Here we summarize some tips in iOS development, which can greatly facilitate our development and continue to update.
Top Blank Processing under the Group Style of UITableView
//Header blank processing of grouping list
UIView *view = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 0, 0.1)];
self.tableView.tableHeaderView = view;
Remove header stagnation in UITableView plain style
- (void)scrollViewDidScroll:(UIScrollView *)scrollView
{
CGFloat sectionHeaderHeight = sectionHead.height;
if (scrollView.contentOffset.y<=sectionHeaderHeight&&scrollView;.contentOffset.y>=0)
{
scrollView.contentInset = UIEdgeInsetsMake(-scrollView.contentOffset.y, 0, 0, 0);
}
else if(scrollView.contentOffset.y>=sectionHeaderHeight)
{
scrollView.contentInset = UIEdgeInsetsMake(-sectionHeaderHeight, 0, 0, 0);
}
}
Well, in fact, it's still Group style.
Get the controller where a view is located
- (UIViewController *)viewController
{
UIViewController *viewController = nil;
UIResponder *next = self.nextResponder;
while (next)
{
if ([next isKindOfClass:[UIViewController class]])
{
viewController = (UIViewController *)next;
break;
}
next = next.nextResponder;
}
return viewController;
}
Two methods to delete all records of NSUserDefaults
//Method 1
NSString *appDomain = [[NSBundle mainBundle] bundleIdentifier];
[[NSUserDefaults standardUserDefaults] removePersistentDomainForName:appDomain];
//Method two
- (void)resetDefaults
{
NSUserDefaults * defs = [NSUserDefaults standardUserDefaults];
NSDictionary * dict = [defs dictionaryRepresentation];
for (id key in dict)
{
[defs removeObjectForKey:key];
}
[defs synchronize];
}
Print all registered font names in the system
#pragma mark - Print all registered font names in the system
void enumerateFonts()
{
for(NSString *familyName in [UIFont familyNames])
{
NSLog(@"%@",familyName);
NSArray *fontNames = [UIFont fontNamesForFamilyName:familyName];
for(NSString *fontName in fontNames)
{
NSLog(@"\t|- %@",fontName);
}
}
}
Get the color of a point in the picture
- (UIColor*) getPixelColorAtLocation:(CGPoint)point inImage:(UIImage *)image
{
UIColor* color = nil;
CGImageRef inImage = image.CGImage;
CGContextRef cgctx = [self createARGBBitmapContextFromImage:inImage];
if (cgctx == NULL) {
return nil; /* error */
}
size_t w = CGImageGetWidth(inImage);
size_t h = CGImageGetHeight(inImage);
CGRect rect = {{0,0},{w,h}};
CGContextDrawImage(cgctx, rect, inImage);
unsigned char* data = CGBitmapContextGetData (cgctx);
if (data != NULL) {
int offset = 4*((w*round(point.y))+round(point.x));
int alpha = data[offset];
int red = data[offset+1];
int green = data[offset+2];
int blue = data[offset+3];
color = [UIColor colorWithRed:(red/255.0f) green:(green/255.0f) blue:
(blue/255.0f) alpha:(alpha/255.0f)];
}
CGContextRelease(cgctx);
if (data) {
free(data);
}
return color;
}
String inversion
The first is:
- (NSString *)reverseWordsInString:(NSString *)str
{
NSMutableString *newString = [[NSMutableString alloc] initWithCapacity:str.length];
for (NSInteger i = str.length - 1; i >= 0 ; i --)
{
unichar ch = [str characterAtIndex:i];
[newString appendFormat:@"%c", ch];
}
return newString;
}
//Second species:
- (NSString*)reverseWordsInString:(NSString*)str
{
NSMutableString *reverString = [NSMutableString stringWithCapacity:str.length];
[str enumerateSubstringsInRange:NSMakeRange(0, str.length) options:NSStringEnumerationReverse | NSStringEnumerationByComposedCharacterSequences usingBlock:^(NSString *substring, NSRange substringRange, NSRange enclosingRange, BOOL *stop) {
[reverString appendString:substring];
}];
return reverString;
}
No locking screen.
By default, iOS locks the screen when the device has no touch action for a period of time. But there are some applications that don't need to lock the screen, such as video players.
[UIApplication sharedApplication].idleTimerDisabled = YES;
//or
[[UIApplication sharedApplication] setIdleTimerDisabled:YES];
Modal Push-out Transparent Interface
UIViewController *vc = [[UIViewController alloc] init];
UINavigationController *na = [[UINavigationController alloc] initWithRootViewController:vc];
if ([[[UIDevice currentDevice] systemVersion] floatValue] >= 8.0)
{
na.modalPresentationStyle = UIModalPresentationOverCurrentContext;
}
else
{
self.modalPresentationStyle=UIModalPresentationCurrentContext;
}
[self presentViewController:na animated:YES completion:nil];
Xcode debugging does not show memory footprint
There is an option in editSCheme called enable zoombie Objects unchecked
Display hidden files
//display
defaults write com.apple.finder AppleShowAllFiles -bool true
killall Finder
//hide
defaults write com.apple.finder AppleShowAllFiles -bool false
killall Finder
Strings are segmented by multiple symbols

iOS Jump to App Store Download App Score
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:@"itms-apps://itunes.apple.com/WebObjects/MZStore.woa/wa/viewContentsUserReviews?type=Purple+Software&id=APPID"]];
iOS Acquisition of Pinyin of Chinese Characters
+ (NSString *)transform:(NSString *)chinese
{
//Replace NSString with NSMutable String
NSMutableString *pinyin = [chinese mutableCopy];
//Converting Chinese Characters into Pinyin (with Phonetic Alphabets)
CFStringTransform((__bridge CFMutableStringRef)pinyin, NULL, kCFStringTransformMandarinLatin, NO);
NSLog(@"%@", pinyin);
//Remove the phonetic alphabet
CFStringTransform((__bridge CFMutableStringRef)pinyin, NULL, kCFStringTransformStripCombiningMarks, NO);
NSLog(@"%@", pinyin);
//Return the latest result
return pinyin;
}
Manually change the color of the iOS status bar
- (void)setStatusBarBackgroundColor:(UIColor *)color
{
UIView *statusBar = [[[UIApplication sharedApplication] valueForKey:@"statusBarWindow"] valueForKey:@"statusBar"];
if ([statusBar respondsToSelector:@selector(setBackgroundColor:)])
{
statusBar.backgroundColor = color;
}
}
Determine whether the current ViewController is push ed or present ed
NSArray *viewcontrollers=self.navigationController.viewControllers;
if (viewcontrollers.count > 1)
{
if ([viewcontrollers objectAtIndex:viewcontrollers.count - 1] == self)
{
//push mode
[self.navigationController popViewControllerAnimated:YES];
}
}
else
{
//present mode
[self dismissViewControllerAnimated:YES completion:nil];
}
Get the actual Launch Image image
- (NSString *)getLaunchImageName
{
CGSize viewSize = self.window.bounds.size;
// Vertical screen
NSString *viewOrientation = @"Portrait";
NSString *launchImageName = nil;
NSArray* imagesDict = [[[NSBundle mainBundle] infoDictionary] valueForKey:@"UILaunchImages"];
for (NSDictionary* dict in imagesDict)
{
CGSize imageSize = CGSizeFromString(dict[@"UILaunchImageSize"]);
if (CGSizeEqualToSize(imageSize, viewSize) && [viewOrientation isEqualToString:dict[@"UILaunchImageOrientation"]])
{
launchImageName = dict[@"UILaunchImageName"];
}
}
return launchImageName;
}
iOS gets the first response on the current screen
UIWindow * keyWindow = [[UIApplication sharedApplication] keyWindow];
UIView * firstResponder = [keyWindow performSelector:@selector(firstResponder)];
Determine whether an object has complied with a protocol
if ([self.selectedController conformsToProtocol:@protocol(RefreshPtotocol)])
{
[self.selectedController performSelector:@selector(onTriggerRefresh)];
}
Determine whether view is a subview of the specified view?
BOOL isView = [textView isDescendantOfView:self.view];
NSArray Quickly Finds the Maximum, Minimum and Mean Values
NSArray *array = [NSArray arrayWithObjects:@"2.0", @"2.3", @"3.0", @"4.0", @"10", nil];
CGFloat sum = [[array valueForKeyPath:@"@sum.floatValue"] floatValue];
CGFloat avg = [[array valueForKeyPath:@"@avg.floatValue"] floatValue];
CGFloat max =[[array valueForKeyPath:@"@max.floatValue"] floatValue];
CGFloat min =[[array valueForKeyPath:@"@min.floatValue"] floatValue];
NSLog(@"%f\n%f\n%f\n%f",sum,avg,max,min);
Modify the text color of Placeholder in UITextField
[textField setValue:[UIColor redColor] forKeyPath:@"_placeholderLabel.textColor"];
Format of NSDateFormatter
G: A.D. Age, e.g. AD yy: the last two places in the year yyyy: the whole year MM: Month, 1-12 MMM: Month, Shown as English Month Abbreviations, e.g. Jan MMMM: Month, shown as the full name of the English month, such as Janualy dd: Day, 2-digit representation, e.g. 02 d: day, 1-2 bit display, such as 2 EEE: Short for what day of the week, such as Sun EEEE: Write all the days of the week, such as Sunday aa: AM/PM in the afternoon H: Hours, 24-hour system, 0-23 K: Hours, 12 hours, 0-11 m: points, 1-2 bits mm: Score, 2 bits s: seconds, 1-2 bits ss: seconds, 2 bits S: milliseconds
Get all subclasses of a class
+ (NSArray *) allSubclasses
{
Class myClass = [self class];
NSMutableArray *mySubclasses = [NSMutableArray array];
unsigned int numOfClasses;
Class *classes = objc_copyClassList(&numOfClasses;);
for (unsigned int ci = 0; ci < numOfClasses; ci++)
{
Class superClass = classes[ci];
do{
superClass = class_getSuperclass(superClass);
} while (superClass && superClass != myClass);
if (superClass)
{
[mySubclasses addObject: classes[ci]];
}
}
free(classes);
return mySubclasses;
}
To monitor whether IOS devices have agents, CFNetwork.framework is required.
NSDictionary *proxySettings = (__bridge NSDictionary *)(CFNetworkCopySystemProxySettings());
NSArray *proxies = (__bridge NSArray *)(CFNetworkCopyProxiesForURL((__bridge CFURLRef _Nonnull)([NSURL URLWithString:@"http://www.baidu.com"]), (__bridge CFDictionaryRef _Nonnull)(proxySettings)));
NSLog(@"\n%@",proxies);
NSDictionary *settings = proxies[0];
NSLog(@"%@",[settings objectForKey:(NSString *)kCFProxyHostNameKey]);
NSLog(@"%@",[settings objectForKey:(NSString *)kCFProxyPortNumberKey]);
NSLog(@"%@",[settings objectForKey:(NSString *)kCFProxyTypeKey]);
if ([[settings objectForKey:(NSString *)kCFProxyTypeKey] isEqualToString:@"kCFProxyTypeNone"])
{
NSLog(@"No agent");
}
else
{
NSLog(@"Agents are set up");
}
Arabic numeral to Chinese format
+(NSString *)translation:(NSString *)arebic
{
NSString *str = arebic;
NSArray *arabic_numerals = @[@"1",@"2",@"3",@"4",@"5",@"6",@"7",@"8",@"9",@"0"];
NSArray *chinese_numerals = @[@"One",@"Two",@"Three",@"Four",@"Five",@"Six",@"Seven",@"Eight",@"Nine",@"Zero"];
NSArray *digits = @[@"individual",@"Ten",@"hundred",@"thousand",@"ten thousand",@"Ten",@"hundred",@"thousand",@"Billion",@"Ten",@"hundred",@"thousand",@"Trillion"];
NSDictionary *dictionary = [NSDictionary dictionaryWithObjects:chinese_numerals forKeys:arabic_numerals];
NSMutableArray *sums = [NSMutableArray array];
for (int i = 0; i < str.length; i ++) {
NSString *substr = [str substringWithRange:NSMakeRange(i, 1)];
NSString *a = [dictionary objectForKey:substr];
NSString *b = digits[str.length -i-1];
NSString *sum = [a stringByAppendingString:b];
if ([a isEqualToString:chinese_numerals[9]])
{
if([b isEqualToString:digits[4]] || [b isEqualToString:digits[8]])
{
sum = b;
if ([[sums lastObject] isEqualToString:chinese_numerals[9]])
{
[sums removeLastObject];
}
}else
{
sum = chinese_numerals[9];
}
if ([[sums lastObject] isEqualToString:sum])
{
continue;
}
}
[sums addObject:sum];
}
NSString *sumStr = [sums componentsJoinedByString:@""];
NSString *chinese = [sumStr substringToIndex:sumStr.length-1];
NSLog(@"%@",str);
NSLog(@"%@",chinese);
return chinese;
}
Conversion of Base64 Encoding to NSString Object or NSData Object
// Create NSData object
NSData *nsdata = [@"iOS Developer Tips encoded in Base64"
dataUsingEncoding:NSUTF8StringEncoding];
// Get NSString from NSData object in Base64
NSString *base64Encoded = [nsdata base64EncodedStringWithOptions:0];
// Print the Base64 encoded string
NSLog(@"Encoded: %@", base64Encoded);
// Let's go the other way...
// NSData from the Base64 encoded str
NSData *nsdataFromBase64String = [[NSData alloc]
initWithBase64EncodedString:base64Encoded options:0];
// Decoded NSString from the NSData
NSString *base64Decoded = [[NSString alloc]
initWithData:nsdataFromBase64String encoding:NSUTF8StringEncoding];
NSLog(@"Decoded: %@", base64Decoded);
Cancel the implicit animation of UICollectionView
UICollectionView adds an implicit fade animation to reloadItems by default, which is sometimes annoying, especially when your cell is a composite cell (for example, when the cell uses UIStackView).
Here are several ways to help you get rid of these animations
//Method 1
[UIView performWithoutAnimation:^{
[collectionView reloadItemsAtIndexPaths:@[[NSIndexPath indexPathForItem:index inSection:0]]];
}];
//Method two
[UIView animateWithDuration:0 animations:^{
[collectionView performBatchUpdates:^{
[collectionView reloadItemsAtIndexPaths:@[[NSIndexPath indexPathForItem:index inSection:0]]];
} completion:nil];
}];
//Method three
[UIView setAnimationsEnabled:NO];
[self.trackPanel performBatchUpdates:^{
[collectionView reloadItemsAtIndexPaths:@[[NSIndexPath indexPathForItem:index inSection:0]]];
} completion:^(BOOL finished) {
[UIView setAnimationsEnabled:YES];
}];
Let the Xcode console support LLDB-type printing
Open the terminal and enter three commands: touch ~/.lldbinit echo display @import UIKit >> ~/.lldbinit echo target stop-hook add -o \"target stop-hook disable\" >> ~/.lldbinit
Slow updating of Cocoa Pods pod install/pod update
pod install --verbose --no-repo-update pod update --verbose --no-repo-update Without the latter parameter, the spec repository of CocoaPods will be upgraded by default, and this step can be omitted by adding one parameter, and then the speed will increase a lot.
Memory footprint of UIImage
UIImage *image = [UIImage imageNamed:@"aa"];
NSUInteger size = CGImageGetHeight(image.CGImage) * CGImageGetBytesPerRow(image.CGImage);
GCD timer timer
dispatch_queue_t queue = dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0);
dispatch_source_t timer = dispatch_source_create(DISPATCH_SOURCE_TYPE_TIMER, 0, 0,queue);
dispatch_source_set_timer(timer,dispatch_walltime(NULL, 0),1.0*NSEC_PER_SEC, 0); //Execution per second
dispatch_source_set_event_handler(timer, ^{
//@ "Close the countdown."
dispatch_source_cancel(timer);
dispatch_async(dispatch_get_main_queue(), ^{
});
});
dispatch_resume(timer);
Draw text on the picture and write a category of UIImage
- (UIImage *)imageWithTitle:(NSString *)title fontSize:(CGFloat)fontSize
{
//canvas size
CGSize size=CGSizeMake(self.size.width,self.size.height);
//Create a bitmap-based context
UIGraphicsBeginImageContextWithOptions(size,NO,0.0);//opaque:NO scale:0.0
[self drawAtPoint:CGPointMake(0.0,0.0)];
//The text is centered on the canvas.
NSMutableParagraphStyle* paragraphStyle = [[NSParagraphStyle defaultParagraphStyle] mutableCopy];
paragraphStyle.lineBreakMode = NSLineBreakByCharWrapping;
paragraphStyle.alignment=NSTextAlignmentCenter;//center
//Calculate the size of the text and center it on the canvas.
CGSize sizeText=[title boundingRectWithSize:self.size options:NSStringDrawingUsesLineFragmentOrigin
attributes:@{NSFontAttributeName:[UIFont systemFontOfSize:fontSize]}context:nil].size;
CGFloat width = self.size.width;
CGFloat height = self.size.height;
CGRect rect = CGRectMake((width-sizeText.width)/2, (height-sizeText.height)/2, sizeText.width, sizeText.height);
//Draw text
[title drawInRect:rect withAttributes:@{ NSFontAttributeName:[UIFont systemFontOfSize:fontSize],NSForegroundColorAttributeName:[ UIColor whiteColor],NSParagraphStyleAttributeName:paragraphStyle}];
//Returns a new drawing
UIImage *newImage= UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return newImage;
}
Find all subviews of a view
- (NSMutableArray *)allSubViewsForView:(UIView *)view
{
NSMutableArray *array = [NSMutableArray arrayWithCapacity:0];
for (UIView *subView in view.subviews)
{
[array addObject:subView];
if (subView.subviews.count > 0)
{
[array addObjectsFromArray:[self allSubViewsForView:subView]];
}
}
return array;
}
Calculate file size
//file size
- (long long)fileSizeAtPath:(NSString *)path
{
NSFileManager *fileManager = [NSFileManager defaultManager];
if ([fileManager fileExistsAtPath:path])
{
long long size = [fileManager attributesOfItemAtPath:path error:nil].fileSize;
return size;
}
return 0;
}
//Folder size
- (long long)folderSizeAtPath:(NSString *)path
{
NSFileManager *fileManager = [NSFileManager defaultManager];
long long folderSize = 0;
if ([fileManager fileExistsAtPath:path])
{
NSArray *childerFiles = [fileManager subpathsAtPath:path];
for (NSString *fileName in childerFiles)
{
NSString *fileAbsolutePath = [path stringByAppendingPathComponent:fileName];
if ([fileManager fileExistsAtPath:fileAbsolutePath])
{
long long size = [fileManager attributesOfItemAtPath:fileAbsolutePath error:nil].fileSize;
folderSize += size;
}
}
}
return folderSize;
}
UIView Sets Partial Corners
Have you ever had this problem, a button or label, just two corners on the right, or just one corner? What should we do? This requires a layer mask to help us.
CGRect rect = view.bounds;
CGSize radio = CGSizeMake(30, 30);//Fillet size
UIRectCorner corner = UIRectCornerTopLeft|UIRectCornerTopRight;//This rounded corner position
UIBezierPath *path = [UIBezierPath bezierPathWithRoundedRect:rect byRoundingCorners:corner cornerRadii:radio];
CAShapeLayer *masklayer = [[CAShapeLayer alloc]init];//Create shapelayer
masklayer.frame = view.bounds;
masklayer.path = path.CGPath;//set up path
view.layer.mask = masklayer;
Take up and take down the whole
Floor(x), sometimes referred to as Floor(x), has the function of "downtaking integers", i.e. taking the largest integers not larger than x, for example: x=3.14,floor(x)=3 y=9.99999,floor(y)=9 The floor function corresponds to the ceil function, i.e. the upper integral function. The function of the ceil function is to find the smallest integer not less than a given real number. ceil(2)=ceil(1.2)=cei(1.5)=2.00 The return values of floor function and ceil function are double
Calculate the length of the string character, one Chinese character for two characters
//Method 1:
- (int)convertToInt:(NSString*)strtemp
{
int strlength = 0;
char* p = (char*)[strtemp cStringUsingEncoding:NSUnicodeStringEncoding];
for (int i=0 ; i<[strtemp lengthOfBytesUsingEncoding:NSUnicodeStringEncoding] ;i++)
{
if (*p)
{
p++;
strlength++;
}
else
{
p++;
}
}
return strlength;
}
//Method two:
-(NSUInteger) unicodeLengthOfString: (NSString *) text
{
NSUInteger asciiLength = 0;
for (NSUInteger i = 0; i < text.length; i++)
{
unichar uc = [text characterAtIndex: i];
asciiLength += isascii(uc) ? 1 : 2;
}
return asciiLength;
}
Set up pictures for UIView
UIImage *image = [UIImage imageNamed:@"image"];
self.MYView.layer.contents = (__bridge id _Nullable)(image.CGImage);
self.MYView.layer.contentsRect = CGRectMake(0, 0, 0.5, 0.5);
Prevent scrollView gesture from covering sideslip gesture
[scrollView.panGestureRecognizerrequireGestureRecognizerToFail:self.navigationController.interactivePopGestureRecognizer];
Remove the back heading returned from the navigation bar
[[UIBarButtonItemappearance]setBackButtonTitlePositionAdjustment:UIOffsetMake(0, -60)forBarMetrics:UIBarMetricsDefault];
Does the string contain Chinese?
+ (BOOL)checkIsChinese:(NSString *)string
{
for (int i=0; i<string.length; i++)
{
unichar ch = [string characterAtIndex:i];
if (0x4E00 <= ch && ch <= 0x9FA5)
{
return YES;
}
}
return NO;
}
Use of dispatch_group
dispatch_group_t dispatchGroup = dispatch_group_create();
dispatch_group_enter(dispatchGroup);
dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(1 * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{
NSLog(@"First request completed");
dispatch_group_leave(dispatchGroup);
});
dispatch_group_enter(dispatchGroup);
dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(10 * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{
NSLog(@"The second request is completed");
dispatch_group_leave(dispatchGroup);
});
dispatch_group_notify(dispatchGroup, dispatch_get_main_queue(), ^(){
NSLog(@"Request completed");
});
UITextField adds a space every four bits to implement proxy
- (BOOL)textField:(UITextField *)textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string
{
// Four digits plus one space
if ([string isEqualToString:@""])
{
// Delete character
if ((textField.text.length - 2) % 5 == 0)
{
textField.text = [textField.text substringToIndex:textField.text.length - 1];
}
return YES;
}
else
{
if (textField.text.length % 5 == 0)
{
textField.text = [NSString stringWithFormat:@"%@ ", textField.text];
}
}
return YES;
}
Get private attributes and member variables # import <objc/runtime.h>.
//Get private properties such as setting the font color of UIDatePicker
- (void)setTextColor
{
//Get all the attributes to see if there are corresponding attributes
unsigned int count = 0;
objc_property_t *propertys = class_copyPropertyList([UIDatePicker class], &count);
for(int i = 0;i < count;i ++)
{
//Get each attribute
objc_property_t property = propertys[i];
//Get the nsstring corresponding to the attribute
NSString *propertyName = [NSString stringWithCString:property_getName(property) encoding:NSUTF8StringEncoding];
//Output printing to see the corresponding properties
NSLog(@"propertyname = %@",propertyName);
if ([propertyName isEqualToString:@"textColor"])
{
[datePicker setValue:[UIColor whiteColor] forKey:propertyName];
}
}
}
//Get member variables such as modifying the button font color of UIAlert Action
unsigned int count = 0;
Ivar *ivars = class_copyIvarList([UIAlertAction class], &count);
for(int i =0;i < count;i ++)
{
Ivar ivar = ivars[i];
NSString *ivarName = [NSString stringWithCString:ivar_getName(ivar) encoding:NSUTF8StringEncoding];
NSLog(@"uialertion.ivarName = %@",ivarName);
if ([ivarName isEqualToString:@"_titleTextColor"])
{
[alertOk setValue:[UIColor blueColor] forKey:@"titleTextColor"];
[alertCancel setValue:[UIColor purpleColor] forKey:@"titleTextColor"];
}
}
Getting Applications for Mobile Installation
Class c =NSClassFromString(@"LSApplicationWorkspace");
id s = [(id)c performSelector:NSSelectorFromString(@"defaultWorkspace")];
NSArray *array = [s performSelector:NSSelectorFromString(@"allInstalledApplications")];
for (id item in array)
{
NSLog(@"%@",[item performSelector:NSSelectorFromString(@"applicationIdentifier")]);
//NSLog(@"%@",[item performSelector:NSSelectorFromString(@"bundleIdentifier")]);
NSLog(@"%@",[item performSelector:NSSelectorFromString(@"bundleVersion")]);
NSLog(@"%@",[item performSelector:NSSelectorFromString(@"shortVersionString")]);
}
Determine whether two dates are written in NSDate's category in the same week
- (BOOL)isSameDateWithDate:(NSDate *)date
{
//Return NO between more than seven days
if (fabs([self timeIntervalSinceDate:date]) >= 7 * 24 *3600)
{
return NO;
}
NSCalendar *calender = [NSCalendar currentCalendar];
calender.firstWeekday = 2;//Set up the first day of the week from Monday
//Calculate two dates for each week of the year
NSUInteger countSelf = [calender ordinalityOfUnit:NSCalendarUnitWeekday inUnit:NSCalendarUnitYear forDate:self];
NSUInteger countDate = [calender ordinalityOfUnit:NSCalendarUnitWeekday inUnit:NSCalendarUnitYear forDate:date];
//Equality is in the same week, inequality is not in the same week.
return countSelf == countDate;
}
Open System Setup Interface in Application
//After iOS8
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:UIApplicationOpenSettingsURLString]];
//If App does not add permissions, the settings interface is displayed. If App has additional permissions (such as notifications), the App settings interface is displayed.
//Before iOS8
//First add a url type as shown below. Call the following code in the code to jump to the corresponding item in the settings page.
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:@"prefs:root=WIFI"]];
//The optional values are as follows:
About — prefs:root=General&path=About
Accessibility — prefs:root=General&path=ACCESSIBILITY
Airplane Mode On — prefs:root=AIRPLANE_MODE
Auto-Lock — prefs:root=General&path=AUTOLOCK
Brightness — prefs:root=Brightness
Bluetooth — prefs:root=General&path=Bluetooth
Date & Time — prefs:root=General&path=DATE_AND_TIME
FaceTime — prefs:root=FACETIME
General — prefs:root=General
Keyboard — prefs:root=General&path=Keyboard
iCloud — prefs:root=CASTLE
iCloud Storage & Backup — prefs:root=CASTLE&path=STORAGE_AND_BACKUP
International — prefs:root=General&path=INTERNATIONAL
Location Services — prefs:root=LOCATION_SERVICES
Music — prefs:root=MUSIC
Music Equalizer — prefs:root=MUSIC&path=EQ
Music Volume Limit — prefs:root=MUSIC&path=VolumeLimit
Network — prefs:root=General&path=Network
Nike + iPod — prefs:root=NIKE_PLUS_IPOD
Notes — prefs:root=NOTES
Notification — prefs:root=NOTIFICATI*****_ID
Phone — prefs:root=Phone
Photos — prefs:root=Photos
Profile — prefs:root=General&path=ManagedConfigurationList
Reset — prefs:root=General&path=Reset
Safari — prefs:root=Safari
Siri — prefs:root=General&path=Assistant
Sounds — prefs:root=Sounds
Software Update — prefs:root=General&path=SOFTWARE_UPDATE_LINK
Store — prefs:root=STORE
Twitter — prefs:root=TWITTER
Usage — prefs:root=General&path=USAGE
VPN — prefs:root=General&path=Network/VPN
Wallpaper — prefs:root=Wallpaper
Wi-Fi — prefs:root=WIFI
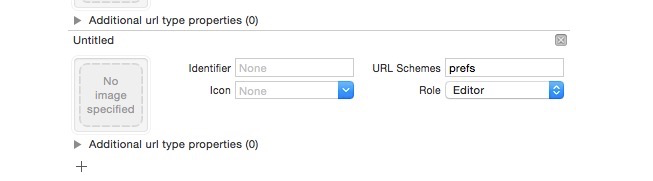
Stop animation and start again
-(void)pauseLayer:(CALayer *)layer
{
CFTimeInterval pausedTime = [layer convertTime:CACurrentMediaTime() fromLayer:nil];
layer.speed = 0.0;
layer.timeOffset = pausedTime;
}
-(void)resumeLayer:(CALayer *)layer
{
CFTimeInterval pausedTime = [layer timeOffset];
layer.speed = 1.0;
layer.timeOffset = 0.0;
layer.beginTime = 0.0;
CFTimeInterval timeSincePause = [layer convertTime:CACurrentMediaTime() fromLayer:nil] - pausedTime;
layer.beginTime = timeSincePause;
}
fillRule principle
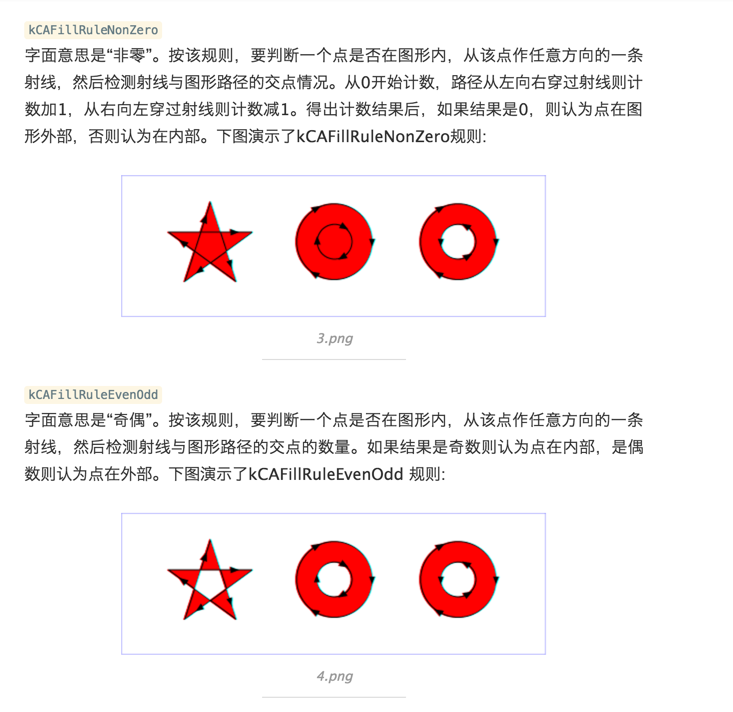
Formatting of Numbers in iOS
//Through NSNumberFormatter, the format of NSNumber output can also be set. For example, the following code:
NSNumberFormatter *formatter = [[NSNumberFormatter alloc] init];
formatter.numberStyle = NSNumberFormatterDecimalStyle;
NSString *string = [formatter stringFromNumber:[NSNumber numberWithInt:123456789]];
NSLog(@"Formatted number string:%@",string);
//The output is: [1223:403] Formatted number string:123,456,789
//Among them, the NSNumberFormatter class has an attribute numberStyle, which is an enumeration type. Setting different values can output different digital formats. The enumeration includes:
typedef NS_ENUM(NSUInteger, NSNumberFormatterStyle) {
NSNumberFormatterNoStyle = kCFNumberFormatterNoStyle,
NSNumberFormatterDecimalStyle = kCFNumberFormatterDecimalStyle,
NSNumberFormatterCurrencyStyle = kCFNumberFormatterCurrencyStyle,
NSNumberFormatterPercentStyle = kCFNumberFormatterPercentStyle,
NSNumberFormatterScientificStyle = kCFNumberFormatterScientificStyle,
NSNumberFormatterSpellOutStyle = kCFNumberFormatterSpellOutStyle
};
//The effect of each enumeration on the output digital format is as follows: the output of the third and last item will vary according to the language area set by the system.
[1243:403] Formatted number string:123456789
[1243:403] Formatted number string:123,456,789
[1243:403] Formatted number string:¥123,456,789.00
[1243:403] Formatted number string:-539,222,988%
[1243:403] Formatted number string:1.23456789E8
[1243:403] Formatted number string:One hundred and twenty-three thousand forty-five thousand seven hundred and eighty-nine
How to get all the picture addresses of WebView?
When the web page is loaded, the way to get pictures and add clicks is identified by js
//UIWebView
- (void)webViewDidFinishLoad:(UIWebView *)webView
{
//Here is js, the main implementation of url acquisition
static NSString * const jsGetImages =
@"function getImages(){\
var objs = document.getElementsByTagName(\"img\");\
var imgScr = '';\
for(var i=0;i<objs.length;i++){\
imgScr = imgScr + objs[i].src + '+';\
};\
return imgScr;\
};";
[webView stringByEvaluatingJavaScriptFromString:jsGetImages];//Injection js method
NSString *urlResult = [webView stringByEvaluatingJavaScriptFromString:@"getImages()"];
NSArray *urlArray = [NSMutableArray arrayWithArray:[urlResult componentsSeparatedByString:@"+"]];
//urlResurlt is a mosaic of URLs that get all the pictures; mUrlArray is an array of all Urls.
}
//WKWebView
- (void)webView:(WKWebView *)webView didFinishNavigation:(null_unspecified WKNavigation *)navigation
{
static NSString * const jsGetImages =
@"function getImages(){\
var objs = document.getElementsByTagName(\"img\");\
var imgScr = '';\
for(var i=0;i<objs.length;i++){\
imgScr = imgScr + objs[i].src + '+';\
};\
return imgScr;\
};";
[webView evaluateJavaScript:jsGetImages completionHandler:nil];
[webView evaluateJavaScript:@"getImages()" completionHandler:^(id _Nullable result, NSError * _Nullable error) {
NSLog(@"%@",result);
}];
}
Get the height of the webview
CGFloat height = [[self.webView stringByEvaluatingJavaScriptFromString:@"document.body.offsetHeight"] floatValue];
Navigation Bar Turns Pure Transparency
//The first method
//Navigation Bar Pure Transparency
[self.navigationBar setBackgroundImage:[UIImage new] forBarMetrics:UIBarMetricsDefault];
//Remove the black line at the bottom of the navigation bar
self.navigationBar.shadowImage = [UIImage new];
//The second method
[[self.navigationBar subviews] objectAtIndex:0].alpha = 0;
tabBar is the same.
[self.tabBar setBackgroundImage:[UIImage new]];
self.tabBar.shadowImage = [UIImage new];
Realization of Navigation Bar Based on Gradual Change of Sliding Distance
//First kind
- (void)scrollViewDidScroll:(UIScrollView *)scrollView
{
CGFloat offsetToShow = 200.0;//Slide as much as you want to show.
CGFloat alpha = 1 - (offsetToShow - scrollView.contentOffset.y) / offsetToShow;
[[self.navigationController.navigationBar subviews] objectAtIndex:0].alpha = alpha;
}
//Second kinds
- (void)scrollViewDidScroll:(UIScrollView *)scrollView
{
CGFloat offsetToShow = 200.0;
CGFloat alpha = 1 - (offsetToShow - scrollView.contentOffset.y) / offsetToShow;
[self.navigationController.navigationBar setShadowImage:[UIImage new]];
[self.navigationController.navigationBar setBackgroundImage:[self imageWithColor:[[UIColor orangeColor]colorWithAlphaComponent:alpha]] forBarMetrics:UIBarMetricsDefault];
}
//Generate a solid color picture
- (UIImage *)imageWithColor:(UIColor *)color
{
CGRect rect = CGRectMake(0.0f, 0.0f, 1.0f, 1.0f);
UIGraphicsBeginImageContext(rect.size);
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetFillColorWithColor(context, [color CGColor]);
CGContextFillRect(context, rect);
UIImage *theImage = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return theImage;
}
Some related paths in iOS development
The position of the simulator: /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneSimulator.platform/Developer/SDKs Document installation location: /Applications/Xcode.app/Contents/Developer/Documentation/DocSets Plug-in save path: ~/Library/ApplicationSupport/Developer/Shared/Xcode/Plug-ins Customize the save path of the code snippet: ~/Library/Developer/Xcode/UserData/CodeSnippets/ If you can't find the CodeSnippets folder, you can create a new CodeSnippets folder yourself. Describe file path ~/Library/MobileDevice/Provisioning Profiles
How does BarButton Item of Navigation Item close to the right or left border of the screen?
Normally, the item on the right keeps a distance from the right side of the screen:
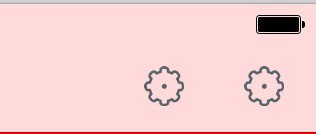
The following problem is solved by adding a fixed spacing item with a negative width, or by changing the width to achieve different spacing:
UIImage *img = [[UIImage imageNamed:@"icon_cog"] imageWithRenderingMode:UIImageRenderingModeAlwaysOriginal];
//System item with fixed spacing and negative width
UIBarButtonItem *rightNegativeSpacer = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemFixedSpace target:nil action:nil];
[rightNegativeSpacer setWidth:-15];
UIBarButtonItem *rightBtnItem1 = [[UIBarButtonItem alloc]initWithImage:img style:UIBarButtonItemStylePlain target:self action:@selector(rightButtonItemClicked:)];
UIBarButtonItem *rightBtnItem2 = [[UIBarButtonItem alloc]initWithImage:img style:UIBarButtonItemStylePlain target:self action:@selector(rightButtonItemClicked:)];
self.navigationItem.rightBarButtonItems = @[rightNegativeSpacer,rightBtnItem1,rightBtnItem2];
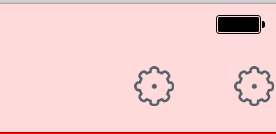