catalogue
preface
This paper records that the author has realized the java connection to the database through the study of JDBC, and completed some simple operations on the database data. The code has been verified by the author. It is the first article for novices. The boss is welcome to criticize and correct.
1, What is JDBC?
JDBC (Java DataBase Connectivity) Java database connection technology is a bridge between Java and database. Through it, we can connect various databases and execute SQL (DML, DDL) statements. It can be understood as a set of specifications formulated by SUN company to connect to the database and execute SQL statements in Java, rather than an implementation. (all the codes in this article are implemented for SQLserver)
2, JDBC programming steps
(1) Design concept
If java wants to connect to the database, it needs to use SUN's JDBC specification, but SUN company does not provide the implementation of JDBC connecting to each database, but needs each database manufacturer to complete it and create the corresponding implementation interface according to their own database
name | type | explain |
---|---|---|
Driver | Interface | Used to represent driver classes |
Connection | Interface | This interface is used to connect to the database |
Statement | Interface | Execute the SQL statement and retrieve the data into the ResultSet |
ResultSet | Interface | Database result set data table, usually generated by executing query database statements |
PrepareStatement | Interface | Execute precompiled SQL statements |
DriverManager | class | Driver manager, through which to get the connection object |
CallableStatement | Interface | Used to execute stored procedures |
Diagram:
(2) Preparatory work
Download the driver jar package from the official website of the corresponding database, which integrates the implementation classes of the JDBC interface made by the database company (take SQLserver as an example here)
(3) Open Idea
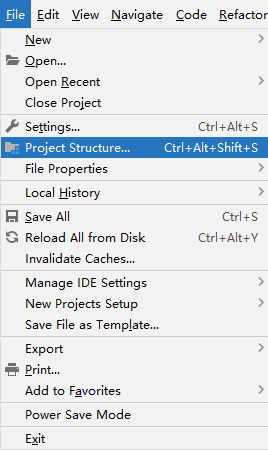
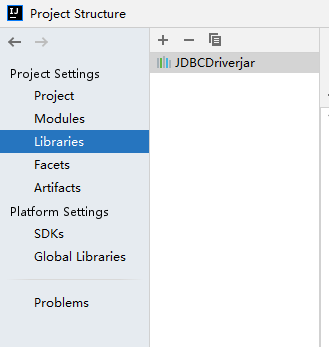
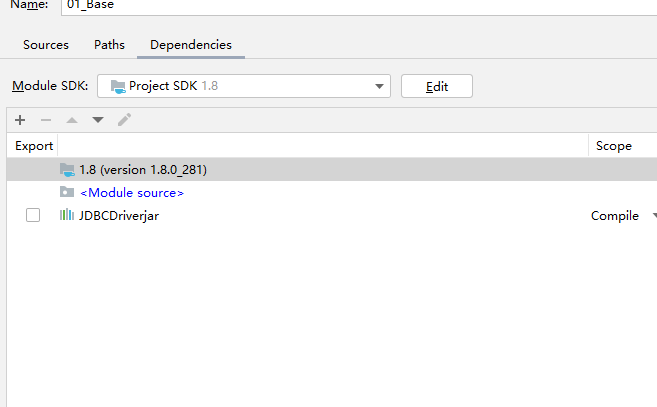
Finally, click OK and ctrl+N to enter SQLserverConnection. If you successfully search the SQLserverConnection interface and its implementation class, congratulations on your successful addition
(4) Code section steps
1. Load driver Class.forName("driver class package name + class name")
a) Static method, class name. Method name
b) Throw compile time exception ClassNotFoundException (alt+enter error code solution)
2. Establish Connection DriverMananger.getConnection(url)
a)Connection con = DriverMananger.getConnection(url,uerName,pwd)
b) Throw a compile time exception SQLException (calling any method in the java.sql package will throw a compile time exception SQLException)
c) url uniform resource locator jdbc:type//ip:port;databaseName=dbName(localhost)
3. Create Statement object con.createStatement()
a) Execute sql:
Write an sql statement for testing: String sql = "delete from teacher where team = '12345678'";
int affects the number of rows = sta.executeUpdate(sql);
4. Close resource
a) Closing resources is in the reverse order of creating resources
b) When an object has no reference to it, it is a garbage resource
package com.ming; /*use 1,Get the driver jar package of the corresponding database (1)Download mysql jdbc driver download from the official website (2)decompression 2,Module reference jar package (1)Create project Libraries Project Structure - > Libraries - > Add (2)Module reference libraries project structure - > modules - > Add lib */ import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement; public class TestJDBC { /* 1,load driver Class.forName("Package name + class name "); (1) The driver class must implement java. sql. Driver (2) Class. forName()Static method (3) A compile time exception ClassNotFoundException will be thrown (4) I'll go to the DriverManager to register 2,ip address for establishing connection: port; databaseName userName pwd (1)URL(Uniform resource locator) (2)getConnection()Static method (3)Throw compile time exception SQLException Calling any method in the java sql package will throw a compile time exception SQLException 3,Create Statement object 4,Execute sql (DML insert update delete/ select) int executeUpdate(insert update delete )sql Number of rows affected by the statement ReultSet executeQuery(select) */ public static void main(String[] args) { Statement sta = null; Connection con = null; try { Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver"); String url = "jdbc:sqlserver://127.0.0.1:1433;databaseName=PAS"; con = DriverManager.getConnection(url,"sa","610521"); System.out.println(con); sta = con.createStatement(); // String SQL = "insert into teacher (teanum, teaname, teattitle, teatypeid, teabtd, PWD, static) values ('12345678 ',' Liu Yiming ',' Professor ', 1,' 1981-7-5 ', 1, 1)"; String sql = "delete from teacher where teanum = '12345678'"; int rows = sta.executeUpdate(sql); if(rows==1){ System.out.println("Operation succeeded"); }else{ System.out.println("operation failed"); } } catch (ClassNotFoundException e) { System.out.println("Driver loading failed"); e.printStackTrace(); } catch (SQLException throwables) { throwables.printStackTrace(); }finally { try { if (sta != null){ sta.close(); sta = null; } if (con != null){ con.close(); con = null; } } catch (SQLException e) { e.printStackTrace(); } } } }
3, Summary
This article only deals with adding, deleting and modifying java database, and the query operation is not involved for the time being