function
1. Verification that the required and length of "name" are at least two digits.
2. Verification that "email" is required and in E-mail format.
3. Verify whether the "web address" is a url.
4. Whether "your comment" needs to be verified.
Result chart
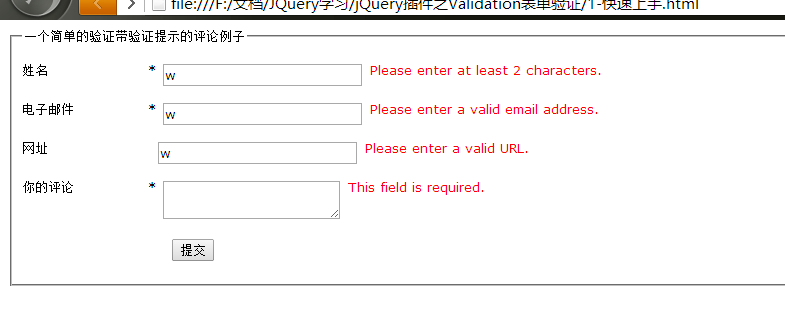
example
- <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
- <html xmlns="http://www.w3.org/1999/xhtml">
- <head>
- <meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
- <title>Get started quickly</title>
- <script type="text/javascript" src="../jquery-1.8/jquery-1.8.0.js"></script>
- <script type="text/javascript" src="../jquery.validate.js"></script>
- <style type="text/css">
- *{font-family:Verdana; font-size:96%;}
- label{width:10em; float:left}
- label.error{float:none; color:red; padding-left:.5em; vertical-align:top;}
- p{clear:both}
- .submit{margin-left:12em;}
- em{font-weight:bold; padding-right:lem; vertical-align:top;}
- </style>
- <script type="text/javascript">
- $(document).ready(function() {
- $("#commentForm").validate();
- });
- </script>
- </head>
- <body>
- <form class="cmxform" id="commentForm" method="get" action="#">
- <fieldset><!--fieldset Elements to group related elements within a form-->
- <legend>A simple example of verification with verification prompt</legend><!--legend Element is fieldset Element definition title-->
- <p>
- <label for="cusername">full name</label><em>*</em>
- <input id="cusername" name="username" size="25" class="required" minlength="2"/>
- </p>
- <p>
- <label for="cemail">E-mail</label><em>*</em>
- <input id="cemail" name="email" size="25" class="required email"/>
- </p>
- <p>
- <label for="curl">website</label><em> </em>
- <input id="curl" name="url" size="25" class="url" value=""/>
- </p>
- <p>
- <label for="ccomment">Your comments</label><em>*</em>
- <textarea id="ccomment" name="comment" cols="22" class="required"></textarea>
- </p>
- <p>
- <input class="submit" type="submit" value="Submit"/>
- </p>
- </fieldset>
- </form>
- </body>
- </html>
code analysis
1.jQuery code
Lines 1 and 2 import the jQuery class library and Validation plug-in.
- <script type="text/javascript" src="../jquery-1.8/jquery-1.8.0.js"></script>
- <script type="text/javascript" src="../jquery.validate.js"></script>
- <script type="text/javascript">
- $(document).ready(function() {
- $("#commentForm").validate();
- });
- </script>
Line 4 is used to execute the code in function when the code is loaded
Line 5 is to determine which form needs to be validated.
2. Code the validation rules for different fields, and set the corresponding properties of the fields
class="required" is required
class="required email" is required and conforms to the E-mail format.
class="url" verify for url format
minlength="2" is the minimum length of 2