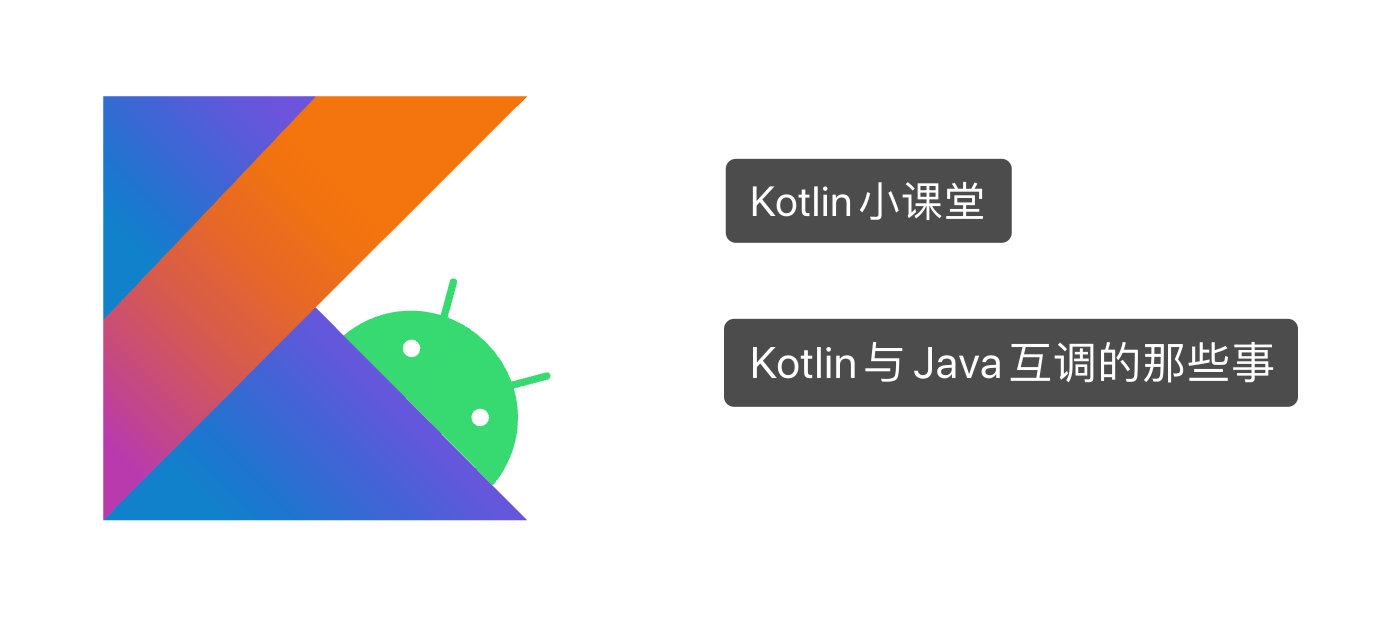
Kt call - handling of non null Java parameters
@NotNull
Java
class TestJava { public void toNotNull(@NotNull String title) {} public void toNull(String title){} }
Call in Kotlin

KT call - variables and methods declared in Java using KT keyword
If you call the java method in kotlin, if you have a Kotlin keyword, you must add an anti quotation mark.
Java
public Object object; //Using keyword naming method in kotlin public void is() { }
Call in Kotlin
testJava.`is`() testJava.`object`
Kt calls Java Sam conversion
When you call a method with interface parameters in Kotlin, if this interface has only one method, you can implement SAM conversion through lambda expression, thus simplifying our code.
Examples are as follows
Java
public class TestJavaSam { void singleFun(@NotNull IListener iListener) {} void noParameter( @NotNull IListener iListener,int sum) {} void noParameterClean(int sum,@NotNull IListener iListener) {} } interface IListener { void onClick(); }
Call in Kotlin
fun main() { val sam = TestJavaSam() sam.singleFun { } sam.noParameter({ //If you change the order of java method parameters, it will be more concise, as follows },123) sam.noParameterClean(123){ } }
Java is prohibited from calling a method in Kt
@JvmSynthetic
In Kotlin, some methods do not want to be exposed to Java calls, so you can add this annotation to the method.
@JvmSynthetic fun toMain() { }
At this point, toMain() cannot be called in Java.
Java calls Kt extension function
@file:JvmName("xx")
When using Kotlin's extension function in java, we will use the corresponding class name + Kt to call relevant methods. Sometimes it is a little troublesome for us to customize the corresponding tool class, as follows:
For example, we have a top-level extension function located in uiexpand KT:
fun Int.px() {}
Call in Java
//Java calls the extension method of kotlin class - (UiExpand)-Int.px() UiExpandKt.px(20);
As shown above, when we call in Java, we must have the filename +kt suffix before it can be called.
By giving uiexpand By adding @ file:JvmName("Ui") to the package name of KT, we can realize the custom generated class name to call
as follows Ui.px()
Java call kt member variable
@JvmField
In Java, when we call the member variables of Kotlin, the compiler will automatically generate the corresponding get and set methods for us, which is very consistent with the writing method of Java Bean, but some of them are. We just want to call them directly, so we can do so at this time.
Kotlin
data class TestKotlinBean( @JvmField val message: String, @JvmField val title: String)
Call in **Java
TestKotlinBean testKotlinBean = new TestKotlinBean("",""); String message = testKotlinBean.message; String title = testKotlinBean.title;
Of course, for the following examples, even without adding the above annotation, they can be called directly in java without getting and set.
lateinit var sum: String object UserPicCache{ const val KEY_CACHE = "CACHE" }
@set:JvmName
@get:JvmName
Sometimes, we just want it to generate one of the set or get methods. What should we do at this time?
Kotlin
data class TestKotlinBean( @set:JvmName("setMessage") var message: String, @get:JvmName("getTitle") val title: String )
Call in Java
TestKotlinBean testKotlinBean = new TestKotlinBean("", ""); testKotlinBean.setMessage("message"); testKotlinBean.getTitle();
Kt companion object of Java call
@JvmStatic
When we call the method or variable of Kotlin associated object in Java, we must pass the class name. Companion.xx can be called. At this time, we can increase
@JvmStatic to call directly.
Kotlin
class Log { companion object { var time: String = "" fun toLog() { } } }
Call in Java
ToLog.toLog(); ToLog.getTime();
However, it should be noted that @ JvmStatic does not improve the performance, because accordingly, the compiler generates another static method. For variable variables, it will generate two static methods, set and get.
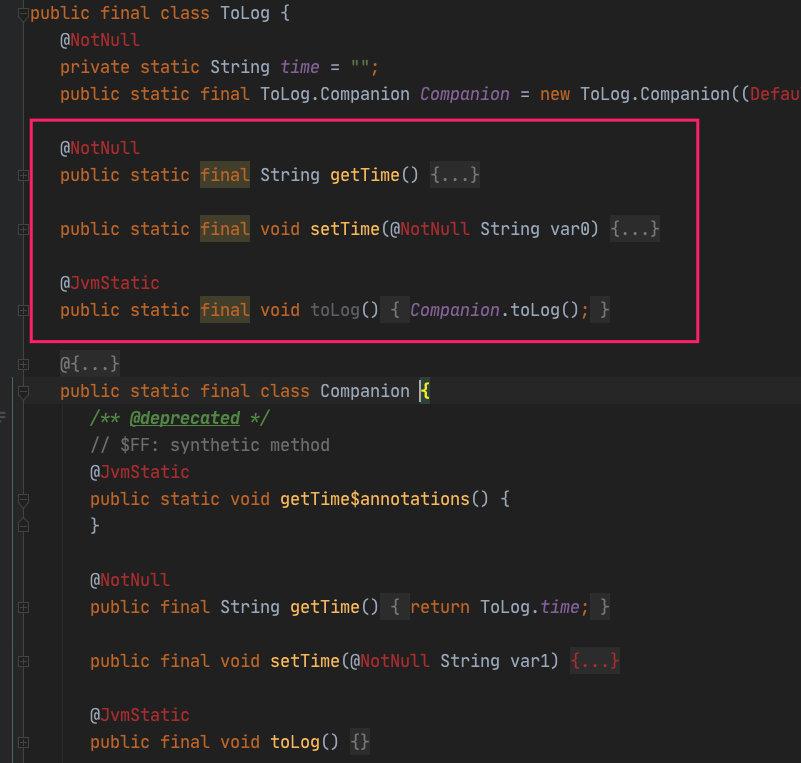
Default parameter value of Kt method called by Java
@JvmOverloads
In Kotlin, we may add some default values for method parameters for better use, but in Java, if the corresponding method parameters are not passed during the call, an error will be prompted. For this use, you can use @ JvmOverloads to modify the method.
kotlin
object DialogUtils { @JvmStatic @JvmOverloads fun showPromptDialog(title: String = "Tips") { } }
Call in java
DialogUtils.showPromptDialog(); DialogUtils.showPromptDialog("title");
See video:
Google developers - how to interoperate between Java and Kotlin