Use of identifiers
1. Identifier: all that can be named by themselves are called identifiers
For example: class name, variable name, method name, interface name, registration, etc.
2. Naming rules of identifiers (conventional rules):
① It consists of 26 English letters in case, 0 ~ 9_ (underlined) or $.
② Number cannot start
③ Keywords and reserved words cannot be used, but they can be included.
④ Java is strictly case sensitive and has no limit on length.
⑤ Identifiers cannot contain spaces.
3. Naming conventions in Java (common name rules):
Package name: in case of multiple words, all letters are lowercase: xxxyyyzzz z
package javastudypackage //Java studypackage is the package name
Class name and interface name: when composed of multiple words, the first letter of all words is capitalized: xxyyyzz.
public class JavaStudyClass //JavaStudyClass is the class name
Variable name and method name: when composed of multiple words, the first word is lowercase, and the first letter after the second word is capitalized: xxxyyyzz
public void javaStudyMethod(){ } //javaStudyMethod is the method name int javaStudyValue, //javaStudyValue is the variable name
Constant name: all letters are capitalized. When there are multiple words, each word is linked with an underscore XXX_YYY_ZZZ
public static final JAVA_STUDY_CONSTANT //JAVA_STUDY_CONSTANT is a constant name
4. When naming, you should see the meaning of the name.
5
public class Two { public static void main(String []args){ int myNumber = 1001; System.out.println(myNumber); //int student number = 1002; //System. out. Println (student number); The identifier can also be in Chinese } }`
Data types in Java
Classification of data types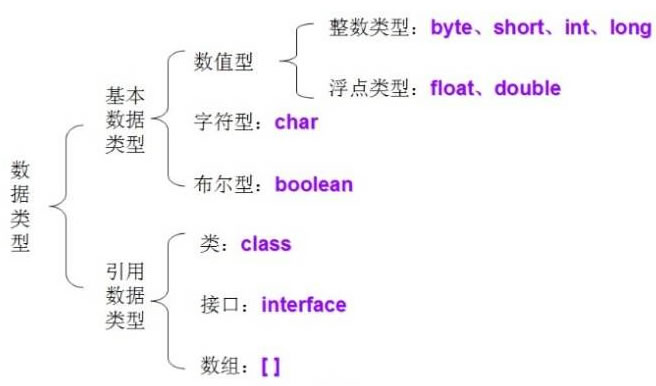
Basic data type
integer
public class value { public static void main(String[] args){ //1 integer: byte (1 byte = 8bit) \ short (2 bytes) \ int (4 bytes) \ long (8 bytes) //byte range: - 128 ~ 127 byte b1 = -128; byte b2 = 127; //byte b3 = 128; This compilation failed System.out.println(b1); System.out.println(b2); //① When declaring a long variable, it must end with 'l' or 'l' //② In general, int is used when defining integer variables. short s1 = 128; int i1 = 12135; long l1 = 4684865489l;//When compiling, "L" or "L" at the end will not compile.
float
public class floatdouble(){ public static void main(String arg[]){ //2 floating point type: float (4 bytes) \ double (8 bytes) //① Floating point type that represents a numeric value with a decimal point //② float indicates that the range of values is larger than long double d1 = 123.3; System.out.println(d1);//Output 123.3 //④ When defining a float class variable, the variable should end with "F" or "F" float f1 = 123.4F; System.out.println(f1);//Output result: 123.4 //⑤ Usually, double is used when defining floating-point variables. } }
Character type (char)
① To define a char type variable, a pair of '' (single quotation marks) is usually used, and only one character can be written inside
char c1 = 'a'; System.out.println(c1); //char c2 = 'ab' //This compilation will not pass because there can only be one character inside char c6 ='\n';// \n is a newline character, which is equivalent to enter char c7 = '\t';// \t is equivalent to Tab and Tab, both of which occupy one character
② Unicode values can be used to represent character variables
char c8 = '\u0043';//In the Unicode table, 0043 = c System.out.println(c8);//c
boolean
① Boolean can only have two values true \ false
② It is often used in condition judgment and loop structure.
boolean bb1 = true; System.out.println(bb1);//true boolean isMarried = true; if(isMarried){ System.out.println("???"); }else{ System.out.println("This is you!"); }//The output result is???
Reference data type
concept
In Java, variables of reference type are very similar to C/C + + pointers. The reference type points to an object, and the variable pointing to the object is the reference variable. These variables are specified as a specific type when declared, such as Employee, purchase, etc. Once a variable is declared, the type cannot be changed.
String is also a reference data type
Write after reference data type. A little big
Use of variables
① java defines the format of variables: data type, variable name = variable value
② Description:
1. Variables must be declared before assignment
2. Variables are defined within their scope (usually a ` ` in braces {}). They are valid within their scope.
3. Two variables with the same name cannot be declared in the same scope
class value { public static void main(String[] args){ int myAge = 18;//Declaration of variables System.out.println(myAge); /*Error case: ①System.out.println(myNumber); Compilation error: myNumber has not been defined before using myNumber ②int myNumber: System.out.println(myNumber); Compilation error: myNumber has not been assigned before using myNumber */ int myNumber = 1220200949; System.out.println(myNumber);//1220200949 } }