Time processing in Python is sometimes a big headache. I remember when I first learned python, I was troubled by this for a long time.
This article will explore the time processing function in python with you, so that you can thoroughly understand time processing.
Contents of this article
- Introduction to datetime module 1.1 datetime.date class 1.2 datetime.datetime class 1.3 datetime.timedelta class
- Date to character
- Character to date
- Value to date
- Application of time function in collection data processing 5.1 reading data 5.2 process the collection time into date format 5.3 get the last collection record of the customer
1, Introduction to datetime module

There is a datetime module in python, which defines the following classes:
- datetime.date: class representing date. Common attributes include year, month and day.
- datetime.time: class representing time. Common attributes include hour, minute, second and microsecond.
- datetime.datetime: represents a datetime class.
- datetime.timedelta: represents the time interval class, that is, the length between two time points.
- datetime.tzinfo: class representing time zone.
In order to familiarize you with the above commonly used classes, here are some examples.
1. Datetime.date class
#datetime.date class import datetime print('1.Now it is',datetime.date.today(),'day') print('2.Now it is',datetime.date.today().year,'year') print('3.Now it is',datetime.date.today().month,'month') print('4.Now it is',datetime.date.today().day,'day') #Results obtained: 1.It's 2021-10-31 day 2.It's 2021 3.It's October 4.It's the 31st
2. Datetime.datetime class
#datetime.datetime class import datetime print('1.Now it is',datetime.datetime.today(),'day') print('2.Now it is',datetime.datetime.today().year,'year') print('3.Now it is',datetime.datetime.today().month,'month') print('4.Now it is',datetime.datetime.today().day,'day') print('5.Now it is',datetime.datetime.today().hour,'Time') print('6.Now it is',datetime.datetime.today().minute,'branch') print('7.Now it is',datetime.datetime.today().second,'second') print('8.Now it is',datetime.datetime.today().microsecond,'Microsecond') #Results obtained: 1.It's 2021-10-31 15:55:23.676360 day 2.It's 2021 3.It's October 4.It's the 31st 5.It's 15 o'clock 6.It's 55 7.It's 23 seconds 8.Now it's 677333 microseconds
3 datetime.timedelta class
#datetime.timedelta class import datetime today = datetime.date.today() yestoday = today + datetime.timedelta(days = -1) tomorrow = today + datetime.timedelta(days = 1) print('1.Today is', today,'day') print('2.Yesterday was', yestoday,'day') print('3.Tomorrow is', tomorrow,'day') #Results obtained: 1.Today is 2021-10-31 day 2.Yesterday was 2021-10-30 day 3.Tomorrow is 2021-11-01 day
2, Date to character

This section describes how to convert dates into characters, mainly using the datetime.datetime.strftime function.
#dateTime to str date_time_now = datetime.datetime.now() str_now1 = datetime.datetime.strftime(date_time_now, '%Y-%m-%d') print(date_time_now) str_now1 #Results obtained: 2021-10-31 16:20:45.391792 '2021-10-31'
3, Character to date

This section describes how to convert characters into dates, mainly using the datetime.datetime.strptime function.
#str to date str_time = '2021-10-21' date_time = datetime.datetime.strptime(str_time, '%Y-%m-%d').date() print(date_time) #Results obtained: 2021-10-21
4, Value to date

This section describes how to convert values to dates.
Read the time from excel data sheet. If it is in 2021 / 10 / 11 format, it will be converted to the corresponding value 44480.
At this time, it needs to be converted to the corresponding date. The specific code is as follows:
#num to date from datetime import datetime from xlrd import xldate_as_datetime, xldate_as_tuple num = 44480 datetime(*xldate_as_tuple(num,0)).strftime('%Y-%m-%d') #Results obtained: '2021-10-11'
5, Application of time function in collection data processing

This section applies the functions described in the previous section to deal with the problems encountered in practical work.
For example, there are a number of credit collection data. Because a customer may not be connected, it is possible to dial multiple times.
We want to analyze the real reason why the customer didn't pay back, so we want to take the reason why the customer was overdue recorded by the collector in the last call.
At this time, you need to sort the data set according to the contract number and the time of making a call, and take the overdue reason of the last call.
1 read data
The first is to read the data. The code is as follows:
import pandas as pd from xlrd import open_workbook file_name = r'F:\official account\43.Time function\Reminders-2021-10-11 To 2021-10-17.xlsx' #File name table = open_workbook(file_name) #Open file sheets_name = table.sheet_names() #Get the sheet name in excel get_sheet1 = table.sheet_by_name('Sheet1') #Get Sheet1 data = list() for i in range(get_each_sheet.nrows): col_values = get_sheet1.row_values(i, start_colx=0, end_colx=None) data.append(col_values) data1 = pd.DataFrame(data) #Read out the data in Sheet1 to generate a data frame data1.columns = data1.iloc[0] data1 = data1.drop(index=0) #The data frame column name is determined and the first row is deleted data2 = data1[['Contract No', 'full name', 'Contact type', 'Contact type', 'Collection time', 'Collection content', 'Collection status', 'Commission date']] #Get the fixed column in the data frame
Note: if the data is needed, the time of processing of collection data can be directly returned to the official account, and it can be obtained free of charge.
Results obtained:
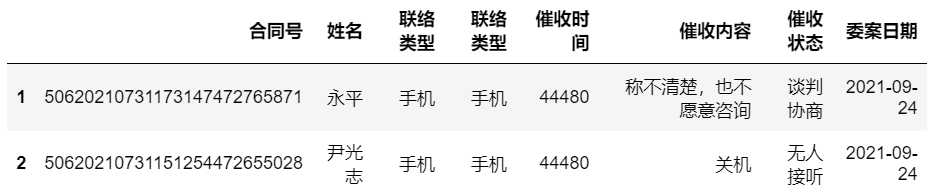
2. Process the collection time into date format
Then, the collection time is processed and changed into date format, with the code as follows:
from datetime import datetime from xlrd import xldate_as_datetime, xldate_as_tuple def num_to_date(num_): return datetime(*xldate_as_tuple(num_,0)).strftime('%Y-%m-%d') data2['Collection time'] = data2['Collection time'].apply(num_to_date) data2.head(2)
Results obtained:

3. Get the last collection record of the customer
Finally, sort the data frame according to the contract number and collection time, and take the last collection record. The code is as follows:
data3 = data2.sort_values(by=['Contract No', 'Collection time'],ascending=[False, False]) data3 = data3.groupby('Contract No',as_index=False).first() print(data2.shape) print(data3.shape)
Results obtained:
(1744, 8) (1455, 8)
So far, the application python processing time has been explained. Interested friends can follow this article to implement it.