Exception type
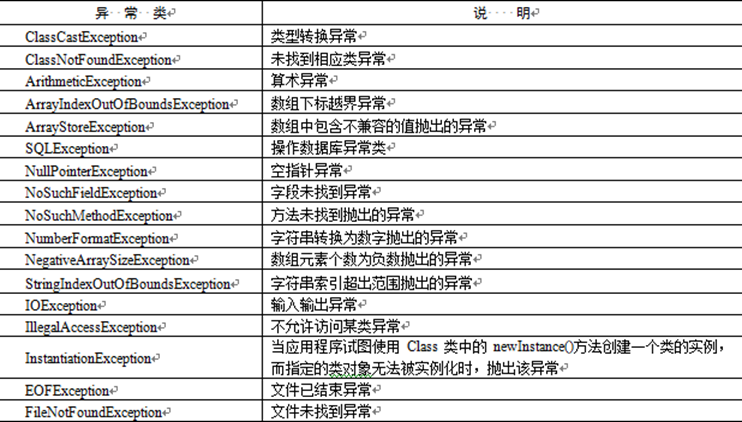
An exception is an object created from an exception class. In this case, the exception class is java.lang.ArithmeticException. The exception is captured by the catch block, and the code block in the catch block is used to handle the exception
An exception may be thrown directly through the throw statement in the block of try, or it may be thrown by calling a method that may throw an exception. The advantages of exception handling enable the method to throw an exception to its caller and the caller to handle the exception
Throwing an exception is to mail the exception from one place to another
The exception capture structure consists of try, catch and finally. The try statement stores the statements with possible exceptions. The catch program block is placed after the try statement to trigger the captured exceptions. The finally statement block is the last execution part of the exception handling structure. No matter how the code in the try statement block exits, the fianlly statement will be executed.
The identifier ex in Exceptiontype1 ex is very similar to the parameter in the method, so this parameter is called the parameter of the catch block
The statement code is as follows:
try{ //Code execution block } catch(Exceptiontype1 e){ //Processing of Exceptiontype1 } catch(Exceptiontype2 e){ //Processing of Exceptiontype2 } ... finally{ //Program block }
try...catch exception capture structure
import java.util.Scanner; public class QuetienWithException { public static int quotient(int number1,int number2) { if (number2 == 0) throw new ArithmeticException("Divisor cannot be zero"); return number1 / number2; } public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("Enter two integers:"); int number1 = input.nextInt(); int number2 = input.nextInt(); try{ int result = quotient(number1,number2); System.out.println(number1+" / "+number2+" is "+result); } catch(ArithmeticException ex){ System.out.println("Exception:an integer"+"cannot be dicided by zero"); } System.out.println("Execution continues......"); } }
ry...catch...finally exception capture structure
In the exception handling syntax structure, only try blocks are required. If there is no try, there can be no subsequent catch blocks and finally blocks; Both catch block and finally block are optional, but at least one catch block and finally block can also occur at the same time. There can be multiple catch blocks. The catch block that catches the parent class exception must be behind the catch child class exception; However, there cannot be only try blocks, neither catch blocks nor finally blocks: multiple catch blocks must be after try blocks, and finally blocks must be after all catch blocks.
package Text; import java.io.FileInputStream; import java.io.IOException; public class FinallyTest{ public static void main(String[] args) { FileInputStream fis = null; try { fis = new FileInputStream("a.txt"); } catch(IOException ioe) { System.out.println(ioe.getMessage()); //The return statement forces the method to return return; //Use exit to exit the virtual machine //System.exit(1); } finally { //Close disk files and recycle resources if(fis!= null) { try{ fis.close(); } catch(IOException ioe) { ioe.printStackTrace(); } } System.out.println("implement finally Resource recovery in block"); } } }
Unless the method of exiting the virtual machine is invoked in tyr block or catch block, any exception handling block will always be executed no matter what code is executed in tyr block or catch block.
send
Throw an exception with the throws declaration
Throws declaration throw can only be used in method signature. Throws can throw multiple exception classes separated by commas. The syntax of throws declaration throw is as follows:
throws ExceptionClass1,ExceptionClass2......
There is a limitation when throwing an exception with the throws declaration, which is a rule in the "two small" when overriding a method: the exception type thrown by the subclass method declaration should be the exception type thrown by the parent class declaration or the same, and the exceptions thrown by the subclass method declaration are not allowed to be more than those thrown by the parent class method.
package Text; import java.io.FileInputStream; import java.io.IOException; public class ThrowsTest1{ public static void main(String[] args) throws IOException { FileInputStream fis = new FileInputStream("a.txt"); } } package Text; import java.io.FileInputStream; import java.io.IOException; public class ThrowsText2{ public static void main(String[] args) throws Exception{ /* * Because the Test() method declaration threw an IOException * So the code calling this method is either in the try... catch block * Or in another method thrown with the throws declaration */ } public static void test() throws IOException{ /* *Because the constructor declaration of FileInputStream threw IOException exception *Therefore, the code calling FileInputStream is either in the try... catch block *Or in another method thrown with the throws declaration */ FileInputStream fis = new FileInputStream("a.txt"); } }
throw exception
If you need to throw an exception in the program, you should use the throw statement. The throw statement can be used alone. The throw statement throws not an exception class, but an exception instance, and only one exception instance can be thrown at a time. The syntax format of throw statement is as follows:
throw ExceptionInstance;
Custom exception class
All user-defined exceptions should inherit the Exception base class. If you want to customize the Runtime Exception, you should inherit the RuntimeException base class. When defining the Exception class, you usually need to provide two constructors: a constructor without parameters and a constructor with a string parameter. This string will be used as the description information of the Exception object (that is, the return value of the getMessage() method of the Exception object).
To use a custom exception
-
Create custom exception class
-
Throw an exception object through the throw keyword in the method
-
If an exception is handled in the method that currently throws an exception, you can use the try catch statement block to catch and handle it. Otherwise, indicate the exception to be excluded from the method caller through the throws keyword in the method declaration, and continue to the next step
-
Catch and handle exceptions in the caller of the exception method
Exception handling rules
-
Do not overuse exceptions
-
Do not use too large try blocks
-
Avoid using Catch All statements
-
Don't ignore caught exceptions