There are security issues with Android's global broadcast. For example, sending some critical data broadcasts may be captured by other applications, or receiving all kinds of garbage broadcasts sent by other programs.
Local broadcast is to solve this security problem. It can only be delivered within the application, and the broadcast receiver can only receive the broadcast from the application.
Local broadcast uses local broadcast manager to manage the broadcast, and provides methods to send the broadcast and register the broadcast receiver.
stay How to send a custom broadcast in Android Based on the project, modify MainActivity:
public class MainActivity extends AppCompatActivity { private LocalReceiver localReceiver = new LocalReceiver(); private LocalBroadcastManager localBroadcastManager = null; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); localBroadcastManager = LocalBroadcastManager.getInstance(this); findViewById(R.id.button).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // sendBroadcast(new Intent("net.deniro.android.MY_BROADCAST")); // sendOrderedBroadcast((new Intent("net.deniro.android.MY_BROADCAST")),null); //Send local broadcast localBroadcastManager.sendBroadcast(new Intent("net.deniro.android.MY_BROADCAST")); } }); //Register for local broadcast IntentFilter intentFilter = new IntentFilter(); intentFilter.addAction("net.deniro.android.MY_BROADCAST"); localBroadcastManager.registerReceiver(localReceiver, intentFilter); } @Override protected void onDestroy() { super.onDestroy(); localBroadcastManager.unregisterReceiver(localReceiver);//Log off local broadcast } /** * Local broadcasting */ private class LocalReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { Toast.makeText(context, "Receive local broadcast O(∩_∩)O Ha-ha~", Toast.LENGTH_SHORT).show(); } } }
An instance of the LocalBroadcastManager class is used to send, register, and unregister local broadcasts.
Run program:
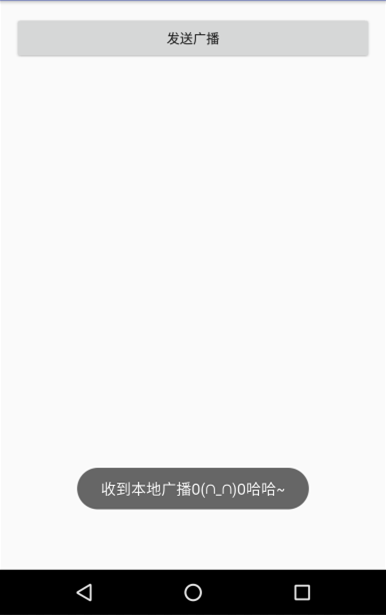
Note: local broadcast cannot be received by static registration. Because static registration is to enable the program to receive broadcast even when it is not started, and if the program is not started, it will not be able to use local broadcast O(∩∩∩∩∩∩∩∩∩∩∩∩∩∩∩
Local broadcasting has these advantages:
- It is clear that the broadcast being sent will not leave the current program, so there is no need to worry about data leakage.
- Other programs can't send broadcast to the inside of our program, so we can't worry about security vulnerabilities.
- Sending local broadcast is more efficient than sending system global broadcast.