catalogue
I am also a chicken, please communicate more ~ ~!! Thank you, roll king!
1, Basic introduction to jQuery
2, Use jQuery to output the first hello world
4, Differences between jQuery and DOM objects
7, DOM addition, deletion and modification
I am also a chicken, please communicate more ~ ~!! Thank you, roll king!
1, Basic introduction to jQuery
- What is jQuery?
- jQuery: JavaScript and Query, which is a js class library to assist JavaScript development. It is very popular at present
- What is the core idea of jQuery?
- His core idea is write less, do more, So it implements many browser compatibility problems.
- Advantages of JQury
- JQuery is free and open source. The syntax design of jQuery can make development more convenient, such as operating document objects, selecting DOM elements, making animation effects, event processing, using Ajax and other functions.
2, Use jQuery to output the first hello world
- Import jQuery file
- Download address: https://www.jb51.net/zt/jquerydown.htm
- Put the downloaded jQuery file into the project and import it into html
// src: jQuery path put into the project <script src="../jQuery/jquery-3.6.0.js"></script>
Click event for object binding: $object name click() ;<html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <script src="../jQuery/jquery-3.6.0.js"></script> <script type="text/javascript"> /*Use js to output hello */ // window.onload = function () { // var btn = document.getElementById("btn"); // btn.onclick = function () { // alert("say Hello") // } // } $(function () { // $(): equivalent to onload event, after the page is loaded. var $btn = $("#btn "); / / get the btn object, which is equivalent to document.getElementById("btn "). $btn.click(function () { //Click event for btn binding alert("hello world !!") }); }); </script> <body> <input type="button" id="btn" value="sayHello"> </body> </html>
III. what is $()
- So what exactly does the symbol $mean?
- $is the core function of jQuery, which can complete many functions of jQuery, $(); Is to call this function
- When a function is passed in $() parentheses
-
$(function(){ //Equivalent to window Onload, which is executed after the page is loaded });
-
- When the passed in parameter is [HTML string]
- This html object will be created
-
$(function () { $(" <div>" + " <span>hello world!</span>" + " </div>").appendTo("body"); });
- When the passed in parameter is [selector string]
- $("#id attribute"): ID selector, query label object according to ID.
- $(". Class attribute"): class selector
- $("tag name"): tag selector
- When a DOM object is passed in a parameter
- The DOM object is converted into a jQuery object
4, Differences between jQuery and DOM objects
- DOM objects are: getelementbyid(), getelementbyname(), getElementByTagName() The objects created by these methods are DOM objects
- Its form is: [object HTML tag name Element]
- JQuery objects are: API s, methods, and parameters provided by jQuery are created by DOM objects
- Its form: [object]
- What is the essence of JQury?
- In fact, it can be seen from deBug that jQuery is actually an array with some extension methods.
- So since it's an array, let's go through it and see what the elements are
- There are DOM objects in jQuery objects. It can be seen that jQuery is actually an array + extension method that encapsulates DOM objects
- The method DOM object of jQuery object cannot be used, and the method jQuery object in DOM object cannot be used
- So how do jQuery objects and DOM objects convert to each other?
- DOM ---> jQuery
- 1. Get the DOM object first
- 2. Convert through the $() function.
- DOM ---> jQuery
-
- jQuery ---> DOM
- 1. Get jQuery object
- 2. Method to get the subscript of the DOM object through the array
- jQuery ---> DOM
5, jQuery selector
5.1 foundation selector
- "#id" ID selector
- ". class" class selector
- Label name label selector
- "*" select all elements
- "selector1, selector2" combination selector
- $(""). css() ; Set CSS style of jQuery object.
Note: when searching for elements, it must be after the page is loaded, otherwise it cannot be loaded.
//The first method $(function(){ //Indicates that after the page is loaded }); //The second method $(document).ready(function(){ });
5.2 level selector
- "ancestor descendant"
- ancestor: select a valid selector
- Descendant: descendant element of the ancestor element
- Simply put, the "ancestor" element contains the "descendant" element.
-
"Parent > child": matches all child elements under a given parent element
-
Parent: select an effective selector parent element
-
Child: child of parent element
-
-
"prev + next": matches all the next elements immediately after the prev element
-
prev: valid selector
-
next: the element following the prev element
-
-
prev ~ siblings
-
prev: valid selector
-
siblings: find elements at the same level as prev elements
-
5.3 basic filter selector
- : first # match the first element found
-
: last} matches the last element found
-
: not all elements matching the given selector are removed
-
: even matches all elements with an even index value, counting from 0
-
: odd , matches all elements with odd index values, counting from 0
-
: eq(index) element matching a given index value [index starts from 0]
-
: gt(index) matches all elements greater than the given index value
-
: lt(index) matches all elements greater than the given index value
-
: header , matches Title elements such as H1, H2 and H3
-
: animated # matches all elements that are performing animation effects
$(document).ready(function(){ //1. Select the first div element $("#btn1").click(function(){ $("div:first").css("background", "#bbffaa"); }); //2. Select the last div element $("#btn2").click(function(){ $("div:last").css("background", "#bbffaa"); }); //3. Select all div elements whose class is not one $("#btn3").click(function(){ $("div:not(.one)").css("background", "#bbffaa"); }); //4. Select div element with even index value /*The first div index is 1 and the second div index is 2*/ /* Commonly used for table discoloration */ $("#btn4").click(function(){ $("div:even").css("background", "#bbffaa"); }); //5. Select div elements with odd index values $("#btn5").click(function(){ $("div:odd").css("background", "#bbffaa"); }); //6. Select div elements with index value greater than 3 $("#btn6").click(function(){ $("div:gt(3)").css("background", "#bbffaa"); }); //7. Select the div element whose index value is equal to 3 $("#btn7").click(function(){ $("div:eq(3)").css("background", "#bbffaa"); }); //8. Select div element with index value less than 3 $("#btn8").click(function(){ $("div:lt(3)").css("background", "#bbffaa"); }); //9. Select all title elements $("#btn9").click(function(){ $(":header").css("background", "#bbffaa"); }); //10. Select all the elements currently executing the animation $("#btn10").click(function(){ $(":animated").css("background", "#bbffaa"); }); });
5.4 content filter selector
- : contains(text) matches the element containing the given text
-
: empty match all empty elements that do not contain child elements or text [empty element]
-
: has(selector) matches the element that contains the element that the selector matches
-
: parent , matching elements with child elements or text [non empty]
$(document).ready(function(){ //1. Select div element with text 'di' $("#btn1").click(function () { $("div:contains(di)").css("background-color","#bbffaa") }); //2. Select a div empty element that does not contain child elements (or text elements) $("#btn2").click(function(){ $("div:empty").css("background","#bbffaa") }); //3. Select div element with class as mini element $("#btn3").click(function(){ /*Note: the last selected element is the div element. Not a class element*/ $("div:has(.mini)").css("background", "#bbffaa"); }); //4. Select a div element that contains child elements (or text elements) $("#btn4").click(function(){ $("div:parent").css("background", "#bbffaa"); }); });
5.5 attribute filter selector
- [attribute] matches the element containing the given attribute
-
[attribute=value] the element matching the given attribute is a specific value
-
[attribute!=value] matches all elements that do not contain the specified attribute or whose attribute is not equal to a specific value.
-
[attribute^=value] matching a given attribute is an element that starts with some value
-
[attribute$=value] matching a given attribute is an element that ends with some value
-
[attribute*=value] matches a given attribute to an element that contains some value
-
[selector1][selector2][selectorN] composite attribute selector, which is used when multiple conditions need to be met at the same time.
$(function() { //1. Select the div element containing the attribute title $("#btn1").click(function() { $("div[title]").css("background", "#bbffaa"); }); //2. Select the div element whose attribute title Value is equal to 'test' $("#btn2").click(function() { $("div[title='test']").css("background", "#bbffaa"); }); //3. Select div elements whose attribute Title Value is not equal to 'test' (* those without attribute title will also be selected) $("#btn3").click(function() { $("div[title!='test']").css("background", "#bbffaa"); }); //4. Select the div element whose attribute title Value starts with 'te' $("#btn4").click(function() { $("div[title^='te']").css("background", "#bbffaa"); }); //5. Select the div element whose attribute title Value ends with 'est' $("#btn5").click(function() { $("div[title$='est']").css("background", "#bbffaa"); }); //6. Select the div element whose attribute title Value contains' es' $("#btn6").click(function() { $("div[title*='es']").css("background", "#bbffaa"); }); //7. First select the div element with attribute id, and then select the div element with attribute title Value containing 'es' in the result $("#btn7").click(function() { $("div[id][title*='es']").css("background", "#bbffaa"); }); //8. Select the div element that contains the title attribute value and the title attribute value is not equal to test $("#btn8").click(function() { $("div[title][title!='test']").css("background", "#bbffaa"); }); });
5.6 form filter selector
- : input matches all input, textarea, select and button elements
- : text match all single line text boxes [type is text type]
- : password match all password boxes [type is password]
- : Radio # match all radio buttons [type is radio]
- : checkbox match all checkboxes [type is checkbox]
- : submit matches all submit buttons [type is submit]
- : image match all image fields [type is image]
- : reset match all image fields [type is reset]
- : button match all buttons [type is button]
- : File match all file fields [type is file]
- : hidden , matches all invisible elements or elements of type hidden
5.7 form object attribute filter selector
- : enabled , match all available elements [input tag plus disabled is unavailable. No is available]
-
: disabled , matches all unavailable elements
-
: checked matches all selected elements (check box, radio box, etc., excluding option in select)
-
: selected matches all selected option elements [drop-down list of selected elements]
//each method in jQuery can be traversed // this represents the current object to loop to $("").each(function(){ alert(this); });
The {this object in the function function in the event: represents the dom object currently responding to the event.
As shown in the figure above, this represents the tag with id checkedAllBox. this.value is the value of this tag.
5.8 element screening
- filter
-
- first() The function is the same as: first to get the first element
- last() The function is the same as: last to get the last element
- eq(index) The function is the same as: eq(), which gets the elements of the given index
- has(expr) The function is the same as: has, which returns the element containing the element matching the selector
- filter(expr) The function is the same as that of ancestor descendant
- is(expr) Judge whether it matches the given selector. As long as there is a match, it returns true
- not(expr) The function is the same as: not to delete the elements matching the selector
-
lookup
- add(expr) Add the element of the selector matching add to the current jquery object
- children([expr]) The function is the same as that of parent > child, which returns the child elements matching the given selector
- find(expr) The function is the same as that of ancestor descendant, which returns the descendant elements matching the given selector
- next([expr]) The function is the same as prev + next, which returns the next sibling element of the current element
- nextAll([expr]) The function is the same as the prev ~ siblings function, which returns all sibling elements behind the current element
- parent([expr]) Return parent element
- prev([expr]) Returns the previous sibling element of the current element
- prevAll([expr]) Returns all sibling elements in front of the current element
- siblings([expr]) Return all sibling elements
6, jQuery property operation
- html() it can set and get the contents in the start tag and end tag, just like innerHTML in DOM
- The contents in the tag can be obtained without writing parameters in parentheses. Write parameters can set the contents of the label
- text() it can set and get the text in the start tag and end tag, just like innerText in DOM
- The contents in the tag can be obtained without writing parameters in parentheses. Write parameters can set the contents of the label
- val() he can set and get the form item [text, multi-choice, drop-down, single choice......] The value attribute value of, just like the value in DOM
- If you do not write parameters in parentheses, you can get the value in the text box. If you write parameters, you can set the value
- attr() can set and obtain the value of the attribute. Operations such as checked, readOnly, selected, disabled, etc. are not recommended
- The attr method can also manipulate non-standard attributes. For example, custom attributes: abc,bbj
- prop() can set and get the value of attributes. It only recommends operations such as checked, readOnly, selected, disabled, and so on
The difference between attr() and {prop():
If an element has no acquired attribute, attr() will return undefined [officials think undefined is an error, which is not conducive to user identification, so the prop() method appears], and prop will return false. Prop is more intuitive.
Both attr() and prop can set attribute values or modify attribute values.
7, DOM addition, deletion and modification
-
Internal insertion
The above is internal insertion, which is inserted into a label.
b: it can be content or object.
a.appendTo(b); Add a after B
a.append(b); Put B behind a
a.prependTo(b): insert a # in front of B #
a.prepend(b): insert B in front of A
-
External insertion
External insertion is to insert the} content outside a label.
a.afert(b); Insert B behind a
a.inertAfter(b); Insert a behind B
a.before(b); Insert B in front of A
a. Insert before (b) insert a in front of B
How to distinguish so many insertion methods?
A simple method is to put the contents in () into the specified position in front of ().
A. replace with (b): replace all a with B [replace with a B no matter how many elements of a there are]
a.replaceAll(b); A replaces all B [one B element is replaced by one a, two B elements are replaced by two a]
-
delete
a.empty() deletes the contents of the a tag
a.remove() deletes the a tag
8, CSS style actions
-
- addClass(class|fn) Add style
- removeClass([class|fn]) Delete style
- toggleClass(class|fn[,sw]) Delete the style if there is one, and add the style if there is none
- offset([coordinates]) Sets and gets the location of the element
<style type="text/css"> div{ width:100px; height:260px; } div.whiteborder{ border: 2px white solid; } div.redDiv{ background-color: #96E555; } div.blueBorder{ border: 5px blue solid; } </style> <script type="text/javascript" src="script/jquery-1.7.2.js"></script> <script type="text/javascript"> $(function(){ var $divEle = $("div:first"); $('#btn01').click(function(){ //addClass() - add one or more classes to the selected element // One or more styles are separated by spaces $divEle.addClass("redDiv blueBorder"); }); $('#btn02').click(function(){ //removeClass() - Deletes one or more classes from the selected element $divEle.remove(); }); $('#btn03').click(function(){ //Toggleclass - toggles the addition / deletion of selected elements $divEle.toggleClass("redDiv blueBorder") }); $('#btn04').click(function(){ //offset() - returns the position of the first matching element relative to the document. var offset = $divEle.offset(); console.log(offset); }); }) </script>
Use offset to get the position: Top indicates the distance from the top of the browser, while left indicates the distance from the left of the browser
//Set position $divEle.offset({ top:100, left:200 });
9, jQuery animation display
-
basic
- show([speed,[easing],[fn]]) Show hidden elements
- hide([speed,[easing],[fn]]) Hide visible elements
- toggle([speed],[easing],[fn]) Hide when visible and show when invisible
-
Fade in fade out
- fadeIn([speed],[eas],[fn]) Fade in
- fadeOut([speed],[eas],[fn]) Fade out
- fadeTo([[spe],opa,[eas],[fn]]) Slowly modify the transparency to the specified value [0-1] within the specified time
- fadeToggle([speed,[eas],[fn]]) Fade in and fade out switch
Parameters can be added in the above methods: [fadeTo: three parameters can be added: time, transparency and function]
First parameter: the execution time of the animation, in milliseconds
The second parameter: the callback function of the animation, and the function to be executed after the animation is executed
$(function(){ //show() $("#btn1").click(function(){ $("#div1").show(1000) }); //Hide $("#btn2").click(function(){ $("#div1").hide(2000) }); //toggle() $("#btn3").click(function(){ $("#div1").hide(2000,function () { alert("Animation finished") }) }); // Infinite animation // function a(){ // $("#div1").toggle(1000,a) // } // a(); //Fade fadeIn() $("#btn4").click(function(){ $("#div1").fadeIn(2000) }); //fadeOut() $("#btn5").click(function(){ $("#div1").fadeOut(2000,function () { alert("Fade out finished") }); }); //Fade to fadeTo() $("#btn6").click(function(){ $("#div1").fadeTo(2000,0.5) }); //Fade toggle () $("#btn7").click(function(){ $("#div1").fadeToggle(2000) }); // Infinite animation // function a(){ // $("#div1").fadeToggle(1000,a) // } // a(); });
10, Events in jQuery
- click() which can bind click events and trigger click events
- mouseover() mouse in event
- mouseout() mouse out event
- bind() can bind one or more events to an element at a time. [multiple events are separated by spaces]
- one() is the same as bind. However, the event bound by the one method will respond only once.
- unbind() is the opposite of the bind method to unbind the event
- live() is also used to bind events. It can be used to bind events of all elements matched by the selector. Even if this element is dynamically created later, it is also effective
$(function(){ // 1. How to bind events $("h5").click(function () { console.log("click Click event") }); //2. Binding method provided by jQuery: bind() function $("h5").bind("click",function () { console.log("bind Bound click event"); }); //3. Merge "mouse in" and "mouse out" events $("h5").bind('mouseover mouseout',function () { console.log("bind Bound mouse in and mouse out events"); }); //4. Merge click events $("h5").bind('mouseover mouseout click',function () { console.log("bind Bound mouse in and mouse out events"); }); //5. Switch element visible state $("div div").toggle(2000); //6. Bind only once $("h5").one('mouseover mouseout',function () { console.log("bind Bound mouse in and mouse out events"); }); // 7. Using live to bind events, all elements that match the matcher will have this event. Include created after /*This newly added h5 has no click event, However, if you bind click events to all h5 with live, the newly added h5 will also have click events */ $("\t<br><br><h5 class=\"head\">What is? jQuery?</h5>").appendTo($("h5")) $("h5").live("click",function () { alert("Click event") }); });
Bubbling of events
- What is the bubbling of events?
-
Event bubbling means that parent and child elements listen to the same event at the same time. When the event of the child element is triggered, the same event is also passed to the event of the parent element to respond
-
-
So how to stop the event from bubbling?
-
In the body of the child element event function, return false; It can prevent the bubbling of events
-
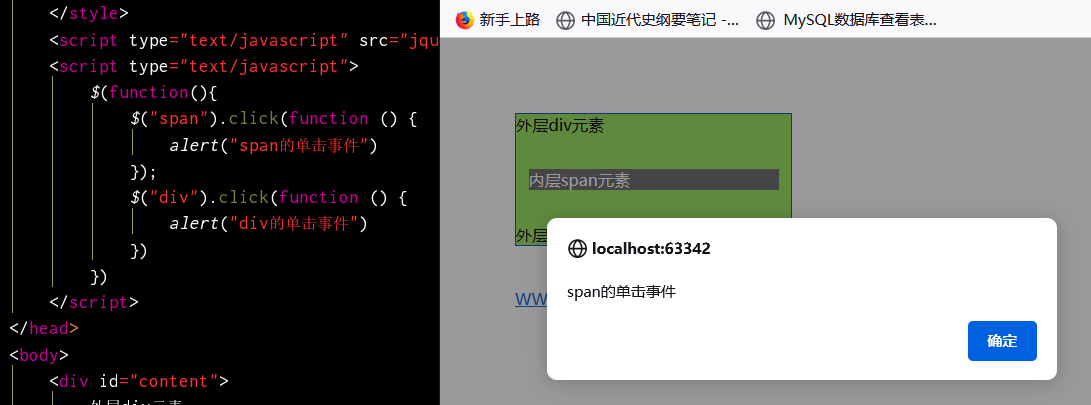
Add a return false to the click event of the child element; It stopped the event from bubbling.