Day 1
This section mainly explains some compiling environments of JAVA and some confusing basic knowledge.
James Gosling received a bachelor's degree in computer science from the University of Calgary in Canada in 1977 and a doctor's degree in computer science from Carnegie Mellon University in 1983. After graduation, he worked at IBM and designed the first generation of IBM workstation NeWS system, but he was not valued. Later, he transferred to Sun company. In 1990, he cooperated with Patrick, Naughton and Mike Sheridan in the "Green Plan". Later, he developed a language called "Oak" and later changed its name to Java.
When we see Java, we will find that Java is divided into three versions: JavaSE, javame and JavaEE. Their relationship is respectively.
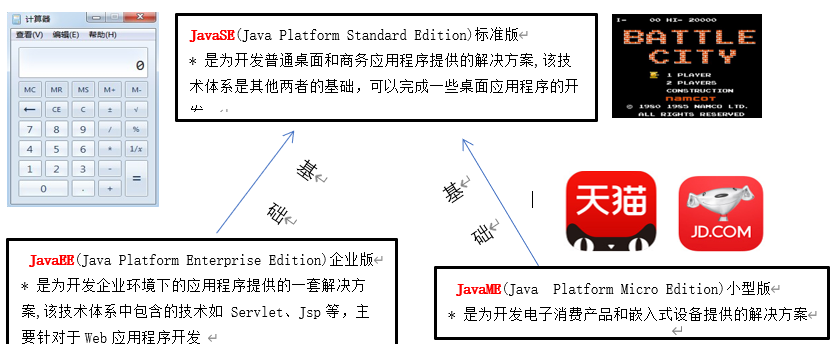
JVM JRE JDK
- What is a JVM
JVM is a java virtual machine. java programs need to run on the virtual machine. Different platforms have their own virtual machines, so java language can cross platforms - What is JRE
Including the Java virtual machine (JVM) and the core class libraries required by Java programs. If you want to run a developed Java program, you only need to install JRE in the computer.
JRE:JVM + class library. - What is JDK
JDK is provided for Java developers, including java development tools and JRE. Therefore, if you install JDK, you don't need to install JRE separately.
The development tools include compiling tool (javac.exe), packaging tool (jar.exe), etc
JDK: the development tool of JRE + Java. - Why does the JDK include a JRE
The developed program needs to be run to see the effect. - Role and relationship of JDK, JRE and JVM
JDK includes JRE and development kit
JRE contains core class libraries and JVM s
The ide of this series is IntelliJ IDEA 2019.1 x64. After downloading the IDE, the first problem encountered is "Project SDK is not defined".
First, read the error reason. The error message shows that the project SDK is not defined and prompts me to install the SDK. Some novice partners are a little confused at this time. They only know that jdk is a java development kit. What is the SDK? I have never used this thing. How can I be prompted to install it at this time. Don't worry at this time. In fact, SDK refers to the Software Development Kit (abbreviation: SDK, full foreign language name: Software Development Kit). It is generally a collection of development tools used by some software engineers to build application software for specific software packages, software frameworks, hardware platforms, operating systems, etc. here SDK refers to our jdk, In other words, the project cannot find the dependent jdk
There are two steps to solve such problems.
Step 1:
Select Project Structure
Click New in Project to connect the JDK installed on the computer. In this step, select the directory as the installation directory of JDK instead of pointing to the bin subdirectory.
1. Java variables
1.1 variable overview
In the process of program execution, the amount whose value can change within a certain range
Essentially, a variable is a small area of memory
1.2 variable definition format
Data type variable name = initialization value;
The variable diagram can be as follows:
1.3 data types of Java
Java language is a strongly typed language. It defines specific data types for each kind of data and allocates different sizes of memory space in memory. Its data types include:
Note:
Integers are of type int by default. When defining long type data, L should be added after the data.
Floating point numbers are double by default. When defining data of float type, F should be added after the data.
public class VariableDemo { public static void main(String[] args) { //Variable of type byte byte b = 10; System.out.println(10); System.out.println(b); //Variable of type short short s = 100; System.out.println(s); //Variable of type int int i = 1000; System.out.println(i); //Variable of type long //long l = 10000; //System.out.println(l); long l = 10000000000L; System.out.println(l); //Variable of type float float f = 12.34F; System.out.println(f); //Variable of type double double d = 12.34; System.out.println(d); //Variable of type char char ch = 'a'; System.out.println(ch); //Variables of type boolean boolean bb = true; System.out.println(bb); } } //Define variables of type boolean boolean bb = false; System.out.println(bb); } }
1.4 precautions for variable definition
• variables are not assigned and cannot be used directly
• a variable is valid only within its range.
The variable belongs to the pair of braces in which the variable is in
• multiple variables can be defined on one line, but it is not recommended
package com.itheima; /* * Precautions for variables: * A:The variable is not assigned and cannot be used directly * B:A variable is only valid in the range it belongs to * The variable belongs to the pair of braces in which it is located * C:Multiple variables can be defined on one line, but it is not recommended */ public class VariableDemo2 { public static void main(String[] args) { //Define a variable int a = 10; System.out.println(a); int b; b = 20; System.out.println(b); { //Code block int c = 30; System.out.println(c); } //System.out.println(c); System.out.println(b); /* int aa,bb; aa = 10; bb = 20; System.out.println(aa); System.out.println(bb); */ int aa = 10; int bb = 20; System.out.println(aa); System.out.println(bb); } }
1.5 data type conversion
1.5.1 implicit data type conversion
When a data type with a small value range is operated with a data type with a large value range, the small data type will be promoted to a large one before the operation:
//Define two variables of type int int a = 10; int b = 20; System.out.println(a+b); //I can output the result of a+b, which shows that there is no problem with the result of this calculation //Well, I should also be able to receive this result. int c = a + b; System.out.println(c); System.out.println("-----------------"); //Define two variables, an int type and a byte type int aa = 10; byte bb = 20; System.out.println(aa+bb); //The following way of writing is wrong //byte cc = aa+bb; int cc = aa + bb; System.out.println(cc);
1.5.2 forced type data conversion
Cast format
Target type variable name = (target type) (converted data);
Considerations for casting
If it exceeds the value range of the assigned data type, the result will be different from what you expect
package com.itheima; /* * Cast: * Target type variable name = (target type) (converted data); * * Although forced conversion can be done, it is not recommended. Data may be lost due to forced conversion. */ public class ConversionDemo2 { public static void main(String[] args) { //Define two variables, an int type and a byte type int a = 10; byte b = 20; int c = a + b; System.out.println(c); byte d = 30; byte e = (byte)(a + b); System.out.println(e); } }
2. Operators in Java
/: division operator. The result is the quotient of two divided data.
In Java, there is no ("/ /, division" and "/, division")
Integer division can only get an integer. To get a decimal, you must use floating-point numbers to participate in the operation.
When characters and strings participate in addition operation
Character participation in operation
In fact, it is operated with the value corresponding to the character
'a' 97
'A' 65
'0' 48
String participation in operation
In fact, what we do here is not addition, but string splicing.
String and other types of data are spliced, and the result is string type.
package com.itheima_01; /* * Character participation in addition operation: it is actually calculated by taking the data value represented by the character stored in the computer. * 'a' 97 * 'A' 65 * '0' 48 * * Strings participate in addition operation: in fact, this is not addition, but the splicing of strings. */ public class OperatorDemo2 { public static void main(String[] args) { //Define two variables, an int type and a char type int a = 10; char ch = 'a'; System.out.println(a + ch); System.out.println("----------------"); //String addition System.out.println("hello"+"world"); System.out.println("hello"+10); System.out.println("hello"+10+20); System.out.println(10+20+"hello"); } }
Overview and usage of self increasing and self decreasing operators
++, – operator: add 1 or subtract 1 to a variable.
++Or – it can be placed either after the variable or in front of the variable.
When used alone, + + or - whether placed before or after variables, the result is the same.
When participating in the operation:
If + + or - is behind the variable, take the variable to participate in the operation first, and then make the variable + + or –
If + + or – is in front of the variable, first make + + or –, and then take the variable to participate in the operation
package com.company; public class yuner { public static void main(String[] args) { //Define two variables of type int int a = 10; int b = 20; int c = ++a; System.out.println(a++); System.out.println(++a); System.out.println(c); System.out.println(a++); System.out.println(a++); System.out.println("a:"+a); System.out.println("b:"+b); } }
Carefully consider the above code.
Overview and use of ternary operators
(relational expression)? Expression 1: expression 2;
If the condition is true, the result of the operation is expression 1;
If the condition is false, the result of the operation is expression 2;
package com.itheima_06; /* * Ternary operator: * Relational expression? Expression 1: expression 2; * * Execution process: * A:Calculate the value of the relationship expression to see whether the result is true or false * B:If true, expression 1 is the result * If false, expression 2 is the result */ public class OperatorDemo { public static void main(String[] args) { //Define two variables int a = 10; int b = 20; int c = (a>b)?a:b; System.out.println("c:"+c); } }
3. Keyboard entry
At present, when we write the program, the data value is fixed, but in the actual development, the data value must change. Therefore, we should improve the data into keyboard entry to improve the flexibility of the program.
Steps for keyboard data entry:
A: Guide Package (position above class definition)
import java.util.Scanner;
B: Create object
Scanner sc = new Scanner(System.in);
C: Receive data
int x = sc.nextInt();
package com.itheima; import java.util.Scanner; /* * In order to improve the flexibility of the program, we improve the data to keyboard entry. * How to realize keyboard input data? Currently, the class Scanner provided by JDK is used. * How to use Scanner to get data? At present, you can remember the use steps. * * Use steps: * A:Guide Package * import java.util.Scanner; * Note: in a class, there is such an order relationship * package > import > class * B:Create keyboard entry object * Scanner sc = new Scanner(System.in); * C:get data * int i = sc.nextInt(); */ public class ScannerDemo { public static void main(String[] args) { //Create keyboard entry object Scanner sc = new Scanner(System.in); //Give tips System.out.println("Please enter an integer:"); //get data int i = sc.nextInt(); //Output the acquired data System.out.println("i:"+i); } }
Enter two data on the keyboard, sum the two data and output the results
Keyboard entry:
- Guide Package
- create object
- receive data
package com.itheima; import java.util.Scanner; /* * Requirement: input two data with keyboard, sum the two data and output the result */ public class ScannerTest { public static void main(String[] args) { //Create keyboard entry object Scanner sc = new Scanner(System.in); //Give tips System.out.println("Please enter the first integer:"); int a = sc.nextInt(); System.out.println("Please enter the second integer:"); int b = sc.nextInt(); //Sum data int sum = a + b; //Output results System.out.println("sum:"+sum); } }