With jQuery, you can easily delete existing HTML elements.
Delete element / content
To delete elements and content, you can generally use the following two jQuery methods:
remove() - delete the selected element (and its children)
empty() - remove child elements from selected elements
jQuery remove() method
The jQuery remove() method deletes the selected element and its children.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <script src="http://cdn.static.runoob.com/libs/jquery/1.10.2/jquery.min.js"> </script> <script> $(document).ready(function(){ $("button").click(function(){ $("#div1").remove(); }); }); </script> </head> <body> <div id="div1" style="height:100px;width:300px;border:1px solid black;background-color:yellow;"> This is div Some of the text in. <p>This is in div A paragraph in.</p> <p>This is in div Another paragraph in.</p> </div> <br> <button>remove div element</button> </body> </html>
Before operation:
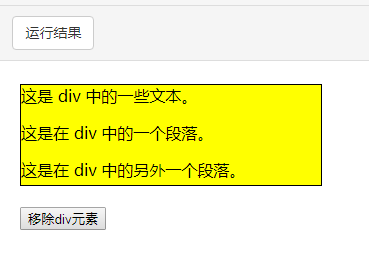
image.png
After operation
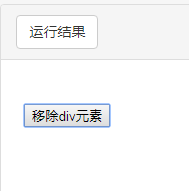
image.png
jQuery empty() method
The jQuery empty() method deletes the child elements of the selected element.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <script src="http://cdn.static.runoob.com/libs/jquery/1.10.2/jquery.min.js"> </script> <script> $(document).ready(function(){ $("button").click(function(){ $("#div1").empty(); }); }); </script> </head> <body> <div id="div1" style="height:100px;width:300px;border:1px solid black;background-color:yellow;"> This is div Some of the text in. <p>This is in div A paragraph in.</p> <p>This is in div Another paragraph in.</p> </div> <br> <button>empty div element</button> </body> </html>
Before execution
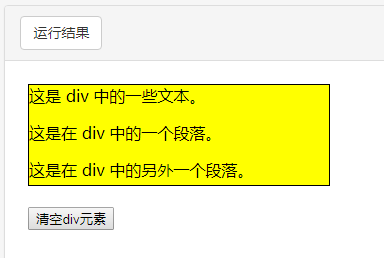
image.png
After execution
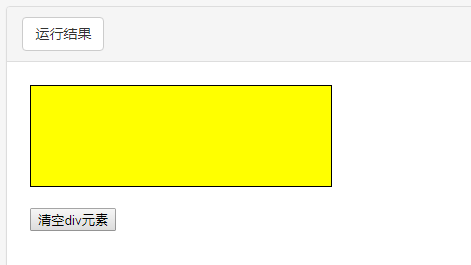
image.png