In the development process, UISearchBar is a rare control. In general, we use the system native style:

But what UI designers might want is this:
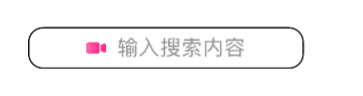
Maybe you think it's very simple: setting background color, border icon or something;
First look at setting the background color:
_It doesn't work if we set the backgroundcolor directly: because this directly sets the last layer view and the front layer gray view is UISearchBarBackground;
So using native backgroundColor does not work.
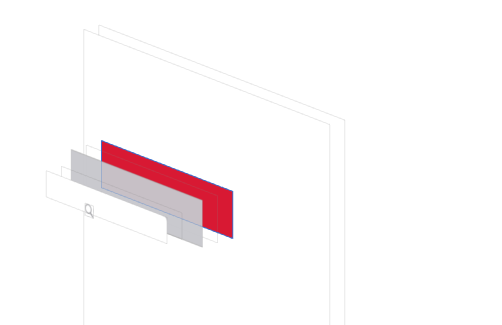
_You may find it very simple: Set the background of UISearchBarBackground directly, but let's look at the controls
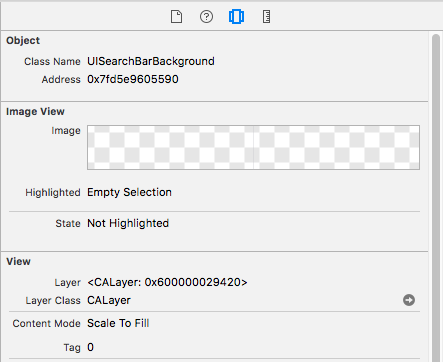
_He's an ImageView, and it doesn't work to set the background color directly. Apple only exposes a setImage interface to the UISearchBarBackground. So you can use the transformation of Image and Color to set it up.
- (void)setBackgroundColor:(UIColor *)backgroundColor
{
self.backgroundImage = [self createImageWithColor:backgroundColor];
}
- (void)setContentInset:(UIEdgeInsets)contentInset
{
_contentEdgeInsets = contentInset;
[self setNeedsDisplay];
}
- (UIImage *)createImageWithColor:(UIColor *)color
{
CGRect rect=CGRectMake(0.0f, 0.0f, 1.0f, 1.0f);
UIGraphicsBeginImageContext(rect.size);
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetFillColorWithColor(context, [color CGColor]);
CGContextFillRect(context, rect);
UIImage *theImage = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return theImage;
}
How to set UITextfield property:
For this TextField, UISearchBar is not used externally, but through view hierarchy:
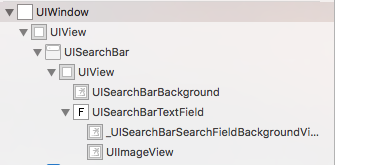
_We can get UITextField through the following ways: so that we can set the input font style, alignment, cursor properties and so on.
(1) Get by KVC key value;
self.searchTextField = [self valueForKey:@"_searchField"];
(2) Get the sub-view through the view;
if (_searchTextField == nil) {
for(UIView* view in self.subviews)
{
for(id subview in view.subviews) {
if([subview isKindOfClass:[UITextField class]])
{
_searchTextField = subview;
return subview;
}
}
}
}
_For the left view of the picture we can set, but the size? What if you want such a thing?

This requires us to rewrite the control. The control I rewrite includes the following interfaces and properties:
@interface SHSearchBar : UISearchBar
// Search input box for UITextField operation
// Modify font size, edit agent, etc.
@property (nonatomic, strong) UITextField *searchTextField;
// Set the background color of the search box;
@property (nonatomic, strong) UIColor *backgroundColor;
/* Set the left search View;
* Customize the search icon view.
* It could be Button imageView and so on. Search icon frame s, styles, backgrounds, and so on can be set through this View.
* Setting priority is higher than leftSearchIcon;
*/
@property (nonatomic, strong) UIView *leftSearchView;
// Set the icon of the left search button to modify only the picture.
@property (nonatomic, strong) UIImage *searchIcon;
// Set the icon of the clear button on the right to modify only the picture.
@property (nonatomic, strong) UIImage *clearIcon;
/* Setting EdgeInsets in the middle editing area,
* Default frame.origin == (8.0, 6.0); not equal to bounds of searchBar
* Textfild margins can be modified by setting Inset
*/
@property (nonatomic, assign) UIEdgeInsets contentEdgeInsets;
// Initialization control
- (instancetype)initWithFrame:(CGRect)frame;
// Set the offset of the left search button
- (void)setLeftSearchIconOffset:(UIOffset)offset;
// Set the Reect of the left search button to customize the layout
- (void)setLeftSearchIconRect:(CGRect)rect;
// Set the offset of the right clear button
- (void)setrithClearIconOffset:(UIOffset)offset;
- (void)setTintColor:(UIColor *)tintColor;
@end
If you like, please download it.
My GitHub code address: https://github.com/lvshaohua/SHSearchBar.git