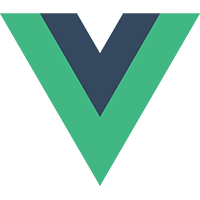
Vuex
Why do vuex modules appear? When there is more code in your project, it is difficult to distinguish maintenance. At this time, the module function of vuex is reflected.
Then let's start!
What is the module?
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, // You can add multiple modules in the following properties. For example, moduleOne module and moduleTwo module. modules: { moduleOne:{}, moduleTwo:{} } })
2, Add state in the module
You can write the state object directly in the module.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, modules: { moduleOne:{ state:{ moduleOnevalue:'1' } }, moduleTwo:{ state:{ moduleTwovalue:'0' } } } })
We refer to it in the page. We can directly find the corresponding module return value or use the mapState method call.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> </div> </template> <script> import {mapState} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ // Namespace is used here return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }) }, methods: { }, mounted() { }, } </script>

3, Add mutations to the module
We add the mutations attribute to the two modules and define the methods with the same name. Let's take a look at the page first.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, modules: { moduleOne:{ state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } } }, moduleTwo:{ state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } } } } })
Reference it on the page
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }) }, methods: { ...mapMutations(['updateValue']) }, mounted() { this.updateValue('I am the changed value: 1') }, } </script>
We see that the values of both modules have been changed. Why? Because VueX by default, the changes in each module are in the global namespace. Then we certainly don't want this. If the method names in the two modules are different, of course, this will not happen, but how can this be avoided?
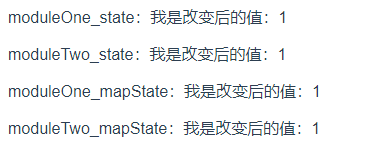
We need to define an attribute named as true.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, modules: { moduleOne:{ namespaced:true, //It is defined as true in each module to avoid duplicate method names state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } } }, moduleTwo:{ namespaced:true, state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } } } } })
It needs to be called in the page using a method of the namespace.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']) }, mounted() { this['moduleOne/updateValue']('I am the changed value: 1'); this['moduleTwo/updateValue']('I am the changed value: 0'); }, } </script>
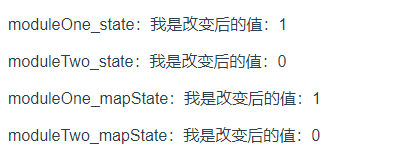
4, Add getters to the module
Named also works in getters. Next, we define methods with the same name in the two modules.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, modules: { moduleOne:{ namespaced:true, state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } }, getters:{ valuePlus(state){ return state.moduleOnevalue+'1' } } }, moduleTwo:{ namespaced:true, state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } }, getters:{ valuePlus(state){ return state.moduleTwovalue+'0' } } } } })
View the effect on the page reference.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> <p>moduleOne_mapGetters: {{OnevaluePlus}}</p> <p>moduleTwo_mapGetters: {{TwovaluePlus}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }), ...mapGetters({ OnevaluePlus:'moduleOne/valuePlus', TwovaluePlus:'moduleTwo/valuePlus' }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']) }, mounted() { // this['moduleOne/updateValue '] (' I am the changed value: 1 '); // this['moduleTwo/updateValue '] (' I am the changed value: 0 '); }, } </script>

We can also obtain global variables. The third parameter is to obtain the parameters of global variables.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, modules: { moduleOne:{ namespaced:true, state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } }, getters:{ valuePlus(state){ return state.moduleOnevalue+'1' }, globalValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.global } } }, moduleTwo:{ namespaced:true, state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } }, getters:{ valuePlus(state){ return state.moduleTwovalue+'0' }, globalValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.global } } } } })
View on the page.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> <p>moduleOne_mapGetters: {{OnevaluePlus}}</p> <p>moduleTwo_mapGetters: {{TwovaluePlus}}</p> <p>moduleOne_mapGetters_global: {{OneglobalValue}}</p> <p>moduleTwo_mapGetters_global: {{TwoglobalValue}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }), ...mapGetters({ OnevaluePlus:'moduleOne/valuePlus', TwovaluePlus:'moduleTwo/valuePlus', OneglobalValue:'moduleOne/globalValuePlus', TwoglobalValue:'moduleTwo/globalValuePlus' }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']) }, mounted() { // this['moduleOne/updateValue '] (' I am the changed value: 1 '); // this['moduleTwo/updateValue '] (' I am the changed value: 0 '); }, } </script>

You can also get variables of other modules.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, modules: { moduleOne:{ namespaced:true, state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } }, getters:{ valuePlus(state){ return state.moduleOnevalue+'1' }, globalValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.moduleTwo.moduleTwovalue }, } }, moduleTwo:{ namespaced:true, state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } }, getters:{ valuePlus(state){ return state.moduleTwovalue+'0' }, globalValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.moduleOne.moduleOnevalue }, } } } })
View on the page.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> <p>moduleOne_mapGetters: {{OnevaluePlus}}</p> <p>moduleTwo_mapGetters: {{TwovaluePlus}}</p> <p>moduleOne_mapGetters_global: {{OneglobalValue}}</p> <p>moduleTwo_mapGetters_global: {{TwoglobalValue}}</p> <p>moduleOne_mapGetters_other: {{OneotherValue}}</p> <p>moduleTwo_mapGetters_other: {{TwootherValue}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }), ...mapGetters({ OnevaluePlus:'moduleOne/valuePlus', TwovaluePlus:'moduleTwo/valuePlus', OneglobalValue:'moduleOne/globalValuePlus', TwoglobalValue:'moduleTwo/globalValuePlus', OneotherValue:'moduleOne/otherValuePlus', TwootherValue:'moduleTwo/otherValuePlus' }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']) }, mounted() { // this['moduleOne/updateValue '] (' I am the changed value: 1 '); // this['moduleTwo/updateValue '] (' I am the changed value: 0 '); }, } </script>

5, Add actions to the module
The method in the actions object has a parameter object ctx. There are {state, commit and rootstate}. Let's expand it directly here. Actions will only submit the changes in the local module by default. If you need to submit global or other modules, you need to add {root:true} to the third parameter of the commit method.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, modules: { moduleOne:{ namespaced:true, state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } }, getters:{ valuePlus(state){ return state.moduleOnevalue+'1' }, globalValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.moduleTwo.moduleTwovalue }, }, actions:{ timeOut({state,commit,rootState}){ setTimeout(()=>{ commit('updateValue','I am asynchronously changing the value: 1') },3000) } } }, moduleTwo:{ namespaced:true, state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } }, getters:{ valuePlus(state){ return state.moduleTwovalue+'0' }, globalValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.moduleOne.moduleOnevalue }, } } } })
Page reference.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> <p>moduleOne_mapGetters: {{OnevaluePlus}}</p> <p>moduleTwo_mapGetters: {{TwovaluePlus}}</p> <p>moduleOne_mapGetters_global: {{OneglobalValue}}</p> <p>moduleTwo_mapGetters_global: {{TwoglobalValue}}</p> <p>moduleOne_mapGetters_other: {{OneotherValue}}</p> <p>moduleTwo_mapGetters_other: {{TwootherValue}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }), ...mapGetters({ OnevaluePlus:'moduleOne/valuePlus', TwovaluePlus:'moduleTwo/valuePlus', OneglobalValue:'moduleOne/globalValuePlus', TwoglobalValue:'moduleTwo/globalValuePlus', OneotherValue:'moduleOne/otherValuePlus', TwootherValue:'moduleTwo/otherValuePlus' }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']), ...mapActions(['moduleOne/timeOut']) }, mounted() { // this['moduleOne/updateValue '] (' I am the changed value: 1 '); // this['moduleTwo/updateValue '] (' I am the changed value: 0 '); this['moduleOne/timeOut'](); }, } </script>
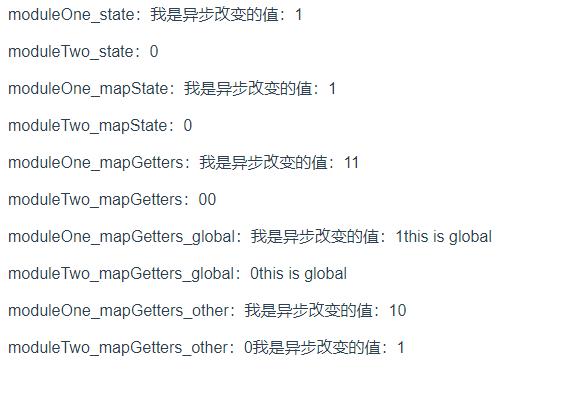
Let's look at how to submit global or other module changes.
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, mutations:{ mode(state,data){ state.global=data } }, modules: { moduleOne:{ namespaced:true, state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } }, getters:{ valuePlus(state){ return state.moduleOnevalue+'1' }, globalValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.moduleTwo.moduleTwovalue }, }, actions:{ timeOut({state,commit,rootState}){ setTimeout(()=>{ commit('updateValue','I am asynchronously changing the value: 1') },3000) }, globaltimeOut({commit}){ setTimeout(()=>{ commit('mode','I changed global Value of',{root:true}) },3000) } } }, moduleTwo:{ namespaced:true, state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } }, getters:{ valuePlus(state){ return state.moduleTwovalue+'0' }, globalValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.moduleOne.moduleOnevalue }, } } } })
Page reference.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> <p>moduleOne_mapGetters: {{OnevaluePlus}}</p> <p>moduleTwo_mapGetters: {{TwovaluePlus}}</p> <p>moduleOne_mapGetters_global: {{OneglobalValue}}</p> <p>moduleTwo_mapGetters_global: {{TwoglobalValue}}</p> <p>moduleOne_mapGetters_other: {{OneotherValue}}</p> <p>moduleTwo_mapGetters_other: {{TwootherValue}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }), ...mapGetters({ OnevaluePlus:'moduleOne/valuePlus', TwovaluePlus:'moduleTwo/valuePlus', OneglobalValue:'moduleOne/globalValuePlus', TwoglobalValue:'moduleTwo/globalValuePlus', OneotherValue:'moduleOne/otherValuePlus', TwootherValue:'moduleTwo/otherValuePlus' }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']), ...mapActions(['moduleOne/timeOut','moduleOne/globaltimeOut']) }, mounted() { // this['moduleOne/updateValue '] (' I am the changed value: 1 '); // this['moduleTwo/updateValue '] (' I am the changed value: 0 '); // this['moduleOne/timeOut'](); this['moduleOne/globaltimeOut'](); }, } </script>

What about submitting other modules?
/* eslint-disable no-unused-vars */ import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state:{ global:'this is global' }, mutations:{ mode(state,data){ state.global=data } }, modules: { moduleOne:{ namespaced:true, state:{ moduleOnevalue:'1' }, mutations:{ updateValue(state,value){ state.moduleOnevalue=value } }, getters:{ valuePlus(state){ return state.moduleOnevalue+'1' }, globalValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleOnevalue+rootState.moduleTwo.moduleTwovalue }, }, actions:{ timeOut({state,commit,rootState}){ setTimeout(()=>{ commit('updateValue','I am asynchronously changing the value: 1') },3000) }, globaltimeOut({commit}){ setTimeout(()=>{ commit('mode','I changed global Value of',{root:true}) },3000) }, othertimeOut({commit}){ setTimeout(()=>{ commit('moduleTwo/updateValue','I changed moduleTwo Value of',{root:true}) },3000) } } }, moduleTwo:{ namespaced:true, state:{ moduleTwovalue:'0' }, mutations:{ updateValue(state,value){ state.moduleTwovalue=value } }, getters:{ valuePlus(state){ return state.moduleTwovalue+'0' }, globalValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.global }, otherValuePlus(state,getters,rootState){ return state.moduleTwovalue+rootState.moduleOne.moduleOnevalue }, } } } })
Page reference.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> <p>moduleOne_mapGetters: {{OnevaluePlus}}</p> <p>moduleTwo_mapGetters: {{TwovaluePlus}}</p> <p>moduleOne_mapGetters_global: {{OneglobalValue}}</p> <p>moduleTwo_mapGetters_global: {{TwoglobalValue}}</p> <p>moduleOne_mapGetters_other: {{OneotherValue}}</p> <p>moduleTwo_mapGetters_other: {{TwootherValue}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue }), ...mapGetters({ OnevaluePlus:'moduleOne/valuePlus', TwovaluePlus:'moduleTwo/valuePlus', OneglobalValue:'moduleOne/globalValuePlus', TwoglobalValue:'moduleTwo/globalValuePlus', OneotherValue:'moduleOne/otherValuePlus', TwootherValue:'moduleTwo/otherValuePlus' }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']), ...mapActions(['moduleOne/timeOut','moduleOne/globaltimeOut','moduleOne/othertimeOut']) }, mounted() { // this['moduleOne/updateValue '] (' I am the changed value: 1 '); // this['moduleTwo/updateValue '] (' I am the changed value: 0 '); // this['moduleOne/timeOut'](); // this['moduleOne/globaltimeOut'](); this['moduleOne/othertimeOut'](); }, } </script>
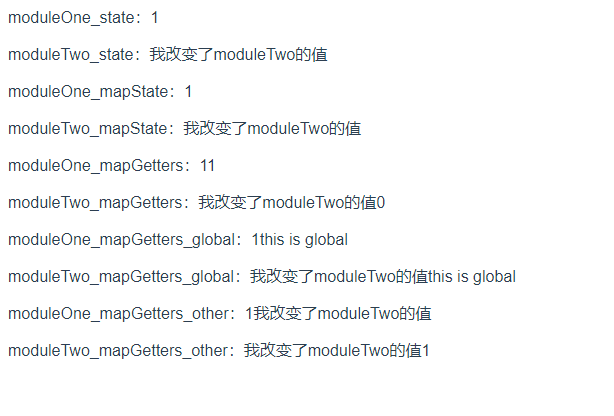
Note: you can continue to add modules in the module, which can loop indefinitely.
6, Dynamic registration module
Sometimes, we use the router to load routes asynchronously. In some places, we don't need the data of some modules, so we use the dynamic registration module of VueX. We come to the entry file main.js.
import Vue from 'vue' import App from './App.vue' import router from './router' import store from './store' Vue.config.productionTip = false // Dynamic registration module store.registerModule('moduleThree',{ state:{ text:"this is moduleThree" } }) new Vue({ router, store, render: h => h(App) }).$mount('#app')
Reference it on the page.
<template> <div class="home"> <p>moduleOne_state: {{moduleOne}}</p> <p>moduleTwo_state: {{moduleTwo}}</p> <p>moduleOne_mapState: {{moduleOnevalue}}</p> <p>moduleTwo_mapState: {{moduleTwovalue}}</p> <p>moduleOne_mapGetters: {{OnevaluePlus}}</p> <p>moduleTwo_mapGetters: {{TwovaluePlus}}</p> <p>moduleOne_mapGetters_global: {{OneglobalValue}}</p> <p>moduleTwo_mapGetters_global: {{TwoglobalValue}}</p> <p>moduleOne_mapGetters_other: {{OneotherValue}}</p> <p>moduleTwo_mapGetters_other: {{TwootherValue}}</p> <p>moduleThree_mapState: {{moduleThreetext}}</p> </div> </template> <script> // eslint-disable-next-line no-unused-vars import {mapState,mapMutations,mapGetters,mapActions} from 'vuex' export default { name:"Home", data() { return { msg:"this is Home" } }, computed: { moduleOne(){ return this.$store.state.moduleOne.moduleOnevalue }, moduleTwo(){ return this.$store.state.moduleTwo.moduleTwovalue }, ...mapState({ moduleOnevalue:(state)=>state.moduleOne.moduleOnevalue, moduleTwovalue:(state)=>state.moduleTwo.moduleTwovalue, moduleThreetext:(state)=>state.moduleThree.text }), ...mapGetters({ OnevaluePlus:'moduleOne/valuePlus', TwovaluePlus:'moduleTwo/valuePlus', OneglobalValue:'moduleOne/globalValuePlus', TwoglobalValue:'moduleTwo/globalValuePlus', OneotherValue:'moduleOne/otherValuePlus', TwootherValue:'moduleTwo/otherValuePlus' }) }, methods: { ...mapMutations(['moduleOne/updateValue','moduleTwo/updateValue']), ...mapActions(['moduleOne/timeOut','moduleOne/globaltimeOut','moduleOne/othertimeOut']) }, mounted() { // this['moduleOne/updateValue '] (' I am the changed value: 1 '); // this['moduleTwo/updateValue '] (' I am the changed value: 0 '); // this['moduleOne/timeOut'](); // this['moduleOne/globaltimeOut'](); // this['moduleOne/othertimeOut'](); }, } </script>
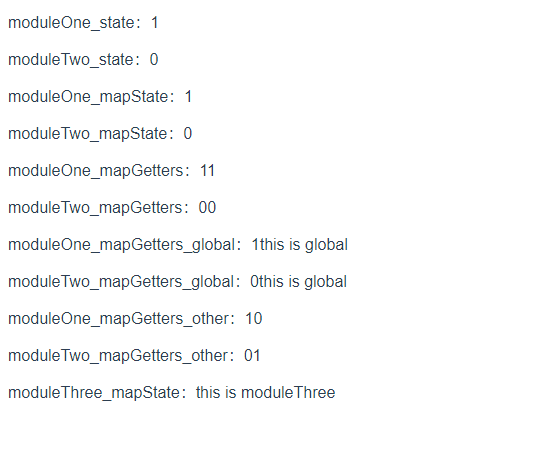