Java Lab 8, Exceptions
I. Experimental purposes
1. Master the compilation of custom exception classes;
2. Master using try-catch statements to handle exceptions.
II. Contents and requirements of the experiment
The station inspects the equipment of dangerous goods and warns if dangerous goods are found. Programming simulation equipment to detect dangerous goods:
1. Write a subclass DangerException of Exception, which can create an exception object, which calls showMessage() method to output "belongs to dangerous goods".
2. Write a Goods class, including the name of luggage and whether it is the property of dangerous goods and the corresponding settings and acquisition methods.
3. Write a Machine class in which the checkGoods method will throw DangerException exception when the baggage is found to be dangerous.
4. Define n pieces of luggage in the main () method of the main class, randomly generate whether they are dangerous goods or not, and use try-catch statement to make the instance of Machine class call checkGoods method for exception handling.
III. Experimental process and results
①DangerException.java
public class DangerException extends Exception{
String message;//String variable, dangerous goods
public DangerException() {
message = "It belongs to dangerous goods.";
}//When the attribute is dangerous goods, the variable value is set as "belongs to dangerous goods".
String showMessage(){
return message;
}//Return display information
}
②Goods.java
public class Goods {
String luggageName;//Luggage name
boolean isDanger;//Luggage attributes. true: dangerous; false: not dangerous
void setLuggageName(String luggageName){
this.luggageName = luggageName;
}//Setting Luggage Name
String getLuggageName(){
return luggageName;
}//Return luggage name
void setisDanger(boolean b){
isDanger = b;
}//Random Setting of Luggage Properties
boolean getIsDanger(){
return isDanger;
}//Return baggage properties
}
③Machine.java
import java.util.Random;
public class Machine {
void checkGoods(Goods goods) throws DangerException{
if (goods.isDanger){//If the luggage is true, throw an exception.
throw new DangerException();
}
}
public static void main(String[] args){
Random random = new Random();
Machine machine = new Machine();
//Optional range of baggage name
String name[] = {"vegetable", "apple", "gun", "candy", "cheery", "drink"};
Goods goods[] = new Goods[10];
System.out.printf("%-26s|%-13s|%s\n", "luggage", "Is it dangerous?", "Abnormal information");
System.out.println("------------------------------------");
for (int i = 0; i < goods.length; i++) {
int index = random.nextInt(6);
boolean b = random.nextBoolean();
goods[i] = new Goods();
goods[i].setLuggageName(name[index]);//Random Setting of Luggage Name
goods[i].setisDanger(b);//Random Setting of Luggage Properties
//Output baggage information
System.out.printf("%-2d. %-12s|%-12s|", i+1, goods[i].getLuggageName(), goods[i].getIsDanger());
if (!goods[i].getIsDanger()) {
System.out.println();
}
try{
machine.checkGoods(goods[i]);//If the hazard attribute is true, throw an exception
} catch (DangerException e){
System.out.println(e.showMessage());//Output exception information
}
}//for
}//main
}
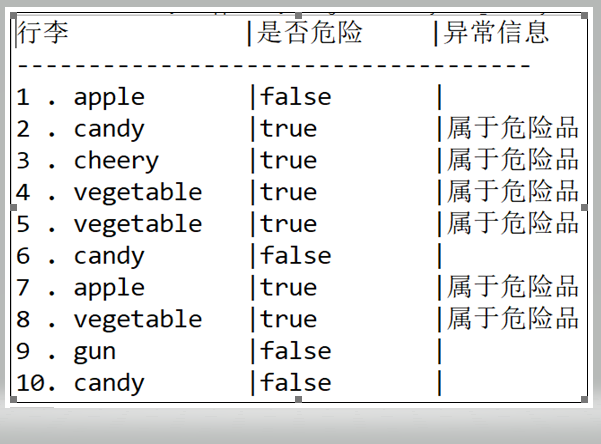
IV. Problems and Experiences in the Experiments
1. try-catch: Place the operation (code) that may have an exception in the try part. Once the try part throws an exception, or calls a method that may throw an exception object, and the method throws an exception object, the try part immediately terminates execution and turns to the catch part (try-catch can be composed of several catches to handle the corresponding exception separately).
2. All exception classes in Java are subclasses of Exception class and belong to the category of inheritance. The use of exception classes helps to deepen the understanding of inheritance.
3. Java allows you to customize exception classes. A custom exception class can handle unique exceptions in the class. A custom exception class needs to be a subclass of the Exception class.
4. java uses throw keyword to throw an instance of an Exception subclass to indicate an exception occurring
Keywords:
Java
Attribute
Programming
Added by skiingguru1611 on Sat, 18 May 2019 07:08:52 +0300