1. privacy settings added in ios10
iOS10 adds a new permission control range. If you try to access these private data, you get the following error:
> This app has crashed because it attempted to access privacy-sensitive > data without a usage description. The app's Info.plist must contain > an NSCameraUsageDescription key with a string value explaining to the > user how the app uses this data
Because it attempts to access sensitive data without information in the application plist Set the privacy key. The newly added privacy setting s are as follows:
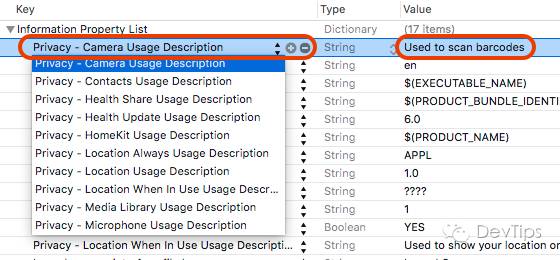
2, OS_ACTIVITY_MODE
Update Xcode 8 if enable appears on the console_ level: 0, persist_ level: 0, default_ ttl: 0, info_ ttl: 0, debug_ ttl: 0, generate_ symptoms: 0 enable_ Oversize: can be set by the following methods:
Edit Scheme-> Run -> Arguments, stay Environment Variables Add inside OS_ACTIVITY_MODE = Disable
3,iOS10 layoutIfNeed
When iOS10 calls layoutIfNeed on a control, it will only calculate the constraint separately, and the constrained control will not take effect. To achieve the previous effect, you need to call layoutIfNeed on the parent control
4, NSDate
Swift3.0 will convert the NSDate of oc to Data type, and some third-party libraries that operate on NSDate will flash back
5, Notification
Swift3. The notification constant of type 0 string is defined as struct
static let MyGreatNotification = Notification.Name("MyGreatNotification")// Use site (no change) NotificationCenter.default().post(name: MyController.MyGreatNotification, object: self)'
6. Zip2sequence (::) is removed
In swift3 0 zip2sequence (::) method is replaced with zip(::)
7, Range<>. Reversed removed
In swift3 0 Range<>. The reversed method is removed and replaced with < Collection > [< range >] indices. reversed().
var array = ["A","B","C","D"]for i in array.indices.reversed() { print("\(i)") }output:3 2 1 0
8. Range is added to four types
Range CountableRange ClosedRange CountableClosedRange
Different expressions generate different ranges
var countableRange = 0..<20 'CountableRange(0..<20)'var countableClosedRange = 0...20 'CountableClosedRange(0...20)'
9. index(:) series methods are added to the collection
The successor(), predecessor(), advancedby (: Limit:), or distanceto (:) methods of Index are removed, and these operations are moved to the Collection
myIndex.successor() => myCollection.index(after: myIndex) myIndex.predecessor() => myCollection.index(before: myIndex) myIndex.advance(by: ...) => myCollection.index(myIndex, offsetBy: ...)
10. Ios10 uistatubar expired
If you need to operate the uistatus bar, you need to change it to
- (UIStatusBarStyle)preferredStatusBarStyle { return UIStatusBarStyleDefault; }
11. Ios10 uicollectionview performance optimization
The biggest change in iOS10 UICollectionView is the addition of pre fetching, If you browse the latest API of UICollectionView, you can find that the following properties have been added:
@property (nonatomic, weak, nullable) id<UICollectionViewDataSourcePrefetching> prefetchDataSource@property (nonatomic, getter=isPrefetchingEnabled) BOOL
In ios10, pre fetching is enabled by default. If you don't want to enable pre fetching for some reasons, you can disable it through the following settings:
collectionView.isPrefetchingEnabled = false
UICollectionViewDataSourcePrefetching protocol is defined as follows:
@protocol UICollectionViewDataSourcePrefetching <NSObject> @required // indexPaths are ordered ascending by geometric distance from the collection view - (void)collectionView:(UICollectionView *)collectionView prefetchItemsAtIndexPaths:(NSArray<NSIndexPath *> *)indexPaths NS_AVAILABLE_IOS(10_0);@optional // indexPaths that previously were considered as candidates for pre-fetching, but were not actually used; may be a subset of the previous call to -collectionView:prefetchItemsAtIndexPaths: - (void)collectionView:(UICollectionView *)collectionView cancelPrefetchingForItemsAtIndexPaths:(NSArray<NSIndexPath *> *)indexPaths NS_AVAILABLE_IOS(10_0);@end
12. Ios10 uitableview performance optimization
Like UICollectionView, UITableView also adds pre fetching technology. UITableView adds the following properties:
@property (nonatomic, weak) id<UITableViewDataSourcePrefetching> prefetchDataSource NS_AVAILABLE_IOS(10_0);
Strangely, the UITableView did not find the definition of the isPrefetchingEnabled property
13. Ios10 uiscrollview added the refreshControl attribute
UIScrollView added refreshControl attribute
@property (nonatomic, strong, nullable) UIRefreshControl *refreshControl NS_AVAILABLE_IOS(10_0) __TVOS_PROHIBITED;
This means that both UICollectionView and UITableView support the refresh function.
We can also use UIRefreshControl without UITableViewController.
14, Swif3.0 new scope access level fileprivate
Currently, there are the following access levels:
- Public
- Internal
- File private
- Private
15,Swift3.0 allows keywords as parameter labels
Swift3. Starting from 0, we will be able to use the keyword except inout var let as the parameter label
// Swift 3 calling with argument label: calculateRevenue(for sales: numberOfCopies, in .dollars) // Swift 3 declaring with argument label: calculateRevenue(for sales: Int, in currency: Currency) func touchesMatching(phase: NSTouchPhase, in view: NSView?) -> Set<NSTouch>
If you insist on using the inout var let keyword, you can use the ` ` package parameter label
func addParameter(name: String, `inout`: Bool)