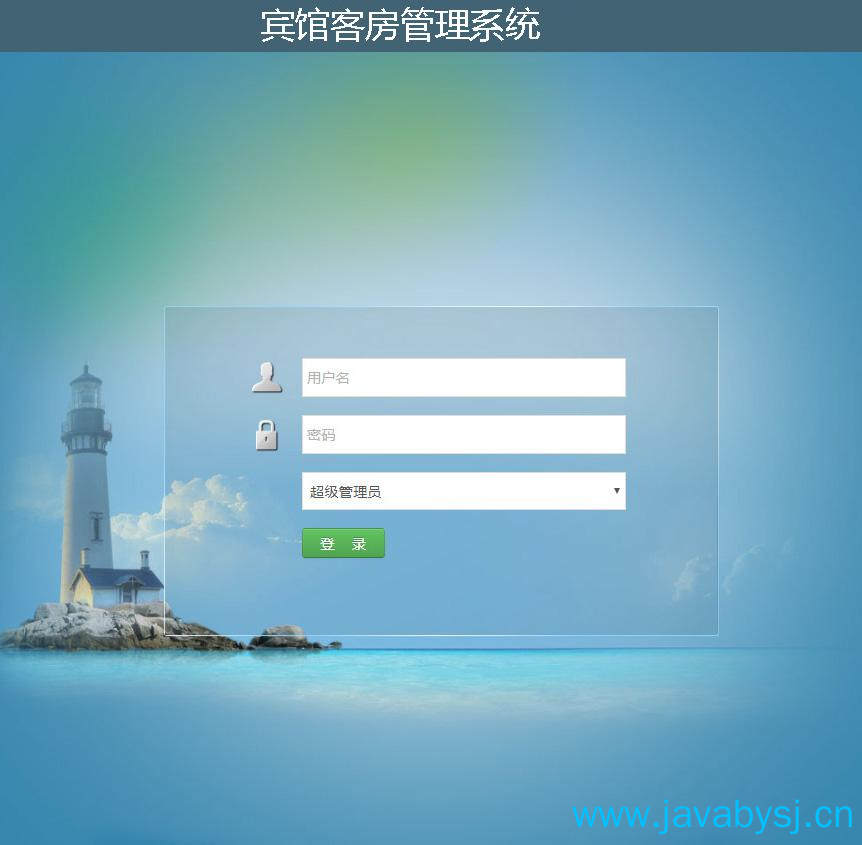
Hotel room management system mysql database version source code:
Super Administrator Table Creation Statement is as follows:
create table t_admin( id int primary key auto_increment comment 'Primary key', username varchar(100) comment 'Super Administrator Account', password varchar(100) comment 'Super Administrator Password' ) comment 'Super Administrator'; insert into t_admin(username,password) values('admin','123456');
The table creation statement is as follows:
create table t_pinglun_product( id int primary key auto_increment comment 'Primary key', teacherId int comment 'Teacher', kcName varchar(100) comment 'Course Name', pic varchar(100) comment 'picture', content varchar(100) comment 'Details', showDate datetime comment 'Course Date', status varchar(100) comment 'state' ) comment '';
The following statement is used to create the integral exchange product table:
create table t_jfdh( id int primary key auto_increment comment 'Primary key', jfName varchar(100) comment 'Integral Product Name', jfCost int comment 'Integral Quantity', jfPic varchar(100) comment 'Product Pictures' ) comment 'Integral Exchange Products';
The information table creation statement is as follows:
create table t_zx( id int primary key auto_increment comment 'Primary key', jfName varchar(100) comment 'Integral Product Name', jfCost int comment 'Integral Quantity', jfPic varchar(100) comment 'Product Pictures', customerId int comment 'I' ) comment 'information';
My message table creation statement is as follows:
create table t_wdxx( id int primary key auto_increment comment 'Primary key', title varchar(100) comment 'Title', pic varchar(100) comment 'picture', content varchar(100) comment 'content', zan int comment 'Fabulous', insertDate datetime comment 'Date of publication', nologin varchar(100) comment 'Visibility of tourists', bkId int comment '', productId int comment 'Comment information' ) comment 'My news';
The customer table creation statement is as follows:
create table t_customer( id int primary key auto_increment comment 'Primary key', customerId int comment 'Commentator', content varchar(100) comment 'Comments', insertDate datetime comment 'Comment Date', xj varchar(100) comment 'Star class', pic varchar(100) comment '', bkName varchar(100) comment 'Category name', title varchar(100) comment 'Title', content varchar(100) comment 'content', pic varchar(100) comment 'picture' ) comment 'Customer';
Classification table creation statement is as follows:
create table t_bk( id int primary key auto_increment comment 'Primary key', showDate varchar(100) comment '' ) comment 'classification';
Course schedule creation statements are as follows:
create table t_kc( id int primary key auto_increment comment 'Primary key', username varchar(100) comment 'Account number', password varchar(100) comment 'Password', customerName varchar(100) comment 'Full name', sex varchar(100) comment 'Gender', address varchar(100) comment 'address', phone varchar(100) comment 'Mobile phone' ) comment 'curriculum';
The following statement is used to create the integral exchange product table:
create table t_jfdh( id int primary key auto_increment comment 'Primary key', account int comment 'account', jf int comment 'integral', headPic varchar(100) comment 'Head portrait' ) comment 'Integral Exchange Products';
Hotel room management system oracle database version source code:
Super Administrator Table Creation Statement is as follows:
create table t_admin( id integer, username varchar(100), password varchar(100) ); insert into t_admin(id,username,password) values(1,'admin','123456'); --Super Administrator Field Annotated comment on column t_admin.id is 'Primary key'; comment on column t_admin.username is 'Super Administrator Account'; comment on column t_admin.password is 'Super Administrator Password'; --Super Administrator Table Annotated comment on table t_admin is 'Super Administrator';
The table creation statement is as follows:
create table t_pinglun_product( id integer, teacherId int, kcName varchar(100), pic varchar(100), content varchar(100), showDate datetime, status varchar(100) ); --Field annotation comment on column t_pinglun_product.id is 'Primary key'; comment on column t_pinglun_product.teacherId is 'Teacher'; comment on column t_pinglun_product.kcName is 'Course Name'; comment on column t_pinglun_product.pic is 'picture'; comment on column t_pinglun_product.content is 'Details'; comment on column t_pinglun_product.showDate is 'Course Date'; comment on column t_pinglun_product.status is 'state'; --Notes on tables comment on table t_pinglun_product is '';
The following statement is used to create the integral exchange product table:
create table t_jfdh( id integer, jfName varchar(100), jfCost int, jfPic varchar(100) ); --Annotation of Integral Exchange Product Field comment on column t_jfdh.id is 'Primary key'; comment on column t_jfdh.jfName is 'Integral Product Name'; comment on column t_jfdh.jfCost is 'Integral Quantity'; comment on column t_jfdh.jfPic is 'Product Pictures'; --Notes on the Points Exchange Product List comment on table t_jfdh is 'Integral Exchange Products';
The information table creation statement is as follows:
create table t_zx( id integer, jfName varchar(100), jfCost int, jfPic varchar(100), customerId int ); --Annotation of Information Fields comment on column t_zx.id is 'Primary key'; comment on column t_zx.jfName is 'Integral Product Name'; comment on column t_zx.jfCost is 'Integral Quantity'; comment on column t_zx.jfPic is 'Product Pictures'; comment on column t_zx.customerId is 'I'; --Notes to Information Sheet comment on table t_zx is 'information';
My message table creation statement is as follows:
create table t_wdxx( id integer, title varchar(100), pic varchar(100), content varchar(100), zan int, insertDate datetime, nologin varchar(100), bkId int, productId int ); --Annotate my message field comment on column t_wdxx.id is 'Primary key'; comment on column t_wdxx.title is 'Title'; comment on column t_wdxx.pic is 'picture'; comment on column t_wdxx.content is 'content'; comment on column t_wdxx.zan is 'Fabulous'; comment on column t_wdxx.insertDate is 'Date of publication'; comment on column t_wdxx.nologin is 'Visibility of tourists'; comment on column t_wdxx.bkId is ''; comment on column t_wdxx.productId is 'Comment information'; --Annotate my message sheet comment on table t_wdxx is 'My news';
The customer table creation statement is as follows:
create table t_customer( id integer, customerId int, content varchar(100), insertDate datetime, xj varchar(100), pic varchar(100), bkName varchar(100), title varchar(100), content varchar(100), pic varchar(100) ); --Customer field annotated comment on column t_customer.id is 'Primary key'; comment on column t_customer.customerId is 'Commentator'; comment on column t_customer.content is 'Comments'; comment on column t_customer.insertDate is 'Comment Date'; comment on column t_customer.xj is 'Star class'; comment on column t_customer.pic is ''; comment on column t_customer.bkName is 'Category name'; comment on column t_customer.title is 'Title'; comment on column t_customer.content is 'content'; comment on column t_customer.pic is 'picture'; --Annotation of Customer List comment on table t_customer is 'Customer';
Classification table creation statement is as follows:
create table t_bk( id integer, showDate varchar(100) ); --Annotation of Classification Fields comment on column t_bk.id is 'Primary key'; comment on column t_bk.showDate is ''; --Annotation of Classification Table comment on table t_bk is 'classification';
Course schedule creation statements are as follows:
create table t_kc( id integer, username varchar(100), password varchar(100), customerName varchar(100), sex varchar(100), address varchar(100), phone varchar(100) ); --Annotation of Course Fields comment on column t_kc.id is 'Primary key'; comment on column t_kc.username is 'Account number'; comment on column t_kc.password is 'Password'; comment on column t_kc.customerName is 'Full name'; comment on column t_kc.sex is 'Gender'; comment on column t_kc.address is 'address'; comment on column t_kc.phone is 'Mobile phone'; --Annotation of Course Schedule comment on table t_kc is 'curriculum';
The following statement is used to create the integral exchange product table:
create table t_jfdh( id integer, account int, jf int, headPic varchar(100) ); --Annotation of Integral Exchange Product Field comment on column t_jfdh.id is 'Primary key'; comment on column t_jfdh.account is 'account'; comment on column t_jfdh.jf is 'integral'; comment on column t_jfdh.headPic is 'Head portrait'; --Notes on the Points Exchange Product List comment on table t_jfdh is 'Integral Exchange Products';
oracle is unique, and the corresponding sequence is as follows:
create sequence s_t_pinglun_product; create sequence s_t_jfdh; create sequence s_t_zx; create sequence s_t_wdxx; create sequence s_t_customer; create sequence s_t_bk; create sequence s_t_kc; create sequence s_t_jfdh;
Hotel room management system SQL Server database version source code:
Super Administrator Table Creation Statement is as follows:
--Super Administrator create table t_admin( id int identity(1,1) primary key not null,--Primary key username varchar(100),--Super Administrator Account password varchar(100)--Super Administrator Password ); insert into t_admin(username,password) values('admin','123456');
The table creation statement is as follows:
--Notes to tables create table t_pinglun_product( id int identity(1,1) primary key not null,--Primary key teacherId int,--Teacher kcName varchar(100),--Course Name pic varchar(100),--picture content varchar(100),--Details showDate datetime,--Course Date status varchar(100)--state );
The following statement is used to create the integral exchange product table:
--Notes to the Product List of Integral Exchange create table t_jfdh( id int identity(1,1) primary key not null,--Primary key jfName varchar(100),--Integral Product Name jfCost int,--Integral Quantity jfPic varchar(100)--Product Pictures );
The information table creation statement is as follows:
--Information sheet notes create table t_zx( id int identity(1,1) primary key not null,--Primary key jfName varchar(100),--Integral Product Name jfCost int,--Integral Quantity jfPic varchar(100),--Product Pictures customerId int--I );
My message table creation statement is as follows:
--My Message Table Comments create table t_wdxx( id int identity(1,1) primary key not null,--Primary key title varchar(100),--Title pic varchar(100),--picture content varchar(100),--content zan int,--Fabulous insertDate datetime,--Date of publication nologin varchar(100),--Visibility of tourists bkId int,-- productId int--Comment information );
The customer table creation statement is as follows:
--Customer table annotations create table t_customer( id int identity(1,1) primary key not null,--Primary key customerId int,--Commentator content varchar(100),--Comments insertDate datetime,--Comment Date xj varchar(100),--Star class pic varchar(100),-- bkName varchar(100),--Category name title varchar(100),--Title content varchar(100),--content pic varchar(100)--picture );
Classification table creation statement is as follows:
--Annotation of Classification Table create table t_bk( id int identity(1,1) primary key not null,--Primary key showDate varchar(100)-- );
Course schedule creation statements are as follows:
--Annotation of Course Schedule create table t_kc( id int identity(1,1) primary key not null,--Primary key username varchar(100),--Account number password varchar(100),--Password customerName varchar(100),--Full name sex varchar(100),--Gender address varchar(100),--address phone varchar(100)--Mobile phone );
The following statement is used to create the integral exchange product table:
--Notes to the Product List of Integral Exchange create table t_jfdh( id int identity(1,1) primary key not null,--Primary key account int,--account jf int,--integral headPic varchar(100)--Head portrait );