371-405 didn't see it
Knowledge points
- Data type conversion
Object oriented (Part 2)
static
Singleton implementation (a class can only be called once)
package com.atguigu.java2; /*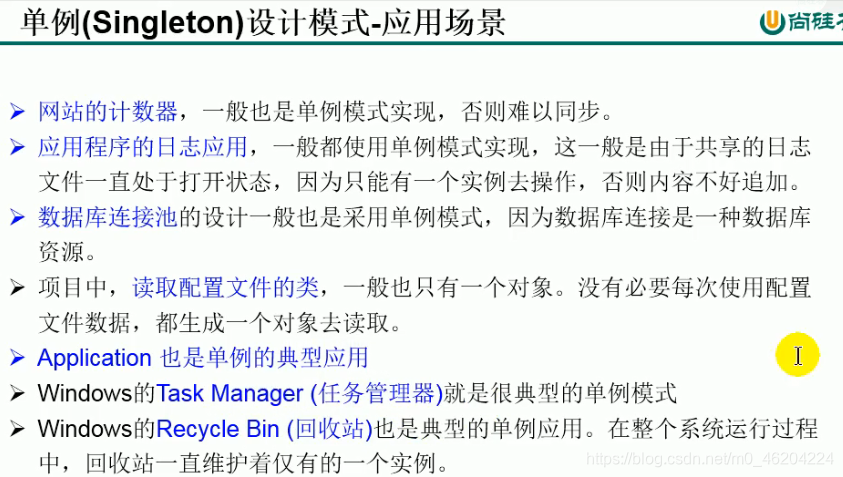 * Single example design mode: * 1. The so-called class singleton design pattern is to take certain methods to ensure that there can only be one object instance for a class in the whole software system. * * 2. How? * Hungry vs lazy * * 3. Distinguish between hungry and lazy * Hungry Han style: * Disadvantage: the object takes too long to load. * Benefit: hungry Chinese style is thread safe * * Lazy: benefit: delay the creation of objects. * The disadvantage of the current writing method: thread is not safe. ---- > When the multithreaded content is, modify it again * * */ public class SingletonTest1 { public static void main(String[] args) { // Bank bank1 = new Bank(); // Bank bank2 = new Bank(); Bank bank1 = Bank.getInstance(); Bank bank2 = Bank.getInstance(); System.out.println(bank1 == bank2); } } //Hungry Han style class Bank{ //1. Constructor of privatization class private Bank(){ } //2. Create class objects internally //4. This object must also be declared static private static Bank instance = new Bank(); //3. Provide public static methods to return class objects public static Bank getInstance(){ return instance; } }
package com.atguigu.java2; /* * Lazy implementation of singleton mode * */ public class SingletonTest2 { public static void main(String[] args) { Order order1 = Order.getInstance(); Order order2 = Order.getInstance(); System.out.println(order1 == order2); } } class Order{ //1. Constructor of privatization class private Order(){ } //2. Declare the current class object without initialization //4. This object must also be declared as static private static Order instance = null; //3. Declare public and static methods that return the current class object public static Order getInstance(){ if(instance == null){ instance = new Order(); } return instance; } }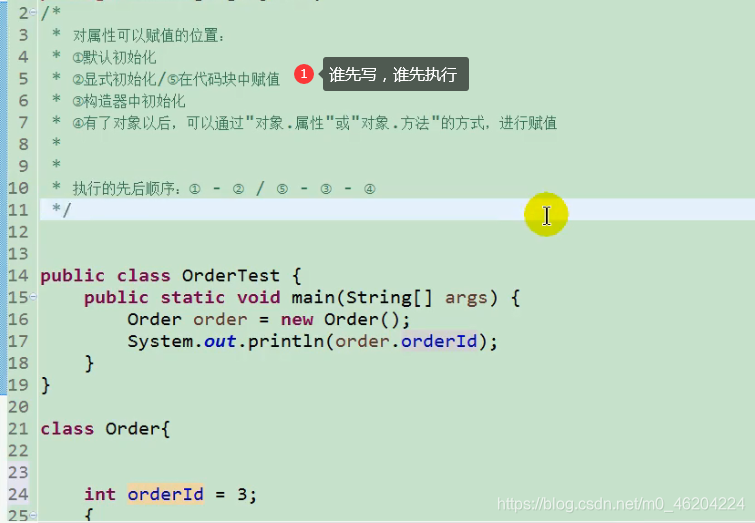
Code block
package com.atguigu.java3; /* * Class: code block (or initialization block) * * 1. Function of code block: used to initialize classes and objects * 2. If the code block is decorated, you can only use static * 3. Classification: static code block vs non static code block * * 4. Static code block * >There can be output statements inside * >Executes as the class loads, and only once * >Function: initialize class information * >If multiple static code blocks are defined in a class, they are executed in the order of declaration * >The execution of static code blocks takes precedence over the execution of non static code blocks * >Static code blocks can only call static attributes and static methods, not non static structures * * 5. Non static code block * >There can be output statements inside * >Executes as the object is created * >Each time an object is created, a non static block of code is executed * >Function: you can initialize the properties of the object when creating the object * >If multiple non static code blocks are defined in a class, they are executed in the order of declaration * >Static attributes, static methods, or non static attributes and non static methods can be called in non static code blocks * */ public class BlockTest { public static void main(String[] args) { String desc = Person.desc; System.out.println(desc); Person p1 = new Person(); Person p2 = new Person(); System.out.println(p1.age); Person.info(); } } class Person{ //attribute String name; int age; static String desc = "I am a person"; //constructor public Person(){ } public Person(String name,int age){ this.name = name; this.age = age; } //Non static code block { System.out.println("hello, block - 2"); } { System.out.println("hello, block - 1"); //Call non static structure age = 1; eat(); //Call static structure desc = "I am a person who loves learning 1"; info(); } //static code block static{ System.out.println("hello,static block-2"); } static{ System.out.println("hello,static block-1"); //Call static structure desc = "I am a person who loves learning"; info(); //Non static structures cannot be called // eat(); // name = "Tom"; } //method public void eat(){ System.out.println("having dinner"); } @Override public String toString() { return "Person [name=" + name + ", age=" + age + "]"; } public static void info(){ System.out.println("I am a happy person!"); } }
Keyword - final
abstrct
Abstract class can write abstract methods, but abstract methods cannot write method bodies (application: when we are not sure how to write method bodies), we can use subclass inheritance and then override methods in subclasses
For example, abstract findArea() in the parent class is uncertain. When calling a subclass, override the abstract findArea() method (find circle and square) according to subclass 1 created by the subclass.
The anonymous class mentioned above refers to the class name that is not displayed when the object of the class is not created,
The anonymous subclass object of the class here is used for the abstract class, because the abstract class cannot be used by new, but only by the subclass extends,
However, it can also be called by the following methods, but the abrasct method must be overridden
interface
A subclass has only one parent class, but can have multiple interface s
Infinterface can only be called after passing through the object of the class, that is, encapsulated in the class
package com.atguigu.java1; /* * Use of interfaces * 1.Interfaces are defined using interface s * 2.Java In, interface and class are two structures in parallel * 3.How to define an interface: define members in an interface * * 3.1 JDK7 And before: only global constants and abstract methods can be defined * >Global constant: public static final However, when writing, it can be omitted * >Abstract method: public abstract * * 3.2 JDK8: In addition to defining global constants and abstract methods, you can also define static methods and default methods (omitted) * * 4. Constructor cannot be defined in interface! This means that the interface cannot be instantiated * * 5. Java In development, interfaces are used by letting classes implement * If the implementation class covers all abstract methods in the interface, the implementation class can be instantiated * If the implementation class does not cover all the abstract methods in the interface, the implementation class is still an abstract class * * 6. Java Class can implement multiple interfaces -- >, which makes up for the limitation of Java single inheritance * Format: Class AA extensions BB implementations CC, DD, EE * * 7. Interfaces can inherit from one interface to another, and multiple interfaces can inherit * * ******************************* * 8. The specific use of the interface reflects polymorphism * 9. Interface can actually be regarded as a specification * * Interview question: what are the similarities and differences between abstract classes and interfaces? * */ public class InterfaceTest { public static void main(String[] args) { System.out.println(Flyable.MAX_SPEED); System.out.println(Flyable.MIN_SPEED); // Flyable.MIN_SPEED = 2; Plane plane = new Plane(); plane.fly(); } } interface Flyable{ //Global constant public static final int MAX_SPEED = 7900;//First cosmic velocity int MIN_SPEED = 1;//public static final is omitted //Abstract method public abstract void fly(); //public abstract is omitted void stop(); //Interfaces cannot have constructors // public Flyable(){ // // } } interface Attackable{ void attack(); } class Plane implements Flyable{ @Override public void fly() { System.out.println("Take off by engine"); } @Override public void stop() { System.out.println("Driver deceleration stop"); } } abstract class Kite implements Flyable{ @Override public void fly() { } } class Bullet extends Object implements Flyable,Attackable,CC{ @Override public void attack() { // TODO Auto-generated method stub } @Override public void fly() { // TODO Auto-generated method stub } @Override public void stop() { // TODO Auto-generated method stub } @Override public void method1() { // TODO Auto-generated method stub } @Override public void method2() { // TODO Auto-generated method stub } } //************************************ interface AA{ void method1(); } interface BB{ void method2(); } interface CC extends AA,BB{ }
Interface polymorphism
package com.atguigu.java1; /* * Use of interfaces * 1.The interface also meets polymorphism * 2.Interface actually defines a specification * 3.Experience interface oriented programming in development! * */ public class USBTest { public static void main(String[] args) { Computer com = new Computer(); //1. Create a non anonymous object of the non anonymous implementation class of the interface Flash flash = new Flash(); com.transferData(flash); //2. Create the anonymous object of the non anonymous implementation class of the interface com.transferData(new Printer()); //3. Create a non anonymous object of the anonymous implementation class of the interface USB phone = new USB(){ @Override public void start() { System.out.println("The phone starts working"); } @Override public void stop() { System.out.println("End of mobile phone work"); } }; com.transferData(phone); //4. Create the anonymous object of the anonymous implementation class of the interface com.transferData(new USB(){ @Override public void start() { System.out.println("mp3 start-up"); } @Override public void stop() { System.out.println("mp3 power cut-off"); } }); } } class Computer{ public void transferData(USB usb){//USB usb = new Flash(); usb.start(); System.out.println("Details of specific transmission data"); usb.stop(); } } interface USB{ //Constant: defines the length, width, maximum and minimum transmission speed, etc void start(); void stop(); } class Flash implements USB{ @Override public void start() { System.out.println("U Disc opening operation"); } @Override public void stop() { System.out.println("U Disk end work"); } } class Printer implements USB{ @Override public void start() { System.out.println("Printer on"); } @Override public void stop() { System.out.println("Printer finished working"); } }
Use of inner classes
package com.atguigu.java2; /* * Inner member of class 5: inner class * 1. Java Class A is allowed to be declared in another class B, then class A is the inner class, and class B is called the outer class * * 2.Classification of internal classes: member internal classes (static and non static) vs local internal classes (within methods, code blocks and constructors) * * 3.Member inner class: * On the one hand, as a member of an external class: * >Calling the structure of an external class * >Can be modified by static * >It can be modified by four different permissions * * On the other hand, as a class: * > Properties, methods, constructors, etc. can be defined within a class * > Can be modified by final to indicate that this class cannot be inherited. The implication is that you can inherit without using final * > Can be modified by abstract * * * 4.Focus on the following three issues * 4.1 How to instantiate an object of a member's inner class * 4.2 How to distinguish the structure of calling external classes in member internal classes * 4.3 See InnerClassTest1.java for the use of local internal classes during development * */ public class InnerClassTest { public static void main(String[] args) { //Create a Dog instance (static member inner class): Person.Dog dog = new Person.Dog(); dog.show(); //Create a Bird instance (non static member inner class): // Person.Bird bird = new Person.Bird();// FALSE Person p = new Person(); Person.Bird bird = p.new Bird(); bird.sing(); System.out.println(); bird.display("Oriole"); } } class Person{ String name = "Xiao Ming"; int age; public void eat(){ System.out.println("Man: eat"); } //Static member inner class static class Dog{ String name; int age; public void show(){ System.out.println("Carla is a dog"); // eat(); } } //Non static member inner class class Bird{ String name = "cuckoo"; public Bird(){ } public void sing(){ System.out.println("I am a little bird"); Person.this.eat();//Calling non static properties of an external class eat(); System.out.println(age); } public void display(String name){ System.out.println(name);//Formal parameters of method System.out.println(this.name);//Properties of inner classes System.out.println(Person.this.name);//Properties of external classes } } public void method(){ //Local inner class class AA{ } } { //Local inner class class BB{ } } public Person(){ //Local inner class class CC{ } } }
exception handling
Advanced (call api to complete multiple function applications)
Multithreading
Create 4 kinds and solve 3 kinds of security problems
Opening 360 is a process. Scanning with 360 at the same time, clearing garbage and checking files is multithreading
Each thread has an independent stack and program counter, and multiple threads share a method area and heap
package atguigu.java; /** * The creation of multithreading, method 1: inherit from the Thread class * 1. Create a subclass that inherits from the Thread class * 2. Override run() -- > of the Thread class to declare the operation performed by this Thread in run() * 3. An object that creates a subclass of the Thread class * 4. start() is called from this object * <p> * Example: traverse all even numbers within 100 * * @author shkstart * @create 2019-02-13 11:46 am */ //1. Create a subclass inherited from Thread class class MyThread extends Thread { //2. Override run() of Thread class @Override public void run() { for (int i = 0; i < 100; i++) { if(i % 2 == 0){ System.out.println(Thread.currentThread().getName() + ":" + i); } } } } public class ThreadTest { public static void main(String[] args) { //3. Create objects of subclasses of Thread class MyThread t1 = new MyThread(); //4. Call start() through this object: ① start the current thread ② call run() of the current thread t1.start(); //Problem 1: we can't start a thread by calling run() directly. // t1.run(); //Problem 2: start another thread and traverse the even number within 100. No, you can also let the thread that has started () execute. IllegalThreadStateException will be reported // t1.start(); //We need to recreate a thread object MyThread t2 = new MyThread(); t2.start(); //The following operations are still performed in the main thread. for (int i = 0; i < 100; i++) { if(i % 2 == 0){ System.out.println(Thread.currentThread().getName() + ":" + i + "***********main()************"); } } } }
## Methods of anonymous subclasses new Thread(){ public void run(){ for (int j=0; j<=100;j++){ if(j%2==1){ System.out.println(Thread.currentThread().getName()+"***"+j); } }}}.start();
If getName()The class is Thread Can be called directly getName()Implement thread viewing The other is Thread.currentThread().setName("Main thread"); 1.Call in object Mythread2 thread2 =new Mythread2(); thread2.setName("Thread two"); 2.main Call in Thread.currentThread().setName("Main thread"); System.out.println(Thread.currentThread().getPriority()); 3.run()Call in method body public void run(){ while (true){ if(tickets>0){ System.out.println(getName()+":The ticket number is:"+tickets);
Implementation summary
1. Inheritance of thread class 2 Interface using Runnable
//Method I: class Window extends Thread{ private static int tickets =100; public void run(){ while (true){ if(tickets>0){ System.out.println(getName()+":The ticket number is:"+tickets); tickets--;} else{ break; } } } } //use Window w1 = new Window(); Window w2 = new Window(); Window w3 = new Window(); w1.setName("Window 1"); w2.setName("Window 2"); w3.setName("Window 3"); System.out.println(w1.getPriority()); System.out.println(w2.getPriority()); System.out.println(w3.getPriority()); w1.start(); w2.start(); w3.start(); //Method II class Run1 implements Runnable{ private int tickets =100; public void run(){ while (true){ if (tickets>0){ System.out.println(Thread.currentThread().getName()+"Ticket sales:"+tickets); tickets--; }else{ break; } } } } Run1 r =new Run1(); Thread t1 = new Thread(r); Thread t2 = new Thread(r); Thread t3 = new Thread(r); t1.setName("Window 1:"); t2.setName("Window 2:"); t3.setName("Window 3:"); //Method 3 implementation of Callable interface package com.linyang; import java.util.concurrent.Callable; import java.util.concurrent.ExecutionException; import java.util.concurrent.FutureTask; /** *The third way to create a thread is to implement the Callable interface--- JDK 5.0 NEW * How to understand that the way to implement the Callable interface to create multithreads is more powerful than the way to implement the Runnable interface to create multithreads? * 1. call()Can have a return value. * 2. call()Exceptions can be thrown and captured by external operations to obtain exception information * 3. Callable Is generic * * realization * 1.Create an implementation class that implements Callable * 2.Implement the call method and declare the operation to be performed by this thread in call() * 3.Create an object of the Callable interface implementation class * 4.The object of this Callable interface implementation class is passed to the FutureTask constructor as an object to create FutureTask * 5.Pass the FutureTask object as a parameter to the constructor of the Thread class, create the Thread object, and call start() * 6.Gets the return value of the call method in the Callable * 7.get()The return value is the return value of call() overridden by the FutureTask constructor parameter Callable implementation class * / class NumThread implements Callable{ @Override public Object call() throws Exception { int sum=0; for (int i =1;i<=100;i++){ if(i%2==0){ System.out.println(i); sum+=i; } } return sum; } } public class ThreadNew { public static void main(String [] args){ NumThread numThread = new NumThread(); FutureTask futureTask = new FutureTask(numThread); new Thread(futureTask).start(); try { Object sum = futureTask.get(); System.out.println(sum); } catch (InterruptedException e) { e.printStackTrace(); } catch (ExecutionException e) { e.printStackTrace(); } } } Method 4: thread pool package com.linyang; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.concurrent.ThreadPoolExecutor; /** * @author linyang * @create 2021-08-03-11:11 */ class NumberThread implements Runnable{ @Override public void run() { for(int i = 0;i <= 100;i++){ if(i % 2 == 0){ System.out.println(Thread.currentThread().getName() + ": " + i); } } } } class NumberThread1 implements Runnable{ @Override public void run() { for(int i = 0;i <= 100;i++){ if(i % 2 != 0){ System.out.println(Thread.currentThread().getName() + ": " + i); } } } } public class ThreadPool { public static void main(String [] args){ //1. Provide a thread pool with a specified number of threads (set the properties of the thread pool) //2. Execute the operation of the specified thread. You need to provide an object that implements the Runnable interface or the Callable interface implementation class //3. Close the connection pool ExecutorService service = Executors.newFixedThreadPool(10); ThreadPoolExecutor service1 = (ThreadPoolExecutor) service; System.out.println(service.getClass()); service1.setCorePoolSize(15); // service1.setKeepAliveTime(10); service.execute(new NumberThread());//Suitable for Runnable service.execute(new NumberThread()); // service.submit(Callable callable);// Suitable for callable service.shutdown(); } }
Process lifecycle
Thread safety
1. Synchronization mechanism
Each thread has an independent stack and program counter, and multiple threads share a method area and heap
public static can ensure that multiple subclasses share the same data
//1 synchronization code block public void run(){ while (true){ //show(); synchronized (this){ if (tickets>0){ try{Thread.sleep(100);} catch (InterruptedException e){ e.printStackTrace();} System.out.println(Thread.currentThread().getName()+"Ticket sales:"+tickets); tickets--; }else{ break; } }} } //2 synchronization method to solve the insecurity of Runnable multithreading private synchronized void show(){//Synchronization monitor: this can not be written if (tickets>0){ try{Thread.sleep(100);} catch (InterruptedException e){ e.printStackTrace();} System.out.println(Thread.currentThread().getName()+"Ticket sales:"+tickets); tickets--; }else{ break; } } //3. For the inherited Thread class, multiple objects will be created in the end, so the same lock cannot be guaranteed private synchronized void show(){//Synchronization monitor: this can not be written You can add one static Indicates unique. //3.lock lock class Run2 implements Runnable{ private int tickets =100; private ReentrantLock lock =new ReentrantLock(); public void run(){ while (true){ try{ lock.lock(); if (tickets>0){ try{Thread.sleep(100);} catch (InterruptedException e){ e.printStackTrace();} System.out.println(Thread.currentThread().getName()+"Ticket sales:"+tickets); tickets--; }else{ break; } }finally { lock.unlock();// } } } }
deadlock
When there are multiple locks, one takes a first and then b, and the other takes b first and then A. they occupy resources with each other and wait for each other to release, a deadlock will occur.
Thread communication
Common Java classes
String memory
String method
==Attention==
- The string is immutable, and the string is unique in the constant pool. As long as str is different, it is represented by different addresses.
When s2 operates on s1, s1 is constant and s2 opens up space separately - String substring(int beginIndex, int endIndex) -- open left and close right
- String is strictly case sensitive
- String replace(char oldChar, char newChar) all STRs are replaced
/* int length(): The length of the returned string: return value length char charAt(int index): Returns the character at an index. return value[index] boolean isEmpty(): Judge whether it is an empty string: return value length == 0 String toLowerCase(): Converts all characters in a String to lowercase using the default locale String toUpperCase(): Converts all characters in a String to uppercase using the default locale String trim(): Returns a copy of a string, ignoring leading and trailing whitespace boolean equals(Object obj): Compare whether the contents of the string are the same boolean equalsIgnoreCase(String anotherString): Similar to the equals method, case is ignored String concat(String str): Concatenates the specified string to the end of this string. Equivalent to "+" int compareTo(String anotherString): Compare the size of two strings, compare the size of the ascll code of a single character from left to right, and then s1-s2. The return value is positive and negative, only To find the comparison is not 0, stop the search String substring(int beginIndex): Returns a new string, which is the last substring of the string intercepted from beginIndex. String substring(int beginIndex, int endIndex) : Returns a new string, which is a substring intercepted from beginIndex to endindex (not included). */ @Test public void test2() { String s1 = "HelloWorld"; String s2 = "helloworld"; System.out.println(s1.equals(s2)); System.out.println(s1.equalsIgnoreCase(s2)); String s3 = "abc"; String s4 = s3.concat("def"); System.out.println(s4); String s5 = "abc"; String s6 = new String("abe"); System.out.println(s5.compareTo(s6));//Involving string sorting String s7 = "Beijing Shang Silicon Valley Education"; String s8 = s7.substring(2); System.out.println(s7); System.out.println(s8); String s9 = s7.substring(2, 5); System.out.println(s9); } @Test public void test1() { String s1 = "HelloWorld"; System.out.println(s1.length()); System.out.println(s1.charAt(0)); System.out.println(s1.charAt(9)); // System.out.println(s1.charAt(10)); // s1 = ""; System.out.println(s1.isEmpty()); String s2 = s1.toLowerCase(); System.out.println(s1);//s1 is immutable and remains the original string System.out.println(s2);//String after lowercase String s3 = " he llo world "; String s4 = s3.trim(); System.out.println("-----" + s3 + "-----"); System.out.println("-----" + s4 + "-----"); } }
boolean endsWith(String suffix): Tests whether this string ends with the specified suffix boolean startsWith(String prefix): Tests whether this string starts with the specified prefix boolean startsWith(String prefix, int toffset): Tests whether the substring of this string starting from the specified index starts with the specified prefix boolean contains(CharSequence s): If and only if this string contains the specified char Value sequence, return true int indexOf(String str): Returns the index of the specified substring at the first occurrence in this string int indexOf(String str, int fromIndex): Returns the index of the specified substring at the first occurrence in this string, starting from the specified index int lastIndexOf(String str): Returns the index of the rightmost occurrence of the specified substring in this string int lastIndexOf(String str, int fromIndex): Returns the index of the last occurrence of the specified substring in this string, and searches backwards from the specified index Note: indexOf and lastIndexOf Methods are returned if not found-1 */ @Test public void test3(){ String str1 = "hellowworld"; boolean b1 = str1.endsWith("rld"); System.out.println(b1); boolean b2 = str1.startsWith("He"); System.out.println(b2); boolean b3 = str1.startsWith("ll",2); System.out.println(b3); String str2 = "wor"; System.out.println(str1.contains(str2)); System.out.println(str1.indexOf("lol")); System.out.println(str1.indexOf("lo",5)); String str3 = "hellorworld"; System.out.println(str3.lastIndexOf("or")); System.out.println(str3.lastIndexOf("or",6)); //When does indexOf(str) and lastIndexOf(str) return the same value? //Case 1: there is a unique str. Case 2: STR does not exist }
Replace: String replace(char oldChar, char newChar): Returns a new string by using newChar Replace all occurrences in this string oldChar Got it. String replace(CharSequence target, CharSequence replacement): Replaces all substrings of this string that match the literal target sequence with the specified literal replacement sequence. String replaceAll(String regex, String replacement): Use the given replacement Replace this string with all substrings that match the given regular expression. String replaceFirst(String regex, String replacement): Use the given replacement Replace this string to match the first substring of the given regular expression. matching: boolean matches(String regex): Tells whether this string matches the given regular expression. section: String[] split(String regex): Splits the string based on the match of the given regular expression. String[] split(String regex, int limit): Splits this string based on matching the given regular expression, up to limit If it exceeds, all the rest will be placed in the last element. */ @Test public void test4(){ String str1 = "Beijing Shang Silicon Valley Education Beijing"; String str2 = str1.replace('north', 'east'); System.out.println(str1); System.out.println(str2); String str3 = str1.replace("Beijing", "Shanghai"); System.out.println(str3); System.out.println("*************************"); String str = "12hello34world5java7891mysql456"; //Replace the number in the string with,, and remove it if there are at the beginning and end of the result String string = str.replaceAll("\\d+", ",").replaceAll("^,|,$", ""); System.out.println(string); System.out.println("*************************"); str = "12345"; //Judge whether all str strings are composed of numbers, that is, 1-n numbers boolean matches = str.matches("\\d+"); System.out.println(matches); String tel = "0571-4534289"; //Judge whether this is a fixed line telephone in Hangzhou boolean result = tel.matches("0571-\\d{7,8}"); System.out.println(result); System.out.println("*************************"); str = "hello|world|java"; String[] strs = str.split("\\|"); for (int i = 0; i < strs.length; i++) { System.out.println(strs[i]); } System.out.println(); str2 = "hello.world.java"; String[] strs2 = str2.split("\\."); for (int i = 0; i < strs2.length; i++) { System.out.println(strs2[i]); } }
As long as one of them is a variable, the result is equivalent to a new process (in the heap), and then find str in the constant pool
String exercise
StringBuffer,StringBuilder
/* StringBuffer Common methods of: StringBuffer append(xxx): Many append() methods are provided for string splicing StringBuffer delete(int start,int end): Delete the contents of the specified location StringBuffer replace(int start, int end, String str): Replace the [start,end) position with str StringBuffer insert(int offset, xxx): Insert xxx at the specified location StringBuffer reverse() : Reverses the current character sequence public int indexOf(String str) public String substring(int start,int end):Returns a substring of the left closed right open interval from start to end public int length() public char charAt(int n ) public void setCharAt(int n ,char ch) Summary: Add: append(xxx) Delete: delete(int start,int end) Change: setcharat (int n, char CH) / replace (int start, int end, string STR) Query: charAt(int n) Insert: insert(int offset, xxx) Length: length(); *Traversal: for() + charAt() / toString() */ @Test public void test2(){ StringBuffer s1 = new StringBuffer("abc"); s1.append(1); s1.append('1'); System.out.println(s1); // s1.delete(2,4); // s1.replace(2,4,"hello"); // s1.insert(2,false); // s1.reverse(); String s2 = s1.substring(1, 3); System.out.println(s1); System.out.println(s1.length()); System.out.println(s2); }
Date
470, 480-488 no
long time = System.currentTimeMillis(); //Returns the time difference in milliseconds between the current time and 0:0:0:0 on January 1, 1970. //This is called a timestamp System.out.println(time);
Java comparator
492-493 didn't understand
System, Math class
Enumeration class & annotation
because Season The constructor is private,So pass new Season()You can't use a constructor, so there is another one defined in it public Multiple objects created in main Pass in function Season.SPRING Method call object. //Custom enumeration class class Season{ //1. Declare the attribute of Season object: private final modifier private final String seasonName; private final String seasonDesc; //2. Privatize the constructor of the class and assign a value to the object attribute private Season(String seasonName,String seasonDesc){ this.seasonName = seasonName; this.seasonDesc = seasonDesc; } //3. Provide multiple objects of the current enumeration class: public static final public static final Season SPRING = new Season("spring","in the warm spring , flowers are coming out with a rush"); public static final Season SUMMER = new Season("summer","Summer heat"); public static final Season AUTUMN = new Season("autumn","fresh autumn weather"); public static final Season WINTER = new Season("winter","a world of ice and snow"); //4. Other requirements 1: get the properties of enumeration objects public String getSeasonName() { return seasonName; } public String getSeasonDesc() { return seasonDesc; } //4. Other claims 1: toString() @Override public String toString() { return "Season{" + "seasonName='" + seasonName + '\'' + ", seasonDesc='" + seasonDesc + '\'' + '}'; } }
emum Season{}
package com.atguigu.java; /** * Define enumeration classes using enum keyword * Note: the defined enumeration class inherits from Java. Net by default Lang. enum class * * @author shkstart * @create 2019 10:35 am */ public class SeasonTest1 { public static void main(String[] args) { Season1 summer = Season1.SUMMER; //toString(): returns the name of the enumeration class object System.out.println(summer.toString()); // System.out.println(Season1.class.getSuperclass()); System.out.println("****************"); //values(): returns an array of all enumeration class objects Season1[] values = Season1.values(); for(int i = 0;i < values.length;i++){ System.out.println(values[i]); values[i].show(); } System.out.println("****************"); Thread.State[] values1 = Thread.State.values(); for (int i = 0; i < values1.length; i++) { System.out.println(values1[i]); } //valueOf(String objName): returns the object whose object name is objName in the enumeration class. Season1 winter = Season1.valueOf("WINTER"); //If there is no enumeration class object of objName, throw an exception: IllegalArgumentException // Season1 winter = Season1.valueOf("WINTER1"); System.out.println(winter); winter.show(); } } interface Info{ void show(); } //Enum classes using enum keyword enum Season1 implements Info{ //1. Provide the object of the current enumeration class. Multiple objects are separated by "," and the end object is ";" end SPRING("spring","in the warm spring , flowers are coming out with a rush"){ @Override public void show() { System.out.println("Where is spring?"); } }, SUMMER("summer","Summer heat"){ @Override public void show() { System.out.println("Ningxia"); } }, AUTUMN("autumn","fresh autumn weather"){ @Override public void show() { System.out.println("Autumn doesn't come back"); } }, WINTER("winter","a world of ice and snow"){ @Override public void show() { System.out.println("About winter"); } }; //2. Declare the attribute of Season object: private final modifier private final String seasonName; private final String seasonDesc; //2. Privatize the constructor of the class and assign a value to the object attribute private Season1(String seasonName,String seasonDesc){ this.seasonName = seasonName; this.seasonDesc = seasonDesc; } //4. Other requirements 1: get the properties of enumeration objects public String getSeasonName() { return seasonName; } public String getSeasonDesc() { return seasonDesc; } // //4. Other claims 1: toString() is provided // // @Override // public String toString() { // return "Season1{" + // "seasonName='" + seasonName + '\'' + // ", seasonDesc='" + seasonDesc + '\'' + // '}'; // } // @Override // public void show() { // System.out.println("this is a season"); // } }
annotation
/** * Use of annotations * * 1. Understanding Annotation: * ① jdk 5.0 New features * * ② Annotation In fact, they are special tags in the code. These tags can be read and processed during compilation, class loading and runtime. By using Annotation, * Programmers can embed some supplementary information in the source file without changing the original logic. * * ③In Java se, annotations are used for simple purposes, such as marking outdated functions, ignoring warnings, etc. In Java EE / Android * Annotations play a more important role, such as configuring any aspect of the application, instead of the cumbersome legacy of the older versions of Java EE * Code and XML configuration, etc. * * 2. Annocation Use examples of * Example 1: generate document related annotations * Example 2: format checking at compile time (three basic annotations built into JDK) @Override: The annotation can only be used for overriding parent class methods @Deprecated: Used to indicate that the modified element (class, method, etc.) is obsolete. Usually because the modified structure is dangerous or there is a better choice @SuppressWarnings: Suppress compiler warnings * Example 3: track code dependencies and implement the function of replacing configuration files * * 3. How to customize annotations: refer to the @ SuppressWarnings definition * ① Annotation declared as: @ interface * ② Internally defined members, usually represented by value * ③ You can specify the default value of the member, which is defined by default * ④ If the custom annotation has no members, it indicates that it is an identification function. If the annotation has members, you need to specify the value of the members when using the annotation. Custom annotations must be accompanied by an annotated information processing process (using reflection) to make sense. The user-defined annotation will indicate two meta annotations: Retention and Target 4. jdk Four meta annotations provided Meta annotation: an annotation that explains existing annotations Retention: Specifies the lifecycle of the decorated Annotation: SOURCE\CLASS (default behavior) \ RUNTIME Only annotations declared as RUNTIME lifecycles can be obtained through reflection. Target:Used to specify which program elements can be modified by the modified Annotation *******The frequency of occurrence is low******* Documented:Indicates that the modified annotation is retained when it is parsed by javadoc. Inherited:The Annotation decorated by it will be inherited. 5.Obtain annotation information through reflection - explain the system when reflecting the content 6. jdk 8 New features of annotations in: repeatable annotations, type annotations 6.1 Repeatable annotation: ① declare @ repeatable on MyAnnotation, and the member value is myannotations class ② MyAnnotation Meta annotations such as Target and Retention are the same as MyAnnotations. 6.2 Type notes: ElementType.TYPE_PARAMETER Indicates that the annotation can be written in the declaration statement of type variables (e.g. generic declaration). ElementType.TYPE_USE Indicates that the annotation can be written in any statement using the type. * * @author shkstart * @create 2019 11:37 am */ public class AnnotationTest { public static void main(String[] args) { Person p = new Student(); p.walk(); Date date = new Date(2020, 10, 11); System.out.println(date); @SuppressWarnings("unused") int num = 10; // System.out.println(num); @SuppressWarnings({ "unused", "rawtypes" }) ArrayList list = new ArrayList(); } @Test public void testGetAnnotation(){ Class clazz = Student.class; Annotation[] annotations = clazz.getAnnotations(); for(int i = 0;i < annotations.length;i++){ System.out.println(annotations[i]); } } } //Before jdk 8: //@MyAnnotations({@MyAnnotation(value="hi"),@MyAnnotation(value="hi")}) @MyAnnotation(value="hi") @MyAnnotation(value="abc") class Person{ private String name; private int age; public Person() { } @MyAnnotation public Person(String name, int age) { this.name = name; this.age = age; } @MyAnnotation public void walk(){ System.out.println("People walk"); } public void eat(){ System.out.println("People eat"); } } interface Info{ void show(); } class Student extends Person implements Info{ @Override public void walk() { System.out.println("Students walk"); } public void show() { } } class Generic<@MyAnnotation T>{ public void show() throws @MyAnnotation RuntimeException{ ArrayList<@MyAnnotation String> list = new ArrayList<>(); int num = (@MyAnnotation int) 10L; } }
Java collections
Collection
Collection should take into account both list and set, so some methods list and set are available separately, as shown below.
Call of New ArrayList() and traversal of iterator
public class Test3 { public static void main(String [] args){ Collection coll =new ArrayList();//Store objects coll.add("AA"); coll.add(124);//Here, integers are automatically boxed into the Integer class coll.add(new Object()); coll.add(new String("Tom")); Collection coll1 =new ArrayList();//Store objects coll1.add("cc"); coll.addAll(coll1); System.out.println(coll.size()); System.out.println(coll);//[AA, 124, java.lang.Object@1540e19d, cc] // When adding data obj to the object of the implementation class of the Collection interface, the class of obj is required to override equals() //1.contains(Object obj): judge whether the current set contains obj //When judging, we will call equals() of the class where the obj object is located. Then, here we also investigate equal. When it is not rewritten, equal is' = = ', and after rewriting, the comparison is the entity of the class System.out.println(coll.contains(new String("Tom")));//true because String overrides equal // System.out.println(coll.contains(new Person("Jerry",20)));//false, because no rewriting is equivalent to comparing addresses, so false System.out.println(coll.contains("124"));//"124" is a String class, and the above is the Integer class, so false is returned System.out.println(coll.containsAll(coll1)); // coll.clear(); System.out.println(coll.isEmpty()); //2.boolean remove(Object obj) can only be removed if there are contains Collection coll3 =new ArrayList(); coll3.add(1); coll3.add(2); coll3.add(3); coll3.add(4); Collection coll4 =new ArrayList(); coll4.add(1); coll4.add(2); // coll3.remove(3);// Take 3, compare it in cool3, and then delete it // coll3.removeAll(coll4);// The difference set, one at a time from coo4, is deleted coll3.retainAll(coll4);//Intersection output [1,2] System.out.println(coll3); //3.equals() because array List () is ordered, so the sequence of the two sets should be the same coll3.equals(coll4); //4. System.out.println(coll3.hashCode()); //5 set - > array Object [] arr=coll4.toArray(); System.out.println(Arrays.toString(arr)); //6 List<String> list =Arrays.asList(new String[]{"1","2b"}); System.out.println(list);//[1, 2b] List<int[]> list2 =Arrays.asList(new int []{1,2}); System.out.println(list2);//[[I@677327b6] //7 Iterator iterator=coll3.iterator(); Use the iterator () method to return the iterator object System.out.println(coll3);//[1,2] Iterator iterator=coll3.iterator(); // System.out.println(iterator.next());//1 // System.out.println(iterator.next());//2 while(iterator.hasNext()){ System.out.println(iterator.next());//1 Object obj =iterator.next();//2 if(obj.equals("tom")){ iterator.remove(); } } } }
List interface (three)
From the perspective of searching, the complexity O(1) of ArrayList is directly located by subscript, but it is O (n) when deleting, and the subsequent data needs to be moved forward
O (N) for Linkedlist lookup and O (1) for deletion
Note that all objects stored in the collection are objects, so list should be used when deleting Remove (new integer (15)), which is a normal list for string remove(“a”)
void add(int index, Object ele):stay index Position insertion ele element boolean addAll(int index, Collection eles):from index Position start will eles Add all elements in Object get(int index):Get specified index Element of location int indexOf(Object obj):return obj First occurrence in the collection int lastIndexOf(Object obj):return obj The last occurrence in the current collection Object remove(int index):Remove assignment index The element of the location and returns this element remove(1):The data with index 1 is deleted remove(new Integer(1))Deleted is object Object set(int index, Object ele):Setting assignment index The element of the location is ele List subList(int fromIndex, int toIndex):Return from fromIndex reach toIndex Subset of locations Summary: common methods Add: add(Object obj) Delete: remove(int index) / remove(Object obj) about string It's normal list.remove("a"); list.remove(new Integer(15)); Change: set(int index, Object ele) Check: get(int index) Insert: add(int index, Object ele) Length: size()Returns the number of storage elements, not the underlying new(10)10 empty arrays of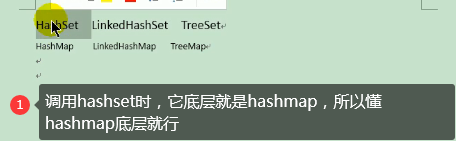 Traversal:① Iterator Iterator pattern ② enhance for loop ③ Ordinary cycle //Mode 1: Iterator iterator mode Iterator iterator = list.iterator(); while(iterator.hasNext()){ System.out.println(iterator.next()); } System.out.println("***************"); //Mode 2: enhanced for loop for(Object obj : list){ System.out.println(obj); } System.out.println("***************"); //Mode 3: normal for loop for(int i = 0;i < list.size();i++){ System.out.println(list.get(i)); } public void test2(){ ArrayList list = new ArrayList(); list.add(123); list.add(456); list.add("AA"); list.add(new Person("Tom",12)); list.add(456); //int indexOf(Object obj): returns the position where obj first appears in the collection. If it does not exist, - 1 int index = list.indexOf(4567); System.out.println(index); //int lastIndexOf(Object obj): returns the last occurrence of obj in the current collection. If it does not exist, - 1 System.out.println(list.lastIndexOf(456)); //Object remove(int index): removes the element at the specified index position and returns this element Object obj = list.remove(0); System.out.println(obj); System.out.println(list); //Object set(int index, Object ele): sets the element at the specified index position to ele list.set(1,"CC"); System.out.println(list); //List subList(int fromIndex, int toIndex): returns a subset of the left closed right open interval from fromIndex to toIndex List subList = list.subList(2, 4); System.out.println(subList); System.out.println(list);
Set
The hash value is calculated by calling the hashcode method of the class The hash values are different, but the values obtained after modulo are the same and should be placed in the same position
- Hash values for different values may be the same
- A data can only have one hash value
Map
Implementation class corresponding to map interface
V Map Methods defined in: Add, delete and modify: Object put(Object key,Object value): Will specify key-value Add to(Or modify)current map Object void putAll(Map m):take m All in key-value Save to current map in Object remove(Object key): Remove assignment key of key-value Yes, and return value void clear(): Clear current map All data in Operation of element query: Object get(Object key): Get specified key Corresponding value boolean containsKey(Object key): Include the specified key boolean containsValue(Object value): Include the specified value int size(): return map in key-value Number of pairs boolean isEmpty(): Judge current map Is it empty boolean equals(Object obj): Judge current map And parameter objects obj Are they equal Method of metaview operation: Set keySet(): Return all key Constitutive Set aggregate Collection values(): Return all value Constitutive Collection aggregate Set entrySet(): Return all key-value To constitute Set aggregate *Summary: common methods: * add to: put(Object key,Object value) * Delete: remove(Object key) * Modification: put(Object key,Object value) * Query: get(Object key) * Length: size() * Traversal: keySet() / values() / entrySet() * * * @author shkstart * @create 2019 11 a.m:15 */ public class MapTest { /* Method of metaview operation: Set keySet(): Returns the Set set composed of all key s Collection values(): Returns a Collection of all value s Set entrySet(): Returns the Set set composed of all key value pairs */ @Test public void test5(){ Map map = new HashMap(); map.put("AA",123); map.put(45,1234); map.put("BB",56); //Traverse all key sets: keySet() Set set = map.keySet(); Iterator iterator = set.iterator(); while(iterator.hasNext()){ System.out.println(iterator.next()); } System.out.println(); //Traverse all value sets: values() Collection values = map.values(); for(Object obj : values){ System.out.println(obj); } System.out.println(); //Traverse all key values //Method 1: entrySet() Set entrySet = map.entrySet(); Iterator iterator1 = entrySet.iterator(); while (iterator1.hasNext()){ Object obj = iterator1.next(); //All elements in the entry set collection are entries Map.Entry entry = (Map.Entry) obj; System.out.println(entry.getKey() + "---->" + entry.getValue()); } System.out.println(); //Mode 2: Set keySet = map.keySet(); Iterator iterator2 = keySet.iterator(); while(iterator2.hasNext()){ Object key = iterator2.next(); Object value = map.get(key); System.out.println(key + "=====" + value); } } /* Operation of element query: Object get(Object key): Gets the value corresponding to the specified key boolean containsKey(Object key): Whether to include the specified key boolean containsValue(Object value): Whether to include the specified value int size(): Returns the number of key value pairs in the map boolean isEmpty(): Judge whether the current map is empty boolean equals(Object obj): Judge whether the current map and parameter object obj are equal */ @Test public void test4(){ Map map = new HashMap(); map.put("AA",123); map.put(45,123); map.put("BB",56); // Object get(Object key) System.out.println(map.get(45)); //containsKey(Object key) boolean isExist = map.containsKey("BB"); System.out.println(isExist); isExist = map.containsValue(123); System.out.println(isExist); map.clear(); System.out.println(map.isEmpty()); } /* Add, delete and modify: Object put(Object key,Object value): Add (or modify) the specified key value to the current map object void putAll(Map m):Store all key value pairs in m in the current map Object remove(Object key): Remove the key value pair of the specified key and return value void clear(): Clear all data in the current map */ @Test public void test3(){ Map map = new HashMap(); //add to map.put("AA",123); map.put(45,123); map.put("BB",56); //modify map.put("AA",87); System.out.println(map); Map map1 = new HashMap(); map1.put("CC",123); map1.put("DD",123); map.putAll(map1); System.out.println(map); //remove(Object key) Object value = map.remove("CC"); System.out.println(value); System.out.println(map); //clear() map.clear();//Different from map = null operation System.out.println(map.size()); System.out.println(map); } @Test public void test2(){ Map map = new HashMap(); map = new LinkedHashMap(); map.put(123,"AA"); map.put(345,"BB"); map.put(12,"CC"); System.out.println(map); } @Test public void test1(){ Map map = new HashMap(); // map = new Hashtable(); map.put(null,123); } }
Collections, Arrays (with s) is the interface for the tool class coolaction to create collections
Because the thread of ArrayList is not safe, but we usually use it, so we use list 1 = collections Synchronizedlist (list) becomes safe
mport java.util.ArrayList; import java.util.Ar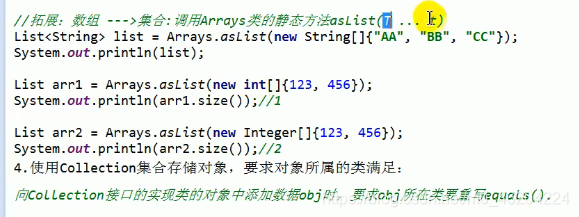 rays; import java.util.Collections; import java.util.List; /** * Collections:Tool classes for operating Collection and Map * * * Interview question: what is the difference between Collection and Collections? * * * @author shkstart * @create 2019 4:19 PM */ public class CollectionsTest { /* reverse(List): Reverse the order of elements in the List shuffle(List): Random sorting of List collection elements sort(List): Sorts the specified List collection elements in ascending order according to the natural order of the elements sort(List,Comparator): Sorts the List collection elements according to the order generated by the specified Comparator swap(List,int, int): Exchange the elements at i and j in the specified list collection Object max(Collection): Returns the largest element in a given set according to the natural order of the elements Object max(Collection,Comparator): Returns the largest element in a given collection in the order specified by the Comparator Object min(Collection) Object min(Collection,Comparator) int frequency(Collection,Object): Returns the number of occurrences of the specified element in the specified collection void copy(List dest,List src): Copy the contents of src to dest boolean replaceAll(List list,Object oldVal,Object newVal): Replace all old values of the List object with the new values */ @Test public void test2(){ List list = new ArrayList(); list.add(123); list.add(43); list.add(765); list.add(-97); list.add(0); //Exception: IndexOutOfBoundsException("Source does not fit in dest") // List dest = new ArrayList(); // Collections.copy(dest,list); //correct: List dest = Arrays.asList(new Object[list.size()]); System.out.println(dest.size());//list.size(); Collections.copy(dest,list); System.out.println(dest); /* Collections Class provides multiple synchronizedXxx() methods, This method can wrap the specified set into a thread synchronized set, which can solve the problem Thread safety in multithreaded concurrent access to collections */ //The returned list1 is the thread safe List List list1 = Collections.synchronizedList(list); } @Test public void test1(){ List list = new ArrayList(); list.add(123); list.add(43); list.add(765); list.add(765); list.add(765); list.add(-97); list.add(0); System.out.println(list); // Collections.reverse(list); // Collections.shuffle(list); // Collections.sort(list); // Collections.reverse(list); // Collections.swap(list,1,2); int frequency = Collections.frequency(list, 123); System.out.println(list); System.out.println(frequency); } }
review
Object is stored in the collection
data structure
generic paradigm
569-582 No
**
//Problem 1: unsafe type
//Problem 2: ClassCastException may occur during forced rotation
so introduction paradigm
The type of a generic type must be a class, not a basic data type.
- The reason why < > can be added is that when you create this method, you define the template public class ArrayList extensions abstractlist**
* Use of generics * 1.jdk 5.0 New features * * 2.Use generics in Collections: * The reason why we can add<>,The premise is that you define the template when you create this method public class ArrayList<E> extends AbstractList<E> * Summary: * ① Collection interface or collection class jdk5.0 It is modified to a structure with generics. * ② When instantiating a collection class, you can specify a specific generic type * ③ After specifying, when defining a class or interface in a collection class or interface, the location where the generic type of the class is used by the internal structure (such as method, constructor, attribute, etc.) is specified as the instantiated generic type. * For example: add(E e) --->After instantiation: add(Integer e) * ④ Note: the type of a generic type must be a class, not a basic data type. Where the basic data type needs to be used, replace it with a wrapper class * ⑤ If the generic type is not specified when instantiating. The default type is java.lang.Object Type. * * 3.How to customize generic structure: generic class and generic interface; Generic methods. see GenericTest1.java * //Problem 1: unsafe type //Problem 2: ClassCastException may occur during forced rotation //Using generics in Collections: take ArrayList as an example @Test public void test2(){ ArrayList<Integer> list = new ArrayList<Integer>(); list.add(78); list.add(87); //When compiling, type checking will be carried out to ensure the safety of data // list.add("Tom"); //Mode 1: // for(Integer score : list){ // //Strong rotation operation is avoided // int stuScore = score; // System.out.println(stuScore); // // } //Mode 2: Iterator<Integer> iterator = list.iterator(); while(iterator.hasNext()){ int stuScore = iterator.next(); System.out.println(stuScore); } } //Using generics in Collections: take HashMap as an example @Test public void test3(){ // Map<String,Integer> map = new HashMap<String,Integer>(); //jdk7 new feature: type inference Map<String,Integer> map = new HashMap<>(); map.put("Tom",87); map.put("Jerry",87); map.put("Jack",67); // map.put(123,"ABC"); //Nesting of generics Set<Map.Entry<String,Integer>> entry = map.entrySet(); Iterator<Map.Entry<String, Integer>> iterator = entry.iterator(); while(iterator.hasNext()){ Map.Entry<String, Integer> e = iterator.next(); String key = e.getKey(); Integer value = e.getValue(); System.out.println(key + "----" + value); } } }
IO stream
594-619 No
From the perspective of memory, see whether the data is input or output
public class Test6 { public static void main(String[] args) throws IOException { //1, Read in //1. Instantiation of file class File file = new File("E:\\LINYANG\\java_code\\workspace_idea\\JavaSenior\\day01\\src\\hello.txt");//"day09\\hello.txt" System.out.println(file.getAbsolutePath()); //2. Provide specific flow FileReader fr =new FileReader(file); //3 read from hard disk to memory (from the perspective of memory) //3.1 return one character read in. If the end of the file is reached, - 1 is returned // int data = fr.read(); // while (data!=-1){ // System.out.println(data); // System.out.print((char)data);// Call fr.read() once to read one data, so it needs to be called in a loop // data =fr.read(); // } //txt-------Helloworld123 //3.1 return the number of characters read into the cbuf array each time. If the end of the file is reached, - 1 is returned char [] cbuffer =new char[5]; int len; while ((len =fr.read(cbuffer))!=-1){ //System.out.print(len);5,5,3,-1 for(int i =0;i<len;i++){//For (int i = 0; I < CBuffer. Length; I + +) finally outputs Helloworld123ld, which takes five at a time and overwrites the original System.out.print(cbuffer[i]); } } //4. Close the stream, otherwise the memory leaks fr.close(); //2, Write data from memory to a file on the hard disk. // explain: // 1. For output operation, the corresponding File may not exist. No exception will be reported // 2. // If the File in the hard disk corresponding to File does not exist, this File will be automatically created during output. // If the File in the hard disk corresponding to File exists: // If the constructor used by the stream is: FileWriter(file,false) / FileWriter(file): overwrite the original file // If the constructor used by the stream is: FileWriter(file,true): add content to the original file File file1 =new File("hello1.txt"); System.out.println(file1.getAbsolutePath()); FileWriter fw = new FileWriter(file1,false); fw.write("I have a dream\n"); fw.write("you need a dream too"); fw.close(); FileReader fr1 =new FileReader(file1); int data=fr1.read(); while (data!=-1){ System.out.print((char)data); data = fr1.read(); } fr1.close(); // Test the use of FileInputStream and FileOutputStream // Conclusion: // 1. For text files (. txt,.java,.c,.cpp), use character stream processing // 2. For non text files (. jpg,.mp3,.mp4,.avi,.doc,.ppt,...), Using byte stream processing //3, Use 8-bit byte stream to read txt. If txt is all letters, it can be read out. If there is Chinese, it will be garbled System.out.println("******************************"); File srcFile = new File("11.png"); File destFile = new File("give the thumbs-up.jpg"); // FileInputStream fis = new FileInputStream(srcFile); FileOutputStream fos = new FileOutputStream(destFile); //Replication process byte[] buffer = new byte[5]; int len2; while((len2 = fis.read(buffer)) != -1){ fos.write(buffer,0,len2);//The number of writes per time is 0-len2, the first read is 5, and the last is len // System.out.println(new String(buffer,0,len)); } fis.close(); fos.close(); } }
Network programming
624-633 No
IP and port number
package com.atguigu.java1; import java.net.InetAddress; import java.net.UnknownHostException; /** * 1, There are two main problems in network programming: * 1.How to accurately locate one or more hosts on the network; Locate a specific application on the host * 2.How to transfer data reliably and efficiently after finding the host * * 2, Two elements in network programming: * 1.Corresponding question 1: IP and port number * 2.Corresponding question 2: provide network communication protocol: TCP/IP reference model (application layer, transport layer, network layer, physical + data link layer) * * * 3, Communication element 1: IP and port number * * 1. IP:Uniquely identifies the computer (communication entity) on the Internet * 2. Use the InetAddress class to represent IP in Java * 3. IP Classification: IPv4 and IPv6; World Wide Web and local area network * 4. Domain name: www.baidu.com com www.mi. com www.sina. com www.jd. com * www.vip.com * 5. Local loop address: 127.0 0.1 corresponds to localhost * * 6. How to instantiate InetAddress: two methods: getByName(String host) and getLocalHost() * Two common methods: getHostName() / getHostAddress() * * 7. Port number: the process running on the computer. * Requirement: different processes have different port numbers * Range: specified as a 16 bit integer 0 ~ 65535. * * 8. The combination of port number and IP address yields a network Socket: Socket */ public class InetAddressTest { public static void main(String[] args) { try { //File file = new File("hello.txt"); InetAddress inet1 = InetAddress.getByName("192.168.10.14"); System.out.println(inet1); InetAddress inet2 = InetAddress.getByName("www.atguigu.com"); System.out.println(inet2); InetAddress inet3 = InetAddress.getByName("127.0.0.1"); System.out.println(inet3); //Get local ip InetAddress inet4 = InetAddress.getLocalHost(); System.out.println(inet4); //getHostName() System.out.println(inet2.getHostName()); //getHostAddress() System.out.println(inet2.getHostAddress()); } catch (UnknownHostException e) { e.printStackTrace(); } } } ```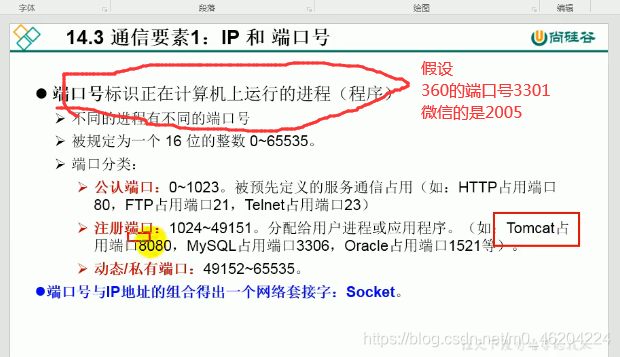 ### Network protocol 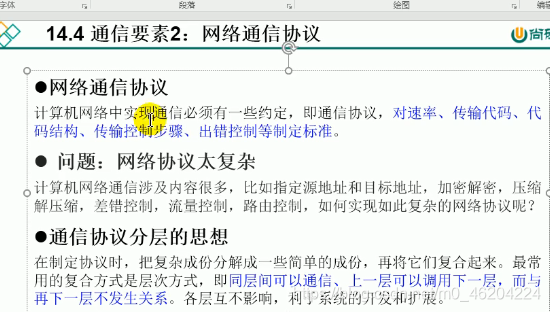 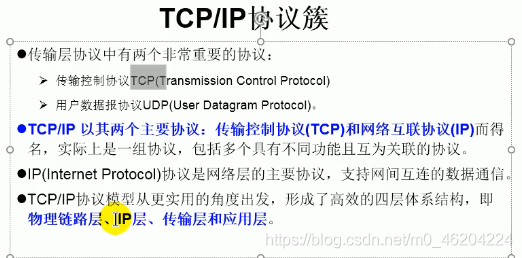 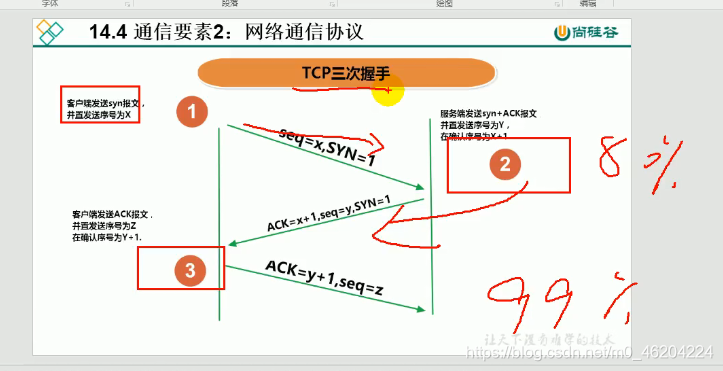 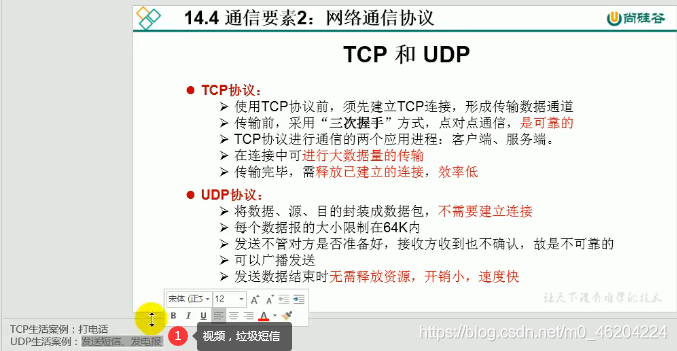 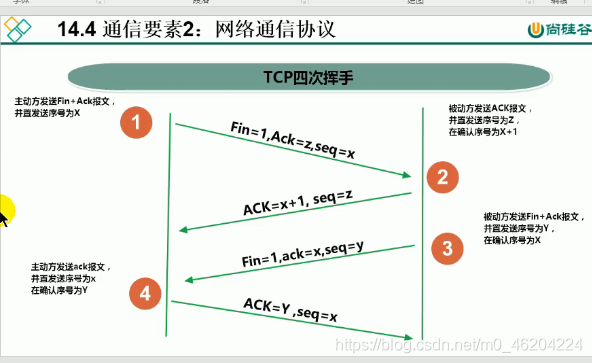 ```java package linyang; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.net.InetAddress; import java.net.ServerSocket; import java.net.Socket; /** * @author linyang * @create 2021-08-10-10:58 */ public class Test1 {public static void main(String [] args) throws IOException { InetAddress inet1 = InetAddress.getByName("192.168.10.14"); System.out.println(inet1); //1. Instantiate InetAddress //InetAddress inet2 = InetAddress.getByName("www.atguigu.com"); InetAddress inet2 = InetAddress.getLocalHost(); System.out.println(inet2);//www.atguigu.com/119.84.129.201 //2. Two common methods System.out.println(inet2.getHostName());//www.atguigu.com System.out.println(inet2.getHostAddress());//119.84.129.201 System.out.println("*************************************************************"); Test1 test=new Test1(); test.server(); //1. Create a Socket object to indicate the ip and port number of the server InetAddress inet =InetAddress.getLocalHost(); Socket socket = new Socket(inet,8899); //2. Obtain an output stream for outputting data OutputStream os = socket.getOutputStream(); //3. Write data os.write("Hello, I'm customer 1".getBytes()); //4. Closure of resources // os.close(); } public void server() throws IOException { //1. Create a ServerSocket on the server side and indicate its own port number ServerSocket ss = new ServerSocket(8899); //2. Call accept() to receive the socket from the client Socket socket1 = ss.accept(); //3. Get input stream InputStream is = socket1.getInputStream(); //4. Read the data in the input stream ByteArrayOutputStream baos = new ByteArrayOutputStream(); byte[] buffer =new byte[5]; int len; while ((len=is.read(buffer))!=-1){ baos.write(buffer,0,len); } System.out.println(baos.toString()); System.out.println("Received from:"+socket1.getInetAddress().getHostAddress()+"Information about"); // baos.close(); } }
Java reflection mechanism
//