When most people use jQuery, they use the first new free construction method to construct directly $(''), which is also a very convenient place for jQuery. When we use the first new free construction method, its essence is equivalent to new jQuery(). How is it implemented inside jQuery? have a look:
(function(window, undefined) { var // ... jQuery = function(selector, context) { // The jQuery object is actually just the init constructor 'enhanced' // Here, the instantiation method jQuery() actually calls its extended prototype method jQuery fn. init return new jQuery.fn.init(selector, context, rootjQuery); }, // jQuery.prototype is the prototype of jQuery. The method attached to it can be used by all generated jQuery objects jQuery.fn = jQuery.prototype = { // Instantiation method, which can be called jQuery object constructor init: function(selector, context, rootjQuery) { // ... } } // This sentence is very key and very winding // Instead of instantiating jQuery with the new operator, jQuery calls its functions directly // To achieve this, jQuery should be regarded as a class and return a correct instance // And the instance should be able to correctly access the properties and methods on the jQuery class prototype // The way of jQuery is to solve the problem through prototype transfer, and transfer the prototype of jQuery to jQuery prototype. init. prototype // So the instance this generated by this method still points to jQuery FN, so you can correctly access the properties and methods on the jQuery class prototype jQuery.fn.init.prototype = jQuery.fn; })(window);
Most people first see
jQuery.fn.init.prototype = jQuery.fn
This sentence will be the card owner, very puzzled. But this sentence is really the beauty of jQuery. It is very important to understand these sentences. Analyze them in points:
-
First of all, it should be clear that the internal call of the instantiation method of $('xxx ') is return new jQuery fn. The sentence init (selector, context, rootjquery) means that the construction instance is handed over to jQuery fn. Init () method to complete.
-
Add jQuery fn. The prototype property of init is set to jQuery FN, then use new jQuery fn. The prototype object of the object generated by init () is jQuery FN, so mount to jQuery FN the above function is equivalent to mounting to jQuery fn. On the jQuery object generated by init(), all use {new jQuery fn. The objects generated by init () can also access jQuery All prototype methods on FN.
-
That is, the instantiation method has such a relationship chain
-
jQuery.fn.init.prototype = jQuery.fn = jQuery.prototype ;
-
new jQuery.fn.init() is equivalent to new jQuery();
-
JQuery () returns new jQuery fn. Init() and var obj = new jQuery(), so the two are equivalent, so we can instantiate jQuery objects without new.
4, Overloading of jQuery methods
==============
1. Basic introduction to method overloading
Another reason why the jQuery source code is obscure and difficult to read is that it uses a lot of method overloading, but it is very convenient to use:
// Gets the value of the title property $('#id').attr('title'); // Set the value of the title property $('#id').attr('title','jQuery'); // Gets the value of a css property $('#id').css('title'); // Set the value of a css property $('#id').css('width','200px');
2. Application scenario
Method overloading is a method that implements multiple functions, often get and set. Although it is very difficult to read, from the perspective of practicality, this is why jQuery is so popular. Most people use jQuery() construction methods to directly instantiate a jQuery object, but in its internal implementation, There are 9 different ways to reload scenarios:
// Accepts a string containing the CSS selector used to match the collection of elements jQuery([selector,[context]]) // Pass in a single DOM jQuery(element) // Pass in DOM array jQuery(elementArray) // Incoming JS object jQuery(object) // Incoming jQuery object jQuery(jQuery object) // Create a DOM element by passing in a string from the original HTML jQuery(html,[ownerDocument]) jQuery(html,[attributes]) // Pass in null parameter jQuery() // Bind a function that executes after the DOM document is loaded jQuery(callback)
Therefore, when reading the source code, it is very important to read it in combination with the jQuery API to understand how many functions are overloaded by methods. At the same time, I want to say that the implementation of some methods in the jQuery source code is particularly long and cumbersome, because jQuery itself, as a framework with strong universality, a method is compatible with many situations and allows users to pass in various parameters, The logic of internal processing is very complex, so when interpreting a method, I feel obvious difficulties. I try to jump out of the stuck code itself and think about what these complex logic is for processing or compatibility, whether it is overload, and why it is written in this way. There will be different gains. Secondly, it is also for this reason that there are many code fragments compatible with lower versions of HACK or very obscure and cumbersome logic in the jQuery source code. The big pit of browser compatibility is extremely easy for a front-end engineer to not learn the essence of programming, so don't be too attached to some leftover materials. Even if compatibility is very important, you should learn and understand it appropriately.
5, JQuery fn. Extend and jQuery extend
==================================
1. Basic analysis
The extend method is a very important method in jQuery. It is used internally by jQuery to extend static methods or instance methods, and we will also use it when developing jQuery plug-ins. But internally, there is jQuery fn. Extend and jQuery Extend two extend methods, and distinguishing these two extend methods is a key part of understanding jQuery. Let's look at the conclusion first:
-
jQuery.extend(object) extends the jQuery class itself and adds a new static method to the class;
-
jQuery.fn.extend(object) adds an instance method to the jQuery object, that is, through the new method added by extend, the instantiated jQuery object can be used because it is attached to jQuery FN (mentioned above, jQuery.fn = jQuery.prototype).
2. Official interpretation
Their official interpretation is:
-
jQuery.extend(): merge two or more objects into the first one,
-
jQuery.fn.extend(): mount the object to the prototype attribute of jQuery to extend a new jQuery instance method.
That is, use jQuery Extend() extends the static method. We can directly use $ Call xxx (xxx is the extended method name),
Instead, use jQuery fn. Extend() extends the instance method. You need to use $() xxx call.
Long source code parsing:
// Extended merge function // Merge the properties of two or more objects into the first object, and most of the subsequent functions of jQuery are extended through this function // Although the implementation methods are the same, we should pay attention to different usages. Then why do two methods point to the same function implementation but realize different functions, // Reading the source code, you can find that this is due to the powerful power of this // If two or more objects are passed in, the attributes of all objects are added to the first object target // If only one object is passed in, the properties of the object are added to the jQuery object, that is, static methods are added // In this way, we can add new methods to the jQuery namespace, which can be used to write jQuery plug-ins // If you don't want to change the incoming object, you can pass in an empty object: $ extend({}, object1, object2); // The default merge operation is not iterative. Even if an attribute of the target is an object or attribute, it will be completely overwritten instead of merged // If the first parameter is true, it is a deep copy // Attributes inherited from the object prototype will be copied, and attributes with undefined values will not be copied // For performance reasons, properties of JavaScript's own types will not be merged jQuery.extend = jQuery.fn.extend = function() { var src, copyIsArray, copy, name, options, clone, target = arguments[0] || {}, i = 1, length = arguments.length, deep = false; // Handle a deep copy situation // target is the first parameter passed in // If the first parameter is Boolean, it indicates whether deep recursion is required, if (typeof target === "boolean") { deep = target; target = arguments[1] || {}; // skip the boolean and the target // If the first parameter of type boolean is passed, i starts from 2 i = 2; } // Handle case when target is a string or something (possible in deep copy) // If the first parameter passed in is a string or other if (typeof target !== "object" && !jQuery.isFunction(target)) { target = {}; } // extend jQuery itself if only one argument is passed // If the length of the parameter is 1, it means that it is a jQuery static method if (length === i) { target = this; --i; } // Multiple replication sources can be passed in // i starts with 1 or 2 for (; i < length; i++) { // Only deal with non-null/undefined values // Copy all the attributes of each source to the target if ((options = arguments[i]) != null) { // Extend the base object for (name in options) { // src is the value of the source (that is, itself) // copy is the value that will be copied in the past src = target[name]; copy = options[name]; // Prevent never-ending loop // Prevent rings, such as extend(true, target, {'target':target}); if (target === copy) { continue; } // Recurse if we're merging plain objects or arrays // Here is a recursive call, which will eventually go to the else if branch below // jQuery. Isplanobject is used to test whether it is a pure object // Pure objects are created through "{}" or "new Object" // In case of deep copy if (deep && copy && (jQuery.isPlainObject(copy) || (copyIsArray = jQuery.isArray(copy)))) { // array if (copyIsArray) { copyIsArray = false; clone = src && jQuery.isArray(src) ? src : []; // object } else { clone = src && jQuery.isPlainObject(src) ? src : {}; } // Never move original objects, clone them // recursion target[name] = jQuery.extend(deep, clone, copy); // Don't bring in undefined values // Eventually to this branch // Simple value override } else if (copy !== undefined) { target[name] = copy; } } } } // Return the modified object // Return new target // If I < length, the unprocessed target is returned directly, that is, arguments[0] // That is, if you do not pass the source to be overwritten, call $ extend is actually a static method to add jQuery return target; };
Note that this sentence is jQuery extend = jQuery.fn.extend = function() {}, that is, jQuery Implementation of extend and jQuery fn. The implementation of extend shares the same method, but why it can realize different functions is due to the powerful (weird?) this of Javascript.
-
In jQuery In extend (), this refers to jQuery object (or jQuery class), so it is extended on jQuery here;
-
In jQuery fn. In extend (), this points to the FN object. JQuery. Net was mentioned earlier fn = jQuery. Prototype, that is, the prototype method, that is, the object method, is added here.
6, Chain calling and backtracking of jQuery
=================
Another reason why we like to use jQuery is its chain call. In fact, the implementation of this is very simple. We can realize the chain call only by returning this in the return result of the method to realize the chain call.
Of course, in addition to chain calls, jQuery even allows backtracking. See:
// Terminate the latest filtering operation in the current chain through the end() method and return the previous object collection $('div').eq(0).show().end().eq(1).hide();
jQuery complete chain call, stack increase and backtracking through return this and return this pushStack() ,return this.prevObject} implementation. Look at the source implementation:
in general,
-
The end() method returns the prevObject attribute, which records the jQuery object collection of the previous operation;
-
The prevObject attribute is generated by the pushStack() method, which adds a collection of DOM elements to a stack managed by jQuery, and tracks the DOM result set returned by the previous method in the chain call by changing the prevObject attribute of jQuery object
-
When we call the end() method in a chain, the prevObject attribute of the current jQuery object is returned internally to complete the backtracking.
7, jQuery regularity and detail optimization
================
I have to mention that jQuery does a good job in detail optimization. There are also many tips worth learning. The next article will focus on some programming skills in jQuery, which will not be repeated here.
Then I want to talk about regular expressions. JQuery uses a lot of regular expressions. I think if you study jQuery, the level of regularity will be greatly improved. If you are a regular white, I suggest to understand the following points before reading:
-
Understand and try to use Javascript regular API s, including the usage of test(), replace(), match(), exec();
-
Distinguish the above four methods, which is RegExp object method and which is String object method;
-
Understand simple zero width assertions, what is matching but not capturing, and matching and capturing.
8, jQuery variable conflict handling
===============
1. Source code analysis
Finally, I want to mention the conflict handling of jQuery variables through the window that saves the global variables at the beginning JQuery and windw. $.
When conflicts need to be handled, call the static method noConflict() to give up the control of variables. The source code is as follows:
(function(window, undefined) { var // Map over jQuery in case of overwrite // Set the alias and map the jQuery and $objects in the window environment through two private variables to prevent the variables from being forcibly overwritten _jQuery = window.jQuery, _$ = window.$; jQuery.extend({ // The noConflict() method gives jQuery control of the variable $, so that other scripts can use it // jQuery is used with full names instead of abbreviations // deep -- boolean indicating whether to allow the jQuery variable to be completely restored (whether to hand over the jQuery object itself while handing over the $reference) noConflict: function(deep) { // Judge whether the global $variable is equal to the jQuery variable // If equal to, the global variable $is restored to the variable before jQuery runs (stored in the internal variable $) if (window.$ === jQuery) { // At this time, the jQuery alias $is invalid window.$ = _$; ### last Xiaobian carefully prepared first-hand materials for everyone 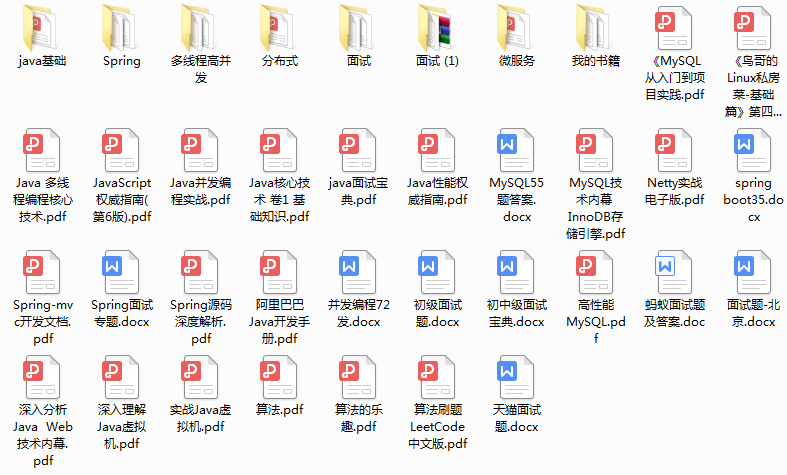 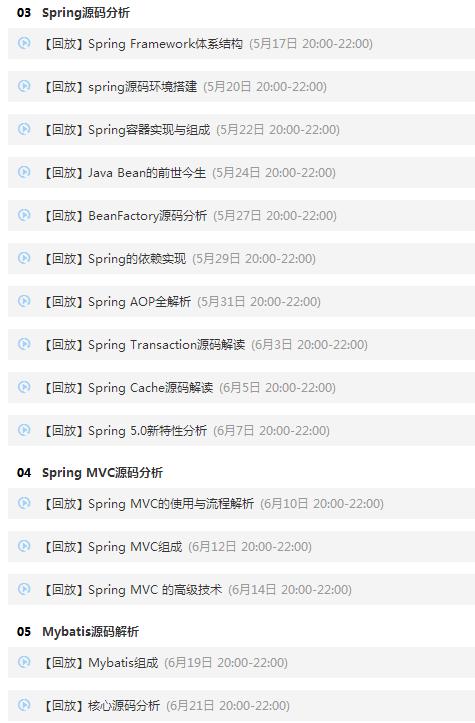 above Java Advanced architecture information, source code, notes, videos. Dubbo,Redis,Design mode Netty,zookeeper,Spring cloud,Distributed, high concurrency and other architecture technologies **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** [Attached] architecture books 1. BAT Analysis of 20 high frequency database questions in interview 2. Java Interview dictionary 3. Netty actual combat 4. algorithm 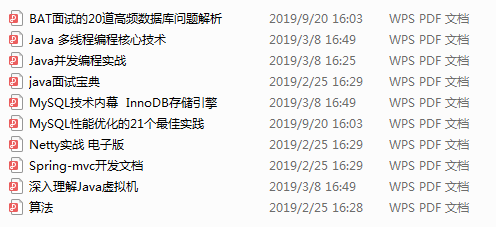 **BATJ Interview points and Java Architect advanced data** 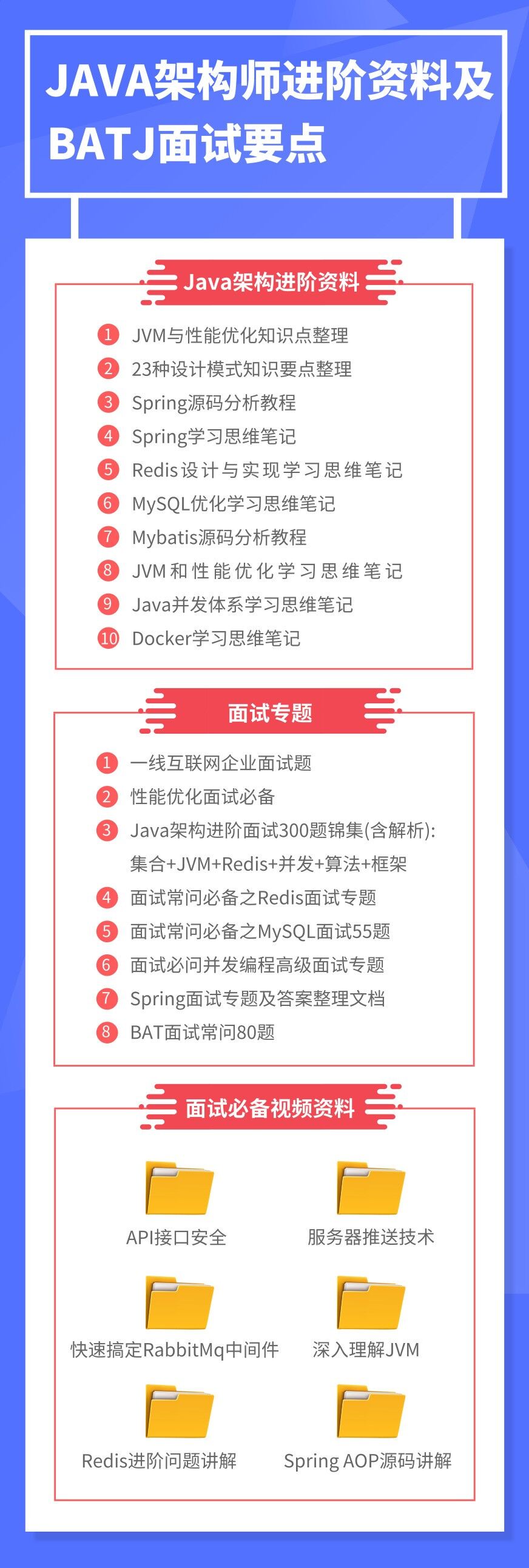 (window.$ === jQuery) { // At this time, the jQuery alias $is invalid window.$ = _$; ### last Xiaobian carefully prepared first-hand materials for everyone [External chain picture transfer...(img-uFLQbhNY-1630469410598)] [External chain picture transfer...(img-iFhhmDaD-1630469410600)] above Java Advanced architecture information, source code, notes, videos. Dubbo,Redis,Design mode Netty,zookeeper,Spring cloud,Distributed, high concurrency and other architecture technologies **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** [Attached] architecture books 1. BAT Analysis of 20 high frequency database questions in interview 2. Java Interview dictionary 3. Netty actual combat 4. algorithm [External chain picture transfer...(img-JqGg1I2D-1630469410601)] **BATJ Interview points and Java Architect advanced data** [External chain picture transfer...(img-j2cszQJf-1630469410602)]