catalogue
Three structures of program
1. Sequential structure
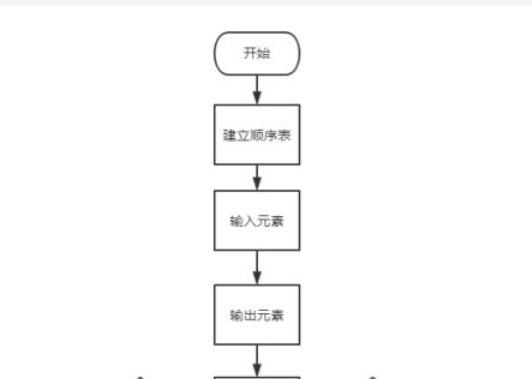
2. Select structure
In programming, we often need to use the selection structure to complete the logical judgment and selection function in the program. Here we need to use the selection statement.
Select statements in Java include
- if statement
- If else statement
- switch Statements
The selection statement is used to control the selection structure, judge the selection conditions, select the program statement to be executed according to the judgment results, and change the execution process of the program.
if select statement
Syntax format
If (conditional expression)
Statement
Or
If (conditional expression){
One or more statements
}
Code
public class Test { public static void main(String[] args) { int weekDay=5; if(weekDay==5){ System.out.println("Finally can rest!"); } if(weekDay<5) System.out.println("I have to work!"); } }
Structure diagram
If else selection statement
If else double branch statement
Syntax format
If (conditional expression)
Statement 1
else
Statement 2
Or
If (conditional expression){
Statement block 1
}else{
Statement block 2
}
Code example
public class Test { public static void main(String[] args) { int a = 2; int b = 3; int max; if(a>b){ max=a; }else{ max=b; } System.out.println(max); } }
Structure diagram
If else multi branch statement
Syntax format
If (conditional expression 1) {/ / if conditional expression 1 holds
Statement block 1 / / executes the code in statement block 1
}Else if (conditional expression 2) {/ / otherwise, if conditional expression 2 holds
Statement block 2 / / executes the code in statement block 2
}
... / / judge other conditions
Else if (conditional expression n-1) {/ / if conditional expression n-1 holds
Statement block n-1 / / executes the code in statement block n-1
}else{/ / if none of the above conditions holds
Statement block n / / execute statement block n
}
Code example
public class Test { public static void main(String[] args) { int score = 95; if(score>=90){ System.out.println("Excellent results"); }else if(score>=80){ System.out.println("Good results"); }else if(score>=70){ System.out.println("Average score"); }else if(score>=60){ System.out.println("Pass the grade"); }else{ System.out.println("Fail in grade"); } } }
Structure diagram
Switch multi branch switch statement
Syntax format
Switch (expression) {/ / byte char short int
case 10: statement group 1;
[ break;]
case constant expression 1: statement group 1;
[ break;]
...
case constant expression 1: statement group 1;
[ break;]
default: statement block n
}
Code example
public class Test { public static void main(String[] args) { char grade = 'A'; switch (grade){ case 'A': System.out.println("The score range is 90-100 branch"); case 'B': System.out.println("The score range is 80-89 branch"); case 'C': System.out.println("The score range is 70-179 branch"); case 'D': System.out.println("The score range is 60-69 branch"); case 'E': System.out.println("The score range is 0-59 branch"); default: System.out.println("The input level is incorrect, please re-enter!"); } } }
Structure diagram
3. Circulation structure
In a program, you can use circular statements when you want to do something repeatedly.
Java loop statements include:
- while loop statement
- Do while loop statement
- for loop statement
whlie loop statement
Syntax format
While (Boolean expression){
Statement or statement block
}
Sample code
public class Test { public static void main(String[] args) { int i=0; while (i<10){ System.out.println("The current number is"+i); i++; } } }
Structure diagram
Do while loop statement
Syntax format
do{
Statement or statement block
} while (Boolean expression);
Sample code
public class Test { public static void main(String[] args) { int i=0; do{ System.out.println("The current number is"+i); i++; } while (i<10); } }
Structure diagram
for loop statement
Syntax format
For (initialization expression; conditional expression; iteration statement){
Loop body statement
}
Sample code
public class Test { public static void main(String[] args) { for (int i = 0; i <10; i++) { System.out.println("The current number is"+i); } } }
Structure diagram
4. Process jump statement
Process jump statements are mainly used to jump out or end the loop structure. They are divided into break jump statements and continue jump statements.
break jump statement
Break statementyou can use a break statement in the loop body of a loop statement. If a break statement is executed in a loop, such as a loop statement that loops 50 times, the whole loop statement ends. It can also be used in a switch statement to exit the switch statement. The program executes from the first statement behind the switch structure.
Sample code
public class Test { public static void main(String[] args) { for (int i = 0; i <10; i++) { if(i>5){ break; } System.out.println("The current number is"+i); } } }
continue jump statement
The continue statement can only be used in the loop structure. It skips the statements that have not been executed in the loop body, restarts the next loop, and starts execution from the first statement in the loop body.
Sample code
public class Test { public static void main(String[] args) { for (int i = 0; i <10; i++) { if(i>5){ continue; } System.out.println("The current number is"+i); } } }