catalogue
1,indexof()
var str1 = 'hello world'; var p = str1.indexOf('l'); console.log(p); //2 p = str1.indexOf('l', 3); console.log(p); //3 p = str1.indexOf('l', 4); console.log(p); //9 p = str1.indexOf('llo'); console.log(p); //2 p = str1.indexOf('lloo'); console.log(p); //-1
Note: in the parameter of indexof(), it can be a single character. If it is found, it will return to its first position, and if it is not found, it will return - 1.
2,charAt()
var str = 'hello world'; var c = str.charAt(7); console.log(c); //o c = str.charAt(-1); console.log(c); // No output, no error c = str.charAt(20); console.log(c); // No output, no error
Note: when the specified index value is not within the range of the string, there will be no error, but there will be no output.
3,concat()
var str = 'hello'; var s = str.concat(' world', '!'); console.log(s); //hello world!
be careful:
(1) Using concat() to splice strings is the same as using a plus sign to splice strings, but concat() is a little more elegant.
(2) However, it should be avoided in development, especially in loops (memory occupation).
4,substring()
var str = 'charset'; console.log(str); var s = str.substring(1); // harset intercepts the character with subscript 1 to the end of the string console.log(s); s = str.substring(1, 5); console.log(s); //hars left closed right open s = str.substring(1, 3); console.log(s); // ha s = str.substring(3, 1); console.log(s); // ha equals s = str.substring(1, 3); s = str.substring(-1); console.log(s); //If the first parameter given is a negative number, it will copy the string and return it.
explain:
(1) If only the first parameter is specified, the substring will run from the specified position to the end;
(2). If both parameters are specified, the substring will be the character from the start position to the end position; (left closed right open)
5,substr()
var str = 'charset'; console.log(str); var s = str.substr(1, 2); console.log(s); //ha s = str.substr(4, 2); console.log(s); //se truncates 2 digits from the index number of 4 s = str.substr(2); console.log(s); // arset intercepts to the end s = str.substr(-2); console.log(s); // et return intercept
be careful:
(1) The parameter of this method is how many characters to intercept from that position.
(2) If both parameters are specified, characters of the specified length are intercepted from the specified position to form a new word
6,slice()
var str = 'The morning is upon us.'; console.log(str); var s = str.slice(5); console.log(s); //orning is upon us. s = str.slice(5, 9); console.log(s); //orni s = str.slice(5, -3); console.log(s); //orning is upon s = str.slice(-1, 5); console.log(s); //No output content
explain:
(1) The function of this method is similar to that of substr(), which is used to intercept substrings.
(2) If only the first parameter is specified, it will go from the specified position to the end of the original string.
(3) If two parameters are specified, characters from the specified position to the end position are extracted.
(4) If the second parameter is a negative number, the character from the specified position to the end of the number from the back to the front is extracted.
(5) If the first parameter is negative, there is no output and no error will be reported.
7,replace()
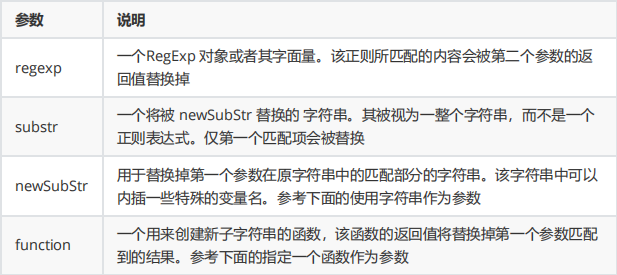
var str = 'Twas the night before Xmas...'; console.log(str); // Replace the letter e with, var s = str.replace('e', ','); console.log(s); //Twas th, night before Xmas... Only one was replaced s = str.replace(/\s+/ig, '-'); console.log(s); //Twas-the-night-before-Xmas... Replaced all spaces
be careful:
8,replaceAll()
var str = 'TwAs the night before Xmas...'; console.log(str); var s = str.replaceAll('as', ','); console.log(s); //TwAs the night before Xm,... s = str.replaceAll(/\s+/g, '-'); console.log(s); //TwAs-the-night-before-Xmas... s = str.replaceAll(/as/ig, ','); console.log(s); //Tw, the night before Xm,...
be careful:
(1) The replaceAll() method will replace all the characters found;
(2) If you want to use regular expressions in the replaceAll() method, you must add the global matching parameter (/ g).
9,split()
var str = "Hello World How are you doing"; console.log(str); var arr = str.split(' '); console.log(arr); arr = str.split(/\s+/); console.log(arr); str = "Hello,World,How,are,you,doing"; console.log(str); arr = str.split(','); console.log(arr);
explain:
(1) The split() method will cut the string according to the specified separator to generate an array;
(2) The split() method supports regular expressions for cutting.
10,trim()
var str = ' char set '; console.log(str); var s = str.trim(); console.log(s); //char set has no spaces before and after, and the spaces in the middle cannot be removed
Note: only the spaces before and after can be deleted, and the spaces in the middle cannot be removed.
11,match()
The match() method retrieves and returns the result of a string matching the regular expression.
var str = 'For more information, see Chapter 3.4.5.1'; console.log(str); // Define a regular expression var regex = /see (chapter \d+(\.\d)*)/i; var flag = str.match(regex); console.log(flag);