form file upload - front end, background and real-time preview
If you want to support file upload, you must set two properties:
-
method="post"
-
enctype="multipart/form-data"
Once this item is set in the form, the Java background cannot pass request Getparameter get parameter
Commonly used in Java background commons-fileupload To receive files uploaded by the client
- Parse request as list < filteitem >:
ServletFileUpload upload = new ServletFileUpload(new DiskFileItemFactory()); List<FileItem> items = upload.parseRequest(request); for (FilteItem item : items) { if (item.isFormField()) { // Non file } else { // file } }
- Solve the problem of garbled Chinese file names
upload.setHeaderEncoding("UTF-8");
The pictures just uploaded may not be previewed in real time (it will take a while to preview successfully)
To realize real-time preview, just turn off Tomcat's cache resource function and click% Tomcat_ HOME%/conf/context. Add Resources tag in XML:
<?xml version="1.0" encoding="UTF-8"?> <Context> <WatchedResource>WEB-INF/web.xml</WatchedResource> <WatchedResource>WEB-INF/tomcat-web.xml</WatchedResource> <WatchedResource>${catalina.base}/conf/web.xml</WatchedResource> <Resources cachingAllowed="false" cacheMaxSize="0" /> </Context>
Normally, you should not be able to directly access XX JSP page, because it is a static page, you must forward access through Servlet to access the page with data.
To prevent The jsp file is accessed and can be placed in the WEB-INF folder
Static resources (. css, img, etc.) in web pages can generally be accessed directly, so they can not be put into WEB-INF
-
Servlet: controller layer
-
Service: business layer, used to complete specific business
-
Dao: data access layer
The reason for the existence of the Service layer is that sometimes a business may call database related operations many times. When the project is very simple (what the Service layer does is directly call the Dao layer), the significance of the existence of the Service is not obvious, but when the project becomes larger, some business operations handled by the Service need to be extracted more clearly.
Interface oriented programming
Why interface oriented programming?
- The interface only defines a specification. For example, the Dao interface specifies what operations to access the database and how to implement these operations. For example, Hibernate, Mybatis, spring JDBC, etc. if there are no interface constraints, a lot of code needs to be changed after switching the implementation scheme of the database; However, if there are interface constraints, you only need to implement the specific operations of each scheme on the database.
=======================================================================
Using Jackson to convert Java objects into Json strings
Person person = new Person(1, "Zhang San", 12); ObjectMapper mapper = new ObjectMapper(); String jsonString = mapper.writeValueAsString(person); // {"id":"1", "name": "Zhang San", "age":"12"}
Get the type of a generic using reflection
The usage scenario is to automatically generate the table name, obtain the class name according to the generic type, and then convert it to the table name of the database
public class Student extends Person<String, Integer> implements Test1<Integer, Double>, Test2<Double, Long, StringBuilder> { // Gets the type of the generic public void printGenericType() { // Parent class ParameterizedType superClassType = (ParameterizedType) getClass().getGenericSuperclass(); Type[] args = superClassType.getActualTypeArguments(); for (Type arg : args) { System.out.println(arg); } System.out.println("-----------------------------"); // Interface Type[] interfaceTypes = (Type[]) getClass().getGenericInterfaces(); for (Type type : interfaceTypes) { ParameterizedType interfaceType = (ParameterizedType) type; Type[] interfaceArgs = interfaceType.getActualTypeArguments(); for (Type arg : interfaceArgs) { System.out.println(arg); } } } }
java.lang.String
java.lang.Integer
java.lang.Integer
java.lang.Double
java.lang.Double
java.lang.Long
java.lang.StringBuilder
**Big hump (MyAge)**,**Small hump (myAge)** Turn into **Underline(my\_age)**:
/**
- Change myage and myage to my_age
**/
public static String underlineCased(String str) {
if (str == null) return null; int len = str.length(); if (len == 0) return str; StringBuilder sb = new StringBuilder(); sb.append(Character.toLowerCase(str.charAt(0))); for (int i = 1; i < len; i++) { char c = str.charAt(i); if (Character.isUpperCase(c)) { sb.append("_"); sb.append(Character.toLowerCase(c)); } else { sb.append(c); } } return sb.toString();
}
[](https://codechina.csdn.net/m0_60958482/java-p7) front end skills ======================================================================= [](https://codechina.csdn.net/m0_60958482/java-p7) use reset to empty the form -------------------------------------------------------------------------------------- jQuery What you get is not native DOM Object, by jQuery Cannot call after getting the form reset,Need to be converted to native DOM:
document.querySelector(’#add-form-box form’). reset() / / the native form DOM object has a reset method
// $('#add-form-box form').reset() / / an error is reported. jQuery does not get the native DOM, and there is no reset method $('#add-form-box form')[0].reset() ``` Note: call reset Method does not reset `type="hidden"` of input Input box, need to use js individualization [](https://codechina.csdn.net/m0_60958482/java-p7) MIMEType of picture ------------------------------------------------------------------------------- Select the of the file input Can pass accept To control the type of file received,`image/*` All picture types ``` <input type="file" name="image" accept="image/*" /> ``` [](https://codechina.csdn.net/m0_60958482/java-p7) the verification code function sends different parameters to prevent caching ----------------------------------------------------------------------------------- Clicking the verification code should refresh the picture, but if the address of each request is the same, the browser will get it from the cache without refreshing. By splicing some different parameters later, the requests sent each time are different. ``` $("#captcha").click(function() { $(this).attr('src', '${ctx}/user/catcha?time=' + new Date().getTime()) }) ``` [](https://codechina.csdn.net/m0_60958482/java-p7) login / password modification function, and use the hidden domain to send the encrypted password ------------------------------------------------------------------------------------------ # More: Java advanced core knowledge set contain: JVM,JAVA Collection, network, JAVA Multithreading concurrency, JAVA Basics, Spring Principles, microservices, Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB,Cassandra,Design pattern, load balancing, database, consistency hash, JAVA Algorithm, data structure, encryption algorithm, distributed cache, etc 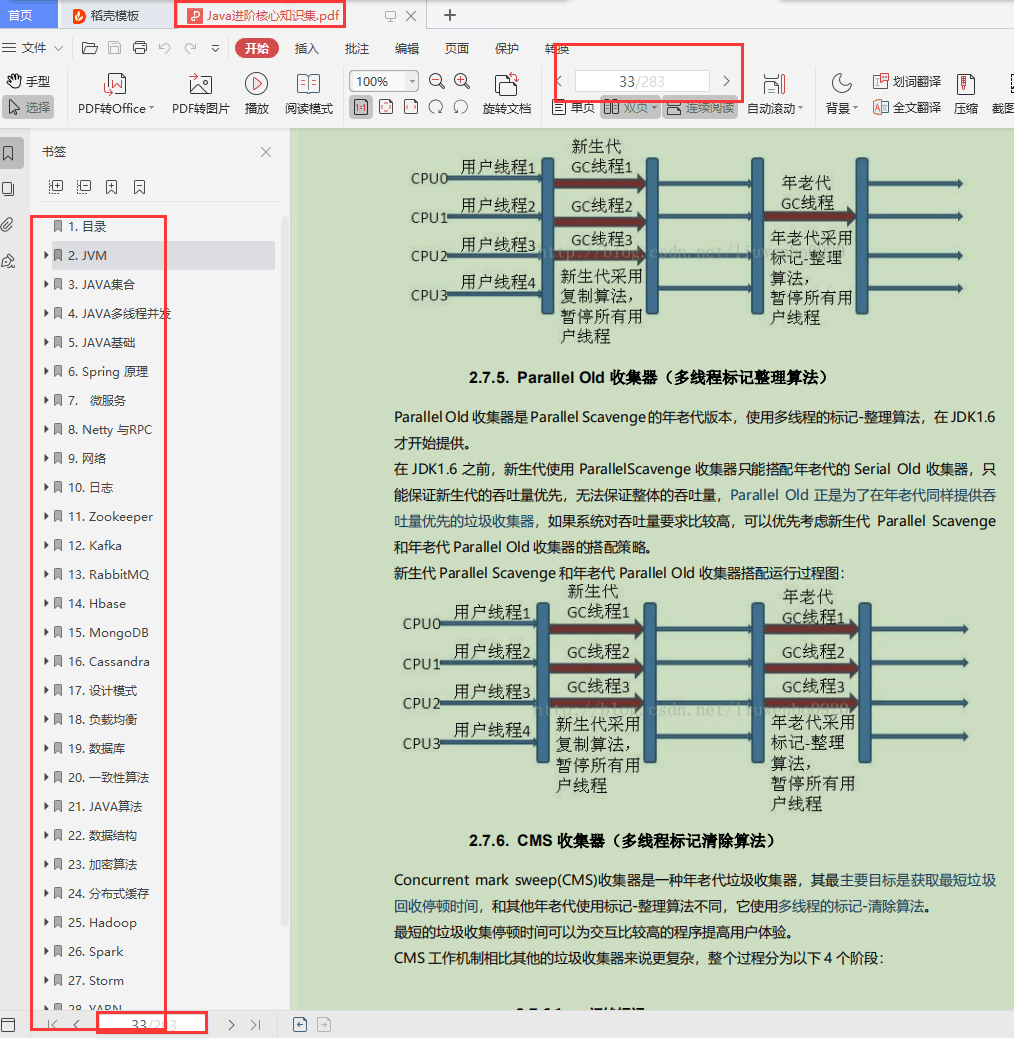 > **[Click to get my study notes for free: study video+Real interview questions for large factories+Microservices+MySQL+Java+Redis+algorithm+network+Linux+Spring Family bucket+JVM+Study note map](https://codechina.csdn.net/m0_60958482/java-p7)** # Efficient learning video ------------------------------------------ # More: Java advanced core knowledge set contain: JVM,JAVA Collection, network, JAVA Multithreading concurrency, JAVA Basics, Spring Principles, microservices, Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB,Cassandra,Design pattern, load balancing, database, consistency hash, JAVA Algorithm, data structure, encryption algorithm, distributed cache, etc [External chain picture transfer...(img-RZuJcIA9-1629250120469)] > **[Click to get my study notes for free: study video+Real interview questions for large factories+Microservices+MySQL+Java+Redis+algorithm+network+Linux+Spring Family bucket+JVM+Study note map](https://codechina.csdn.net/m0_60958482/java-p7)** # Efficient learning video 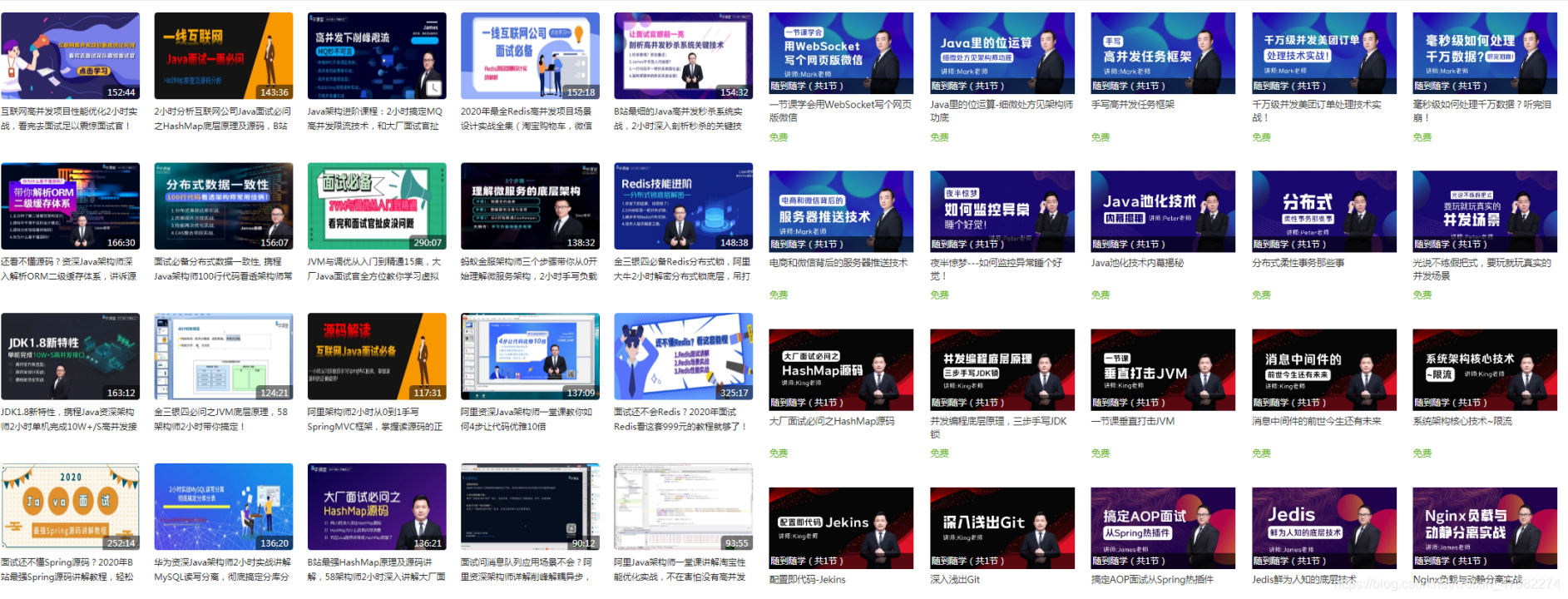