java implements the function of sending mail
java implements the function of sending mail
E-mail development is a common phenomenon and function in the background, such as user registration, the system automatically sends an e-mail to the user's mailbox; and then, for example, password retrieval, the system will automatically send the password to the user's mailbox;... Wait a minute, so as a java programmer, it's still necessary to learn this skill.
I am an Android developer. We all know that Http protocol is used when client and background interact with data. Correspondingly, mailbox transmission also has its own protocol, such as SMTP, POP3, IMAP. In native java JDK, the official encapsulated the Http protocol for us, called URLConnection. Natural official also encapsulated the exclusive protocol for mail. However, we can't find this in native java JDK, because it requires us to download it separately.
Currently, the latest version of JavaMail is 1.1.7. We download it as follows:
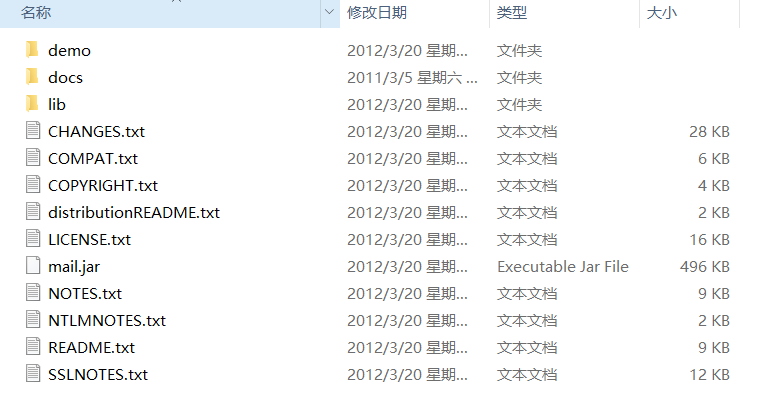
mail.jar is what we need, as well as documentation and official demo, so we don't know much about it here, but we'll just start this demo.
Here I use JUnit Test directly in existing projects, you can create new common java project exercises.
import java.util.Date;
import java.util.Properties;
import javax.mail.Message.RecipientType;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import org.junit.Test;
public class EmailTest {
public static final String SMTPSERVER = "smtp.163.com";
public static final String SMTPPORT = "465";
public static final String ACCOUT = "******@163.com";
public static final String PWD = "*******";
@Test
public void testSendEmail() throws Exception {
// Create mail configuration
Properties props = new Properties();
props.setProperty("mail.transport.protocol", "smtp"); // Protocol used (JavaMail specification requirements)
props.setProperty("mail.smtp.host", SMTPSERVER); // SMTP server address of sender's mailbox
props.setProperty("mail.smtp.port", SMTPPORT);
props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
props.setProperty("mail.smtp.auth", "true"); // Request authentication
props.setProperty("mail.smtp.ssl.enable", "true");// Open ssl
// Create sessions according to mail configuration, and pay attention to session-specific error-leading packages
Session session = Session.getDefaultInstance(props);
// Open debug mode to see more detailed input logs
session.setDebug(true);
//Create mail
MimeMessage message = createEmail(session);
//Get the transmission channel
Transport transport = session.getTransport();
transport.connect(SMTPSERVER,ACCOUT, PWD);
//Connect and send mail
transport.sendMessage(message, message.getAllRecipients());
transport.close();
}
public MimeMessage createEmail(Session session) throws Exception {
// Create mail based on session
MimeMessage msg = new MimeMessage(session);
// Addresses email address, personal email nickname, charset encoding
InternetAddress fromAddress = new InternetAddress(ACCOUT,
"kimi", "utf-8");
// Setting up the sender
msg.setFrom(fromAddress);
InternetAddress receiveAddress = new InternetAddress(
"********@qq.com", "test", "utf-8");
// Setting up Mail Receiver
msg.setRecipient(RecipientType.TO, receiveAddress);
// Setting Mail Title
msg.setSubject("Test title", "utf-8");
msg.setText("I'm a programmer. One day I sat on the roadside drinking water and checking the program painstakingly. Then a beggar sat down beside me and began to ask for food. I felt pity and gave him a dollar. Then debug the program. Maybe he's not doing well. He just bores himself to see what I'm doing. After a while, he slowly points to my screen and says, "There's a semicolon missing here."");
// Set the dispatch time for display
msg.setSentDate(new Date());
// Save Settings
msg.saveChanges();
return msg;
}
}
The amount of code is not very large, and then JUnit Test Run:

I did receive the email. Of course, there are still some words in it. Here, according to the mistakes I have encountered, let me briefly say:
Errors such as: DEBUG SMTP: Attempt to authenticate using mechanisms: LOGIN PLAIN DIGEST-MD5 NTLM XOAUTH2
Login failed for many reasons. First of all, we need to ensure that the sender's mail, POP3/SMTP service and IMAP/SMTP service must be opened, otherwise the sending fails. Take Netease mailbox as an example, open this service in the following places:
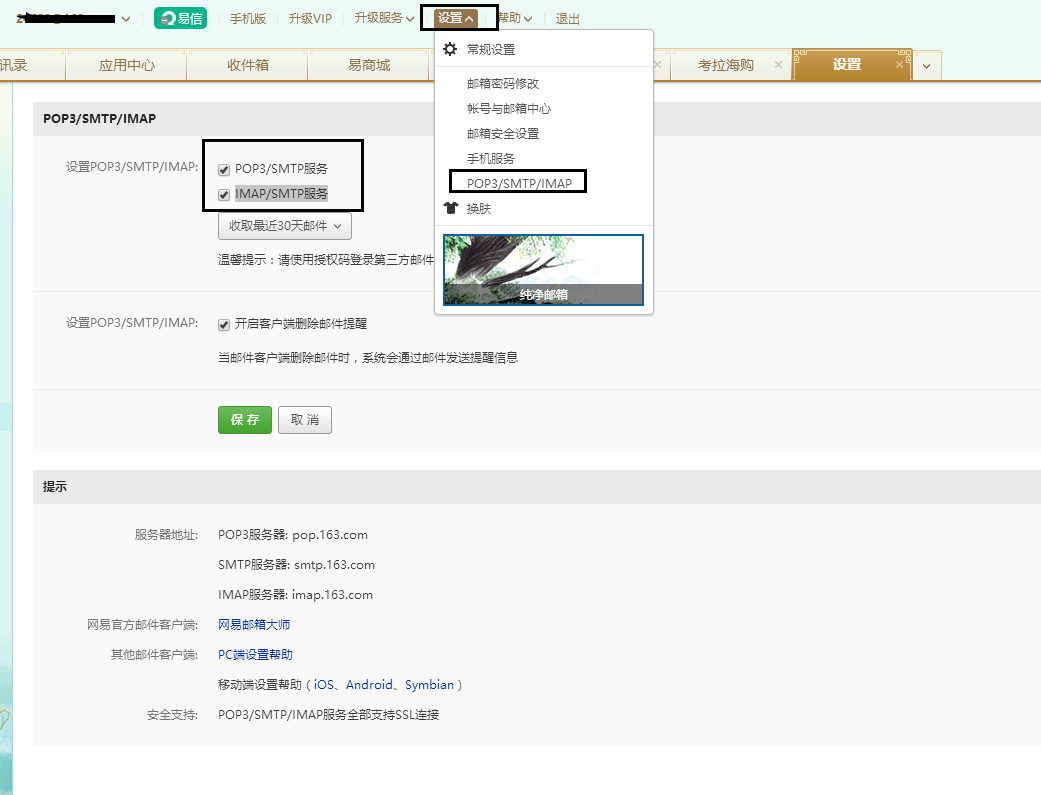
If the above confirmation is opened, then we need to confirm whether the password is correct, because my 163 mailbox has opened the client authorization password, so here we must pay attention to the need to fill in the client authorization password, otherwise there will be such an error.
The problem that I encounter is one of the above. If you have any questions or opinions, you are welcome to communicate at any time.
Keywords:
Session
Java
Junit
SSL
Added by hewzett on Mon, 17 Jun 2019 02:50:06 +0300