1. Common category
1.1Date
Date deals exclusively with dates
This class is about to be discarded. The official manual says that other classes will replace the Calendar class
import java.util.Date; public class Demo1 { public static void main(String[] args) { //1. Instantiate Date object Date date = new Date(); //Tue Aug 17 09:37:55 CST 2021 this time is unfriendly System.out.println(date); Date date1 = new Date(2000, 10,5);//Has been deprecated System.out.println(date1); //Tue Aug 17 09:42:02 CST 2021 //System.currentTimeMillis() timestamp after 1970 Date date2 = new Date(System.currentTimeMillis()); System.out.println(date2); System.out.println(date2.getYear() + 1900); System.out.println(date2.getMonth() + 1);//0 represents January and July represents August } }
1.2Calendar type
A class dedicated to time and date
Calendar is an abstract class and cannot be instantiated directly, but the official manual tells us how to create objects
-
The getInstance method of Calendar returns a Calendar object whose Calendar field has been initialized with the current date and time:
import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; public class Demo5 { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); System.out.println(calendar.get(calendar.YEAR)); System.out.println(calendar.get(calendar.MONTH)+1);//Since the packaging starts from 0, the month needs to be added with 1 System.out.println(calendar.get(calendar.DATE)); System.out.println(calendar.get(calendar.DAY_OF_MONTH)); System.out.println(calendar.get(calendar.DAY_OF_WEEK)-1);//Foreigners regard Sunday as our Monday, so China needs to subtract one System.out.println(calendar.get(calendar.DAY_OF_YEAR)); System.out.println(calendar.get(calendar.HOUR));//Default 12 hour timing System.out.println(calendar.get(calendar.MINUTE)); System.out.println(calendar.get(calendar.SECOND)); Date date = calendar.getTime();//Get time System.out.println(date); //English is very unfriendly and can't understand SimpleDateFormat simpleDateFormat = new SimpleDateFormat("YYYY-MM-dd HH:mm:ss"); System.out.println(simpleDateFormat.format(date)); } }
Common classes are not commonly used, but when learning these things, we should learn ideas. If we give you a class you haven't seen before,
You should consider the following points:
1. What is this class? For example, String processing String Date processing Date time System is related to the System. Special classes do this kind of related things
2. Look at the construction method of this class. Can you new?
3. It is estimated that java under this class should encapsulate many methods. Learn common methods
2. Thread and process [ key ]
Interview must ask!!!
3.1 what is a process
Applications that run independently are called processes
What is an application is that we open various software, such as Google browser, 360, qq music and so on. They are independent software running, called application,
Demo1 and demo2 classes have been written in the code. They can also be called an application or a process
The process needs to obtain the cpu, memory, graphics card, network, etc. in the system through system allocation.
Independence: qq has nothing to do with Google browser. They are independent
Mutex: the operation of our software is to seize the cpu, and whoever steals it will execute first.
Until why sometimes a software card makes you wait. Because the software is preempting the cpu
At the time of, I didn't preempt other software. So either close the software and wait for a response.
3.2 what are threads
A process is composed of multiple threads, and a process must have at least one thread
Thread is the soul of the process, invisible and untouchable. The process can be seen in the execution of this application
But how does memory work? Threads are actually running
A thread is the smallest unit of a process.
If a person is compared to a process, the cell is a thread
What are the characteristics of threads:
1. Preemptive operation
When the cpu executes the application, it executes according to the time slice, and the threads of the unit time slice run preemptively.
There are only three pits in the toilet, but 300 school students seize this pit. Whoever grabs it will use it first. After you release it, others will grab it. The thread is very tired. It is constantly seizing the cpu and releasing.
2. Resource sharing
A thread can share current resources, CPU memory.
The qq process, for example, uses 3MB of running memory. Multiple threads can jointly seize this 3MB of running memory.
Can resources be shared between processes? Can resources be shared between threads?
You cannot share idea and qq resources between processes. These are two processes. Can you share resources? may not
Threads can preempt the same resource in an application
How many threads are there at least?
There are two threads, one is the main function thread, and the other is the JVM garbage collection thread.
3.3 difference between process and thread [interview question]
A process is a complete application, and a process has at least one thread. A process can be regarded as a production workshop. Thread is an executive function in a process, which can be regarded as a production line in a production workshop.
A production workshop is composed of multiple production lines. An application consists of multiple threads.
Process application is a resource of windows system
A thread requests resources for a process
When multiple threads are executing, the cpu will execute randomly in turn according to the allocated time slice of each thread.
The maximum time slice occupied by each thread is about 20ms. After this time slice, it will switch to another thread.
The toilet is compared to an application, a process.
In this process, there are 300 (student) threads. The resources to apply for this toilet are 3MB (3 pits).
Each student seizes the pit and only gives you about 20ms. Ensure that the process is implemented consistently,
If you don't hang up, you can't feel it visually. It's preempting. You feel that our application has been executing all the time.
3.4 concurrency and parallelism
Concurrency: simultaneous, alternating execution.
Parallelism: real simultaneous execution
for instance:
For example, if you order two dishes, you have to finish them in the end,.
From the boss's point of view. It belongs to eating at the same time. But strictly speaking, take turns to eat. It belongs to concurrency.
Parallel: for example, you and your two people at the same table order a dish, you eat yours and I eat mine, parallel
3.5 advantages and disadvantages of threads
advantage:
1. Improve the utilization of resources so that the cpu will not be idle
2. The computer can perform multiple functions
3. Improve user experience
inferiority:
1. Increase the burden of cpu
2. Reducing the CPU's probability of executing other threads will lead to the jamming of our software
3. Sharing resources [key points!!!]
4. Deadlock [avoidance]
3.6 two ways to create threads
Thread
Method 1: no!!!
1. Customize Thread class to inherit Thread class. Override run method
2. Create an instance in the main function and call the start() method to start the thread
class Thread1 extends Thread{ @Override public void run() { System.out.println("I am Thread1 thread "); } } public class MyThread { public static void main(String[] args) { Thread1 thread1 = new Thread1(); thread1.start(); System.out.println("I am main Main thread"); } }
Method 2: [used in general development]
1. Customize a thread class to implement the Runnable interface and rewrite the run method
2. Instantiate the Thread class. The instantiated class object will be passed to the Thread class as a parameter
class MThread implements Runnable{ @Override public void run() { for (int i = 0; i < 100; i++) { System.out.println("I am MThread Thread number:"+i); } } } class MThread1 implements Runnable{ @Override public void run() { for (int i = 0; i < 100; i++) { System.out.println("Others are MThread1 Thread number:"+i); } } } public class Thread2 { public static void main(String[] args) { Thread thread = new Thread(new MThread()); thread.start(); Thread thread1 = new Thread(new MThread1()); thread1.start(); for (int i = 0; i < 100; i++) { System.out.println("I am main Main thread number:"+i); } } }
At the same time, you can also see that the thread is preemptive to obtain resources. When the MThread thread executes on the 31st, it becomes MThread1 thread
3.7 common methods of thread class
Construction method:
Thread();
Created a thread object
Thread(Rnnable target);
Create a thread object and pass in the implementation class of the Runnable interface
Thread(Rnnable target, String name);
Create a thread object, pass in the implementation class of the Runnable interface, and give a name to the current thread
Member method:
static Thread currentThread();
Gets the name of the current thread object
void setName(String name);
Set the name of the thread
String getName();
Gets the name of the thread
void setPriority(int newPriority);
Set the priority of the current thread. The priority can only increase the execution probability of the thread, not necessarily the priority
The default priority of a thread is 5. The value range is 1 ~ 10. 10s is the highest. The lowest priority of 1 only increases the probability of the thread. It is not executed according to the priority. The thread still runs in a preemptive manner
int getPriority();
Gets the priority of the current thread
static void sleep(); Lets the current thread sleep. The number of milliseconds passed in from sleep
class MyThread2 implements Runnable{ @Override public void run() { Thread thread = Thread.currentThread();//Gets the current thread object thread.setName("Zhang San");//Assign a name to the current thread System.out.println(thread.getPriority());//Gets the current thread priority. The default value is 5 thread.setPriority(8);//Set thread priority for (int i = 0; i < 50; i++) { try { Thread.sleep(100);//Set the thread sleep time. Note that using the sleep method in the run method cannot throw exceptions. Only try catch can be used System.out.println(thread.getName()+i);//thread.getName gets the name of the current thread } catch (InterruptedException e) { e.printStackTrace(); } } } } public class Demo1 { public static void main(String[] args) throws InterruptedException { Thread thread = new Thread(new MyThread2()); thread.start(); for (int i = 0; i < 50; i++) { Thread.sleep(100); System.out.println(Thread.currentThread().getName()+i); } } }
3.8 exception of sleep method
class MyThread1 implements Runnable { @Override public void run() { // TODO Auto-generated method stub //The following code has only one try catch and no throws /** * Because of rewriting, strict mode is turned on, * Overriding requires that the methods of the child and parent classes be consistent * In the Runnable interface, there are no throws behind the run method, * So subclasses can't be thrown * * In the future development, we will encounter this situation. We have to figure out why we can't throw it away. We can only try catch * * */ try { Thread.sleep(10000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } public class Demo2 { public static void main(String[] args) { } }
3.9 synchronization code block [difficult]
Used to deal with the problem of thread sharing resources.
Shared machine: ATM machine. When a person is handling business, you go in and lock the door and lock the resource. Others need to queue up
Toilet, three pits. I occupy one pit. After that, I have to queue up in the back. If you don't line up,
Several people have grabbed this resource, which means that several people are using a pit!!! It's a mess!!!!
Is thread unsafe!!!
To see a movie, you need to buy movie tickets:
"Anti triad storm" [ share resources ]
Network: taopiao, meituan, cat's eye
There are three ways to book tickets. We need to sell 100 tickets through three channels,
100 tickets are the shared resources of the three channels. If the three channels are compared to three threads, the three threads will share 100 tickets.
ticket = 100
Taopiao No. 99
Meituan 99
Cat's eye 99
Thread insecurity!!!
Lock!!! Thread synchronization
Question 1:
How to save these 100 tickets and what data type to select?
Data of type int
Member variables? Create three threads. If ticket is a member variable,
Every sales thread has a ticket variable, which is not a shared resource.
Local variable? It is defined in the method body. It is defined in the run method. Every time the run method is run, the ticket must be redefined, and then destroyed. There is no sustainability.
Class variable? Static variables? sure
Static member variables are saved in the data area. It can be provided to the current class object for use, and it can be modified everywhere!!!
class MovieTicket implements Runnable{ private static int ticketNum = 100; @Override public void run() { while (true) { synchronized ("lock") { if (ticketNum > 0) { System.out.println(Thread.currentThread().getName() + ticketNum); ticketNum--; } else { System.out.println("out of stock!"); break; } } } } } public class Demo1 { public static void main(String[] args) { Thread thread1 = new Thread(new MovieTicket(),"Meituan"); Thread thread2 = new Thread(new MovieTicket(),"cat eye"); Thread thread3 = new Thread(new MovieTicket(),"Amoy ticket"); thread1.start(); thread2.start(); thread3.start(); } }
The same movie ticket will be sold without locking, which will lead to thread insecurity.
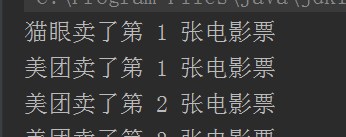
This situation can be solved if the lock is added, because only one thread is executing the code block in the lock after the lock is added, and then the next execution, so there will be no case of selling the same ticket
If the lock is not applied, the resources will not be synchronized and the thread will not be safe.
After adding the lock, the thread unsafe problem will be solved. But your execution efficiency is lower than that without lock
Pursue safety, not efficiency
Like selling train tickets.
You can read this blog and learn more!!!
https://www.cnblogs.com/ysocean/p/6883729.html
Understanding is paramount. The code can't be written, but you must know how the thread goes
3.10 daemon thread
For example:
qq, Netcom these chat software, the main program is called non Guardian thread. All chat window threads are called daemon threads.
If the main program closes, the chat window will close.
If the main thread hangs, the daemon thread also hangs.
jvm, the garbage collection thread is the daemon thread. When an application finishes executing, gc the thread stops
The daemon thread is like a minion. It doesn't matter whether he lives or dies. It depends on the whole main thread. But it plays a great role.
For example, in ancient times, when the emperor died, he would be buried with him. The guardian thread is buried with this
Software update in future development. Daemon threads are required for log updates.
3.11 deadlock
I will ask during the interview!!! Don't write deadlock when developing!!!
Deadlock is the existence of a bug
1. What is deadlock
2. What are the hazards of deadlock
3. The code implements an inevitable deadlock.
3.11 what is deadlock
Keywords: concurrent scenario, multithreading, no compromise
Deadlocks must occur in concurrency scenarios, especially locks. The purpose is to ensure thread safety. Things will turn upside down when they reach the extreme.
Deadlock is a state in which two threads hold each other's resources, but do not actively release the resources in their hands, so that everyone can't use them. The thread cannot continue. It is called deadlock!!!
Write a deadlock [java code]
class DeadLocking implements Runnable { private boolean flag; private Object object1; private Object object2; public DeadLocking(boolean flag, Object object1, Object object2) { this.flag = flag; this.object1 = object1; this.object2 = object2; } @Override public void run() { if (flag) { synchronized (object1) { System.out.println("I am" + Thread.currentThread().getName() + "Acquired lock"); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("I'm going to get Thread-1 Lock of"); synchronized (object2) { System.out.println(Thread.currentThread().getName() + "Got all the locks"); } } } if (!flag) { synchronized (object2) { System.out.println("I am" + Thread.currentThread().getName() + "Acquired lock"); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("I'm going to get Thread-0 Lock of"); synchronized (object1) { System.out.println(Thread.currentThread().getName() + "Got all the locks"); } } } } } public class Demo3 { public static void main(String[] args) { Object object1 = new Object(); Object object2 = new Object(); DeadLocking deadLocking1 = new DeadLocking(true, object1, object2); DeadLocking deadLocking2 = new DeadLocking(false, object1, object2); Thread thread1 = new Thread(deadLocking1); Thread thread2 = new Thread(deadLocking2); thread1.start(); thread2.start(); } }
You can see that the program has been running without stopping
Just look at the above code. Don't imitate it. This situation is prohibited in development. You can't write deadlocks
Thread 1 locks lock1 but wants to apply for lock2. At this time, thread 2 has obtained lock2. Before releasing lock2, thread 2 has to obtain lock1, forming a closed loop and falling into a deadlock cycle
Found the lock company and gave you a box. But you need to prove your identity to unlock. But your identification is in the box. Unlock company: you prove your identity first
You: the identification thing is in the box. I can prove it after you open it
Unlock company: it's stipulated that you must prove your identity first.
Deadlock!!!
3.12 thread life cycle
1. Create thread
2. Thread start
3. Operable state
4. Operation status
5. sleep() deadlock may be blocked in the middle. As long as your thread cannot execute, it is blocked.
6. Thread destruction
The declaration cycle is like a person's life.
Mr. Ren comes out, (people grow up, go to school and don't earn money) can be in running state, (work to earn money) running state
(lost his job and can't make money) blocked, the man hung up. This man's life
3.13 three important methods of thread
Is the following method of Object
wait()
The thread calling this method is in a waiting state [blocking state] and needs to call this method with an object
Moreover, this object must be a lock object. The wait method is generally used with the lock
notify()
To wake up a thread, it needs to be called through an object, and this object is a lock object
Wake up a thread
notifyAll()
Wake up multiple threads
Message class
public class Message { private String message;//Declare a class, and then instantiate the class and treat it as a lock object public Message(){} public Message(String message){ this.message=message; } public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } }
Waitter class and Waitter2 class
import java.text.SimpleDateFormat; public class Waitter implements Runnable{//A thread that is blocked and needs to use noitify to wake up the thread private Message message; public Waitter(Message message) { this.message = message; } @Override public void run() { String name = Thread.currentThread().getName(); synchronized (message){ SimpleDateFormat simpleDateFormat = new SimpleDateFormat("YYYY-MM-dd HH:mm:ss"); System.out.println(name+" The time to obtain the lock is:"+simpleDateFormat.format(System.currentTimeMillis())); System.out.println("====================="); try { message.wait();//When the thread comes to this place, it suddenly blocks. The thread suddenly pauses. //This wait method requires another thread to wake him up. After waking him up, continue to execute the following code } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println(name+"Wake up!!!"+message.getMessage()); } }
import java.text.SimpleDateFormat; public class Waitter2 implements Runnable{ private Message message; public Waitter2(Message message) { this.message = message; } @Override public void run() { String name = Thread.currentThread().getName(); synchronized (message){ SimpleDateFormat simpleDateFormat = new SimpleDateFormat("YYYY-MM-dd HH:mm:ss"); System.out.println(name+" The time to obtain the lock is:"+simpleDateFormat.format(System.currentTimeMillis())); System.out.println("====================="); try { message.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println(name+"Wake up!!!"+message.getMessage()); } }
Notify class
public class Notify implements Runnable{ private Message message; public Notify(Message message) { this.message = message; } @Override public void run() { String name = Thread.currentThread().getName(); try { Thread.sleep(10000);//Let thread 2 sleep for 10s in order to wait for thread 1 to enter the waiting state synchronized (message){ //message.notify(); //Another thread is awakened, which is random message.notifyAll(); //message.notifyAll(); The method is to wake up all wait threads System.out.println(name+" Wake up a wave directly!"); System.out.println("===================="); } } catch (InterruptedException e) { e.printStackTrace(); } } }
Client class
public class Client { public static void main(String[] args) { Message message = new Message("I'm your father"); Waitter waitter = new Waitter(message); Waitter2 waitter2 = new Waitter2(message); Notify notify = new Notify(message); Thread thread1 = new Thread(waitter,"Thread 1"); Thread thread2 = new Thread(waitter2,"Thread 2"); Thread thread3 = new Thread(notify,"Thread 3"); thread1.start(); thread2.start(); System.out.println("===================="); thread3.start(); /** * * be just like: * You are a waiting thread: write a wait method * Originally, classes were finished online. When the epidemic broke out, there would be congestion. After receiving the government's notice, you can't go out * The government is a wake-up thread: write the notify method * Say that the epidemic is over, the community is unsealed, and I can go out only after I give you a notice * * Summary: at least two threads: one waiting thread (wait) * The other must be wake-up thread notify() * These two methods must be used together, otherwise the code you write is meaningless and the code will not be executed * * */ } }
You can also browse this blog for details