Be careful! If you choose a non-default engine, you need to set up a template engine in AutoGenerator.
AutoGenerator generator = new AutoGenerator(); // set freemarker engine generator.setTemplateEngine(new FreemarkerTemplateEngine()); // set beetl engine generator.setTemplateEngine(new BeetlTemplateEngine()); // set custom engine (reference class is your custom engine class) generator.setTemplateEngine(new CustomTemplateEngine()); // other config
Write Configuration
- Configure GlobalConfig
GlobalConfig globalConfig = new GlobalConfig(); globalConfig.setOutputDir(System.getProperty("user.dir") + "/src/main/java"); globalConfig.setAuthor("jobob"); globalConfig.setOpen(false);
- Configure DataSourceConfig
DataSourceConfig dataSourceConfig = new DataSourceConfig(); dataSourceConfig.setUrl("jdbc:mysql://localhost:3306/ant?useUnicode=true&useSSL=false&characterEncoding=utf8"); dataSourceConfig.setDriverName("com.mysql.jdbc.Driver"); dataSourceConfig.setUsername("root"); dataSourceConfig.setPassword("password");
Custom Template Engine
Please inherit class com.baomidou.mybatisplus.generator.engine.AbstractTemplateEngine
Custom Code Template
//Specify custom template path, location: /resources/templates/entity2. Java. FTL (or.vm) //Be careful not to bring it with you. FTL (or.vm), which is automatically identified by the template engine used TemplateConfig templateConfig = new TemplateConfig() .setEntity("templates/entity2.java"); AutoGenerator mpg = new AutoGenerator(); //Configure Custom Templates mpg.setTemplate(templateConfig);
Custom Property Injection
InjectionConfig injectionConfig = new InjectionConfig() { //Custom attribute injection: abc //In. Get properties from ${cfg.abc} in a FTL (or.vm) template @Override public void initMap() { Map<String, Object> map = new HashMap<>(); map.put("abc", this.getConfig().getGlobalConfig().getAuthor() + "-mp"); this.setMap(map); } }; AutoGenerator mpg = new AutoGenerator(); //Configure custom property injection mpg.setCfg(injectionConfig);
entity2.java.ftl
Custom attribute injection abc=${cfg.abc}
entity2.java.vm
Custom attribute injection abc=$!{cfg.abc}
Field Other Information Query Injection ----------- 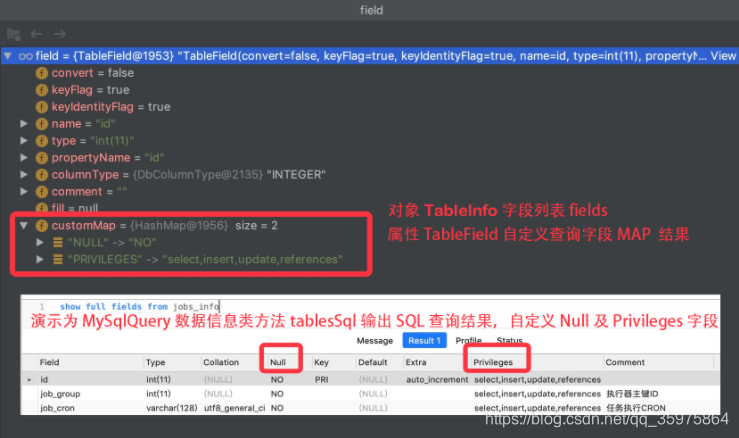
new DataSourceConfig().setDbQuery(new MySqlQuery() {
/** * Override parent reserved query custom field <br> * The SQL queried here corresponds to the query field of the parent tableFieldsSql, which cannot be met by default. Please rewrite it <br> * Called in the template: table.fields gets all the field information, * Then loop the fields to get the fields. CusmMap gets the injection fields from the MAP as NULL or PRIVILEGES */ @Override public String[] fieldCustom() { return new String[]{"NULL", "PRIVILEGES"}; }
})
mybaits Common Notes =========== 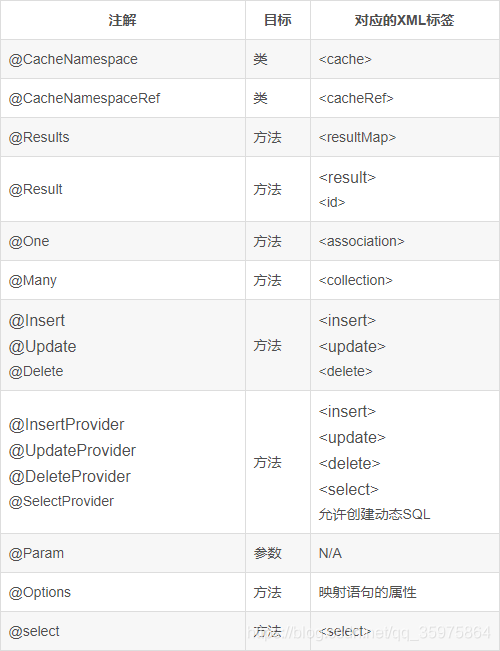 ==================================================================================================================================================================================================== Write a simple login registration function ============== First set up the database, create User surface 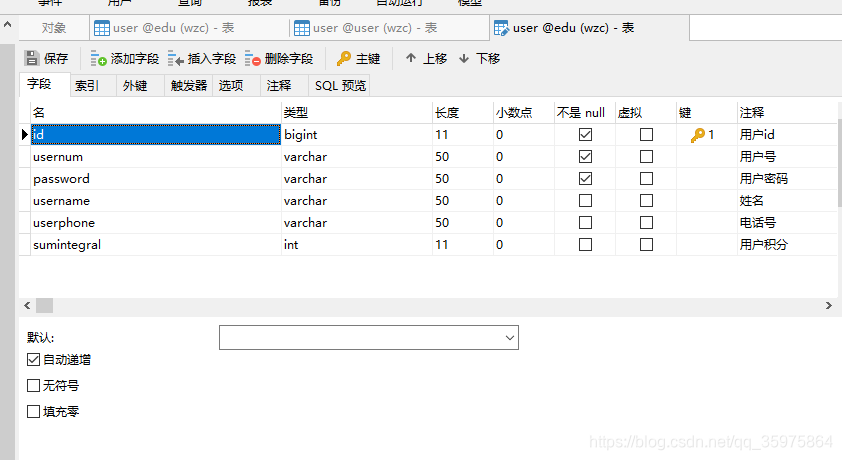 again idea Create frame in 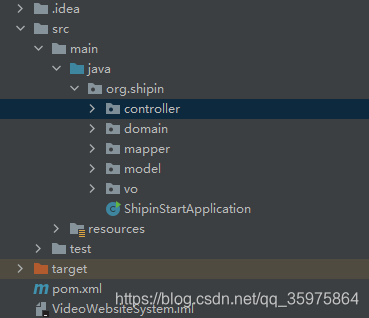 model In User.java
package org.shipin.model;
import lombok.Data;
@Data
public class User {
private Integer id; private String usernum; private String password; private String username; private String userphone;
}
mappaer In UserMapper.java This is the interface
package org.shipin.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
import org.shipin.model.User;
@Mapper
public interface UserMapper extends BaseMapper {
/** * Query users by phone * @param phone * @return */ @Select("select * from `user` where `usernum` = #{usernum}") User selectUserByUsernum(String phone);
}
controller In UserController.java
package org.shipin.controller;
import cn.hutool.crypto.SecureUtil;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import io.swagger.annotations.ApiOperation;
import org.shipin.domain.ResponseMessage;
import org.shipin.mapper.UserMapper;
import org.shipin.model.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
@RequestMapping("/user")
public class UserController {
@Autowired private UserMapper userMapper; @ApiOperation("User Login") @PostMapping("/login") @ResponseBody public Object login(String usernum,String password){ QueryWrapper<User> queryWrapper = new QueryWrapper<>(); queryWrapper.eq("usernum",usernum).eq("password",SecureUtil.md5(password)); User user = userMapper.selectOne(queryWrapper); if(user == null){
//Failure
return ResponseMessage.error("Logon Failure"); }else {
//into
return ResponseMessage.success(1); } } @ApiOperation("Registered User") @PostMapping("/register") @ResponseBody public ResponseMessage register(String usernum,String password,String username,String userphone){ if(usernum == null || usernum.length() < 6){
Write at the end
As an upcoming programmer, where will your job opportunities and outlets appear in 2019, which may be very different from recent years? In this new environment, should I work in a big or small company? How should veterans who have worked for several years maintain and improve their competitiveness and turn passive into active?
In the current environment, job-hopping is much more difficult to succeed than in previous years. One obvious feeling is that this year's interviews, both on one side and on the other, test the technical skills of Java programmers.
Recently, I organized a review interview and high frequency interview questions and technical points into a "Java Classic Interview Questions (with Answer Resolution). PDF and a"Java Programmer Interview Written Test Gallery.pdf"collected online. (Actually a lot more effort than expected) Including advanced dry goods for distributed architecture, high scalability, high performance, high concurrency, Jvm performance tuning, Spring, MyBatis, Nginx source analysis, Redis, ActiveMQ, Mycat, Netty, Kafka, Mysql, Zookeeper, Tomcat, Docker, Dubbo, Nginx, etc.
Due to the limited space, in order to facilitate your viewing, here is a picture showing you part of the catalog and answer screenshots! Friends in need can stamp here for free
Java Classic Interview Questions with Answer Resolution
Alibaba Technical Writing Experience
Sequential Interview Written Test Exercise Gallery. pdf** (which actually takes a lot more effort than expected) includes advanced dry goods with distributed architecture, high scalability, high performance, high concurrency, Jvm performance tuning, Spring, MyBatis, Nginx source analysis, Redis, ActiveMQ, Mycat, Netty, Kafka, Mysql, Zookeeper, Tomcat, Docker, Dubbo, Nginx and many other knowledge points!
Due to the limited space, in order to facilitate your viewing, here is a picture showing you part of the catalog and answer screenshots! Friends in need can stamp here for free
[img-FsVqLGDG-1628077748570)]
Java Classic Interview Questions with Answer Resolution
[img-xgSXj0hl-1628077748573)]
Alibaba Technical Writing Experience
[img-XVocXQtE-1628077748574)]