[JUnit] JUnit 5 Foundation 4 - Maven integration, parametric test, repeated test [End]
Previous series:
- [JUnit] JUnit5 foundation 1 - use of junit5 structures and assertions
- [JUnit] JUnit5 foundation 2 - lifecycle annotation and DisplayName annotation
- [JUnit] JUnit 5 Foundation 3 - dependency injection, assume, enable / disable testing and nested testing
Maven integration
Maven is an automatic management tool. Its main purposes are:
- Describe how software should be built
- Describes the dependencies of the project
Maven uses XML files for project management. The general entry file name is pom.xml.
I wrote notes before the steps of integrating Maven with VSCode: [VSCode] Java JDK/JRE configuration and Maven initialization project , I won't repeat it here.
Junit5 dependency
To run Junit5, Maven must configure at least the following three dependencies:
-
junit-jupiter-api
Defines Junit's API
-
junit-jupiter-engine
Test case for executing Junit
-
maven-surefire-plugin
Used to run Junit when maven components
Also, Junit 5 needs more than JAVA8 to run. If you want to be compatible with Junit 4, you need to use additional dependencies. Individual configurations are relatively troublesome. Junit also provides an aggregator: JUnit Jupiter (Aggregator) , the aggregator integrates other Junit dependencies required, including:
- junit-jupiter-api
- junit-jupiter-params
- junit-jupiter-engine
- junit-bom
Parametric test
In addition to the three basic dependencies, the JUnit Jupiter params dependency is also recommended for parametric testing JUnit Jupiter (Aggregator) The reason for this.
Parametric testing using @ ValueSource
@ValueSource will pass a set of arrays to @ parametrizedtest. The parameter types it accepts include:
- short
- byte
- int
- long
- float
- double
- char
- java.lang.String
- java.lang.Class
Suppose a class here contains a function to test whether an integer is odd. The test method is as follows:
package com.example; import static org.junit.jupiter.api.Assertions.assertTrue; import org.junit.jupiter.api.Test; import org.junit.jupiter.params.ParameterizedTest; import org.junit.jupiter.params.provider.ValueSource; public class OddNumberTest { @ParameterizedTest @ValueSource(ints = {1,3,5,7,10}) @Test void testOddNumber(int number) { OddNumber oddNum = new OddNumber(); assertTrue(oddNum.isOdd(number)); } }
The operation results are as follows:
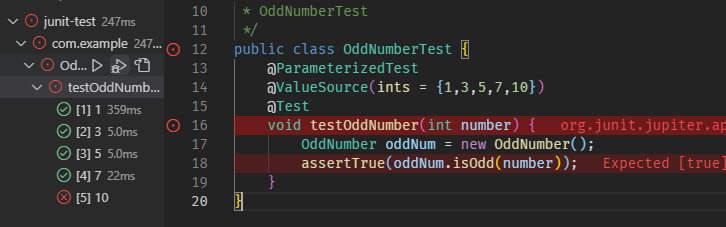
You can see that the odd number of test cases passed, but the even number of test cases failed.
Parametric testing using @ EnumSource
@EnumSource accepts a set of enum instances, such as:
package com.example; public enum ChessPiece { QUEEN, KING, KNIGHT, PAWN, ROOK, }
Test case:
package com.example; import static org.junit.jupiter.api.Assertions.assertNotNull; import org.junit.jupiter.params.ParameterizedTest; import org.junit.jupiter.params.provider.EnumSource; public class EnumTest { @ParameterizedTest @EnumSource(ChessPiece.class) void testEnum(ChessPiece piece) { assertNotNull(piece); } }
Operation results:
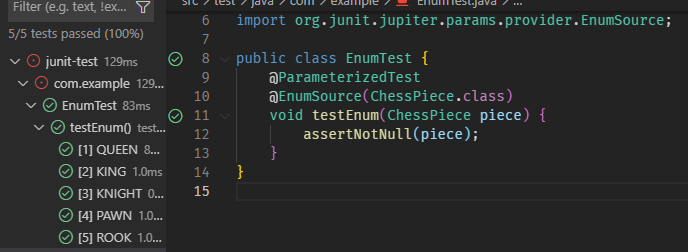
Personally, I think enum is very helpful for testing supported by multiple databases.
Parametric testing using @ MethodSource
@MethodSource supports the use of factory method for value transfer. Note that the factory method must be a static method, such as:
package com.example; import static org.junit.jupiter.api.DynamicTest.stream; import java.util.stream.Stream; import org.junit.jupiter.params.ParameterizedTest; import org.junit.jupiter.params.provider.MethodSource; public class MethodTest { @ParameterizedTest @MethodSource("paramProvider") void testMethodSource(String arg) { System.out.println(arg); } static Stream<String> paramProvider() { return Stream.of("para1", "para2"); } }
Operation results:
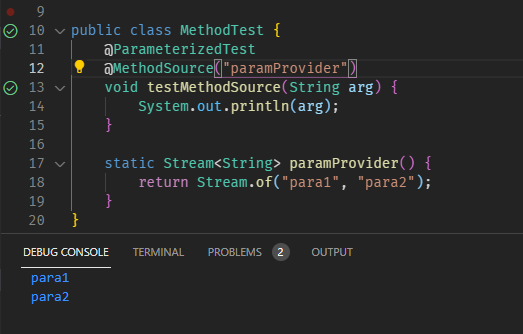
Parametric testing using @ CsvSource
@CsvSource supports passing in a set of comma separated key value pair data, such as:
package com.example; import org.junit.jupiter.params.ParameterizedTest; import org.junit.jupiter.params.provider.CsvSource; public class CsvMethod { @ParameterizedTest @CsvSource({"one, 1", "two, 2", "'test, test', 10"}) void testCsvSource(String first, int second) { System.out.println(first + ", " + second); } }
The operation results are as follows:
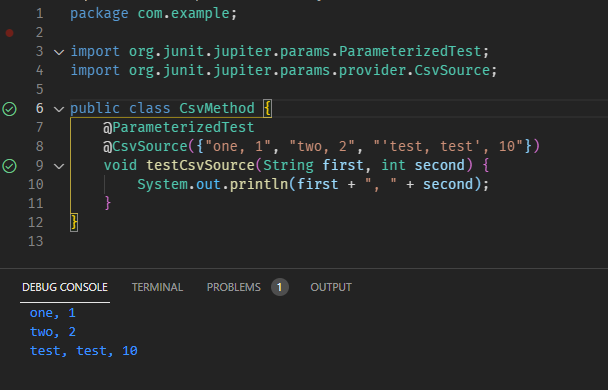
Parametric testing using @ CsvFileSource
@CsvFileSource is almost the same as @ CsvFileSource, except that @ CsvFileSource accepts the path address of a CSV file.
Repeat test
Many projects will be stress tested before going online. The method of repeated testing in JUnit 5 is also very simple:
package com.example; import org.junit.jupiter.api.DisplayName; import org.junit.jupiter.api.RepeatedTest; public class RepeatedTestExample { @RepeatedTest(name = "{displayName} - {currentRepetition}/{totalRepetitions}", value = 5) @DisplayName("Repeated Test") public void simpleRepeatedTest() { System.out.println("hello world"); } }
Operation results:
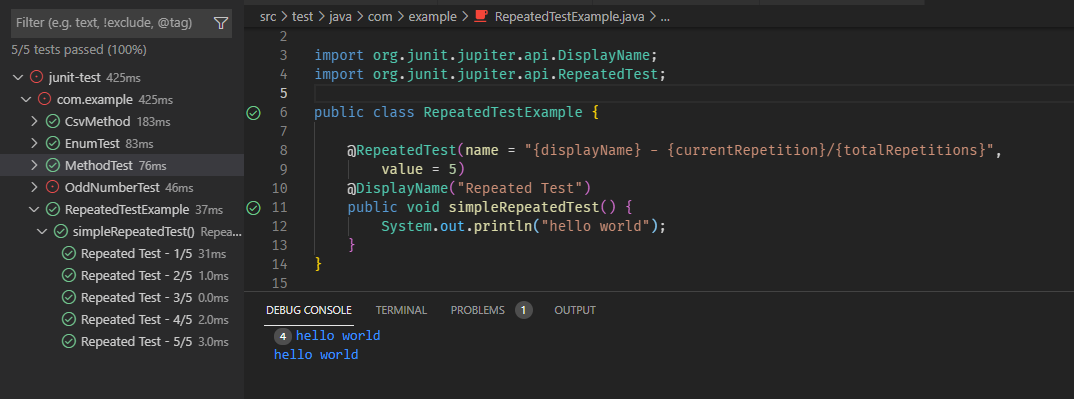
Note that using the keywords displayName, currentrepetition and totalrepetitions can easily identify the currently running tests.