java Basics
4, Process control
4.1. Basic structure
-
Sequential structure
- The command line is executed sequentially
-
Branch structure (select structure)
-
Different results can be obtained by selecting different execution processes according to conditions
-
category
- Single branch statement
if–else
-
Multi branch statement
switch
-
-
Cyclic structure
- After a certain number of repetition conditions are met by the user, the machine can automatically execute certain conditions
-
Circular statement
For example: while, for
4.2. Branch (select) statement if
-
if statement ----- single branch
-
Format:
If (conditional expression)
{s1 statement;}
If the conditional expression is true, the s1 statement will be executed; if false, the s1 statement will be skipped
If can be used alone, but else cannot be used alone. It must be used together with if
package jiaocai; public class test19if sentence { public static void main(String[]args) { int num=6; if(num%2==0) { System.out.println("num Even number"); } /*if There is only one statement after it, {} is omitted, and there will be no error, if(num%2==0) System.out.println("num Is an even number ""); It is suggested not to omit, cultivate good habits and reduce unnecessary Trouble to*/ System.out.println("num The value of is"+num); int balance=500; if(balance==500); { System.out.println("buy a car"); System.out.println("Eat and sleep"); /* The second if statement has no control, but will be executed, However, whether the {} statement is executed or not has nothing to do with if and must be executed if(balance==500);{ Observe carefully: Because the semicolon is used after the bracket of if, At this time, the if statement controls an empty statement. If is true, Execute an empty statement. If it is not true, skip the empty statement controlled by if if(balance==500);Add a semicolon after if(), It won't report an error, but it's a bug that makes if not as expected Control function ---------------------------------------- System.out.println("Buy a car "; --- statement 1 System.out.println("Eat and sleep "; --- statement 2 } At this time, the content in {} belongs to "{} non static code block" Whether the second if is true or not, statements 1 and 2 Will be implemented */ } } }
num is an even number
The value of num is 6
buy a car
Eat and sleep -
-
if – else -- double branch
- Format:
If (conditional expression){
s1;
}else{
s2;
}
If the conditional expression is true, execute S1; if false, execute S2
Either black or white, choose one
package jiaocai; public class test20if_else sentence { public static void main(String[] args) { // Method stub automatically generated by TODO int balance=50; if(balance==500) { System.out.println("Buy a good car"); }else { System.out.println("Buy a bike"); } System.out.println("Eat and sleep"); }//The choice between two is either black or white //The {} scope is the control scope, that is, the scope }
Buy a bike
Eat and sleep -
If – else if ------ multi branch
Format:
If (conditional expression 1){
s1; }else if(Conditional expression 2){ s2;
}else{
s3;
}
- If conditional expression 1 is true, execute S1 and end the multi branch structure.
- If conditional expression 1 is false, judge conditional expression 2. If conditional expression 2 is true, execute S2 and end the multi branch structure
- If conditional expression 1 is false and conditional expression 2 is false, execute S3 and end the multi branch structure
-
Note: 1. In this process control chapter, s1/s2/s3/s4, etc. are only a symbol, representing a statement block {}, not a single statement2. In the principle of proximity, each else is paired with its nearest if
3. No matter how complex the judgment process is, the final execution can only be one statement block {}
package jiaocai; //else is matched according to the principle of proximity. else cannot be used alone, //Must be used with if public class test21if_elseif sentence { public static void main(String[] args) { // Method stub automatically generated by TODO int balance=500; if(balance==500) { System.out.println("Buy Lamborghini"); }else if(balance>=100&&balance<500) { System.out.println("Buy Land Rover"); }else { System.out.println("Don't buy a car for the time being"); } } } /* *int balance=500; if(balance==500) { Statement 1 System.out.println("buy Lamborghini"); }else if(balance>=100&&balance<500) { Statement 2 System.out.println("buy Land Rover"); }else { Statement 3 System.out.println("not buying a car temporarily"); No matter how complex the judgment process is, "the final execution can only be Is the understanding of a statement block '{}': In this example, only one of statement 1, statement 2 and statement 3 can be executed */
Buy Lamborghini
-
if – nesting of else statements
format
If (conditional expression){
`Other control statements;
}else{
Control statement;
}
Note: the statements involved must be complete, if – else statements must be complete, and the structure of other control statements must also be complete
-
Correct writing of Boolean in if
- boolean A=true;
if(A) is the statement block that executes if control when the conditional expression is true
if(A==true);
There are no grammatical errors, but it is not recommended. If you accidentally miss one=
become:
if(A=true)
Finished, because an equal sign means assignment in programming. I like to mention a big bug in the program and completely lose the if selection function
- To reverse, if (! A)
-
be careful:
-
If requires true or false judgment, while else and else if do not
-
else cannot be used alone or in combination with if
package jiaocai; public class test22if Boolean hide Correct format { public static void main(String[] args) { // Method stub automatically generated by TODO boolean flag=true; if(flag)// ! flag reverse System.out.println("flag The value of is"+flag); //Wrong format if(flag=true) nonstandard format if(flag==true) else { System.out.println("flag The value of is"+flag); } } }
The value of flag is true
-
4.3. Multilayer judgment switch
The score of "excellent" is 0-7, and the score of "failure" is 0-7 according to the background
-
Two ways to solve
- Use if else to make multiple judgments, but the operation is complex
- Use Switch statement (recommended)
-
Switch statement
-
Select one from many
-
Enhanced version of if else if, which is specially used to deal with the problem of multiple choice
-
Format:
Switch (parameter for judgment)
//Parameters can be integers, enumerations, strings
case constant expression 1: statement 1; [break;]//break; Function: after executing statement 1, jump out of Switch and do not execute the following statements
case constant expression 2: Statement 2; [break;]//If you do not write break, you will continue to execute the following statements and will not jump out of the Switch immediately
[default] (optional, equivalent to default): statement n+1; [break],//If the input parameters cannot match any of the above case s, use default to remind and identify. At this time, whether to write or not to write break is of little significance. It's all at the end. No matter what the situation is, you have to end the Switch statement
-
be careful:
- Uniqueness. Two case s cannot correspond to the same value
- The constant expression must be an int or character type or string of basic data type (character type can be converted to int), which can only be supported in later versions of JDK7
- The judgment conditions of the switch statement can accept int, byte, char and short, and cannot accept other types, except those that can be implicitly converted to the above types
package jiaocai; //Processing of multi-layer judgment -- grade division public class test23Switch Use of statements { public static void main(String[] args) { // Method stub automatically generated by TODO int grade=7; if(grade>=0&&grade<=5) //Realize the simplified processing of 0-5 points grade='A'; //It is not optional, that is, it will be executed if it meets the if conditions, and skip if it does not meet the requirements, //However, when the Switch starts, it will be executed. It has nothing to do with whether the if condition is met or not //Scope determines switch(grade) { case 10:System.out.println("Excellent performance,The results are:"+grade);break; case 9:System.out.println("Excellent performance,The results are:"+grade);break; case 8:System.out.println("Good grades,The results are:"+grade);break; //Jump out after executing this statement, jump out the following statement, //The above statement will not be executed because it matches the corresponding case case 7:System.out.println("The score is medium,The results are:"+grade);break; case 6:System.out.println("The score is medium,The results are:"+grade);break; /*case 5:System.out.println("The score is unqualified, and the score is: "+ grade);break; case 4:System.out.println("The score is unqualified, and the score is: "+ grade);break; case 3:System.out.println("The score is unqualified, and the score is: "+ grade);break; case 2:System.out.println("The score is unqualified, and the score is: "+ grade);break; case 1:System.out.println("The score is unqualified, and the score is: "+ grade);break; case 0:System.out.println("The score is unqualified, and the score is: "+ grade);break; */ case 'A':System.out.println("The result is unqualified,The results are:"+'A'+"That is, the score is 0~5 Between points");break; /*A Not equivalent to 'a', the former is the letter A, the latter is the character a, and the character a can be used in Unicode code Corresponding int type Important: "Boolean cannot be converted to other types. If it involves size comparison, the result must be Boolean" case grade>=0&&grade<=5:System.out.println("The score is unqualified, and the score is: "+ grade);break; An error is reported (boolean cannot be converted to int type) grade > = 0. The result must be boolean. boolean cannot be converted to any type case The following constant expression must be int type or character type that can be converted to int type ? case if(grade>=0&&grade<=5):System.out.println("The score is unqualified, and the score is: "+ grade);break; */ default:System.out.println("The grade is invalid,The results are:"+grade); //If you do not match any of the above case s, use default, and use break for the last side. Please feel free } } }
Score: medium, score: 7
Optimize for the above:
package jiaocai;//Use break to control the output of the same content public class test23a_Switch supplement { public static void main(String[] args) { // Method stub automatically generated by TODO int grade=45; switch(grade) { case 10: case 9:System.out.println("Excellent performance");break; case 8: case 7:System.out.println("Good grades");break; case 6:System.out.println("Qualified results");break; case 5: case 4: case 3: case 2: case 1: case 0:System.out.println("Unqualified results");break; //When grade = 0 --- 5, the same content will be output, which only needs to be processed in case 0: default:System.out.println("Invalid score"); } } }
Invalid score
4.4. Circular statement
Background: deal with the problem circularly and repeat the steps
4.4.1. Statement while
- format
while (Boolean expression){
Returns a Boolean value (usually used for self increasing and self decreasing variable i) to judge whether to continue the cycle
Statements that can be executed circularly;
}
be careful:
- Boolean expression cannot be empty, otherwise an error will be reported
- Boolean expressions cannot be constants, such as true. Otherwise, they will be another program bug. Either they will not cycle or they will be dead cycles, which will consume machine resources on a large scale
- while(); Semicolons are not allowed after parentheses. For the same reason as if statements, using semicolons means that while controls an empty statement, which makes no sense
- For example, while(x==5);
- No programming, no syntax violations
- Because empty statements are executed (there is no loop termination condition, and while will enter an endless loop), a lot of memory space is wasted. If you want to find and modify in a large project, you will waste a lot of debugging time
- while(false) {}, direct error reporting
- The loop body should have the expression of infinite approximation to the critical condition (often self increasing and self decreasing), otherwise it is also a dead loop
package jiaocai; public class test24while sentence { public static void main(String[] args) { // Method stub automatically generated by TODO long i=1L,sum=0L; //When the value is large, remember to use long type to avoid inaccurate accuracy while(i<=100) { //Boolean expression variables, control type i + + at the end, loop termination condition //------Between {} is the circular body sum=sum+i; System.out.println("i be equal to:"+i+"When"+"sum The value of is:"+sum); i++; //Expression of infinite approximation loop termination condition } System.out.println("The sum of one to one hundred is:\n"+sum); } }
When i is equal to: 1, the value of sum is: 1
When i is equal to: 2, the value of sum is: 3
When i is equal to: 3, the value of sum is: 6
When i is equal to: 4, the value of sum is: 10
When i is equal to: 5, the value of sum is: 15
When i is equal to: 6, the value of sum is: 21
When i is equal to: 7, the value of sum is: 28
When i is equal to: 8, the value of sum is: 36
When i is equal to 9, sum is 45
When i is equal to: 10, the value of sum is: 55
When i is equal to: 11, the value of sum is: 66
When i is equal to: 12, the value of sum is: 78
When i is equal to: 13, the value of sum is: 91
When i is equal to: 14, the value of sum is: 105
When i is equal to: 15, the value of sum is: 120
When i is equal to 16, the value of sum is 136
When i is equal to: 17, the value of sum is: 153
When i is equal to 18, the value of sum is 171
When i is equal to: 19, the value of sum is: 190
When i is equal to: 20, the value of sum is: 210
When i is equal to: 21, the value of sum is: 231
When i is equal to: 22, the value of sum is: 253
When the value of i is equal to 23: sum
When i is equal to: 24, the value of sum is: 300
When i is equal to: 25, the value of sum is: 325
When i is equal to: 26, the value of sum is: 351
When i is equal to: 27, the value of sum is: 378
When i is equal to: 28, the value of sum is: 406
When i is equal to: 29, the value of sum is: 435
When i is equal to: 30, the value of sum is: 465
When i is equal to: 31, the value of sum is: 496
When i is equal to: 32, the value of sum is: 528
When i is equal to: 33, the value of sum is: 561
When i is equal to: 34, the value of sum is: 595
When i is equal to 35, sum is 630
When i is equal to 36, the value of sum is 666
When i is equal to: 37, the value of sum is: 703
When i is equal to: 38, the value of sum is: 741
When i is equal to: 39, the value of sum is: 780
When i is equal to: 40, the value of sum is: 820
When i is equal to: 41, the value of sum is: 861
When i is equal to: 42, the value of sum is: 903
When i is equal to: 43, the value of sum is: 946
When i is equal to: 44, the value of sum is: 990
When i is equal to: 45, the value of sum is: 1035
When i is equal to: 46, the value of sum is: 1081
When i is equal to: 47, the value of sum is: 1128
When i is equal to: 48, the value of sum is: 1176
When i is equal to: 49, the value of sum is: 1225
When i is equal to: 50, the value of sum is: 1275
When i is equal to: 51, the value of sum is: 1326
When i is equal to: 52, the value of sum is: 1378
When i is equal to: 53, the value of sum is: 1431
When i is equal to: 54, the value of sum is: 1485
When i is equal to: 55, the value of sum is: 1540
When i is equal to: 56, the value of sum is: 1596
When i equals: 57, the value of sum is 1653
When i is equal to: 58, the value of sum is: 1711
When i equals: 59, the value of sum is 1770
When i is equal to: 60, the value of sum is: 1830
When i is equal to: 61, the value of sum is: 1891
When i is equal to: 62, the value of sum is: 1953
When i equals: 63, the value of sum is: 2016
When i is equal to: 64, the value of sum is: 2080
When i is equal to: 65, the value of sum is: 2145
When i is equal to: 66, the value of sum is: 2211
When i is equal to: 67, the value of sum is: 2278
When i is equal to: 68, the value of sum is: 2346
When i is equal to: 69, the value of sum is: 2415
When i is equal to 70, sum is 2485
When i is equal to: 71, the value of sum is: 2556
When i equals: 72, the value of sum is: 2628
When i is equal to: 73, the value of sum is: 2701
When i is equal to: 74, the value of sum is: 2775
When i is equal to 75, the value of sum is 2850
When i is equal to: 76, the value of sum is: 2926
When i is equal to: 77, the value of sum is: 3003
When i equals: 78, the value of sum is: 3081
When i is equal to 79, the value of sum is 3160
When i is equal to: 80, the value of sum is: 3240
When i is equal to: 81, the value of sum is: 3321
When i is equal to: 82, the value of sum is: 3403
When i is equal to 83, sum is 3486
When i is equal to: 84, the value of sum is: 3570
When i is equal to 85, sum is 3655
When i is equal to: 86, the value of sum is: 3741
When i is equal to: 87, the value of sum is: 3828
When i is equal to: 88, the value of sum is: 3916
When i is equal to: 89, the value of sum is: 4005
When i is equal to 90, sum is 4095
When i is equal to: 91, the value of sum is: 4186
When i is equal to: 92, the value of sum is: 4278
When i is equal to: 93, the value of sum is: 4371
When i is equal to 94, the value of sum is 4465
When i is equal to: 95, the value of sum is: 4560
When i is equal to: 96, the value of sum is: 4656
When i equals: 97, the value of sum is: 4753
When i equals: 98, the value of sum is: 4851
When i is equal to: 99, the value of sum is: 4950
When i is equal to: 100, the value of sum is: 5050
The sum of one to one hundred is:
5050
4.4.2.do while statement
Background: how to verify that the passwords entered twice are consistent?
- Format:
be careful:
- Regardless of the Boolean expression judged by while, do will be executed at least once
- Loop termination condition: while(!pwd1.equals(pwd2)); To judge whether to cycle or not, use epuals instead of "= =", which will be explained in the string chapter of the next year
- do while(); The semicolon cannot be omitted, or an error will be reported. It is different from while and should not be confused
- Boolean expression can be null and cannot be null
- Boolean expressions cannot be constants. There must be a statement to change the conditional expression in the loop body, otherwise it will form an endless loop
package jiaocai; import java.util.Scanner; //Import emulator's package public class test25do_while Realize twice password verification { @SuppressWarnings("resource")//Method 2 public static void main(String[] args) { // Method stub automatically generated by TODO //@SuppressWarnings("resource") / / method 1 Scanner sc=new Scanner(System.in); //Methods 1 and 2 to solve the sc alarm receive data from the keyboard String pwd1="0"; //Define the string and give the initial value String pwd2="-1"; do { System.out.println("Please enter your registration QQ Password for"); pwd1=sc.nextLine(); System.out.println("Please enter the confirmation password again"); pwd2=sc.nextLine(); //System.out.println("the passwords you entered twice are inconsistent, please re-enter!"); if(!pwd1.equals(pwd2)) { //A.equals(B) determines whether the two strings a and B are equal System.out.println("The passwords you entered twice are inconsistent or you did not enter the password, please re-enter!"); } }while(!pwd1.equals(pwd2)); //Judge the termination condition of the do while loop. The loop body is the do {} part System.out.println("Yours QQ Password setting succeeded!\n"+"Welcome to use QQ"); } }
Please enter your password to register QQ
123
Please enter the confirmation password again
234
Your password is not the same, please re-enter it twice!
Please enter your password to register QQ
123
Please enter the confirmation password again
123
Your QQ password is set successfully!
Welcome to QQ
4.4.3.for statement
4.4.3.1. Ordinary for statement
Format:
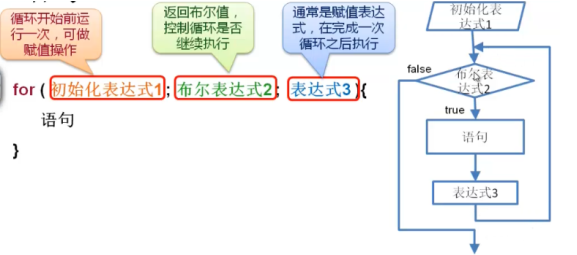
-
special
-
Infinite loop
For (write two English semicolons directly here){
...
} -
For optimization, use the break statement
-
package jiaocai; public class demo12 { public static void main(String[] args) { int x=0 ; for(;;){ //Set to dead cycle x++; //Self increasing expression x=x+1; System.out.println(x); if(x==4) { break; } /* * Set additional cycle conditions to turn the dead cycle into a cycle that can be stopped */ } } }
-
1
2
3
4
-
package jiaocai; public class test26for Statement summation { public static void main(String[] args) { // Method stub automatically generated by TODO int sum=0; for(int i=1;i<=5;i++) { //Define i and assign value, loop termination condition, wireless approximation termination condition sum+=i; //Simple writing method: sum=sum+i; System.out.println("i="+i+"Time,"+"sum The value of is:"+sum); } } }
When i=1, sum is 1
When i=2, sum is 3
When i=3, sum is 6
When i=4, sum is 10
When i=5, sum is 15
package jiaocai; //Exhaustive method public class test28 A hundred dollars for a hundred chickens { public static void main(String[] args) { // Method stub automatically generated by TODO int cock,hen,chick; for( cock=1;cock<=20;cock++) { //Cocks start from 1 and control the number of cocks for( hen=1;hen<=33;hen++) { //The number of hens starts from 1 and the number of hens is controlled for( chick=3;chick<=99;chick+=3) //The number of chicks starts from 3 and the number of chicks is controlled if(cock*5+hen*3+chick/3==100) { //Control hundred money if(cock+hen+chick==100) { //Control hundred chickens System.out.println("Cock buy"+cock+"Hen buy"+hen+"Chicken buy"+chick); } } } } } }
Cock buy 4 hen buy 18 chick buy 78
The cock buys 8, the hen buys 11, and the chick buys 81
The cock buys 12, the hen buys 4, and the chick buys 84
4.4.3.2.foreach statement
-
Operating environment: jdk1 The version after 5 is an enhanced version of the extension board of the for statement, which reduces the complexity of the for statement code. At present, it is widely used, especially on the array problem
-
format
-
-
Array: a collection of elements of the same data type arranged in a certain order
-
Traversal: access and read each element in the array or collection one by one
package jiaocai; public class test27foreach loop { public static void main(String[] args) { // Method stub automatically generated by TODO long arr[]= {12,23,45}; //Define an array called arr for(long x:arr) { //Assign the elements in the arr array to the long variable x in turn System.out.println("x The value of is:\n"+x); } } }
The value of x is:
12
The value of x is:
23
The value of x is:
45
-
one-dimensional
Traverse each element directly -
two-dimensional
- thinking
Convert two dimensions into one dimension - process
The first foreach traverses the rows of a two-dimensional array
The second foreach traverses each element of each row traversed in the previous step
- thinking
package Chapter VI_array; public class T4 Traversal array { public static void main(String[] args) { // Method stub automatically generated by TODO char ch[][]=new char[4][];//Create a 4-row array. The column is unknown and legal ch[0]=new char[] {'spring','Sleep','no','sleep','Dawn'};//Initialize rows ch[1]=new char[] {'place','place','smell','Cry','bird'}; ch[2]=new char[] {'night','come','wind','rain','sound'}; ch[3]=new char[] {'flower','fall','know','many','less'}; System.out.println("----foreach Statement traversal----"); System.out.println("foreach Statement traverses the principle of two-dimensional array, converts two-dimensional into one-dimensional, and then outputs it in turn"); for(char a[]:ch) { //Control the row, use the foreach statement to traverse all the elements, and assign all the rows (4 rows in total) of the ch array as elements to an array a for(char b:a) { //Output the elements of array a (this is a one-dimensional array), that is, the value of each index position System.out.print(b);//No line breaks, output } System.out.println();//Output a line and wrap it } } }
----foreach statement traversal----
foreach statement traverses the principle of two-dimensional array, converts two-dimensional into one-dimensional, and then outputs it in turn
Spring sleep without dawn
Birds sing everywhere
The sound of wind and rain at night
How many flowers do you know
4.5. Cycle control
4.5.1.break statement
Function: used to control the circulation or not
package jiaocai; public class test29break sentence { public static void main(String[]args) { int i=1; while(i>0) { System.out.println(i); i++; if(i==10) break; } System.out.println("i=10 When, comply with if The termination condition of the statement, break termination while Cycle"); } }
1
2
3
4
5
6
7
8
9
When i=10, if the termination conditions of the if statement are met, break terminates the while loop
-
Intelligent jump out
-
package jiaocai; public class test29a_break Statement Supplement 1 { public static void main(String[] args) { // Method stub automatically generated by TODO A:for(int i=0;i<3;i++) { System.out.println("i="+i); for(int j=0;j<5;j++) { //If there is no label, the break will jump out of the loop in which it is in System.out.println("\t"+"j="+j); //"\ t" function: 8 empty spaces for easy observation, i =? Which is the corresponding j if(j==3) break A ; //If you add A tag, you will jump out of the outer loop A, and only one J corresponding to i will be output } //j will never reach j=4. When j=3, it jumps out of the loop of j } } }
i=0
j=0
j=1
j=2
j=3
4.5.2.continue Statement
difference:
-
The most essential difference between and break
-
sentence difference break Jump out of this cycle continue Jump out of this loop, (the statement after continue in the body of this loop will not be executed) - The label is used in the same way as break
package jiaocai; public class test30continue sentence { public static void main(String[] args) { // Method stub automatically generated by TODO int i; for(i=0;i<=10;i++) { if(i%2!=0) continue; System.out.println(i); System.out.println("&&*"); /*From the output results, as long as it meets continue The condition of the previous sentence, that is, the value of i is an odd number The line continue statement starts with continue and goes down Any statement on the face will jump out, Do not execute, first perform incremental i + + operation, and then Judge and directly carry out the next cycle, otherwise The has 10 lines "& & *"*/ //The use of tags is the same as break } } }
0
&&*
2
&&*
4
&&*
6
&&*
8
&&*
10
&&*
C ontinue statement
difference:
-
The most essential difference between and break
-
sentence difference break Jump out of this cycle continue Jump out of this loop, (the statement after continue in the body of this loop will not be executed) - The label is used in the same way as break
package jiaocai; public class test30continue sentence { public static void main(String[] args) { // Method stub automatically generated by TODO int i; for(i=0;i<=10;i++) { if(i%2!=0) continue; System.out.println(i); System.out.println("&&*"); /*From the output results, as long as it meets continue The condition of the previous sentence, that is, the value of i is an odd number The line continue statement starts with continue and goes down Any statement on the face will jump out, Do not execute, first perform incremental i + + operation, and then Judge and directly carry out the next cycle, otherwise The has 10 lines "& & *"*/ //The use of tags is the same as break } } }
0
&&*
2
&&*
4
&&*
6
&&*
8
&&*
10
&&*