1, What is BOM
BOM (Browser Object Model) is the interface between JS and browser window.
Some special effects related to browser size change and scroll bar scrolling need the help of BOM technology.
2, window object
The window object is the window where the current JS script runs, and this window contains the DOM structure, window The document attribute is the document object.
In the browser with tag function, each tag has its own window object; In other words, the tabs of the same window will not share a window object.
3, Global variables are attributes of window
The global variable becomes a property of the window object.
var a = 10; console.log(window.a == a); // true
This means that multiple js files share the global scope, that is, js files have no scope isolation function.
4, Built in functions are generally window methods
Built in functions such as setInterval() and alert() are generally window methods.
console.log(window.alert == alert); // true console.log(window.setInterval == setInterval); // true
5, Window size related properties
attribute | significance |
---|---|
innerHeight | The height of the content area of the browser window, including the horizontal scroll bar, if any |
innerWidth | The width of the content area of the browser window, including the vertical scroll bar, if any |
outerHeight | The external height of the browser window |
outerWidth | The outer width of the browser window |
To get the window width without scroll bars, use:
document.documentElement.clientWidth
The outer width of the browser refers to the width of the browser window border.
When the browser window is full screen: outer width of browser = = inner width of browser (including scroll bar)
When the browser window is not full screen: Browser outer width > browser inner width (including scroll bar)
6, resize event
After the window size changes, the resize event will be triggered. You can use window Onresize or window Addeventlistener ('resize ') to bind the event handler.
7, Rolled height
window. The scrolly property indicates the pixel value that has been scrolled in the vertical direction.
document. documentElement. The scrolltop property also indicates the window scroll height.
// The height of the window scrolling can be obtained in this way var scrollTop = window.scrollY || document.documentElement.scrollTop;
- document.documentElement.scrollTop is a value that can be given manually to jump to any specified rolling height
- window.scrollY is read-only and cannot be given a value manually
8, scroll event
After the window is scrolled, the scroll event will be triggered. You can use:
window.onscroll or window Addeventlistener ('scroll ') to bind the event handler.
9, Navigator object
window. The navigator property can retrieve the Navigator object, which contains the relevant properties and identification of the browser of the user's activity.
attribute | significance |
---|---|
appName | Browser official name |
appVersion | Browser version |
userAgent | Browser user agent (including kernel information and shell information) |
platform | User operating system |
[case]
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <script> console.log('Browser brand', navigator.appName); console.log('Browser version', navigator.appVersion); console.log('user agent ', navigator.userAgent); console.log('operating system', navigator.platform); </script> </body> </html>
10, Identify user browser brand
Navigator is usually used to identify the brand of the user's browser Useragent properties.
var sUsrAg = navigator.userAgent; if (sUsrAg.indexOf("Firefox") > -1) { } else if (sUsrAg.indexOf("Opera") > -1) { } else if (sUsrAg.indexOf("Edge") > -1) { } else if (sUsrAg.indexOf("Chrome") > -1) { } else if (sUsrAg.indexOf("Safari") > -1) { } else { }
11, History object
window. The history object provides an interface to manipulate the browser session history.
The common operation is to simulate the browser fallback button.
history.back(); // Equivalent to clicking the browser's back button history.go(-1); // Equivalent to history back();
[case]
- temp.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <h1>I am temp Webpage</h1> <a href="history method.html">Go see history Method page</a> </body> </html>
- history.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <h1>I am history Method web page</h1> <button id="btn">Back off</button> <!-- Links can use infills javascript Script mode --> <a href="javascript:history.back();">Links: fallback</a> <script> var btn = document.getElementById('btn'); btn.onclick = function () { // history.back(); history.go(-1); }; </script> </body> </html>
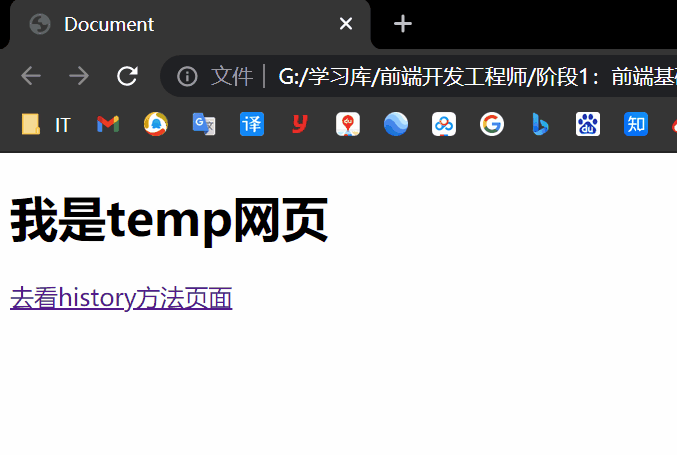
12, Location object
window.location identifies the current web address. You can command the browser to jump to the page by assigning a value to this attribute.
window.locaiton = 'http://www.imooc.com'; window.location.href = 'http://www.imooc.com';
13, Reload current page
You can call the reload method of location to reload the current page. The parameter true means to force the load from the server.
window.location.reload(true);
[case]
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <button id="btn1">I'll go to class at seven</button> <button id="btn2">Refresh</button> <script> var btn1 = document.getElementById('btn1'); var btn2 = document.getElementById('btn2'); btn1.onclick = function () { window.location = 'http://www.imooc.com'; }; btn2.onclick = function () { window.location.reload(true); }; </script> </body> </html>
14, GET request query parameters
window. location. The search property is the GET request query parameter of the current browser.
For example, website: https://www.imooc.com/?a=1&b=2
console.log(window.location.search); // "?a=1&b=2"
The details of GET and POST are introduced in Ajax.
15, BOM special effects development
15.1 back to top button making
Return to the top principle: change document documentElement. Scrolltop property. If this value is changed step by step through the timer, it will be returned to the top in the form of animation.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> body { height: 5000px; background-image: linear-gradient(to bottom, rgb(255, 0, 149), rgb(7, 185, 255), rgb(0, 255, 76)); } .backtotop { width: 60px; height: 60px; background-color: rgba(255, 255, 255, .6); position: fixed; bottom: 100px; right: 100px; /* Small hand */ cursor: pointer; } </style> </head> <body> <div class="backtotop" id="backtotopBtn">Back to top</div> <script> var backtotopBtn = document.getElementById('backtotopBtn'); var timer; backtotopBtn.onclick = function () { // Set the meter to close first clearInterval(timer); // set timer timer = setInterval(function () { // Continuously reduce scrollTop document.documentElement.scrollTop -= 200; // The timer must stop if (document.documentElement.scrollTop <= 0) { clearInterval(timer); } }, 20); }; </script> </body> </html>
15.2 small effect of floor navigation
DOM elements have an offsetTop attribute, which indicates the vertical distance from this element to the positioning ancestor element.
Locate ancestor element: in the ancestor, the element closest to itself and having the location attribute.
That is, the offsetTop attribute can get the distance between the element and the top of the nearest ancestor element with positioning.
If no ancestor has a location, you can directly get the distance value of the element from the top of the page.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> * { margin: 0; padding: 0; } .content-part { width: 1000px; margin: 0px auto; margin-bottom: 30px; background-color: #ccc; font-size: 50px; } .floornav { position: fixed; right: 40px; top: 50%; margin-top: -100px; width: 120px; height: 200px; background-color: orange; } .floornav ul { list-style: none; } .floornav ul li { width: 120px; height: 40px; line-height: 40px; text-align: center; font-size: 26px; /* Small hand pointer */ cursor: pointer; } .floornav ul li.current { background: purple; color: white; } </style> </head> <body> <nav class="floornav"> <ul id="list"> <li data-n="science and technology" class="current">science and technology</li> <li data-n="Sports">Sports</li> <li data-n="Journalism">Journalism</li> <li data-n="entertainment">entertainment</li> <li data-n="video">video</li> </ul> </nav> <section class="content-part" style="height:674px;" data-n="science and technology"> Science and technology column </section> <section class="content-part" style="height:567px;" data-n="Sports"> Sports column </section> <section class="content-part" style="height:739px;" data-n="Journalism"> News column </section> <section class="content-part" style="height:574px;" data-n="entertainment"> Entertainment column </section> <section class="content-part" style="height:1294px;" data-n="video"> Video column </section> <script> // Use event delegation to add listening to li var list = document.getElementById('list'); var contentParts = document.querySelectorAll('.content-part'); var lis = document.querySelectorAll('#list li'); list.onclick = function (e) { if (e.target.tagName.toLowerCase() == 'li') { // getAttribute means to get an attribute value on the tag var n = e.target.getAttribute('data-n'); // You can use the attribute selector (that is, the square bracket selector) to find content parts with the same data-n var contentPart = document.querySelector('.content-part[data-n=' + n + ']'); // Let the scroll of the page automatically become the offsetTop value of the box document.documentElement.scrollTop = contentPart.offsetTop; } } // After the page is loaded, push the offsetTop values of all content part boxes into the array var offsetTopArr = []; // Traverse all contentparts and push their net positions into the array for (var i = 0; i < contentParts.length; i++) { offsetTopArr.push(contentParts[i].offsetTop); } // For the last term to facilitate comparison, we can push an infinity offsetTopArr.push(Infinity); console.log(offsetTopArr); // Current floor var nowfloor = -1; // Scrolling of windows window.onscroll = function () { // Get the current window scroll value var scrollTop = document.documentElement.scrollTop; // Traverse the offsetTopArr array to see which two floors the current scrollTop value is between for (var i = 0; i < offsetTopArr.length; i++) { if (scrollTop >= offsetTopArr[i] && scrollTop < offsetTopArr[i + 1]) { break; } } // When exiting the cycle, i is the number, which indicates the current floor number // If the current floor is not i, it indicates that it is around the building if (nowfloor != i) { console.log(i); // Change the global variable to this floor number nowfloor = i; // Items with subscript i have cur for (var j = 0; j < lis.length; j++) { if (j == i) { lis[j].className = 'current'; } else { lis[j].className = ''; } } } }; </script> </body> </html>