preface
In the "vue" framework, if you need to traverse the rendering template data, you need to use the v-for command. There is also the "key" problem. Let's see how to use it and solve the problem!!
v-for and key attributes of Vue instruction
- Iterative array
<ul> <li v-for="(item, i) in list">Indexes:{{i}} --- full name:{{item.name}} --- Age:{{item.age}}</li> </ul>
- Iterating over attributes in objects
<!-- Loop through the properties on the object --> <div v-for="(val, key, i) in userInfo">{{val}} --- {{key}} --- {{i}}</div>
- Iterative number
<p v-for="i in 10">This is the second {{i}} individual P label</p>
❝ in version 2.2.0 +, key is now required when "v-for" is used in components. ❞
When Vue When JS is updating the rendered element list with v-for, it uses the "reuse in place" policy by default. If the order of data items is changed, Vue will "not move DOM elements to match the order of data items", but "simply reuse each element here" and ensure that it displays each element that has been rendered under a specific index.
To give Vue a hint, "so that it can track the identity of each node and reuse and reorder existing elements", you need to provide a unique key attribute for each item.
Example 1: iterative array
<!DOCTYPE html> <html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <ul> <li v-for="(item, i) in list">Indexes:{{i}} --- full name:{{item.name}} --- Age:{{item.age}}</li> </ul> </div> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: { list: [ { "name":"Zhang Yi", "age": 31}, { "name":"Zhang Er", "age": 21}, { "name":"Zhang San", "age": 41}, { "name":"Zhang Si", "age": 51}, { "name":"Zhang Wu", "age": 61}, ], }, methods:{} }) </script> </body> </html>
The browser displays as follows:

Example 2: iterating over attributes in an object
<!DOCTYPE html> <html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <!-- Loop through the properties on the object --> <div v-for="(val, key, i) in userInfo">value Value:{{val}} --- key Value:{{key}} --- Indexes:{{i}}</div> </div> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: { userInfo: { userid: 1, username: "Zhang San", age: 30 } }, methods:{} }) </script> </body> </html>
The browser displays as follows:
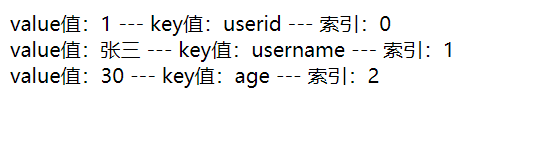
Example 3: iteration number
<!DOCTYPE html> <html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <p v-for="i in 15">This is the second {{i}} individual P label</p> </div> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: {}, methods:{} }) </script> </body> </html>
The browser displays as follows:
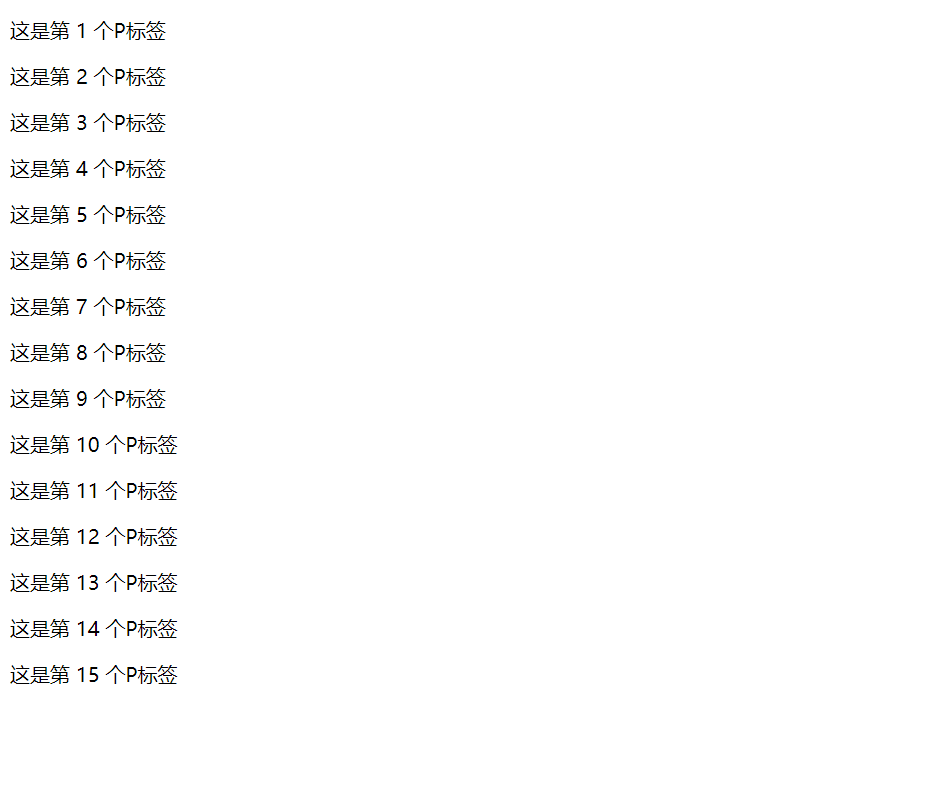
Precautions for using key in v-for
In version 2.2.0 +, when v-for is used in components, the key is now necessary, because without a key to ensure uniqueness in the loop, the components will be disrupted.
Let's take a look at an example to clarify what problems will occur when key s are not used.
Examples of problems without key s
<!DOCTYPE html> <html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <div> <label>Id: <input type="text" v-model="id"> </label> <label>Name: <input type="text" v-model="name"> </label> <input type="button" value="add to" @click="add"> </div> <p v-for="item in list" > <input type="checkbox">{{item.id}} --- {{item.name}} </p> </div> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: { id: '', name: '', list: [ { id: 1, name: 'LISS' }, { id: 2, name: 'Ying Zheng' }, { id: 3, name: 'eunuch who conspired with Li Si to influence the succession to the First Emperor' }, { id: 4, name: 'Han Fei' }, { id: 5, name: 'a pre-Qin philosopher' } ] }, methods:{ add(){ // Add new data to the end of the queue this.list.push({ id: this.id, name: this.name }) } } }) </script> </body> </html>
The browser displays as follows:
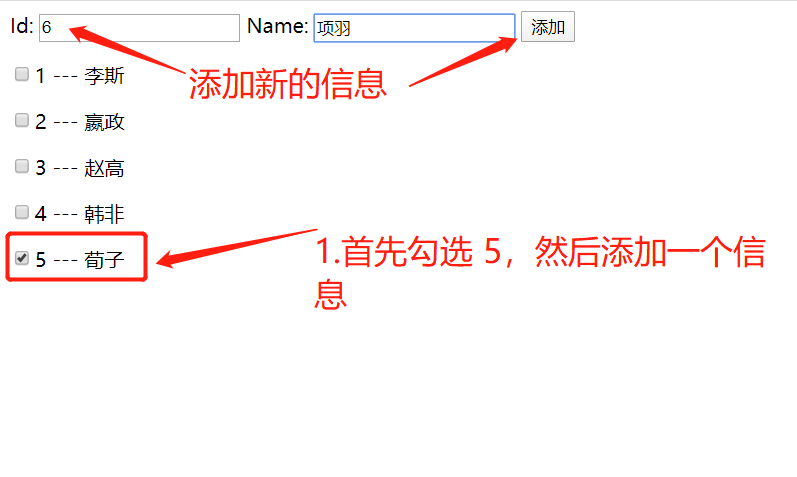
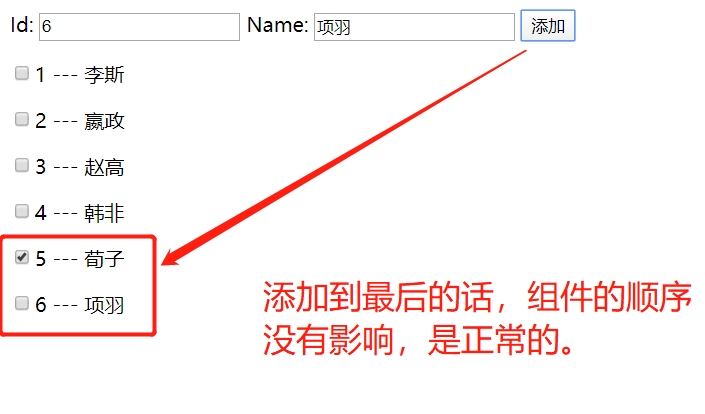
As can be seen from the above example, when adding data to the end, the originally checked "5 - Xunzi" does not affect the order.
Let's see what happens if you insert data into the front?
Use the unshift() method to add data to the front of the queue, as follows:
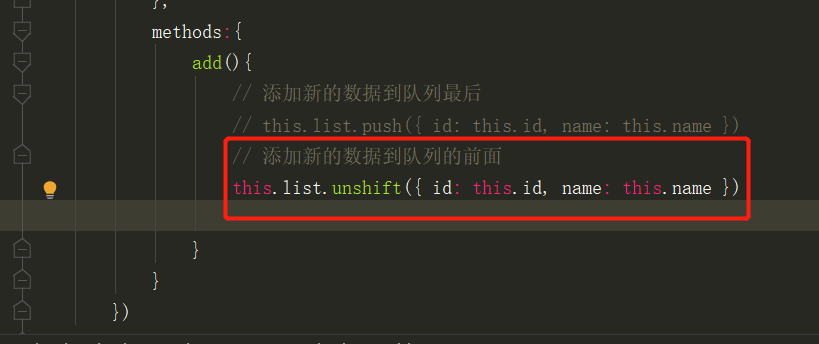
Then, execute the above example again, as follows:
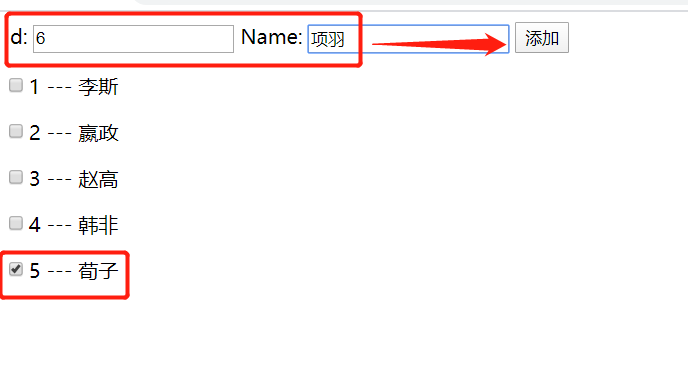
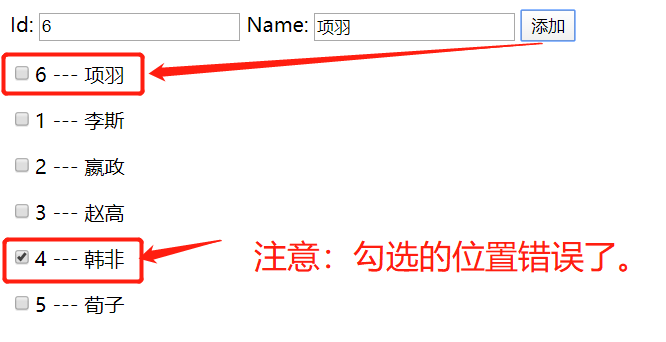
<p v-for="item in list" > <input type="checkbox">{{item.id}} --- {{item.name}} </p>
At this time, set a "key" for the above components and bind a "string/number" type of data to ensure the uniqueness of circular data.
This ensures rendering.
Use v-bind to set the value of key to ensure the data order of rendering
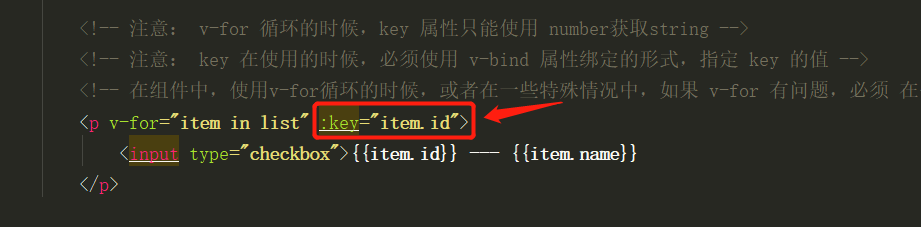
<!-- be careful: v-for When cycling, key Property can only be used number obtain string --> <!-- be careful: key When in use, it must be used v-bind Property binding, specifying key Value of --> <!-- In a component, use v-for When cycling, or in some special cases, if v-for If there is a problem, it must be used v-for At the same time, specify a unique string/Number type :key value --> <p v-for="item in list" :key="item.id"> <input type="checkbox">{{item.id}} --- {{item.name}} </p>
Perform the error procedure again, as follows:
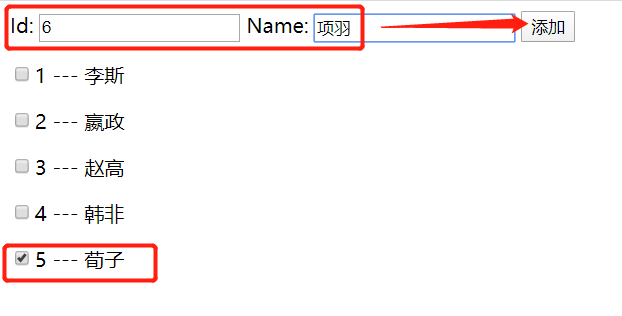
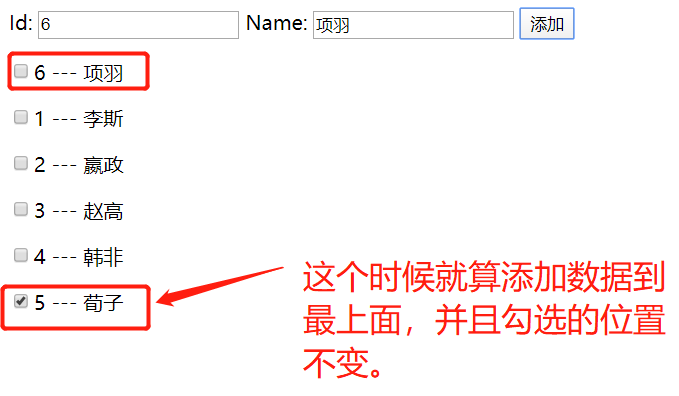