What is expression language (EL)?
Expression language (EL) is a mechanism that simplifies storage in Java Accessibility of data in Bean components and other objects (such as requests, sessions, applications, etc.). There are many operators in JSP that are used in EL, such as arithmetic operators and logical operators to execute expressions. It was introduced in JSP 2.0
In this tutorial, you will learn-
- JSP syntax of expression language (EL)
- JSP If-else
- JSP Switch
- JSP For loop
- JSP simultaneous loop
- JSP operator
Syntax of JSP (EL)
Syntax of EL: $(expression)
- In JSP, anything that exists in braces is evaluated when it is sent to the output stream at run time.
- Expressions are valid EL expressions that can be mixed with static text and can be combined with other expressions to form larger expressions.
To better understand how expressions work in JSP, we will see the following example.
In this example, we will see how to use EL as an operator to add two numbers (1 + 2) and get the output respectively.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Guru JSP1</title> </head> <body> <a>Expression is:</a> ${1+2}; </body> </html>
Code Description:
- Line 11: the expression language (EL) is set where we add two numbers 1 + 2, so it will output 3.
When the above code is executed, it will have the following output.
Output:
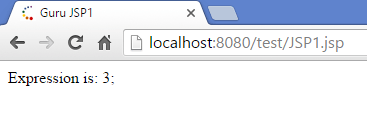
- The expression is: 3 (the number 1 + 2 will be added and used as output)
Flow control statement:
JSP provides the powerful function of Java and can be embedded into the application. We can use all API s and building blocks of Java in JSP programming, including control flow statements, including decision and loop statements.
Two types of flow control statements are described below;
- Decision statement
- Circular statement
Decision statement:
The decision statements in JSP are based on whether the condition set is true or false. The statement will run accordingly.
There are two types of decision statements as follows:
- If – otherwise
- switch
JSP If-else
The "if else" statement is the basis of all control flow statements. It tells the program to execute a part of the code only when the calculation result of a specific test is true.
This condition is used to test multiple conditions, whether they are true or false.
- If the first condition is true, execute "if block" and then
- If false, execute "else block"
if – syntax of else statement:
If(test condition) { //Block of statements } else { //Block of statements }
In this example,
We will test the "if else" condition by taking the variable and checking whether the value of the variable matches what it initializes:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Guru JSP2</title> </head> <body> <%! int month=5; %> <% if(month==2){ %> <a>Its February</a> <% }else{ %> <a>Any month other than February</a> <%} %> </body> </html>
Code Description:
- Line 10: the variable named month is initialized to 5 in the expression tag
- Code line 11: in the EL tag, "if condition" indicates that if the month is equal to 2 (the condition is tested as true or false here)
- Code line 12: if the condition is true, that is, the variable month is 2, it will print to the output stream
- Line 13-15: if the above if condition fails, it moves to the else section for all other cases where the statement will print to the output stream and the condition is closed
When the above code is executed, it will have the following output.
Output:
- Since our month is may, this is not equal to #2 (February). Therefore, we have the output "any month other than February" (the month is mentioned as 5, so other months are executed)
JSP Switch
The body of a switch statement is called a "switch block".
- Switch case is used to check the number of possible execution paths.
- Switches are available for all data types
- The switch statement contains multiple cases and a default case
- It evaluates the expression and then executes all statements after matching case
Syntax of switch statement:
switch (operator) { Case 1: Block of statements break; Case 2: Block of statements break; case n: Block of statements break; default: Block of statements break; }
- The switch block starts with a parameter, which is the operator to be passed, and
- Then, different conditions are provided, and the situation matching the operator will execute the situation.
In the following example,
We define a variable week, which matches the situation, whichever is true. In this example, the week is 2, so it is 2nd case matching, and the output is Tuesday:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Guru JSP3</title> </head> <body> <%! int week=2; %> <% switch(week){ case 0: out.println("Sunday"); break; case 1: out.println("Monday"); break; case 2: out.println("Tuesday"); break; case 3: out.println("wednesday"); break; case 4: out.println("Thursday"); break; case 5: out.println("Friday"); break; default: out.println("Saturday"); } %> </body> </html>
Code Description:
- Line 10: the variable named week is initialized to 2 in the expression tag
- Code line 11: in the EL tag, the switch condition starts, where the week is passed as a parameter
- Code line 12 – 29: from case 0 to case 5, all cases have been mentioned, where the value of the week parameter matches the case, so the output is printed. In this case, the value is 2, so case 2 will be executed in this case. "out" here is the JSP class, which writes the output stream for the generated response, and "println" is the method of this class.
- Line 30-32: if all of the above fails, it will move to the default section and execute, where the statement will print to the output stream and close the condition
When the above code is executed, it will have the following output.
Output:
- In this case, the output is Tuesday because the second case is called.
Circular statement
JSP For loop
It is used to iterate over elements with specific conditions and has three parameters.
- Variable counter initialized
- Condition until the loop must be executed
- The counter must be incremented
For loop syntax:
For(inti=0;i<n;i++) { //block of statements }
In this example,
We have a for loop that iterates until the counter is less than the given number:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Guru JSP4</title> </head> <body> <%! int num=5; %> <% out.println("Numbers are:"); for(int i=0;i<num;i++){ out.println(i); }%> </body> </html>
Code Description:
- Line 10: the variable named "num" is initialized to 5 in the expression tag
- Code line 11-14: in the EL tag, "out" is the class of JSP, "println" is the method output in the output stream, and the for loop starts. It has three parameters:
- The variable i is initialized to 0,
- The condition is given, where i is less than the local variable num,
- And i will increase every time the loop iterates.
In the body of "forloop", there is a kind of JSP, which uses the method println to print to the output stream. We are printing the variable i.
When the above code is executed, it will have the following output.
Output:
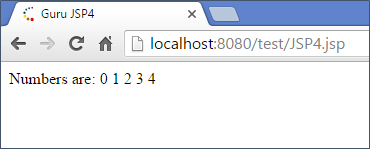
- The output number is 0 1 2 3 4. In this example, the condition we give is that the "for loop" must be executed until the counter is less than or equal to the variable. The number is 5, so the cycle will run from 0 to 4 (5 times). Therefore, output.
JSP simultaneous loop
It is used to iterate over elements, where it has one parameter of the condition.
Syntax:
While(i<n) { //Block of statements }
In this example,
We have a while loop that iterates to a day greater than the counter:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Guru JSP5</title> </head> <body> <%! int day=2; int i=1; %> <% while(day>=i){ if(day==i){ out.println("Its Monday"); break;} i++;} %> </body> </html>
Code Description:
- Code line 10: in the expression tag, the variable named i is initialized to 1 and day is 2
- Line 11-17: in the EL tag, "while loop" will iterate until we set a condition, as if the date is greater than or equal to i, and the variable will be true. (days > = i)
If "if condition" (day equals i) and "if condition" are true, it will print the output stream and exit the while loop. Otherwise, the i variable will be incremented and iterated.
When we execute the code, we will get the following output
Output is:
- The output of this code will be "its Monday".
JSP operator
JSP operators support most of their arithmetic and logical operators, which are supported by java in expression language (EL) tags.
Common operators are mentioned below:
Here are the operators:
. | Access Bean properties or map entries |
[] | Access array or List elements |
( ) | Group subexpressions to change the evaluation order |
+ | addition |
– | Minus or negative value |
* | multiplication |
/Or div | divide |
%Or mod | Modulus (remainder) |
==Or eq | Equality test |
!= Or ne | Inequality test |
< or lt | Test less than |
>Or gt | Test greater than |
< = or le | Test less than or equal to |
>=Or ge | Test greater than or equal to |
&&Or and | Test logic AND |
||Or or | Test logic OR |
! Still not | Unary Boolean complement |
empty | Test null variable value |
In this example,
- We declare two variables num1 and num2, and then take a variable num3, where we use the JSP operator + by to add num1 and num2 to get num3.
- Then, we use JSP operators (! =, >) to check whether num3 is not equal to 0, and then
- Then take another variable num4, multiply by two num1 and num2, and we get num4.
All these numbers should be printed out as our output:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Guru JSP6</title> </head> <body> <% int num1=10; int num2 = 50; int num3 = num1+num2; if(num3 != 0 || num3 > 0){ int num4= num1*num2; out.println("Number 4 is " +num4); out.println("Number 3 is " +num3); }%> </body> </html>
Code Description:
- Code line 10: in the expression tag, the variable named num1 is initialized to 10 and num2 is initialized to 50
- Line 11: the variable num3 is the sum of num1 and num2, where we use the addition operator
- We use the arithmetic operators Num3 and num3-12 to check whether the condition is greater than one line of the code. When any condition is true, OR will be used. In this case, it will enter "if case", where we multiply the two numbers "num1" and "num2" and get the output in "num4", which will print the output stream.
When the above code is executed, it will have the following output.
Output:
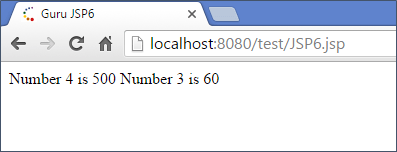
- The first output is the number 4 is 500 (the variable num4 is num1 * num2)
- The second output is the number 3 is 60 (variable num3, where num1+num2)
Summary:
- JSP expression language (EL) makes it easy for applications to access data stored in javabeans components.
- It also allows you to create expressions that are both arithmetic and logical.
- In EL tags, we can use integers. Floating point numbers, strings, and Booleans.
- In JSP, we can also use loops and decision statements using EL tags