1, Experiment purpose and requirements
1. Experimental purpose
- Master how to use classes to create and encapsulate objects;
- Master how to define and construct a class;
- Master method overload and polymorphism;
- Master the combination of objects and the method of parameter value transfer;
- Understand and master the differences between class variables and instance variables, and between class methods and instance methods.
2. Experimental requirements
- Understand and master various basic concepts of class and object;
- Master how to define classes and create objects;
- Master how to write programs to verify the basic concepts and determine whether the concepts are correctly understood;
- Various problems in the experiment are analyzed and summarized.
2, Experimental environment
- Hardware requirements: one computer (with Windows 10 operating system)
- Software requirements: JDK-14.0.2, Intellij IDEA 2020.1.2
3, Experimental contents (experimental scheme, experimental steps, design ideas, etc.)
- Experimental scheme: generate and run the prepared Java class file to see whether it meets the expected effect.
- Experimental steps: create java project and Java class file, write code and generate Java program, run and test.
- Design idea:
- Create java project, package and Java class file first;
- Program to create a class Phone and a class Property;
a) Programming to define various instance variables, instance functions, class variables and class functions;
b) Programming to realize the functions of object combination, constructor, overload and polymorphism;
c) Create a phone class object phone in the main function to set the relevant data of the mobile phone;
d) Output through the show() function;
e) Through Qusetion_one() and question_ The two() function asks whether to connect to the Internet and play music;
f) Enter yes and no through the keyboard to select; - Improve relevant functions and codes, generate Java programs, check whether the results are correct, and complete the experimental report.
4, Experimental results and analysis
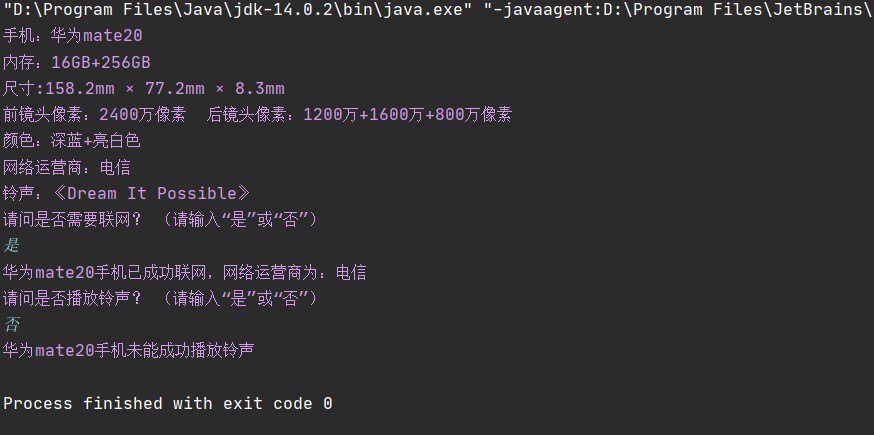
5, Attached source code
package experiment.two;
import java.util.Scanner;
class Property//Define class
{ //Define properties
protected String name;//Brand name
protected String Network_Support;//Supported network operators
protected String Volume;//Ringing tone
public Property(String name,String Network_Support,String Volume)
{//Parametric construction method
this.name=name;
this.Network_Support=Network_Support;
this.Volume=Volume;
}
}
class Phone//Define class
{
//Define properties
protected String size;//Instance variables: Dimensions
protected String color;//Instance variables: color
protected String RAM;//Instance variables: memory
protected String pixel_front;//Instance variable: front lens pixels
protected String pixel_rear;//Instance variable: rear lens pixels
protected static String length;//Class variables: length
protected static String width;//Class variable: width
protected static String thickness;//Class variable: thickness
protected Property property;//Object composition declares the object of the Property class
public Phone(String name,String Network_Support,String Volume)
{//Parametric construction method
this.property=new Property(name,Network_Support,Volume);//Object combination
}
public static String get_Size(double l,double w,double t)
{//Overloaded method class method
length=String.valueOf(l);//Convert numbers to strings
width=String.valueOf(w);//Convert numbers to strings
thickness=String.valueOf(t);//Convert numbers to strings
return length+"mm"+" × "+width+"mm"+" × "+thickness+"mm";
}
public void get_Phone(int ram1,int ram2)
{//Overloaded method instance method
String r1,r2;
r1=String.valueOf(ram1);//Convert numbers to strings
r2=String.valueOf(ram2);//Convert numbers to strings
RAM=r1+"GB+"+r2+"GB";
}
public void get_Phone(String c1, String c2)
{//Overloaded method instance method
String cs1,cs2;
cs1=c1.trim().substring(0,c1.indexOf("colour"));//Remove all spaces before and after the string and intercept the string
cs2=c2.trim().substring(0,c2.indexOf("colour"));//Remove all spaces before and after the string and intercept the string
color=cs1+"+"+cs2+"colour";
}
public void get_Phone(int f,int r1,int r2,int r3)
{//Overloaded method instance method
String Front,Rear1,Rear2,Rear3;
Front=String.valueOf(f);//Convert numbers to strings
Rear1=String.valueOf(r1);//Convert numbers to strings
Rear2=String.valueOf(r2);//Convert numbers to strings
Rear3=String.valueOf(r3);//Convert numbers to strings
pixel_front=Front+"Megapixel";
pixel_rear=Rear1+"ten thousand+"+Rear2+"ten thousand+"+Rear3+"Megapixel";
}
public void get_Network(String whether)//Behavior: Networking
{
switch (whether)
{
case "yes":
System.out.println(property.name+"The mobile phone has been successfully connected to the Internet. The network operator is:"+property.Network_Support);
break;
case "no":
System.out.println(property.name+"The mobile phone failed to connect to the Internet successfully");
break;
default:break;
}
}
public void get_Volume(String whether)
{//Behavior: play ringtones
switch (whether)
{
case "yes":
System.out.println(property.name+"Ringtone played successfully:"+property.Volume);
break;
case "no":
System.out.println(property.name+"The phone failed to play the ringtone successfully");
break;
default:break;
}
}
public void show() //Output relevant parameters of mobile phone
{
System.out.println("mobile phone:"+property.name);
System.out.println("Memory:"+RAM);
System.out.println("size:"+size);
System.out.println("Front lens pixels:"+pixel_front+" Rear lens pixels:"+pixel_rear);
System.out.println("Color:"+color);
System.out.println("Network operator:"+property.Network_Support);
System.out.println("Ringtone:"+property.Volume);
}
public void Question_one()//Definition question: ask whether you want to connect to the Internet
{
System.out.println("Do you need to connect to the Internet? (please enter "yes" or "no")");
}
public void Question_two()//Definition question: ask whether to play the ringtone
{
System.out.println("Do you want to play the ringtone? (please enter "yes" or "no")");
}
}
public class program_1 {
public static void main(String[] args) {
Phone phone=new Phone("Huawei mate20","telecom","<Dream It Possible>");
//Create object and initialize
phone.get_Phone(16,256);//polymorphic
phone.get_Phone("navy blue","Bright white");//polymorphic
phone.get_Phone(2400,1200,1600,800);
phone.size=phone.get_Size(158.2,77.2,8.3);//Call class function
phone.show();//Call the function to output the relevant parameters of the mobile phone
phone.Question_one();//Call the function and ask whether it is online
Scanner scanner1=new Scanner(System.in);//Call the system class and input data through the keyboard
String w1=scanner1.nextLine().trim();//Read the input and remove the front and back spaces
phone.get_Network(w1);//Call function, networking
phone.Question_two();//Call the function to ask whether to play the ringtone
Scanner scanner2=new Scanner(System.in);//Call the system class and input data through the keyboard
String w2=scanner2.nextLine().trim();//Read the input and remove the front and back spaces
phone.get_Volume(w2);//Call the function to play the ringtone
}
}