1, Polymorphism overview
2, Embodiment of polymorphism
Polymorphism in Code: parent class reference point Subclass object
Polymorphic format:
Parent class name object name = new subclass name ();
perhaps
Interface name object name = new implementation class name ();
code implementation
Create parent class
public class Fu { public void method(){ System.out.println("Parent method"); } public void methodFu(){ System.out.println("Parent class specific methods"); } }
Create subclass
public class Zi extends Fu{ @Override public void method() { System.out.println("Parent method override)"); } }
Test class
public class Demo01Multi { public static void main(String[] args) { //Polymorphism: parent class name object name = new subclass name (); //Parent class reference on the left, child class object on the right Fu obj = new Zi(); //Calling a parent class method is actually overridden by a child class obj.method(); //Calling parent class specific methods obj.methodFu(); } }
Operation results
Parent method override) Parent class specific methods Process finished with exit code 0
3, Polymorphic access member variables and methods
There are two ways to access member variables:
1. Directly access the member variable through the object name: see who is on the left of the equal sign and who is preferred. If not, look up.
2. Access member variables indirectly through member methods: see who the method belongs to and who uses it first. If not, look up.
Upper code
Create parent class
public class Fu { int num = 10; public void showNum(){ System.out.println(num); } public void method(){ System.out.println("Parent method"); } public void methodFu(){ System.out.println("Parent class specific methods"); } }
Create subclass
public class Zi extends Fu{ int num = 20; int age = 30; @Override public void method() { System.out.println("Subclass method"); } @Override public void showNum() { System.out.println(num); } public void methodZi() { System.out.println("Subclass specific methods"); } }
Create a test class to access member variables
public class Demo01MultiField { public static void main(String[] args) { Fu obj = new Zi(); //A subclass cannot override a member variable that overrides a parent class //Directly access the member variables through the object name. Whoever is on the left of the equal sign has priority to use, without looking up System.out.println(obj.num);//10 //The parent class member variable does not exist, and the child class cannot be accessed. Wrong writing // System.out.println(obj.age); System.out.println("============"); //Access member variables indirectly through member methods: see who the method belongs to and who uses it first. If not, look up. obj.showNum();//10 before overwriting obj.showNum();//20 after overwriting } }
Create test class access member method
public class Demo02MultiField { public static void main(String[] args) { //polymorphic Fu obj = new Zi(); //Compile to the left and run to the right obj.method();//Both father and son have priority over son obj.methodFu();//If the parent has children, look up for the parent class // obj.methodZi(); The parent class on the left does not have this method } }
4, Benefits of polymorphism
5, Reference type conversion
Upper code
Create parent class:
public abstract class Animal { public abstract void eat(); }
Create subclass 1:
public class Cat extends Animal { @Override public void eat() { System.out.println("Cats eat fish"); } public void play(){ System.out.println("Special method: cat catches mouse"); } }
Create subclass 2:
public class Dog extends Animal{ @Override public void eat() { System.out.println("Dogs eat meat"); } public void play(){ System.out.println("Woof, woof"); } }
Create test class 1:
/* Upward transformation must be safe, no problem and correct. But there is also a drawback: Once an object is transformed upward into a parent class, it cannot call the original unique content of the child class. Solution: use the downward transformation of objects [restore]. */ public class Demo01Main { public static void main(String[] args) { //The upward transformation is that the parent class reference points to the child class object Animal animal = new Cat();//It was originally created as a cat animal.eat(); // animal.play();// Wrong writing //Transition down, similar to cast //Format: subclass name new object name = (subclass name) old object name Cat cat = (Cat)animal; //At this point, subclass specific methods can be called cat.play();//Special method: cat catches mouse //Wrong downward transformation Dog dog = (Dog)animal;//No error reported here //dog.eat();// Exception at runtime: ClassCastException: class conversion exception } }
Create test class 2:
/* How can I know what subclasses an object referenced by a parent class is originally? Format: Object instanceof class name This will result in a boolean value, that is, whether the previous object can be used as an instance of the following type. */ public class Demo02Instanteof { public static void main(String[] args) { //Upward transformation Animal animal = new Cat(); // Animal animal = new Dog(); //Downward transformation //Judge whether the animal is a cat or a dog //If it's a dog, turn it into a dog if(animal instanceof Dog){ System.out.println("This animal is a dog"); Dog dog = (Dog)animal; dog.eat(); dog.play(); } else if(animal instanceof Cat){ System.out.println("This animal is a cat"); Cat cat = (Cat)animal; cat.eat(); cat.play(); } sendAnimal(animal); } public static void sendAnimal(Animal animal){ //Downward transformation //Judge whether the animal is a cat or a dog //If it's a dog, turn it into a dog if(animal instanceof Dog){ System.out.println("This animal is a dog"); Dog dog = (Dog)animal; dog.eat(); dog.play(); } else if(animal instanceof Cat){ System.out.println("This animal is a cat"); Cat cat = (Cat)animal; cat.eat(); cat.play(); } } }
6, Interface, a comprehensive case of polymorphism: laptop
Notebook computer:
Case study:
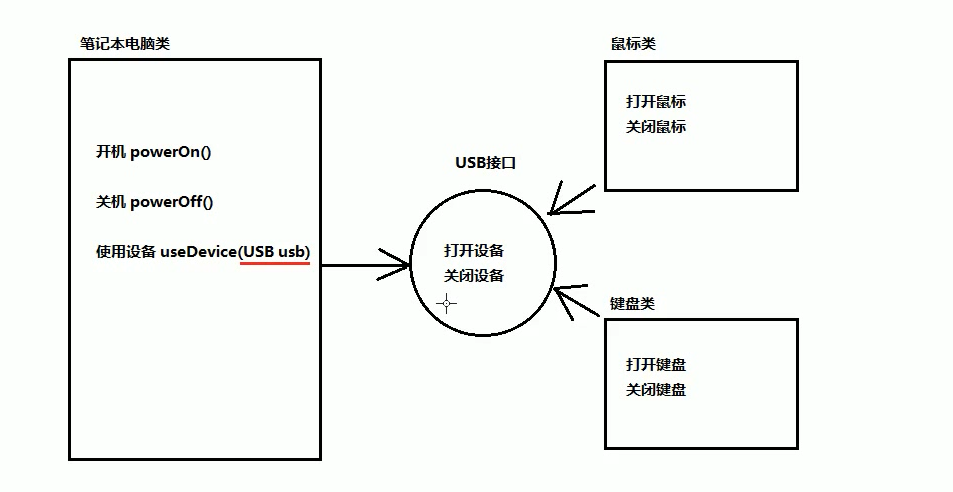
Code implementation:
Create USB interface
public interface USB { public abstract void open(); public abstract void close(); }
Create mouse class
public class Mouse implements USB{ @Override public void open() { System.out.println("Open mouse"); } @Override public void close() { System.out.println("Turn off the mouse"); } public void play2(){ System.out.println("Click the mouse"); } }
Create keyboard class
public class Keyboard implements USB{ @Override public void open() { System.out.println("Open keyboard"); } @Override public void close() { System.out.println("turn off keyboard"); } public void play1(){ System.out.println("keyboard entry"); } }
Create computer class
public class Computer { public void putOn(){//Turn on the computer System.out.println("Power on"); } public void putOff(){//Turn off the computer System.out.println("Shut down"); } public void usbMain(USB usb){//Method of using USB usb.open(); if(usb instanceof Keyboard){ Keyboard keyboard = (Keyboard) usb; keyboard.play1(); } else if(usb instanceof Mouse){ Mouse mouse = (Mouse) usb; mouse.play2(); } usb.close(); } }
Create test class
public class DemoMain { public static void main(String[] args) { //Create computer Computer computer = new Computer(); //Turn on the computer computer.putOn(); //Prepare the mouse for use by the computer // Mouse mouse = new Mouse(); // USB usb = mouse; //perhaps USB usb = new Mouse(); // usb.open(); computer.usbMain(usb); //Create a keyboard Keyboard keyboard = new Keyboard(); computer.usbMain(keyboard); //Turn off the computer computer.putOff(); } }
At the end of this article, we can communicate and discuss technical issues with our favorite partners. Thank you for your support!