I/O model
Java BIO programming
1. Basic description of the model
- The simple understanding of I/O model is that what channel is used to send and receive data, which largely determines the performance of program communication.
- Java supports three network programming model I/O modes: BIO, NIO and AIO
- Java BIO: synchronous and blocking (traditional blocking). The server implementation mode is one connection and one thread, that is, when the client has a connection request, the server needs to start a thread for processing. If the connection does not do anything, it will cause unnecessary thread overhead. [simple schematic diagram]
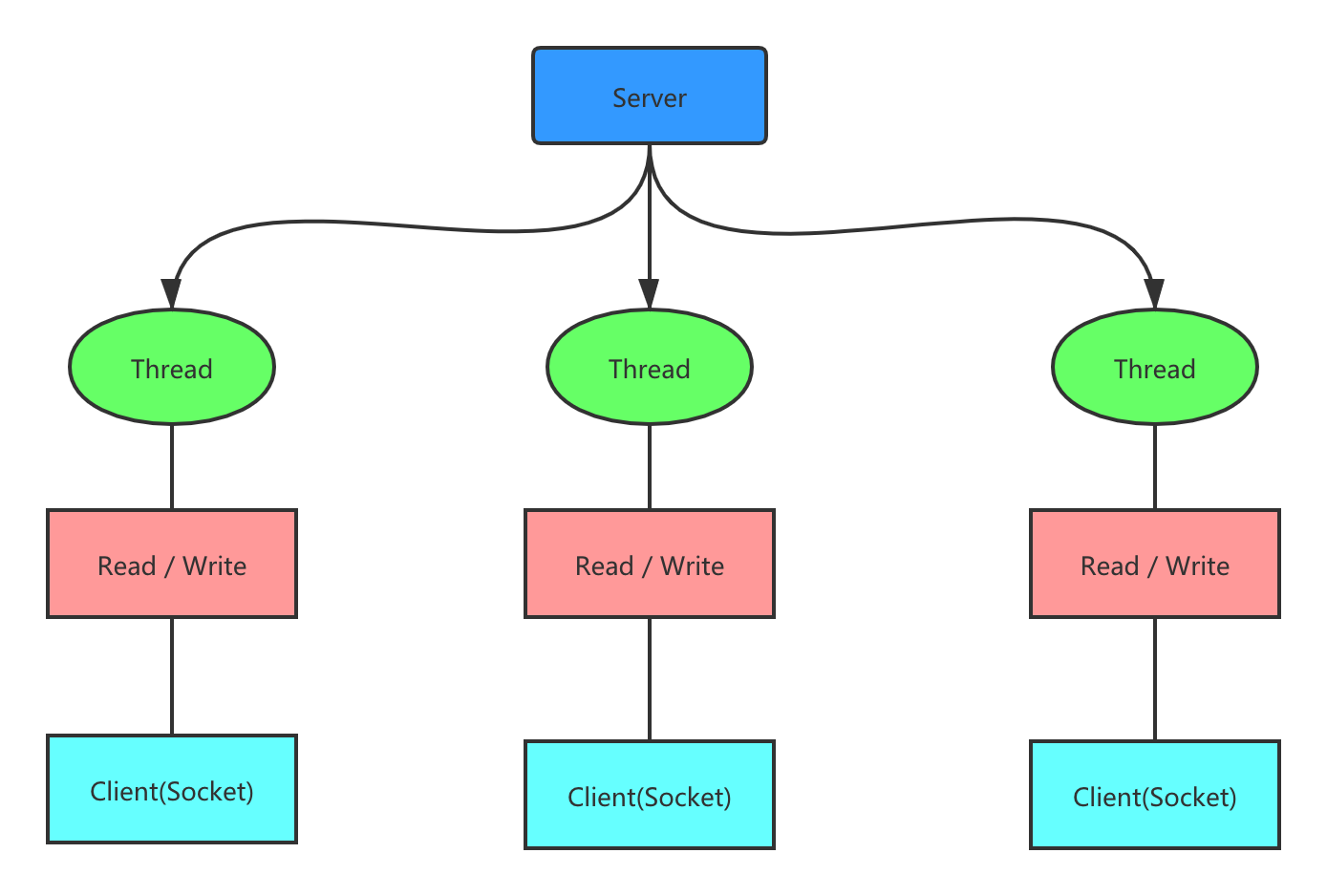
- Java NIO: synchronous non blocking. The server implementation mode is that one thread processes multiple requests (connections), that is, the connection requests sent by the client will be registered on the multiplexer, and the multiplexer will process the I/O requests when it polls the connection. [simple schematic diagram]
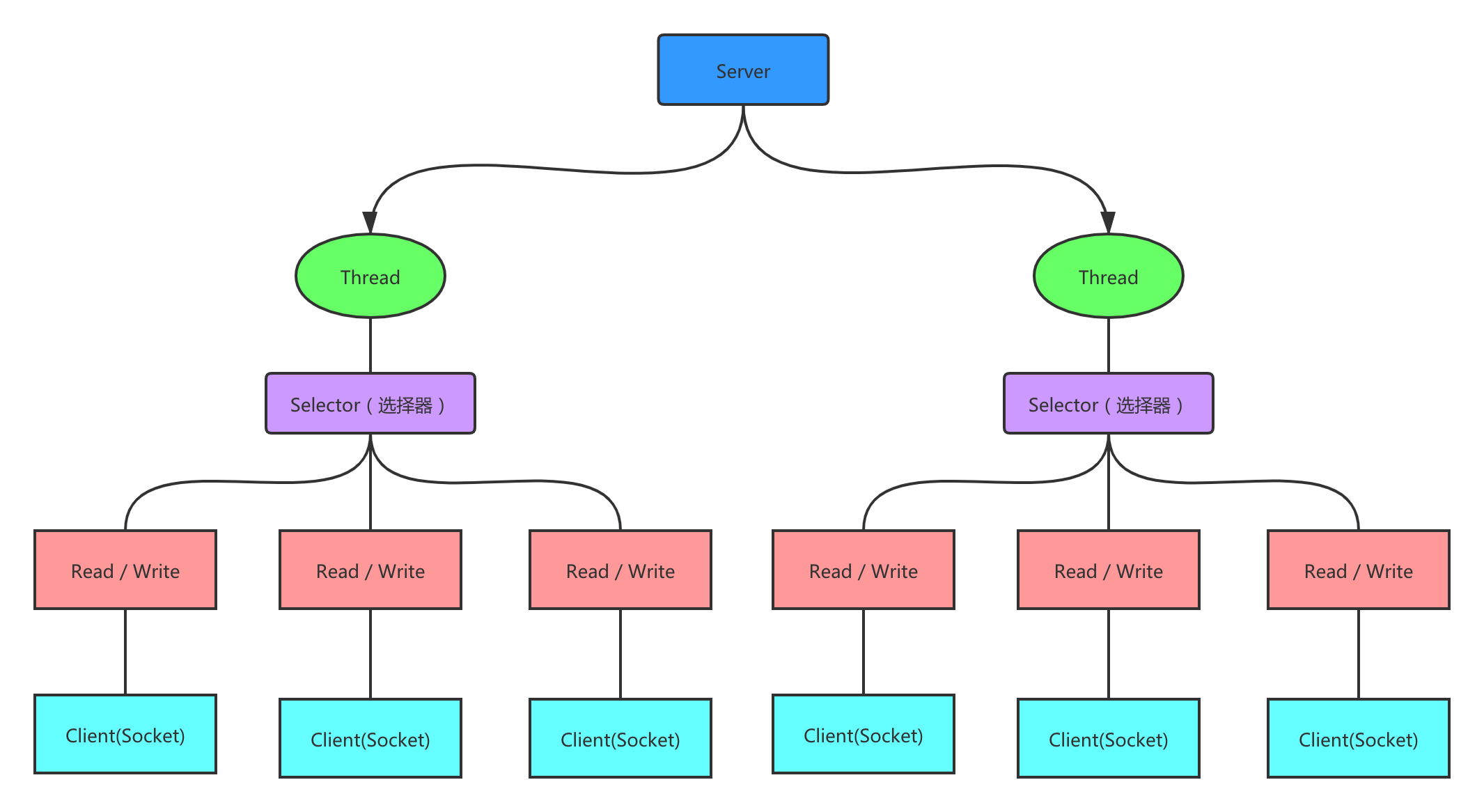
- Java AIO(NIO.2): asynchronous and non blocking. AIO introduces the concept of asynchronous channel and adopts the Proactor mode, which simplifies the programming and starts the thread only after an effective request. Its feature is that the operating system notifies the server program to start the thread for processing after it is completed. It is generally suitable for applications with a large number of connections and a long connection time.
2. Analysis of bio, NIO and AIO usage scenarios
- BIO mode is applicable to the architecture with a small and fixed number of connections. This mode has high requirements on server resources, and concurrency is limited to applications. It is the only choice before JDK1.4, but the program is simple and easy to understand.
- NIO mode is applicable to the architecture with a large number of connections and short connections (light operation), such as chat server, bullet screen system, inter server communication, etc. The programming is complex, and JDK1.4 starts to support it.
- AIO mode is used in architectures with a large number of connections and long connections (re operation), such as photo album server. It fully calls the OS to participate in concurrent operations. The programming is complex, and JDK7 starts to support it.
3. Basic introduction to Java Bio
- Java BIO is the traditional Java I/O programming, and its related classes and interfaces are in java.io.
- Bio (blocking I / O): synchronous blocking. The server implementation mode is one connection and one thread. That is, when the client has a connection request, the server needs to start a thread for processing. If the connection does not do anything, it will cause unnecessary thread overhead. It can be improved through the thread pool mechanism (multiple clients connect to the server). [application examples follow]
- BIO mode is applicable to the architecture with a small and fixed number of connections. This mode has high requirements on server resources, and concurrency is limited to applications. It is the only choice before JDK1.4, and the program is simple and easy to understand.
4. Java bio working mechanism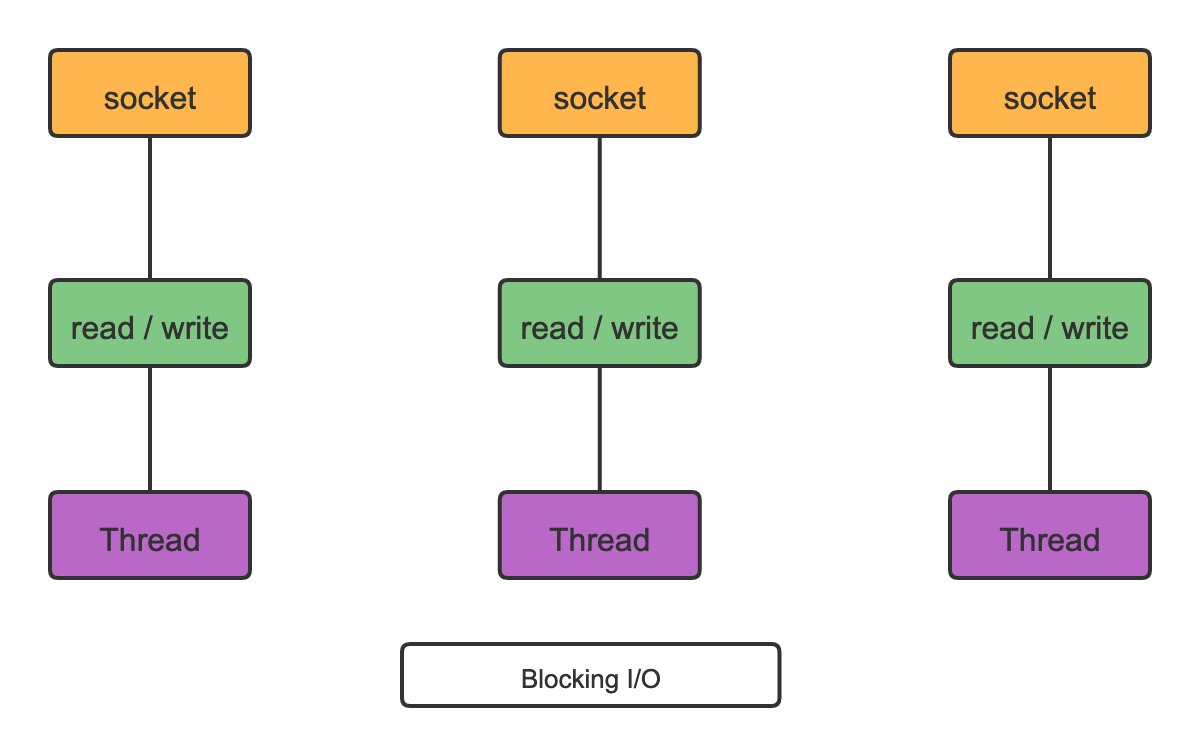
5. Sorting out BIO programming process
- Start a ServerSocket on the server side.
- The client starts the Socket to communicate with the server. By default, the server needs to establish a thread for each client to communicate with it.
- After the client sends a request, it first asks the server whether there is a thread response. If not, it will wait or be rejected.
- If there is a response, the client thread will wait for the request to end before continuing execution.
6 . BIO Demo
- Write a server side using BIO model, listen to port 6666, and start a thread to communicate with it when there is a client connection.
- It is required to use the thread pool mechanism to improve, and multiple clients can be connected.
- The server can receive the data sent by the client (telnet).
package com.atguigu.bio;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class BIOServer {
public static void main(String[] args) throws Exception {
//Thread pool mechanism
//thinking
//1. Create a thread pool
//2. If there is a client connection, create a thread to communicate with it (write a separate method)
ExecutorService newCachedThreadPool = Executors.newCachedThreadPool();
//Create ServerSocket
ServerSocket serverSocket = new ServerSocket(6666);
System.out.println("The server started");
while (true) {
System.out.println("Thread information id = " + Thread.currentThread().getId() + "name = " + Thread.currentThread().getName());
//Listen and wait for the client to connect
System.out.println("Waiting for connection....");
final Socket socket = serverSocket.accept();
System.out.println("Connect to a client");
//Create a thread and communicate with it (write a separate method)
newCachedThreadPool.execute(new Runnable() {
public void run() {//We rewrite
//Can communicate with clients
handler(socket);
}
});
}
}
//Write a handler method to communicate with the client
public static void handler(Socket socket) {
try {
System.out.println("Thread information id = " + Thread.currentThread().getId() + "name = " + Thread.currentThread().getName());
byte[] bytes = new byte[1024];
//Get input stream through socket
InputStream inputStream = socket.getInputStream();
//Cyclic reading of data sent by the client
while (true) {
System.out.println("Thread information id = " + Thread.currentThread().getId() + "name = " + Thread.currentThread().getName());
System.out.println("read....");
int read = inputStream.read(bytes);
if (read != -1) {
System.out.println(new String(bytes, 0, read));//Output data sent by client
} else {
break;
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
System.out.println("Close and client Connection of");
try {
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
7. Java bio problem analysis
- Each request needs to create an independent thread to Read, process and Write data with the corresponding client.
- When the number of concurrent connections is large, a large number of threads need to be created to process connections, which takes up a large amount of system resources.
- After the connection is established, if the current thread has no data readable temporarily, the thread will block the Read operation, resulting in a waste of thread resources.