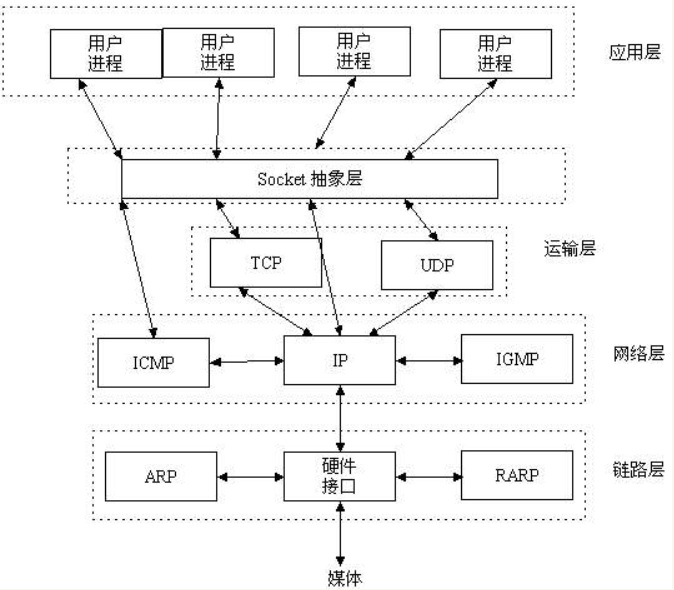
I. Socket sockets
- Two programs on the network communicate through a two-way connection to realize data exchange. One end of the connection is called a socket.
- Using socket to develop TCP program in java, this method can easily establish reliable, bidirectional, persistent, point-to-point communication connection.
- In the development of socket program, the server side uses ServerSocket to wait for the connection of the client. For the java network program, each client is represented by a socket object.
Code examples
2.1 Complete a communication between server and client
- Communicate with the client as a server.
- The server waits for the connection of the client and sends the data to the client after the connection.
Server
package com.rrs.socketserver;
import java.io.IOException;
import java.io.OutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import org.junit.Test;
/**
* @author lzx
* on 2019/9/25.
*/
public class SocketServer {
@Test
public void test01() throws IOException {
System.out.println("socket server start...");
ServerSocket serverSocket = new ServerSocket(8888);
Socket socket = serverSocket.accept();
System.out.println("Client detected socket: " + socket);
OutputStream out = socket.getOutputStream();
String mString = "Hello Client";
byte[] bytes = mString.getBytes();
out.write(bytes);
socket.shutdownOutput();
out.close();
}
}
Client
package com.rrs.sockerclient;
import java.io.IOException;
import java.io.InputStream;
import java.net.Socket;
import java.net.SocketAddress;
/**
* Created by lzx on 2019/9/25.
*/
public class SocketClient {
public static void main(String[] args) throws IOException {
System.out.println("socket client start...");
Socket socketClient = null;
socketClient = new Socket("127.0.0.1", 8888);
InputStream in = socketClient.getInputStream();
byte[] bytes = new byte[1024];
int len = in.read(bytes);
String msg = new String(bytes, 0, len);
System.out.println(msg);
}
}
2.2 Complete picture transmission from client to server
Server server waits for client to send pictures
package com.rrs.socketserver.server;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
import org.junit.Test;
/**
* @author lzx
*
*/
public class ServerPic {
@Test
public void serverPic() throws IOException {
System.out.println("server start...");
// Create socket
ServerSocket server = new ServerSocket(8899);
// Blocking listening client
Socket socket = server.accept();
// Get the input stream
InputStream in = socket.getInputStream();
byte[] buf = new byte[1024];
int len = 0;
// Reading while writing
// Write to server disk
// Get the file output stream
File file = new File("java.jpg");
FileOutputStream out = new FileOutputStream(file);
while ((len = in.read(buf)) != -1) {
out.write(buf, 0, len);
}
System.out.println("Picture Acceptance Completed...");
socket.shutdownInput();
in.close();
out.close();
}
}
Client client sends pictures
package com.rrs.socketserver.client;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.net.Socket;
import org.junit.Test;
/**
* @author lzx
*
*/
public class ClientPic {
@Test
public void clientPic() throws IOException {
System.out.println("client start...");
// Create a socket client
Socket client = new Socket("127.0.0.1", 8899);
// Send a file from a local read file (read while write)
// read file
File file = new File("D:/Desktop/java.jpg");
FileInputStream in = new FileInputStream(file);
// Send 1k at a time
byte[] buf = new byte[1024];
int len = 0;
OutputStream out = client.getOutputStream();
while ((len = in.read(buf)) != -1) {
out.write(buf, 0, len);
}
System.out.println("Picture Sending Completed...");
out.close();
in.close();
client.shutdownOutput();
}
}