The builder annotation in lombok essentially generates a constructor builder class for you. Through this class, we can construct objects with this annotation. In essence, it implements a classic design pattern: builder pattern
1. Recognize:
① In a word: encapsulate the construction process of a complex object and construct it step by step. Because objects need to be built step by step, it is called the builder pattern.
② Encapsulating and decomposing the construction process of complex products into different methods makes the creation process very clear and allows us to more accurately control the creation process of complex product objects. At the same time, it isolates the creation and use of complex product objects, so that the same creation process can create different products. However, if the internal changes are complex, there will be many construction classes.
2.UML class diagram:
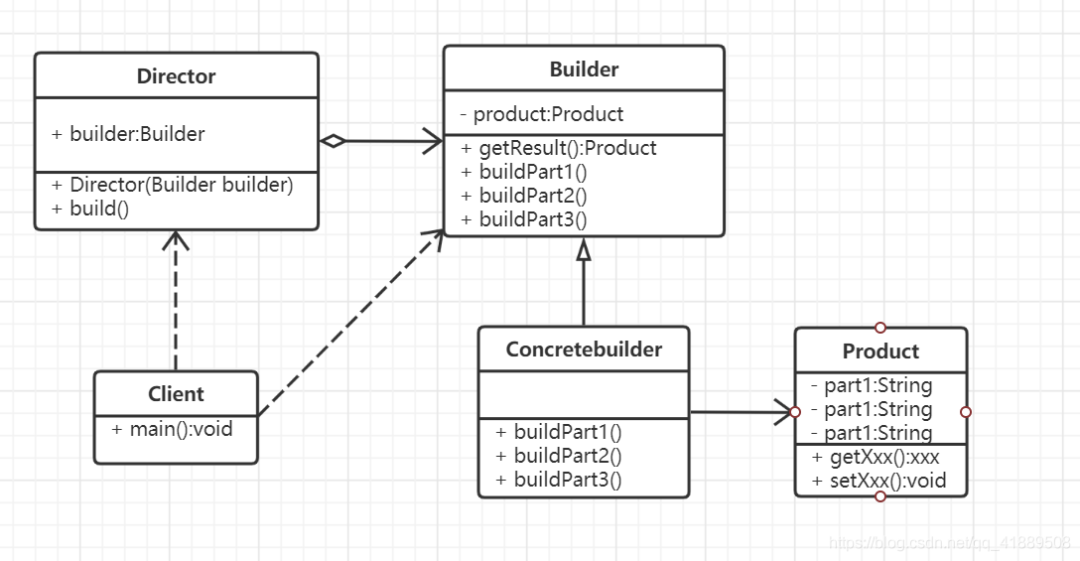
UML Description: Product (Product role): a specific Product object. Builder (Abstract builder): create an abstract interface specified by each part of a Product object. Concrete Builder: implement abstract interfaces, build and assemble various components. Director: build an object that uses the builder interface. It is mainly used to create a complex object. It has two main functions: one is to isolate the production process of customers and objects; the other is to control the production process of Product objects.
3. The code is as follows:
1. Product category:
public class Product { private String part1;//Can be any type private String part2; private String part3; /**set get Method omission }
2. Abstract builder
public abstract class Builder{ Product product = new Product(); public abstract void buildPart1(); public abstract void buildPart2(); public abstract void buildPart3(); public Product getResult(){ return product; }; }
3. Specific builder
public class ConcreteBuilder extends Builder { @Override public void buildPart1() { System.out.println("build part1"); } @Override public void buildPart2() { System.out.println("build part2"); } @Override public void buildPart3() { System.out.println("build part3"); } }
4. Commander:
public class Director { private Builder builder; public Director(Builder builder) { this.builder = builder; } public Product build(){ builder.buildPart1(); builder.buildPart2(); builder.buildPart3(); return builder.getResult(); } }
5. Client
public class Client { @Test public void test() { Builder builder = new ConcreteBuilder(); Director director = new Director(builder); director.build(); } }
6. Implementation results
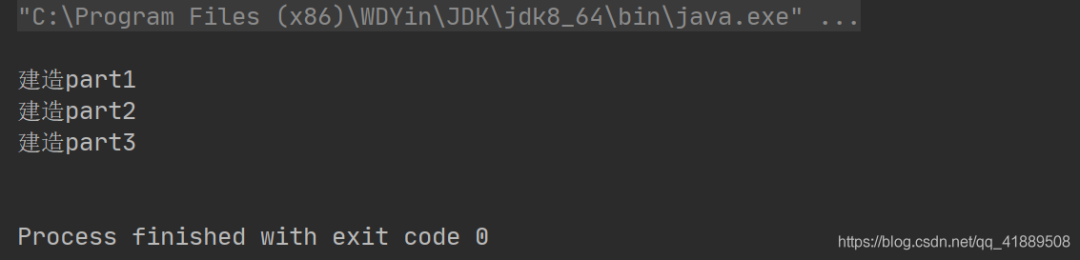
4. Summary
Advantages: 1. The builder is independent and easy to expand. Encapsulating and decomposing the construction process of complex products into different methods makes the creation process very clear and allows us to more accurately control the creation process of complex product objects.
2. Easy to control detail risk. It isolates the creation and use of complex product objects, so that the same creation process can create different products.
Disadvantages: 1. The products must have something in common and the scope is limited.
2. If the internal changes are complex, there will be many construction classes, resulting in a huge system.
Application scenario 1. The object to be generated has a complex internal structure. 2. The internal properties of the object to be generated depend on each other.
5. Application scenarios
StringBuilder in JAVA.
6, Personal experience
Design pattern is a way of thinking and solving problems. Don't copy mechanically. Design pattern is for the sake of design pattern.
PS: reprint, please indicate the source, author: TigerChain
Address: www.jianshu.com/p/300cbb9ee...
This article comes from TigerChain brief book everyone can design patterns
Tutorial introduction
- 1. Reading object this tutorial is suitable for beginners and veterans
- 2. The difficulty of the course is primary, and my level is limited. It is inevitable that there will be problems in the content of the article. If there are any problems, please point them out. Thank you
- 3. Demo address: github.com/githubchen0...
text
1, What is the builder model
1. Builder mode in life
1. Build a house
When we build a house in life, we usually lay the foundation, build the frame "with brick or reinforced concrete", and then paint it. This is basically the way. Of course, we can do all these jobs ourselves, but we can also find a few workers to do them. Of course, we can also directly find a designer, directly say that I want such a house, and then ignore it. Finally, ask the designer "if the designer gives a piece of paper to the workers, the workers will do it" to check and accept the house. "As for how you build the process, I don't care, I just want the results." ---This is the builder model
2. Assemble the computer
The computers we buy are all composed of mainboard, memory, cpu, graphics card, etc. How to assemble these things to users is the role of the builder model. Different people have different requirements for computer configuration. "Those who play games have high requirements for graphics card", but the computer components are fixed, We find the installation personnel of the computer city to install the computer. This process is the construction mode
3. Software development
We need technical directors, product managers and hard-working programmers to develop a product. Here, the product manager is the "Director" who communicates with customers and understands product requirements. The technical Director is an abstract Builder, which allows apes to do miscellaneous work, while the programmer is a manual worker, "that is, the specific Builder does it according to the tasks issued by the technical Director" -- this is a near perfect Builder model "Why do you say close? Because there is no 100%, shit: I forgot to take medicine again."
2. Builder mode in program
Definition of builder mode
Separating the construction of a complex object from its representation, so that the same construction process can create different representations, which is an official definition. In popular terms, the builder mode is how to build an object containing multiple components step by step, and the same construction process can create different products
Characteristics of builder model
The builder mode is a creation mode, which is applicable to those processes that are fixed and "the order is not necessarily fixed", and the target object of construction will change. This kind of scene "such as drawing a dog, the target remains the same, but the difference is that there are yellow dog, fat dog, thin dog, etc." another scene is to replace the multi parameter constructor
Role of builder model
- 1. Without knowing the construction process and details of the object, users can create complex objects "shielding the specific details of construction"
- 2. Users only need to give the content and type of complex objects to create objects
- 3. The builder and modeler create complex objects step by step according to the process
Structure of builder model
role | category | explain |
---|---|---|
Builder | Interface or abstract class | Abstract builders are not necessary |
ConcreateBuilder | Specific builder | There can be multiple "because each construction style may be different" |
Product | Ordinary class | The specific product "is the object to be built" |
Director | The director is also called the conductor | It is not necessary to command the builder to build the target |
Builder pattern simple UML
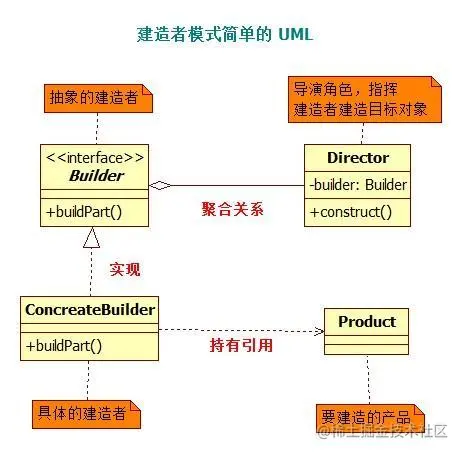
2, Example of builder model
1. Assemble the computer
Xiao Ming wanted to assemble a desktop computer. Xiao Ming knew nothing about computer configuration, so he went directly to the computer city to install it. The boss said I wanted a computer that played games very well. Please install it. You can recommend the configuration to me. So the boss asked its employee "Xiao Mei" According to Xiao Ming's requirements, a computer with grey changniu B was installed. One hour later, the computer was installed. Xiao Ming paid for the computer and left. After a while, Xiao Zhang came again and asked for a computer that could meet the requirements of writing articles at ordinary times. The boss gave different installation configurations according to Xiao Zhang's requirements. Different people have different configuration schemes "but the installation process is the same" , this is a typical builder model
Simple UML for assembling computers
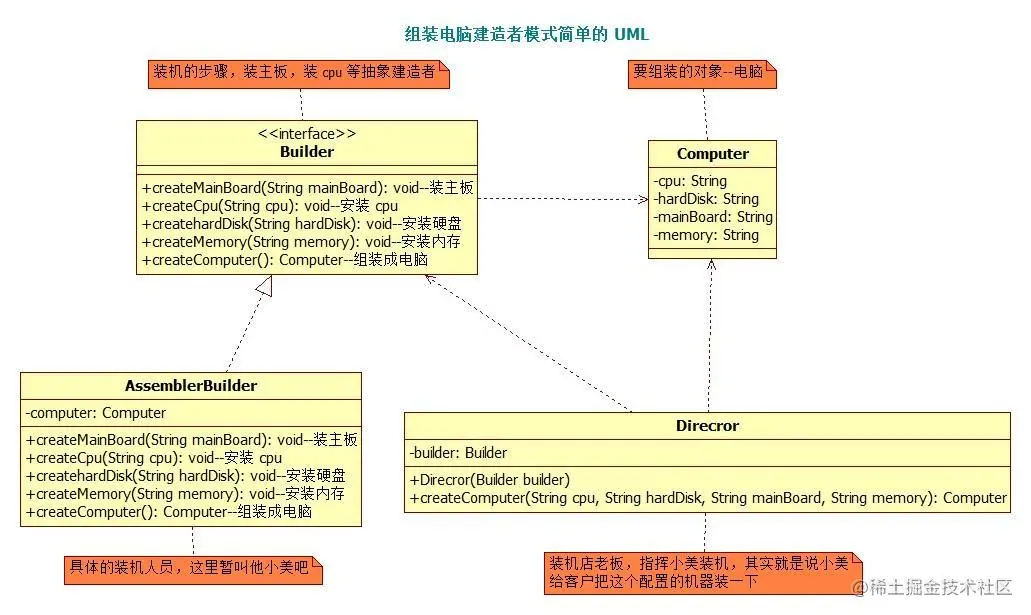
According to UML code
- 1. Create the built object computer --- computer java
/** * Created by TigerChain * Product class -- object to be built */ public class Computer { private String cpu ; // cpu private String hardDisk ; //Hard disk private String mainBoard ; // a main board private String memory ; // Memory ... ellipsis getter and setter } Copy code
- 2. Abstract builder --- builder java
/** * Created by TigerChain * Abstract builder, that is, the steps of installing a computer * As for what type of motherboard to install, I don't care, but the specific builder cares */ public interface Builder { // Install the motherboard void createMainBoard(String mainBoard) ; // Install cpu void createCpu(String cpu) ; // Installing the hard disk void createhardDisk(String hardDisk) ; // Install memory void createMemory(String memory) ; // Make up a computer Computer createComputer() ; } Copy code
- 3. The specific builder, that is, the installer Xiaomei --- assembler builder java
/** * Created by TigerChain * The specific builder, here is an installer of the mall */ public class AssemblerBuilder implements Builder { private Computer computer = new Computer() ; @Override public void createCpu(String cpu) { computer.setCpu(cpu); } @Override public void createhardDisk(String hardDisk) { computer.setHardDisk(hardDisk); } @Override public void createMainBoard(String mainBoard) { computer.setMainBoard(mainBoard); } @Override public void createMemory(String memory) { computer.setMemory(memory); } @Override public Computer createComputer() { return computer; } } Copy code
- 4. There is also the boss "the finger pointing man" who arranges the work of the installer - -- direcror java
/** * Created by TigerChain * Declare a director class "conductor, the boss who can install the computer" to direct the assembly process, that is, the process of assembling the computer */ public class Director { private Builder builder ; // There are a lot of installers. I take care of you, Xiaomei, Xiaolan and piggy. I accept them all public Direcror(Builder builder){ this.builder = builder ; } // Finally, the boss just wants to see the finished product - to be delivered to the customer public Computer createComputer(String cpu,String hardDisk,String mainBoard,String memory){ // The specific work is done by the installer this.builder.createMainBoard(mainBoard); this.builder.createCpu(cpu) ; this.builder.createMemory(memory); this.builder.createhardDisk(hardDisk); return this.builder.createComputer() ; } } Copy code
- 5. Test class
/** * Created by TigerChain * Test class */ public class Test { public static void main(String args[]){ // Installer Xiaomei Builder builder = new AssemblerBuilder() ; // The boss transferred Xiao Ming's demand to Xiao Mei Direcror direcror = new Direcror(builder) ; // The boss finally got the finished machine and Xiaomei did all the work Computer computer = direcror.createComputer("Intel CoRE i9 7900X","Samsung M9T 2TB (HN-M201RAD)","Jijia AORUS Z270X-Gaming 7","Kefu Cras II Red light 16 GB DDR4 3000") ; System.out.println("Xiaoming's computer uses:\n"+computer.getMainBoard()+" a main board\n"+computer.getCpu()+" CPU\n"+computer.getHardDisk()+"Hard disk\n"+computer.getMainBoard()+" Memory\n"); } } Copy code
- 6. Run to view results
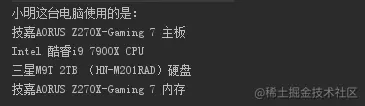
As for Xiao Zhang, piggy needs to install the machine and give the configuration he wants to the boss. Then you don't have to worry about how the boss installs the machine. Just wait and collect the installed machine
2. Build a house
The basic steps and processes of building a house are fixed, which is nothing more than building a foundation, building a frame, and then pouring "as for building a bungalow or a building, that is the specific needs of each customer". Generally speaking, there are three ways to build a house:
- 1. Building a house by yourself "there is no way. Some people are Niu B. they design and do it by themselves. Of course, this is a small house. Let me see it by building a 32 story house by yourself."
- 2. The person who wants to build a house is a contractor. He can find a group of workers and get the house done by himself
- 3. The person who wants to build a house is an ordinary person who can't do anything. Find a designer and say, "I'm going to build a house. It's transparent from north to South and four shows are evergreen". The designer said there's no problem. The designer threw the designed drawings to the contractor and said, "build it like this". The contractor took the drawings to the workers to divide the work and finally complete the work
Simple UML model of House Builder
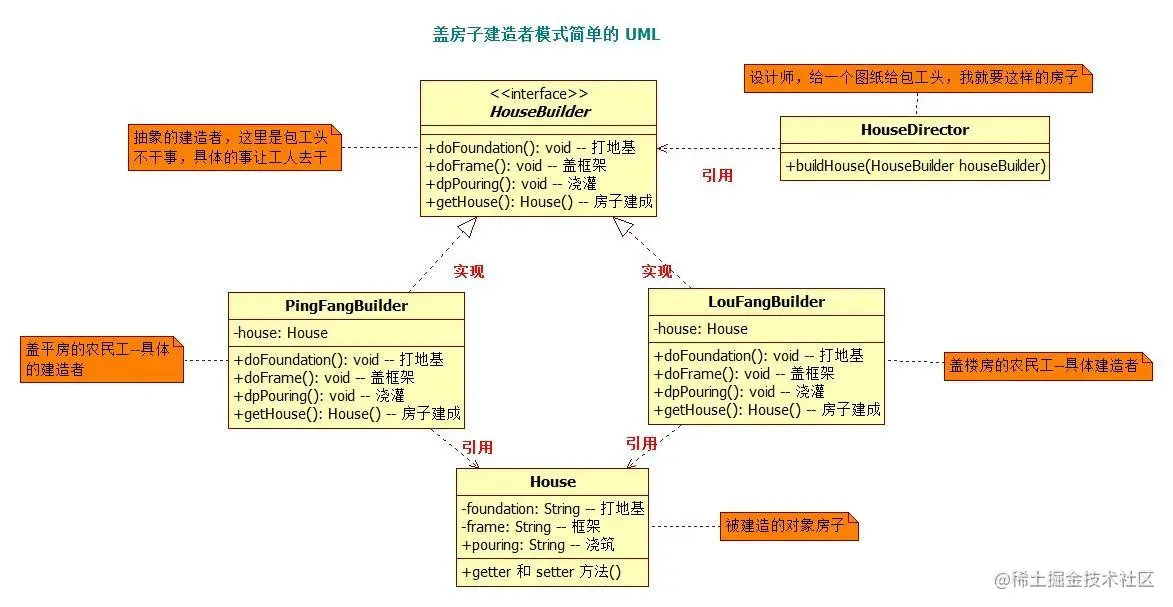
According to UML code
- 1. House object: house java
/** * Created by TigerChain * The final product --- house */ public class House { // Laying foundation private String foundation ; // Cover frame private String frame ; // pouring private String pouring ; ... ellipsis setter and getter } Copy code
- 2. Abstract builder "Contractor" housebuilder java
public interface HouseBuilder { // Laying foundation void doFoundation() ; // Cover frame void doFrame() ; // water void dpPouring() ; // House built House getHouse() ; } Copy code
- 3. The specific builder is "worker" -- pingfangbuilder java
/** * Created by TigerChain * Build a bungalow */ public class PingFangBuilder implements HouseBuilder { private House house = new House() ; @Override public void doFoundation() { house.setFoundation("Foundations for Bungalows"); } @Override public void doFrame() { house.setFrame("Frame of Bungalow"); } @Override public void dpPouring() { house.setPouring("The bungalow does not need watering, but can be directly brushed manually"); } @Override public House getHouse() { return house; } } Copy code
- 4. The specific builder is "worker" -- loufangbuilder java
/** * Created by TigerChain * Building construction */ public class LouFangBuilder implements HouseBuilder { private House house = new House() ; @Override public void doFoundation() { house.setFoundation("The foundation of the building is ten meters deep"); } @Override public void doFrame() { house.setFrame("The frame of the building shall be made of very strong reinforced concrete"); } @Override public void dpPouring() { house.setPouring("Take a tanker for the building and fill the frame with concrete"); } @Override public House getHouse() { return house; } } Copy code
- 5. Conductor "designer" housedirector java
/** * Created by TigerChain * designer */ public class HouseDirector { // Command contractor public void buildHouse(HouseBuilder houseBuilder){ houseBuilder.doFoundation(); houseBuilder.doFrame(); houseBuilder.dpPouring(); } } Copy code
- 6. Test java
/** * Created by TigerChain * test */ public class Test { public static void main(String args[]){ // Method 1: customers build their own houses and do it themselves System.out.println("========Customers who build their own houses must know the details of building houses========"); House house = new House() ; house.setFoundation("Users build their own houses: laying foundations"); house.setFrame("Users build their own houses: build frames"); house.setPouring("Users build their own houses: pouring"); System.out.println(house.getFoundation()); System.out.println(house.getFrame()); System.out.println(house.getPouring()); // Method 2: the customer asks a builder to build a house "as a contractor", but he should know how to build a house "call the builder to build a house in order" System.out.println("========The customer went straight to the House Builder「builder」,To call the builder's method to build a house, the customer must know how to build the house========"); HouseBuilder houseBuilder = new PingFangBuilder() ; houseBuilder.doFoundation(); houseBuilder.doFrame(); houseBuilder.dpPouring(); House house1 = houseBuilder.getHouse() ; System.out.println(house1.getFoundation()); System.out.println(house1.getFrame()); System.out.println(house1.getPouring()); // Method 3: using the builder model, find a designer to "pull a group of builders to work" and tell him what kind of house I want. Finally, the customer only asks the designer for a house System.out.println("========The customer directly looks for a designer. The designer commands the builder to build a house. The house is miscellaneous. The customer doesn't care. Finally, he just asks the designer for a house========"); HouseBuilder pingFangBuilder = new PingFangBuilder() ; HouseDirector houseDirector = new HouseDirector() ; houseDirector.buildHouse(pingFangBuilder); House houseCreateByBuilder = pingFangBuilder.getHouse() ; System.out.println(houseCreateByBuilder.getFoundation()); System.out.println(houseCreateByBuilder.getFrame()); System.out.println(houseCreateByBuilder.getPouring()); } } Copy code
We compared three ways: building a house by ourselves, looking for workers to build a house, and looking for designers to build a house to gradually feel the advantages of the builder model
- 6. Run to view results
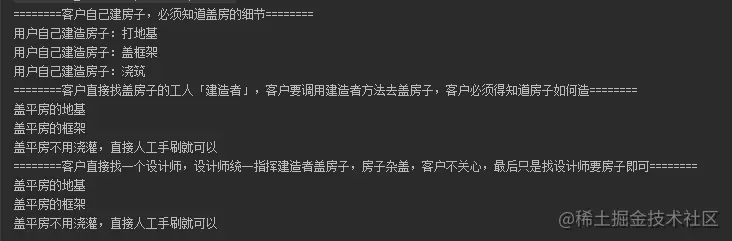
You can see that the last one is the most comfortable. When building a house, you can directly outsource it to the designer, and you don't have to worry about it. At that time, you can ask the designer for the finished house to be built. In this way, I don't need to know how to build a house for customers. Shielding the details "money is capricious"