Network sockets using QT s require the addition of a sentence in the. pro file:
I. Client
1. The client code is slightly simpler than the server. Generally speaking, using the QTcpSocket class in the QT to communicate with the server requires only the following five steps:
(1) Create a QTcpSocket socket object
-
socket = new QTcpSocket();
(2) Connect the server with this object
-
socket->connectToHost(IP, port);
(3) Send data to server using write function
(4) When new data arrives in socket receiving buffer, readRead() signal will be issued, so slot function is added to read data for the signal.
-
QObject::connect(socket, &QTcpSocket::readyRead, this, &MainWindow::socket_Read_Data);
-
-
void MainWindow::socket_Read_Data()
-
{
-
QByteArray buffer;
-
-
buffer = socket->readAll();
-
}
(5) Disconnect the connection with the server (for the difference between close() and disconnectFromHost(), you can see the help in F1)
-
socket->disconnectFromHost();
2. The following are client routines
First, the mainwindow.h file:
-
-
#ifndef MAINWINDOW_H
-
#define MAINWINDOW_H
-
-
#include <QMainWindow>
-
#include <QTcpSocket>
-
-
namespace Ui {
-
class MainWindow;
-
}
-
-
class MainWindow : public QMainWindow
-
{
-
Q_OBJECT
-
-
public:
-
explicit MainWindow(QWidget *parent = 0);
-
~MainWindow();
-
-
private slots:
-
-
void on_pushButton_Connect_clicked();
-
-
void on_pushButton_Send_clicked();
-
-
void socket_Read_Data();
-
-
void socket_Disconnected();
-
-
private:
-
Ui::MainWindow *ui;
-
QTcpSocket *socket;
-
};
-
-
#endif // MAINWINDOW_H
Then the mainwindow.cpp file:
-
-
#include "mainwindow.h"
-
#include "ui_mainwindow.h"
-
-
MainWindow::MainWindow(QWidget *parent) :
-
QMainWindow(parent),
-
ui(new Ui::MainWindow)
-
{
-
ui->setupUi(this);
-
-
socket = new QTcpSocket();
-
-
-
QObject::connect(socket, &QTcpSocket::readyRead, this, &MainWindow::socket_Read_Data);
-
QObject::connect(socket, &QTcpSocket::disconnected, this, &MainWindow::socket_Disconnected);
-
-
ui->pushButton_Send->setEnabled(false);
-
ui->lineEdit_IP->setText("127.0.0.1");
-
ui->lineEdit_Port->setText("8765");
-
-
}
-
-
MainWindow::~MainWindow()
-
{
-
delete this->socket;
-
delete ui;
-
}
-
-
void MainWindow::on_pushButton_Connect_clicked()
-
{
-
if(ui->pushButton_Connect->text() == tr("Connect"))
-
{
-
QString IP;
-
int port;
-
-
-
IP = ui->lineEdit_IP->text();
-
-
port = ui->lineEdit_Port->text().toInt();
-
-
-
socket->abort();
-
-
socket->connectToHost(IP, port);
-
-
-
if(!socket->waitForConnected(30000))
-
{
-
qDebug() << "Connection failed!";
-
return;
-
}
-
qDebug() << "Connect successfully!";
-
-
-
ui->pushButton_Send->setEnabled(true);
-
-
ui->pushButton_Connect->setText("Disconnect");
-
}
-
else
-
{
-
-
socket->disconnectFromHost();
-
-
ui->pushButton_Connect->setText("Connect");
-
ui->pushButton_Send->setEnabled(false);
-
}
-
}
-
-
void MainWindow::on_pushButton_Send_clicked()
-
{
-
qDebug() << "Send: " << ui->textEdit_Send->toPlainText();
-
-
socket->write(ui->textEdit_Send->toPlainText().toLatin1());
-
socket->flush();
-
}
-
-
void MainWindow::socket_Read_Data()
-
{
-
QByteArray buffer;
-
-
buffer = socket->readAll();
-
if(!buffer.isEmpty())
-
{
-
QString str = ui->textEdit_Recv->toPlainText();
-
str+=tr(buffer);
-
-
ui->textEdit_Recv->setText(str);
-
}
-
}
-
-
void MainWindow::socket_Disconnected()
-
{
-
-
ui->pushButton_Send->setEnabled(false);
-
-
ui->pushButton_Connect->setText("Connect");
-
qDebug() << "Disconnected!";
-
}
Finally, the design of ui:
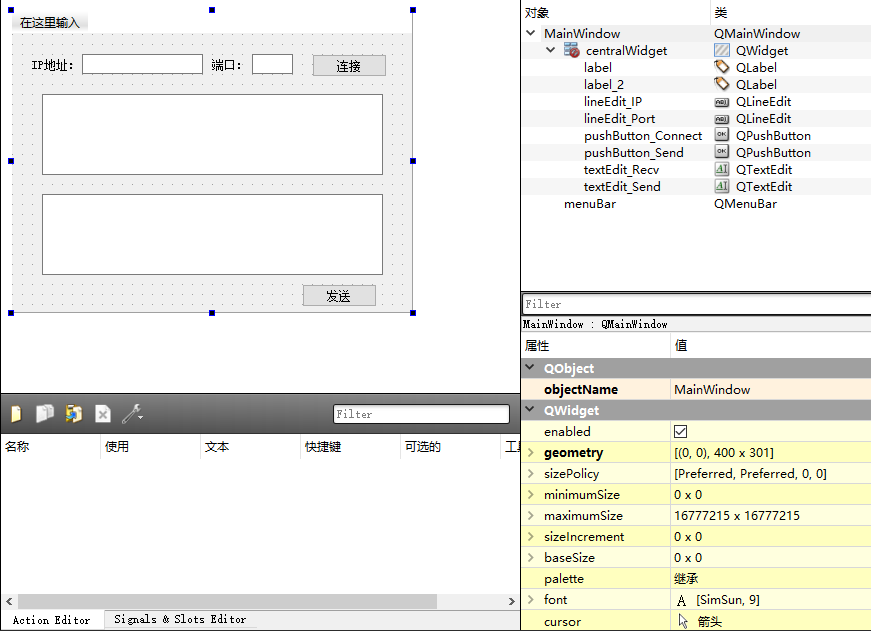
Server
1. In addition to using the QTcpSocket class, the server also needs to use the QTcpSever class. Even so, it is only a little more complicated than the client, using six steps:
(1) Create QTcpSever objects
-
server = new QTcpServer();
(2) Listen on a port so that the client can access the server using this port
-
server->listen(QHostAddress::Any, port)
(3) When the server is accessed by the client, a new Connection () signal is issued, so slot function is added to the signal and a QTcpSocket object is used to receive client access.
-
connect(server,&QTcpServer::newConnection,this,&MainWindow::server_New_Connect);
-
-
void MainWindow::server_New_Connect()
-
{
-
-
socket = server->nextPendingConnection();
-
}
(4) Send data to client using write function of socket
(5) When new data arrives in socket receiving buffer, readRead() signal will be issued, so slot function is added to read data for the signal.
-
QObject::connect(socket, &QTcpSocket::readyRead, this, &MainWindow::socket_Read_Data);
-
-
void MainWindow::socket_Read_Data()
-
{
-
QByteArray buffer;
-
-
buffer = socket->readAll();
-
}
(6) Cancellation of interception
2. The following is the routine of the server
First, the mainwindow.h file:
-
-
#ifndef MAINWINDOW_H
-
#define MAINWINDOW_H
-
-
#include <QMainWindow>
-
#include <QTcpServer>
-
#include <QTcpSocket>
-
-
namespace Ui {
-
class MainWindow;
-
}
-
-
class MainWindow : public QMainWindow
-
{
-
Q_OBJECT
-
-
public:
-
explicit MainWindow(QWidget *parent = 0);
-
~MainWindow();
-
-
private slots:
-
void on_pushButton_Listen_clicked();
-
-
void on_pushButton_Send_clicked();
-
-
void server_New_Connect();
-
-
void socket_Read_Data();
-
-
void socket_Disconnected();
-
-
private:
-
Ui::MainWindow *ui;
-
QTcpServer* server;
-
QTcpSocket* socket;
-
};
-
-
#endif // MAINWINDOW_H
Then the mainwindow.cpp file:
-
#include "mainwindow.h"
-
#include "ui_mainwindow.h"
-
-
MainWindow::MainWindow(QWidget *parent) :
-
QMainWindow(parent),
-
ui(new Ui::MainWindow)
-
{
-
ui->setupUi(this);
-
-
ui->lineEdit_Port->setText("8765");
-
ui->pushButton_Send->setEnabled(false);
-
-
server = new QTcpServer();
-
-
-
connect(server,&QTcpServer::newConnection,this,&MainWindow::server_New_Connect);
-
}
-
-
MainWindow::~MainWindow()
-
{
-
server->close();
-
server->deleteLater();
-
delete ui;
-
}
-
-
void MainWindow::on_pushButton_Listen_clicked()
-
{
-
if(ui->pushButton_Listen->text() == tr("Interception"))
-
{
-
-
int port = ui->lineEdit_Port->text().toInt();
-
-
-
if(!server->listen(QHostAddress::Any, port))
-
{
-
-
qDebug()<<server->errorString();
-
return;
-
}
-
-
ui->pushButton_Listen->setText("Cancel interception");
-
qDebug()<< "Listen succeessfully!";
-
}
-
else
-
{
-
-
if(socket->state() == QAbstractSocket::ConnectedState)
-
{
-
-
socket->disconnectFromHost();
-
}
-
-
server->close();
-
-
ui->pushButton_Listen->setText("Interception");
-
-
ui->pushButton_Send->setEnabled(false);
-
}
-
-
}
-
-
void MainWindow::on_pushButton_Send_clicked()
-
{
-
qDebug() << "Send: " << ui->textEdit_Send->toPlainText();
-
-
socket->write(ui->textEdit_Send->toPlainText().toLatin1());
-
socket->flush();
-
}
-
-
void MainWindow::server_New_Connect()
-
{
-
-
socket = server->nextPendingConnection();
-
-
QObject::connect(socket, &QTcpSocket::readyRead, this, &MainWindow::socket_Read_Data);
-
QObject::connect(socket, &QTcpSocket::disconnected, this, &MainWindow::socket_Disconnected);
-
-
ui->pushButton_Send->setEnabled(true);
-
-
qDebug() << "A Client connect!";
-
}
-
-
void MainWindow::socket_Read_Data()
-
{
-
QByteArray buffer;
-
-
buffer = socket->readAll();
-
if(!buffer.isEmpty())
-
{
-
QString str = ui->textEdit_Recv->toPlainText();
-
str+=tr(buffer);
-
-
ui->textEdit_Recv->setText(str);
-
}
-
}
-
-
void MainWindow::socket_Disconnected()
-
{
-
-
ui->pushButton_Send->setEnabled(false);
-
qDebug() << "Disconnected!";
-
}
Finally, the design of ui:
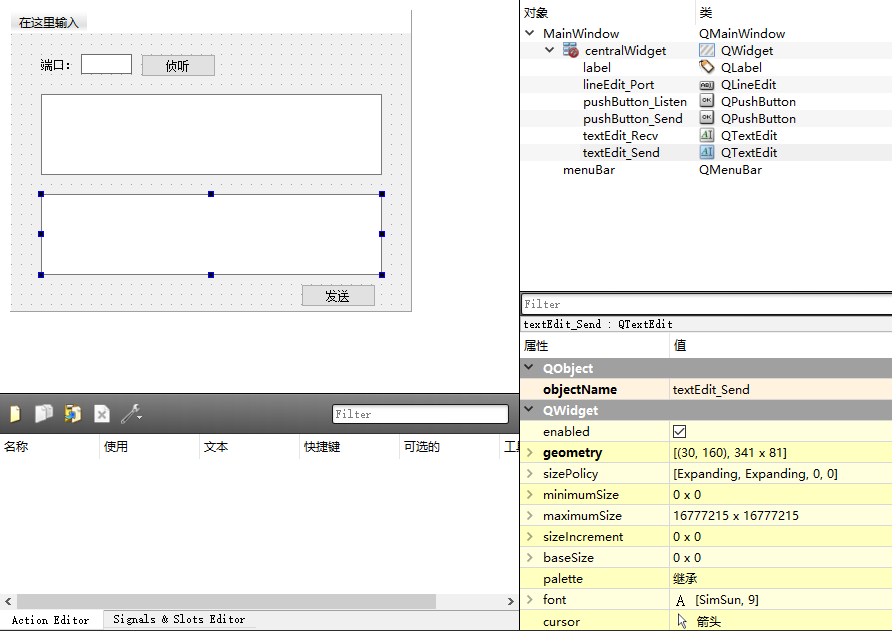
3. Operation results
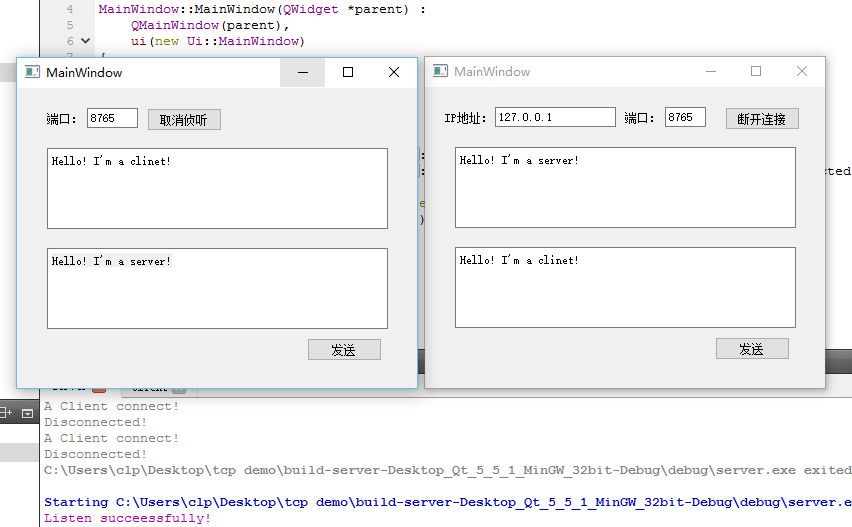