December 25, 2021
1, Algorithm problem
(1) Output max min
1. Compare the three numbers and output the maximum number
var a =1; var b= 2; var c =3; if (a > b) { if (a > c) { console.log(a); } else { console.log(c); } } else { if (b > a) { if (b > c) { console.log(b); } else { console.log(c); } } }
2. Enter three numbers and print out the largest of the three numbers:
Method 1: if else judgment:
var a = prompt('Please enter the first number'); var b = prompt('Please enter the second number'); var c = prompt('Please enter the third number'); if (a > b) { if (a > c) { alert(a); } else { alert(c); } } else { if (b > a) { if (b > c) { alert(b); } else { alert(c); } } }
Method 2: input three values circularly and output the maximum value
var Max=0; var a; for(var i = 0;i<3;i++){ a =prompt('Please enter') if(a>Max){ Max=a; } } alert(Max);
3. Import a string of data and output the (or minimum) value of the data
function fn() { var max = 0; var a; for (var i = 0; i < arguments.length; i++) { if (arguments[i] > max) max = arguments[i] } console.log(max); } fn(1, 2, 3, 4, 6, 8, 0)
4. The user inputs the number of numbers in the input box, and then manually circularly inputs a series of numbers to output the maximum value
var nums = prompt("Please enter the number of numbers:") var arr = [] var num for (var i = 0; i < nums; i++) { num = prompt(`Please enter page ${i + 1}number`) arr.push(parseInt(num)) } console.log(arr); function fn(arr) { var max = arr[0]; var a; for (var i = 0; i < arr.length; i++) { if (arr[i] > max) max = arr[i] } alert(`The maximum value is ${max}`) } fn(arr)
5. Randomly copy a string of data, such as 1, 2, 56, 89560... And enter the input box to output the maximum or minimum value
Method 1:
let res = prompt("input,Comma separated"); console.log(res.split(',')); console.log(Math.max.apply(null, res.split(',')));
Method 2: array
var a = prompt("Please enter the number to compare") arr = a.split(" ") var arr2 = [] for (var i = 0; i < arr.length; i++) { arr2.push(parseInt(arr[i])) } function fn(arr2) { var max = arr2[0]; var a; for (var i = 0; i < arr2.length; i++) { if (arr2[i] > max) max = arr2[i] } alert(`The maximum value is ${max}`) } fn(arr2)
(2) Output prime
//Print prime numbers within 100 //The idea of thinking is that only the prime number i is divided by j (the number from 1 to i) twice. More than two times is not prime var count = 0; for (var i = 2; i < 100; i++) {//1 is not a prime number for (var j = 1; j <= i; j++) { //Because it is necessary to judge 1 and itself, 1 = < J < = I if (i % j == 0) { count++ //c is the number of divisions } } if (count == 2) { console.log(i); } count = 0;//You have to clear c because you have to remember how many times it is divided in each cycle }
2, Some problems
tcp shook hands three times and waved four times
The difference between tcp and udp
Which layer is the http protocol and which layer is the tcp protocol
OSI seven layer model
Let's talk about several design patterns
css animate
Handwritten url parameter parsing
What does HTTP only mean
css selector priority
eventbus principle
vue. What happened
One way data flow of Vue
What is the difference between action and mutation in Vuex
3, Reference value
(1) Array
var arr = [1, 2, 3, 4, 5, 6, undefined, null]; // (standard writing: there is a space after each comma) console.log(arr[5]);//6
1. Assign a value to the array
arr[3]=null; console.log(arr[3]);//null
2. Find the length of the array
console.log(arr.length);//6
3. Loop out the data in the array
for(var i = 0;i < arr.length;i++){ console.log(arr[i]); }
4. Cyclic output assignment
for(var i = 0 ;i < arr.length;i++){ arr[i]+=2; console.log[arr[i]]; }
==Note: = = when console Log [arr] the following figure will appear in the for loop,
Therefore, when outputting the data in the array circularly, it is best to put console Log [arr] outside for {}
Or console Log (arr [i]) is placed in for {}
for (var i = 0; i < arr.length; i++) { //Array maximum subscript = array length - 1 console.log(arr); }
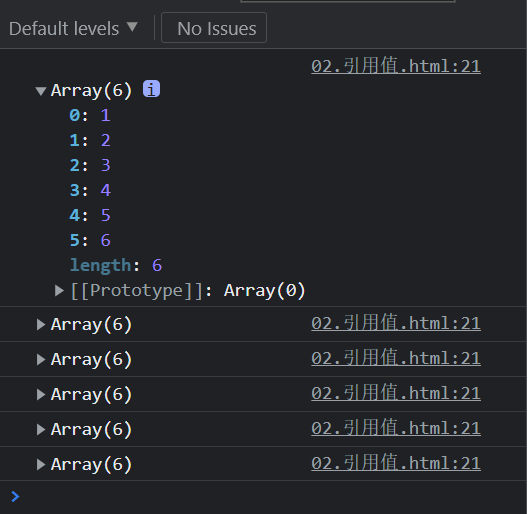
(2) object
1. Attribute name (key name): attribute value (key value),
var person = { name: 'sz',//Attribute name / key name: attribute value / key value, age: 23, height: 175, weight: 140, job: 'web Development Engineer' }
2. Assignment
person.name='ym';//Assign a value to an attribute console.log(person.name);//From sz to ym
(4)function
(5) Regexp (regular)
4, typeof
(1) Data type
console.log(typeof ('123'));//string console.log(typeof (123));//number console.log(typeof (true));//boolean
(2)object
1. The following object is not a specific declared object. It represents a reference type
Both object and array belong to large object (reference type)
console.log({});//object console.log(typeof ([]));//object
2. Think about why the following is an object,
Isn't null the original value (the null value should return false), how does it become a reference type?
console.log(typeof (null));//object
Reason: null is a bug. When it first came out, it was created to specify an empty object. In fact, it refers to a pointer to an empty object and a placeholder for an empty object
In fact, it was first described as a reference type. However, when es6 proposed in early 2014 and 2015, many people said to change null to null, but ECMA rejected it.
Why refuse? The reason is: This is a legacy of history. It is impossible es6 to require everyone to change the js of the browser, including all the code. It's very hard.
(3)undefined
console.log(typeof (undefined));//undefined;
(4)function
console.log(typeof (function () {}));//function
5, Data type conversion
(1) Implicit type conversion
console.log(typeof("1"-"1"));//number
Test site:
console.log(a);//report errors console.log(typeof(a));//Undefined undefined
Any typeof(typeof()) output is of type string
console.log(typeof(typeof(a)));//string console.log(typeof (typeof (123)));//string
(2) Display type conversion
1.Number()
var a = '123'; console.log(Number(a) + '-' + typeof (Number(a)));//123-number var a = true; console.log(Number(a) + '-' + typeof (Number(a)));//1-number var a =null; Number(a)//Convert a to 0 console.log(Number(a) + '-' + typeof (Number(a)));//0-number var a = undefined; Number(a)//a to Nan Nan number var a = 'a'; Number(a);//Convert to NAN var a = '1a'; Number(a);//Convert to Nan Nan number var a ='3.14'; Number(a);//3.14 3.14-number console.log(Number('120px'));//Nan, praseint ('120px ') output is 120 //Any type in Number() that has nothing to do with numbers will become NAN
2.parseInt
Why not convert true to 1 like number (), but NAN?
var a = true; console.log(parseInt(a) + ' - ' + typeof (parseInt(a)));//NAN-number
Reason: parseInt, no matter how much, only wants to be converted into an integer, so it must be related to numbers. (it only knows numbers) false is the same
And Number() is a boolean type. Anything that has nothing to do with numbers will become NAN, such as 120px with units
var a = null; console.log(parseInt(a));//NAN var a = undefined; console.log(parseInt(a))//NAN
Binary conversion: where a represents 1 0; (to the power of 16 + 0)
var a = '10'; console.log(parseInt(a, 16));//The output 16 represents the conversion from hexadecimal to hexadecimal
be careful:
Why does NAN return the number type?
Explanation:
1. Because the data type of NaN is number, that is, number; He is a special number. Because data types in js can be converted to each other, it is convenient for other data types to be converted to number (non number conversion to number type is NaN)
2. The Nan attribute is a special value that represents a non numeric value. This property indicates that a value is not a Number.NaN is under the Number object, that is, Number NaN. It represents a special non numeric value and is also a type of Number.