Article directory
4 judgment and circulation
4.1 judgement
Judge
- Use flow chart
Judgment conditions and conditional statements
- The code implementation of the above flow chart:
if(score>50){ //Returns true if the condition is true document.write('Congratulations!'); //Return true to execute this statement }else{ //Otherwise (return false) document.write('Retest···'); //Execute this statement }
- Judgment condition: usually use comparison operator to compare two values, return true or false
- Conditional statement: Based on the concept of if (if) then (then) else (otherwise)
Comparison operators: judging conditions
- Operator
- ==Equal to: compare whether two values (number, string, boolean type) are the same
- ! = not equal to: compare whether two values (number, string, boolean type) are different
- ===Strictly equal to: compare two values and check whether the data type and value are identical
- ! = = strictly not equal to: compare two values and check whether the data type and value are completely different
- > greater than
- < less than
- >=Greater than or equal to
- < = less than or equal to
- Exceptions
- No value can be "treated as" true or false
- In short circuit judgment, the condition may not need to be executed
- Organize comparison operators
- Syntax: (operand comparison operand)
- Example: (score > = pass)
- Result of expression: a single value, here is true/false
- Syntax: (operand comparison operand)
- Use comparison operators
var pass=50; //Points passed var score=90; //User's score //Confirm whether the user passes the test var hasPassed= score>=pass; //Update the content and display the passing status on the page var el=document.getElementById('answer'); el.textContent='Adoption:'+hasPassed;
- Use expression:
- Operands can also be expressions, because the result of each expression is a single value
((score1+score2) > (highScore1+highScore2))
- Compare two expressions
var score1=90; var score2=95; var highScore1=75; var highScore2=95; //Check if the total score is higher than the highest score so far //When you assign a comparison result to a variable, you do not need to wrap it in the outermost level of parentheses var comparison = (score1+score2)>(highScore1+highScore2); //Show results on page var el=document.getElementById('answer'); el.textContent="Get the highest new score:"+comparison;
- Operands can also be expressions, because the result of each expression is a single value
Logical operators
-
Function: To compare the results of multiple comparison operators together
- Example: three expressions, each of which will get the result of true/false
((5<2) && (2>=3))
- Example: three expressions, each of which will get the result of true/false
-
Three logical operators
- And & &: detect multiple conditions
Expression Result true&&true true true&&false false false&&false false false&&true false - Or |: detect at least one condition
Expression Result false||false false true||true true true||false true false||true true - Non!: operate on a single boolean variable and negate it
Expression Result !false true !true false -
Short circuit condition
- Logical expressions are evaluated from left to right. If the first condition can provide enough information for the final result, it is unnecessary to calculate the subsequent conditions
- False & & any condition: the final result is false
- True | | any condition: the final result is true
-
Use logical operator example
- And & &
//Judge whether users pass the test twice var score1=8; var score2=8; var pass1=6; var pass2=6; //Check whether the user has passed the test twice, and save the result in the variable passBoth var passBoth = ((score1>=pass1)&&(score2>=pass2)); //Create message var msg='Two tests passed:'+passBoth; //Show pass through message on page var el=document.getElementById('answer'); el.textContent=msg;
- Or not!
//Judge whether the user has passed at least one test var score1=8; var score2=8; var pass1=6; var pass2=6; //Check whether the user has passed at least one test and save the result in the variable minPass var minPass = ((score1>=pass1)||(score2>=pass2)); //Create message: if you have passed at least once, you do not need to test again var msg='Do you want to test again:'+!(minPass); //Show pass through message on page var el=document.getElementById('answer'); el.textContent=msg;
- And & &
if statement
-
Function: judge the condition. If the result of the condition is true, execute the content in the subsequent code block (if the result is false, do not execute)
//Execute the {} inner statement if the score > = 50 condition is met if(score>=50){ congratulate(); }
-
Use
- Satisfied condition: execute {} inner statement
var score=75; var msg; if(score>=50){ msg='Congratulate!'; msg+='Go to the next step'; } var el=document.getElementById('answer'); el.textContent=msg;
- Example: code does not execute in the order it was written
//Another version of the above code var score=75; var msg; function congratulate(){ msg+='Congratulate!'; } if(score>=50){ congratulate(); msg+='Go to the next step'; } var el=document.getElementById('answer'); el.textContent=msg;
- Satisfied condition: execute {} inner statement
-
If else statement
Conditional result content of execution true First code block false Second code block if(score>=50){ congratulate(); //Code executed when result is true }else{ encourage(); //Code executed when the result is false }
- Use
var score=75; var pass=50; var msg; //Select the message content to display based on the score if(score>=50){ msg='Congratulations!'; }else{ msg='keep trying!'; } var el=document.getElementById('answer'); el.textContent=msg;
- Use
switch Statements
- Effect
- The switch statement begins with a variable called a branch value. Each case represents a condition. When the value in the condition matches the value of the variable, the subsequent statement is executed
-
break keyword: tell the JavaScript interpreter that the switch statement is finished
switch(level){ //level: Branch value case 'one': //If the string of level is "one", execute the code in the first case title='level 1'; break; case 'two': //If the level string is "two", execute the code in the second case title='level 2'; break; case 'three': //If the level string is "three", execute the code in the third case title='level 3'; break; default: //If none of the above is true, execute the code in default title='test'; break; }
- Use
//Display different information according to different levels of users var msg; //The msg variable is used to hold the message to be displayed var level=2; //The variable level contains a number indicating the user level, which is then used as a branch value //Display information according to different levels switch(level){ case 1: msg='first!'; break; case 2: msg='second!'; break; case 3: msg='third!'; break; default: msg='good luck!'; }
Compare if else and switch
-
if-else
- else not required
- When multiple if statements are used consecutively, even if a match has been found, each if statement will be detected by execution, so the efficiency is slow
-
switch
- Use default to handle all cases except cases
- If a match is found, execute the corresponding code, and then the break statement stops executing other branches. Compared with the if statement with the same function, the performance is better
Casts and weak types
-
Cast
- Meaning: if the data type used is different from the data type required by JavaScript, JavaScript will convert the data type behind it
- Example: ('1 '> 0), the string' 1 'will be converted to the number 1, making the expression result true
-
Weak type:
- JavaScript is called a weakly typed language because the data type of a value can change
- Be careful
- When detecting whether two values are equal, it is recommended to use more strict = =! = = instead of = =! = (you can detect both values and types at the same time)
-
True and false
- True: treated as true, can be treated as number 1 (almost all values outside the false table are true)
value describe var highScore = true; Traditional Boolean value true var highScore = 1; Non 0 digits var highScore = 'carrot '; Non empty string var highScore = 10/5; Number operation (result is not 0) var highScore = 'true'; String true var highScore = 'false'; String false var highScore = '0'; String 0 - False value: regarded as false, can be regarded as the number 0
value describe var highScore = false; Traditional Boolean value false var highScore = 0; Number 0 var highScore = ' '; Empty string var highScore = 10/'score'; NaN var highScore; Variable not assigned -
Detect equality and presence
-
Objects and arrays are treated as true values, so they are often used to determine whether elements in a page exist
if(document.getElementById('header')){ //Action after element found }else{ //Element not found, do other operations }
-
Consider cast
Expression Result General situation false == 0 true false === 0 false false == ' ' true false === ' ' false null,undefined
(not equal to any value except itself)undefined == null true undefined === null false null == false,undefined == false false null == 0,undefined == 0 false NaN
(not equal to any value)NaN == null false NaN == NaN false
-
-
Short circuit value
- Short circuit: logical operators operate from left to right, and "short circuit" (stop operation) occurs immediately when obtaining the determined result, but return the value of stop operation (not necessarily true/false)
- Example:
//If artist has a value, return the same value as artist var artist='rembrandt'; var artistA = (artist||'unkown'); //If artist is an empty string, return the string unkown var artist=''; var artistA = (artist||'unkown'); //If artist has no value, you can create an empty object var artist=''; var artistA = (artist||{});
//When the script executes to valueB of logical operation, the statement will be short circuited and execute the statement in {} var valueA=0; var valueB=1; //1 is considered true var valueC=2; if(valueA||valueB||valueC){ //Do something }
-
Recommendations for use
- In the "or" operation, put the most likely to return true in the first position; in the "and" operation, put the most likely to return false in the first position
- Put the most time-consuming judgment operation at the end
4.2 cycle
Three types: for, while, do while
-
for
- Function: run a piece of code for a specific number of times, and check the cycle conditions according to the counter
//When condition I < 10 is no longer true, the loop ends and the script continues to execute subsequent code for(var i=0;i<10;i++){ //() is a counter, which is used to calculate how many times the cycle needs to be carried out document.write(i); //Code executed during loop }
- Cycle counter (take var I = 0; I < 10; I + + as an example)
- Initialize var i=0;
- Condition i<10;
- Update i++
- Use:
- Often used to traverse items in an array
//Save the scores of each round of tests in the array scores var scores=[24,32,17]; var arrayLength=scores.length; //Get the total number of array entries through the length property of the array var roundNumber=0; //What is the current round of the recording test var msg=''; var i; for(i=0;i<arrayLength;i++){ roundNumber=i+1; msg+='The first'+roundNumber+'Round results:'+scores[i]; } document.getElementById('answer').innerHTML=msg;
- Often used to traverse items in an array
- Function: run a piece of code for a specific number of times, and check the cycle conditions according to the counter
-
while
- Function: it is used when you do not know how many times the code needs to be cycled. If the judgment condition is true, the corresponding code will be run repeatedly
- Use:
//Equation showing multiplication of 1-9 and 5, multiplication table of output: 5 var i=1; var msg=''; while(i<10){ msg += i+'x5='+(i*5)+'<br/>'; i++; } document.getElementById('answer').innerHTML=msg;
-
do while
- Function: similar to the while loop, but in the do while loop, even if the condition returns false, the corresponding code will run at least once
- Use:
//Even if i=1 does not meet the I < 1 condition, the loop will execute once, output: 1x5=5 var i=1; var msg=''; do{ msg += i+'x5='+(i*5)+'<br/>'; i++; }while(i<1);
Important concepts
- Keyword
- break: causes the loop to end and tells the interpreter to continue executing subsequent code outside the loop body
- continue: tells the interpreter to execute the next iteration of the loop immediately, and then checks the condition (if it returns true, execute again)
- Loops and arrays: loop through the same code for each entry in the array
- Performance considerations:
- When the browser encounters a JavaScript script, it stops all its work until the script runs
- Any variable that can be assigned outside the loop and will not be changed during the loop should be assigned outside the loop
4.3 example
example4.html file
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>example4</title> </head> <body> <section id="page"> <h1>This is the addition table!</h1> <section id="blackboard"></section> </section> <script src="example4.js"></script> </body> </html>
example4.js file
//Use judgment and loop to display the addition table or multiplication table of a given number var table=3; var operator='addition'; //Add by default var i=1; var msg=''; if(operator==='addition'){ //Addition table while(i<11){ msg += i+'+'+table+'='+(i+table)+'<br/>'; i++; } }else{ //Multiplication table while(i<11){ msg += i+'x'+table+'='+(i*table)+'<br/>'; i++; } } var el=document.getElementById('blackboard'); el.innerHTML=msg;
Operation result
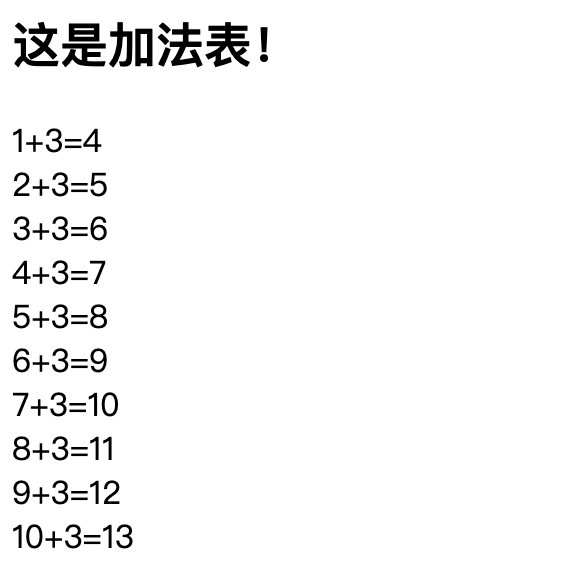