1, Simple use of Hystrix
Create an empty Maven project and add the dependency of Hystrix in the project, as shown in the following code:
<dependency> <groupId>com.netflix.hystrix</groupId> <artifactId>hystrix-core</artifactId> <version>1.5.13</version> </dependency>
Write the first HystrixCommand, as shown in the following code:
public class MyHystrixCommand extends HystrixCommand<String> { private final String name; public MyHystrixCommand(String name) { super (Hystr ixCommandGroupKey .Factory .asKey( "MyGroup")); this.name = name; } @Override protected String run() { return this.name + ":" + Thread. currentThread() .getName(); } }
First, you need to inherit the HystrixCommand and set a Groupkey through the constructor. For the specific logic, in the run method, we return a value of the current thread name. Write a main method to call the MyHystrixCommand program written above, as shown in the following code:
public static void main(String[] args)throws InterruptedException, ExecutionException { String result = new MyHystrixCommand("yinjihuan").execute(); System.out.println(result); }
The output is: yinjihuan: hystrix mygroup. As you can see, the group name set in the constructor becomes the name of the thread.
The above is synchronous call. If asynchronous call is required, you can use the method shown in the following code:
public static void main(String[] args)throws InterruptedException, ExecutionException { Future<String>future = new MyHystrixCommand("yinjihuan").queue(); System.out.println( future.get()); }
2, Fallback support
Next, we simulate the call failure by increasing the execution time. First, we modify MyHystrixCommand and add the getFallback method to return the fallback content, as shown in the following code:
public class MyHystrixCommand extends HystrixCommand<String> { private final String name ; pub1ic MyHystrixCommand( String name) { super (HystrixCommandGroupKey.Factory.asKey("MyGroup")); this.name = name; } @Override protected String run() { try { Thread. sleep(1000 * 10); } catch (InterruptedExceptione) { e.printStackTrace(); } return this.name + ":" + Thread.currentThread() . getName(); @Override protected String getFallback() { return"failed"; } }
After re executing the calling code, you can find that the returned content is "failed", which proves that fallback has been triggered.
3, Semaphore policy configuration
The semaphore policy configuration method is shown in the following code:
public MyHystrixCommand(String name) { super ( HystrixCommand . Setter .Wi thGroupKey(Hystr ixCommandGroupKey .Factory .asKey( "MyGroup")) . andCommandPropertiesDefaults ( Hystr ixCommandProperties . Setter( ) . withExecut ionIsolationStrategy( Hystr ixCommandProperties . ExecutionIsolat ionStrategy . SEMAPHORE ) ) ); this.name = name; }
Previously, the thread name was deliberately output in the run method. Through this name, you can determine whether the current thread isolation or semaphore isolation is.
4, Thread isolation policy configuration
The system adopts thread isolation policy by default. We can configure some parameters of thread pool through andThreadPoolPropertiesDefaults, as shown in the following code:
public MyHystrixCommand(String name) { super(HystrixCommand.Setter.withGroupKey ( HystrixCommandGroupKey.Factory .asKey( "MyGroup")) .andCommandPropertiesDefaults ( HystrixCommandProperties.Setter() .withExecutionIsolationStrategy ( HystrixCommandProperties.ExecutionIsolationStrategy.THREAD ) ) . andThreadPoolPropertiesDefaults ( HystrixThreadPoolProperties. Setter() . withCoreSize(10 ) . withMaxQueueSize(100) .withMax. imumSize(100) ) ); this.name = name ; }
5, Result cache
Cache is often used in development. We often use Redis, a third-party cache database, to cache data. Method level caching is also provided in Hystrix. Determine whether to return cached data by rewriting getCacheKey. getCacheKey can be generated according to parameters, so that the same parameters can be used for caching.
The MyHystrixCommand before the transformation adds the rewriting implementation of getCacheKey, as shown in the following code:
@verride protected String getCacheKey() { return String.valueOf(this.name); }
In the above code, the name parameter passed in when we create the object is used as the cached key.
In order to prove that the cache can be used, add a line of output in the run method. If the console outputs only once after multiple calls, you can know that the following is the cache logic, as shown in the following code:
@override protected String run() { System.err.print1n("get data"); return this.name + ":" + Thread. currentThread().getName(); }
Execute the main method and find an error:
According to the error prompt, the cache processing depends on the request context. We must initialize the hystrix requestcontext.
Modify the calling code in the main method and initialize the HystrixRequestContext, as shown in the following code:
public static void main(String[] args)throws InterruptedException, ExecutionException { HystrixRequestContext context = HystrixRequestContext. initializeContext(); String result = new MyHystrixCommand("yinjihuan").execute(); System.out.println(result); Future<string>future = new MyHystrixCommand("yinjihuan") .queue(); System.out.println(future.get()); context . shutdown() ; }
After the transformation, rewrite and execute the main method, and it can run normally. The output results are as follows
You can see that get data is output only once and the cache takes effect.
6, Cache clear
In the previous section, we learned how to use Hystrix to implement data caching. If there is a cache, there must be an action to clear the cache. When the data changes, the data in the cache must also be updated, otherwise the problem of dirty data will occur. Similarly, Hystrix also has the function of clearing the cache.
Add a class that supports cache cleanup, as shown in the following code:
public class ClearCacheHystrixCommand extends HystrixCommand<String> { private final String name ; private static final HystrixCommandKey GETTER_ KEY = HystrixCommandKey.Factory . asKey("MyKey"); public ClearCacheHystrixCommand (String name) { super ( HystrixCommand . Setter .withGroupKey ( HystrixCommandGroupKey . Factory .asKey( "MyGroup" )) . andCommandKey (GETTER_ KEY ) # last Looking at the interview questions of perfect group, byte and Tencent, do you feel that you ask a lot of questions? Maybe we have to start the mode of making rockets in the interview and screwing in the work to prepare for the next interview. It was mentioned at the beginning that I memorized 1000 questions, which is still a little useful. I looked at it. Most of the above questions can be found from the questions I recited, so I'll share them with you today**1000 necessary interview questions for Internet engineers**. > Note: no matter what I said about the 1000 Internet interview questions, or the algorithms and data structures, design patterns and more mentioned later Java Learning notes, etc. can be shared with all friends,**[You can download it for free](https://gitee.com/vip204888/java-p7)** 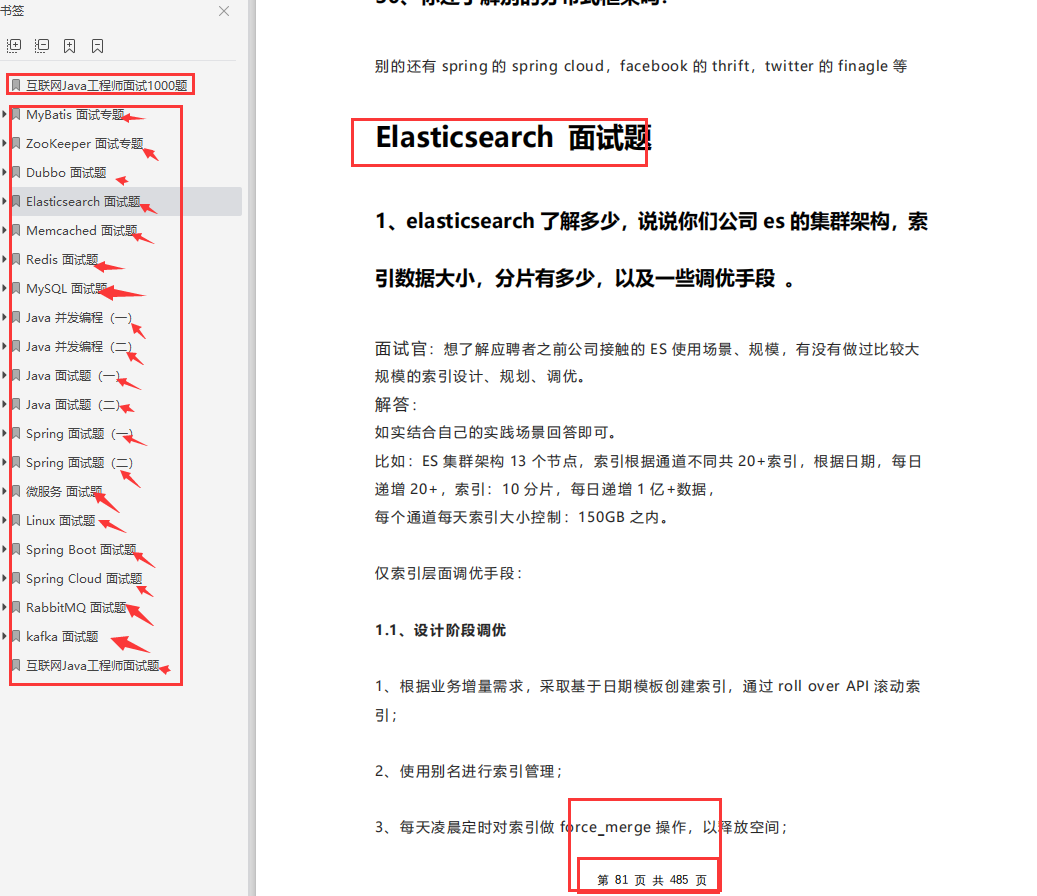 1000 necessary interview questions for Internet engineers And from the above three,**Algorithms and data structures are essential**Ah, so I suggest you go**Brush the programmer code interview guide written by Zuo Chengyun IT Optimal solution of famous enterprise algorithm and data structure problem**,Inside near**200 A real classic code interview question**. va-p7)** [External chain picture transfer...(img-q761x6kp-1628667695956)] 1000 necessary interview questions for Internet engineers And from the above three,**Algorithms and data structures are essential**Ah, so I suggest you go**Brush the programmer code interview guide written by Zuo Chengyun IT Optimal solution of famous enterprise algorithm and data structure problem**,Inside near**200 A real classic code interview question**. 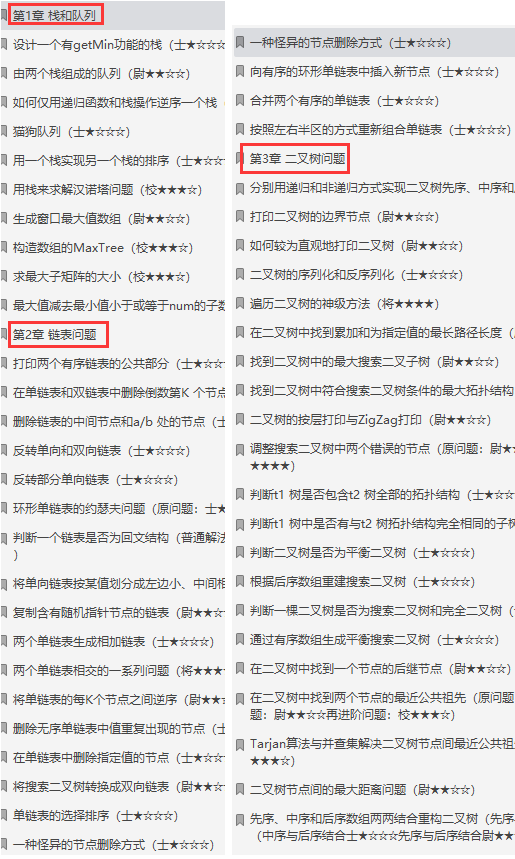