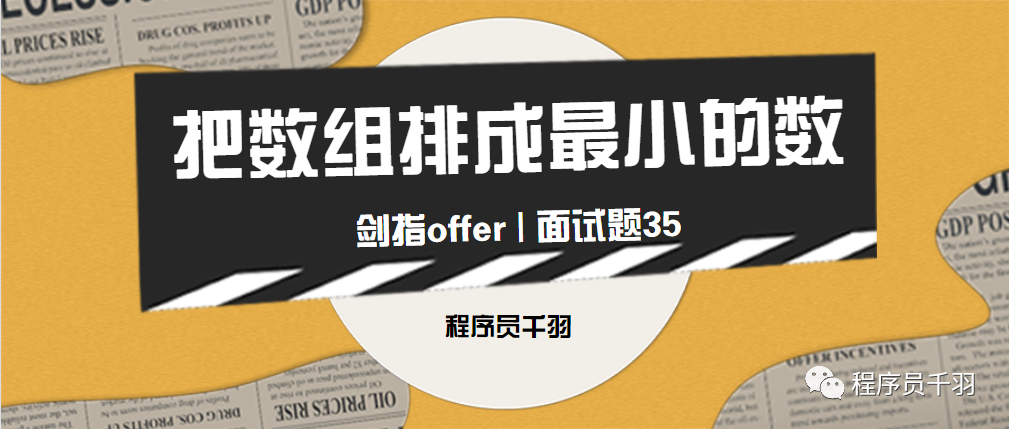
Knock algorithm series articles
- Ten classic sorting algorithms for dry goods and hand tearing
- Sword finger offer | understanding interview
- Sword finger offer | interview question 2: realizing Singleton mode
- Sword finger offer | interview question 3: finding two-dimensional array
- Sword finger offer | interview question 4: replace spaces
- Sword finger offer | interview question 5: print linked list from end to end
- Jianzhi offer | interview question 6: Rebuilding binary tree
- Sword finger offer | interview question 7: realizing queue with two stacks
- Sword finger offer | interview question 8: minimum number of rotation array
- Sword finger offer | interview question 9: Fibonacci sequence
- Sword finger offer | interview question 10: frog jumping steps
- Sword finger offer | interview question 11: matrix coverage
- Sword finger offer | interview question 12: the number of 1 in binary
- Sword finger offer | interview question 13: integer power of value
- Sword finger offer | interview question 14: print from 1 to the maximum n digits
- Sword finger offer | interview question 15: delete the node of the linked list
- Sword finger offer | interview question 16: put the odd number in the array before the even number
- Sword finger offer | interview question 17: the penultimate node in the lin k ed list
- Sword finger offer | interview question 18: reverse linked list
- Sword finger offer | interview question 19: merge two ordered linked lists
- Sword finger offer | interview question 20: judge whether binary tree A contains subtree B
- Sword finger offer | interview question 21: mirror image of binary tree
- Sword finger offer | interview question 22: print matrix clockwise
- Sword finger offer | interview question 23: stack containing min function
- Sword finger offer | interview question 24: Press in and pop-up sequence of stack
- Sword finger offer | interview question 25: print binary tree from top to bottom
- Interview question 26: post order traversal sequence of binary search tree
- Sword finger offer | interview question 27: paths with a certain value in a binary tree
- Sword finger offer | interview question 28: replication of complex linked list
- Sword finger offer | interview question 29: convert binary search tree into two-way linked list
- Sword finger offer | interview question 30: arrangement of strings
- Sword finger offer | interview question 31: numbers that appear more than half in the array
- Sword finger offer | interview question 32: minimum number of k
- Sword finger offer | interview question 33: the maximum sum of continuous subarrays
- Sword finger offer | number of occurrences of 1 in interview question 34:1 ~ n integer
"Leetcode : https://leetcode-cn.com/problems/ba-shu-zu-pai-cheng-zui-xiao-de-shu-lcof/
"GitHub : https://github.com/nateshao/leetcode/blob/main/algo-notes/src/main/java/com/nateshao/sword_offer/topic_35_minNumber/Solution.java
Arrange the array into the smallest number
"Title Description: input a non negative integer array, splice all the numbers in the array into a number, and print the smallest of all the numbers that can be spliced. Difficulty: medium
Example 1:
input: [10,2] output: "102"
Example 2:
input: [3,30,34,5,9] output: "3033459"
Idea: this problem is essentially a sorting problem. If the string of any two numbers in array nums is x and y, the sorting judgment rule is specified as follows:
- If the splicing string x+y > y + X, then x is greater than y; (for example, x = "7",y="6"; x+y = "76" > y + x = "67", i.e. x > y: 7 > 6)
- Conversely, if x + y < y + X, X is less than y; X "less than" Y means: after sorting, X in the array should be on the Y side; "Greater than" is the opposite.
According to the above rules, you can apply any sorting method to sort nums.
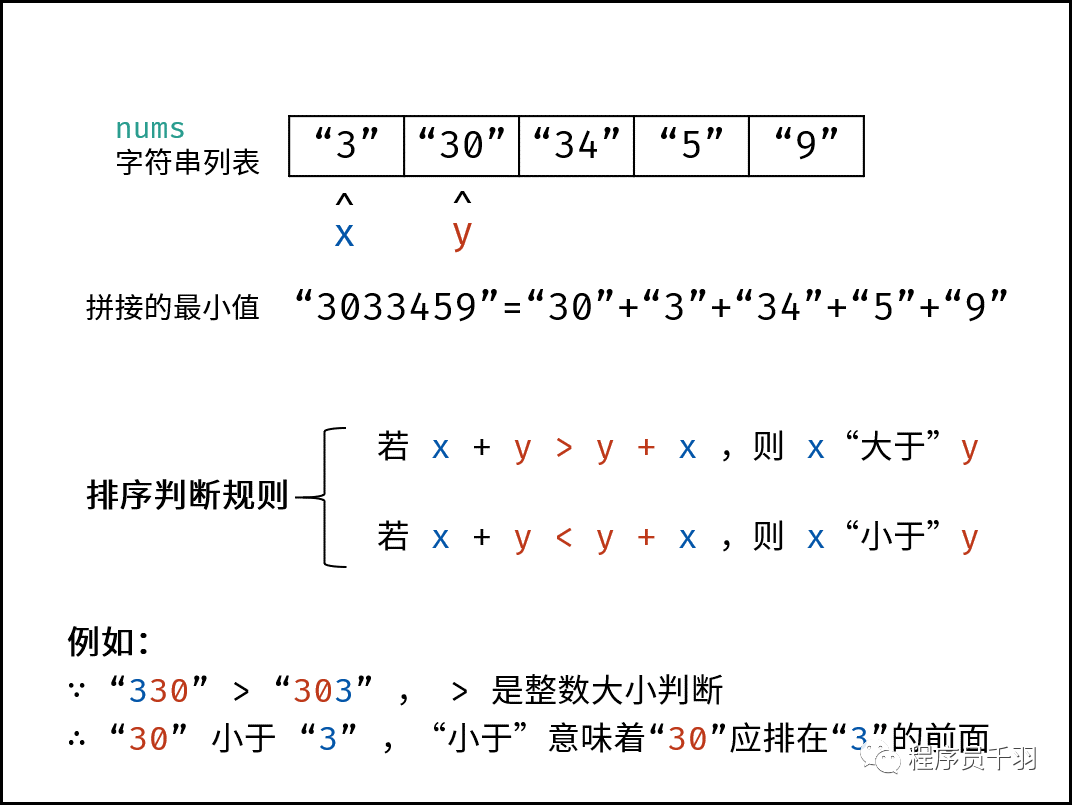
Algorithm flow:
- Initialization: String List strs, which saves the string format of each number;
- List sorting: use the above "sorting judgment rules" to sort strs;
- Return value: splice all strings in strs and return.
Complexity analysis:
- Time complexity O(NlogN): N is the number of characters of the final return value (length of strs list ≤ N); The average time complexity of using fast scheduling or built-in functions is O(NlogN), and the worst is O(N^2).
- Space complexity O(N): the string list strs takes up additional space of linear size.
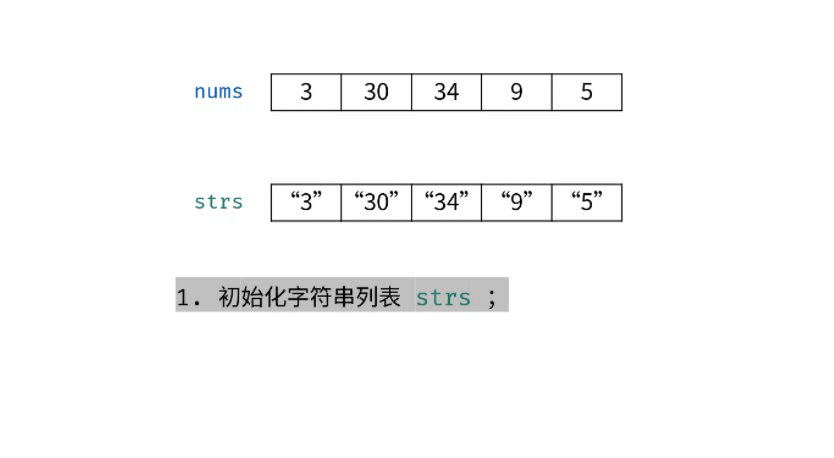
package com.nateshao.sword_offer.topic_35_minNumber; import java.util.Arrays; import java.util.Comparator; /** * @date Created by Shao Tongjie on 2021/12/11 17:03 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: Sword finger Offer 45 Arrange the array into the smallest number * https://leetcode-cn.com/problems/ba-shu-zu-pai-cheng-zui-xiao-de-shu-lcof/ */ public class Solution { public static void main(String[] args) { int[] nums = {10, 2}; int[] nums2 = {3, 30, 34, 5, 9}; System.out.println("minNumber(nums) = " + minNumber(nums));// minNumber(nums) = 102 System.out.println("minNumber(nums2) = " + minNumber(nums2));// minNumber(nums2) = 3033459 System.out.println("minNumber1(nums2) = " + minNumber1(nums2)); System.out.println("minNumber3(nums2) = " + minNumber3(nums2)); } public static String minNumber1(int[] nums) { String[] strs = new String[nums.length]; for(int i = 0; i < nums.length; i++) strs[i] = String.valueOf(nums[i]);// Convert to String type quickSort(strs, 0, strs.length - 1); StringBuilder res = new StringBuilder(); for(String s : strs){ res.append(s); } return res.toString(); } /** * Quick row * @param strs * @param left * @param right */ public static void quickSort(String[] strs, int left, int right) { if(left >= right) return; int i = left, j = right; String tmp = strs[i]; while(i < j) { while((strs[j] + strs[left]).compareTo(strs[left] + strs[j]) >= 0 && i < j) j--; while((strs[i] + strs[left]).compareTo(strs[left] + strs[i]) <= 0 && i < j) i++; tmp = strs[i]; strs[i] = strs[j]; strs[j] = tmp; } strs[i] = strs[left]; strs[left] = tmp; quickSort(strs, left, i - 1); quickSort(strs, i + 1, right); } /** * Idea: first convert the integer array into a String array, then sort the String array, and finally sort it * String array. The key is to make sorting rules. Or use the idea of comparison and fast platoon * The number of faces is compared with the last number. If it is small, it will be placed in the front, and finally called recursively. * @param nums * @return */ public static String minNumber(int[] nums) { if (nums == null || nums.length == 0) return ""; int len = nums.length; String[] str = new String[len]; StringBuilder sb = new StringBuilder(); for (int i = 0; i < len; i++) { str[i] = String.valueOf(nums[i]); } Arrays.sort(str, new Comparator<String>() { @Override public int compare(String s1, String s2) { String c1 = s1 + s2; String c2 = s2 + s1; return c1.compareTo(c2); } }); for (int i = 0; i < len; i++) { sb.append(str[i]); } return sb.toString(); } /** * greedy * @param nums * @return */ public static String minNumber3(int[] nums) { String[] strs = new String[nums.length]; for (int i = 0; i < nums.length; i++) { strs[i] = String.valueOf(nums[i]); } Arrays.sort(strs, (o1, o2) -> (o1 + o2).compareTo(o2 + o1)); StringBuilder sb = new StringBuilder(); for (String str : strs) { sb.append(str); } return sb.toString(); } }
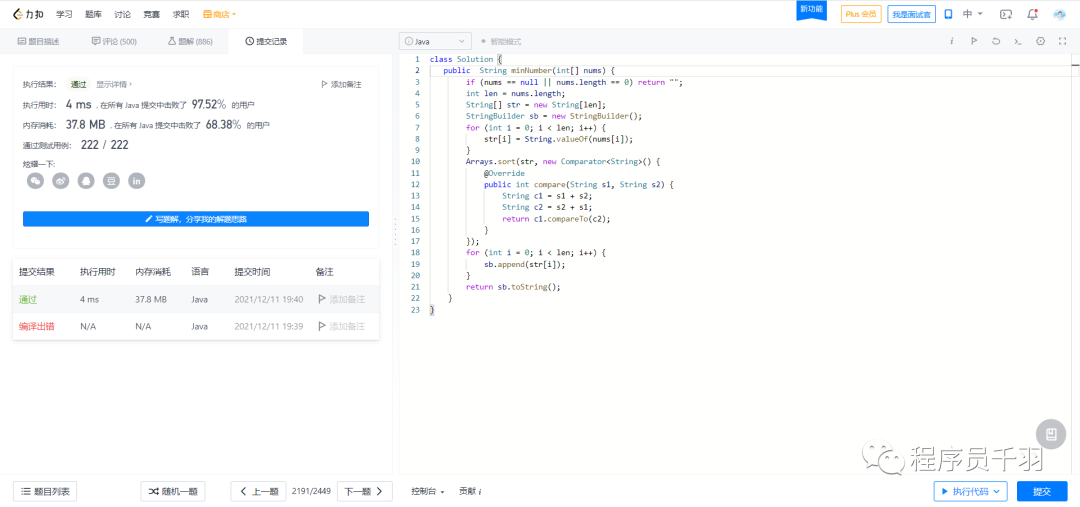
reference: https://leetcode-cn.com/problems/ba-shu-zu-pai-cheng-zui-xiao-de-shu-lcof/solution/mian-shi-ti-45-ba-shu-zu-pai-cheng-zui-xiao-de-s-4/